This repository was archived by the owner on Jun 15, 2023. It is now read-only.
Commit 75b3385
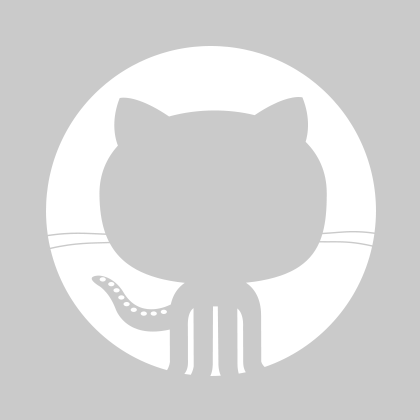
Takuto Ikuta
1 parent 3b32002 commit 75b3385
File tree
5 files changed
+20
-20
lines changed- src
5 files changed
+20
-20
lines changed+3-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
| 22 | + | |
22 | 23 |
| |
23 | 24 |
| |
24 | 25 |
| |
| |||
56 | 57 |
| |
57 | 58 |
| |
58 | 59 |
| |
59 |
| - | |
| 60 | + | |
60 | 61 |
| |
61 | 62 |
| |
62 | 63 |
| |
63 | 64 |
| |
64 | 65 |
| |
65 | 66 |
| |
66 | 67 |
| |
67 |
| - | |
| 68 | + | |
68 | 69 |
| |
69 | 70 |
| |
70 | 71 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
38 | 38 |
| |
39 | 39 |
| |
40 | 40 |
| |
41 |
| - | |
| 41 | + | |
42 | 42 |
| |
43 | 43 |
| |
44 | 44 |
| |
45 |
| - | |
| 45 | + | |
46 | 46 |
| |
47 | 47 |
| |
48 | 48 |
| |
49 | 49 |
| |
50 | 50 |
| |
51 | 51 |
| |
52 | 52 |
| |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
| 53 | + | |
59 | 54 |
| |
60 | 55 |
| |
61 | 56 |
| |
62 | 57 |
| |
63 |
| - | |
64 | 58 |
| |
65 | 59 |
| |
66 | 60 |
| |
| |||
76 | 70 |
| |
77 | 71 |
| |
78 | 72 |
| |
79 |
| - | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
80 | 76 |
| |
81 |
| - | |
| 77 | + | |
82 | 78 |
| |
83 | 79 |
| |
84 | 80 |
| |
| |||
110 | 106 |
| |
111 | 107 |
| |
112 | 108 |
| |
113 |
| - | |
| 109 | + | |
114 | 110 |
| |
115 | 111 |
| |
116 | 112 |
| |
117 |
| - | |
| 113 | + | |
118 | 114 |
| |
119 | 115 |
| |
120 | 116 |
| |
| |||
132 | 128 |
| |
133 | 129 |
| |
134 | 130 |
| |
135 |
| - | |
| 131 | + | |
| 132 | + | |
136 | 133 |
| |
137 | 134 |
| |
138 | 135 |
| |
| |||
172 | 169 |
| |
173 | 170 |
| |
174 | 171 |
| |
175 |
| - | |
| 172 | + | |
176 | 173 |
| |
177 | 174 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
30 | 30 |
| |
31 | 31 |
| |
32 | 32 |
| |
33 |
| - | |
| 33 | + | |
34 | 34 |
| |
35 | 35 |
| |
36 | 36 |
| |
37 | 37 |
| |
38 |
| - | |
| 38 | + | |
39 | 39 |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
471 | 471 |
| |
472 | 472 |
| |
473 | 473 |
| |
474 |
| - | |
| 474 | + | |
475 | 475 |
| |
476 | 476 |
| |
477 | 477 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
70 | 70 |
| |
71 | 71 |
| |
72 | 72 |
| |
| 73 | + | |
| 74 | + | |
73 | 75 |
| |
74 | 76 |
| |
75 | 77 |
| |
|
0 commit comments