You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
A little NodeJS package to convert OpenAPI Schema Object to JSON Schema.
3
+
A little NodeJS package to convert JSON Schema to [OpenAPI Schema Objects](https://swagger.io/specification/#schemaObject).
4
4
5
-
Currently converts from [OpenAPI 3.0](https://github.com/OAI/OpenAPI-Specification/blob/master/versions/3.0.0.md) to [JSON Schema Draft 4](http://json-schema.org/specification-links.html#draft-4).
OpenAPI is a specification for describing RESTful APIs. OpenAPI 3.0 allows us to describe the structures of request and response payloads in a detailed manner. This would, theoretically, mean that we should be able to automatically validate request and response payloads. However, as of writing there aren't many validators around.
10
+
OpenAPI is often described as an extension of JSON Schema, but both specs have changed over time and grown independently. OpenAPI v2 based based on JSON Schema draft 4 with a long list of deviations, but OpenAPI v3 shrank that list, upping their support to draft v4 and making the list of discrepancies shorter. Despite OpenAPI v3 closing the gap, the issue of JSON Schema divergence has not been resolved fully.
10
11
11
-
The good news is that there are many validators for JSON Schema for different languages. The bad news is that OpenAPI 3.0 is not entirely compatible with JSON Schema. The Schema Object of OpenAPI 3.0 is an extended subset of JSON Schema Specification Wright Draft 00 with some differences.
12
+
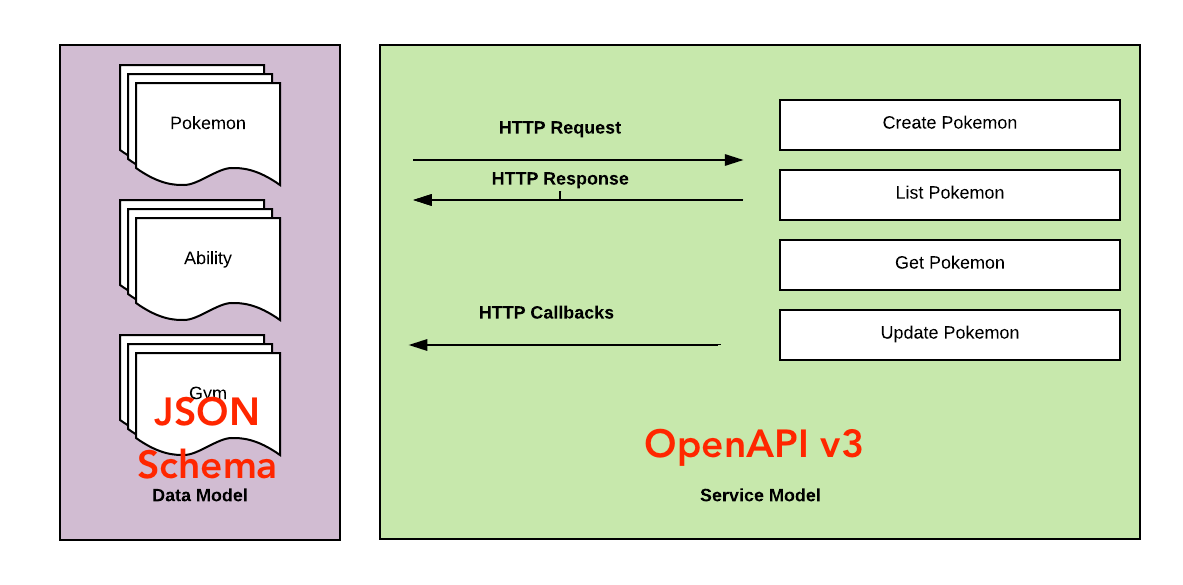
12
13
13
-
The purpose of this project is to fill the grap by doing the conversion between these two formats.
14
+
Carefully writing JSON Schema for your data model kiiiinda works, but it is possible to write JSON Schema that creates invalid OpenAPI, and vice versa. For more on this, read the article [_OpenAPI and JSON Schema Divergence_](https://blog.apisyouwonthate.com/openapi-and-json-schema-divergence-part-1-1daf6678d86e).
15
+
16
+
This tool sets out to allow folks to convert from JSON Schema (their one source of truth for everything) to OpenAPI (a thing for HTML docs and making SDKs).
17
+
18
+
## Converting Back
19
+
20
+
To convert the other way, check out [openapi-schema-to-json-schema](https://github.com/mikunn/openapi-schema-to-json-schema), which this package was based on.
* switches `type: ['foo', 'null']` to `type: foo` and `nullable: true`
21
26
* supports deep structures with nested `allOf`s etc.
22
-
* removes [OpenAPI specific properties](https://github.com/OAI/OpenAPI-Specification/blob/master/versions/3.0.0.md#fixed-fields-20) such as `discriminator`, `deprecated` etc. unless specified otherwise
23
-
* optionally supports `patternProperties` with `x-patternProperties` in the Schema Object
27
+
* switches `patternProperties` to `x-patternProperties` in the Schema Object
24
28
25
29
**NOTE**: `$ref`s are not dereferenced. Use a dereferencer such as [json-schema-ref-parser](https://www.npmjs.com/package/json-schema-ref-parser) prior to using this package.
Provide the function the schema object with possible options.
62
-
63
65
### Options
64
66
65
67
The function accepts `options` object as the second argument.
66
68
67
69
#### `cloneSchema` (boolean)
68
70
69
71
If set to `false`, converts the provided schema in place. If `true`, clones the schema by converting it to JSON and back. The overhead of the cloning is usually negligible. Defaults to `true`.
70
-
71
-
#### `dateToDateTime` (boolean)
72
-
73
-
This is `false` by default and leaves `date` type/format as is. If set to `true`, sets `type: "string"` and `format: "date-time"` if
74
-
*`type: "string"` AND `format: "date"` OR
75
-
*`type: "date"`
76
-
77
-
For example
78
-
79
-
```js
80
-
var schema = {
81
-
type:'date'
82
-
};
83
-
84
-
var convertedSchema =toJsonSchema(schema, {dateToDateTime:true});
By default, the following fields are removed from the result schema: `nullable`, `discriminator`, `readOnly`, `writeOnly`, `xml`, `externalDocs`, `example` and `deprecated` as they are not supported by JSON Schema Draft 4. Provide an array of the ones you want to keep (as strings) and they won't be removed.
102
-
103
-
#### `removeReadOnly` (boolean)
104
-
105
-
If set to `true`, will remove properties set as `readOnly`. If the property is set as `required`, it will be removed from the `required` array as well. The property will be removed even if `readOnly` is set to be kept with `keepNotSupported`.
106
-
107
-
#### `removeWriteOnly` (boolean)
108
-
109
-
Similar to `removeReadOnly`, but for `writeOnly` properties.
110
-
111
-
#### `supportPatternProperties` (boolean)
112
-
113
-
If set to `true` and `x-patternProperties` property is present, change `x-patternProperties` to `patternProperties` and call `patternPropertiesHandler`. If `patternPropertiesHandler` is not defined, call the default handler. See `patternPropertiesHandler` for more information.
114
-
115
-
#### `patternPropertiesHandler` (function)
116
-
117
-
Provide a function to handle pattern properties and set `supportPatternProperties` to take effect. The function takes the schema where `x-patternProperties` is defined on the root level. At this point `x-patternProperties` is changed to `patternProperties`. It must return the modified schema.
118
-
119
-
If the handler is not provided, the default handler is used. If `additionalProperties` is set and is an object, the default handler sets it to false if the `additionaProperties` object has deep equality with a pattern object inside `patternProperties`. This is because we might want to define `additionalProperties` in OpenAPI spec file, but want to validate against a pattern. The pattern would turn out to be useless if `additionalProperties` of the same structure were allowed. Create you own handler to override this functionality.
120
-
121
-
See `test/pattern_properties.js` for examples how this works.
0 commit comments