diff --git a/LeetCode/Doc/doc-sample.md b/LeetCode/Doc/doc-sample.md
index 03f0a1a..60703dc 100644
--- a/LeetCode/Doc/doc-sample.md
+++ b/LeetCode/Doc/doc-sample.md
@@ -1,4 +1,4 @@
-# candy
+#
知识点:
@@ -13,4 +13,4 @@
## 代码
-[这里](../Code/n.py)
\ No newline at end of file
+[这里](../src/n/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/java\344\270\255\345\270\270\350\247\201\346\225\260\346\215\256\347\273\223\346\236\204\344\275\277\347\224\250.md" "b/LeetCode/Doc/java\344\270\255\345\270\270\350\247\201\346\225\260\346\215\256\347\273\223\346\236\204\344\275\277\347\224\250.md"
new file mode 100644
index 0000000..c9977e3
--- /dev/null
+++ "b/LeetCode/Doc/java\344\270\255\345\270\270\350\247\201\346\225\260\346\215\256\347\273\223\346\236\204\344\275\277\347\224\250.md"
@@ -0,0 +1,29 @@
+# Java中常见数据结构的使用方法
+
+## 队列
+```java
+Queue queue = new LinkedList();
+//添加元素
+queue.offer("a");
+ //返回第一个元素,并在队列中删除
+String first=queue.poll();
+queue.element(); //返回第一个元素
+queue.peek(); //返回第一个元素
+
+```
+## 栈
+
+Stack st = new Stack();
+
+| 序号 | 方法描述 |
+| :--- | :----------------------------------------------------------- |
+| 1 | boolean empty() 测试堆栈是否为空。 |
+| 2 | Object peek( ) 查看堆栈顶部的对象,但不从堆栈中移除它。 |
+| 3 | Object pop( ) 移除堆栈顶部的对象,并作为此函数的值返回该对象。 |
+| 4 | Object push(Object element) 把项压入堆栈顶部。 |
+| 5 | int search(Object element) 返回对象在堆栈中的位置,以 1 为基数。 |
+
+
+
+
+
diff --git "a/LeetCode/Doc/\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\260\217\346\267\261\345\272\246.md" "b/LeetCode/Doc/\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\260\217\346\267\261\345\272\246.md"
new file mode 100644
index 0000000..707a0d7
--- /dev/null
+++ "b/LeetCode/Doc/\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\260\217\346\267\261\345\272\246.md"
@@ -0,0 +1,18 @@
+# 二叉树的最小深度(minimum-depth-of-binary-tree)
+
+知识点:树
+
+
+## 题目描述
+
+Given a binary tree, find its minimum depth.The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.
+
+给定一个二叉树,找出其最小的深度。最小的深度指的是从根节点到距离其最近的叶子节点之间路径中的节点数。
+
+## 解题思路
+
+利用层序遍历的思想,数据结构采用队列,从第一层开始在每一层的最后一个节点入队之后再多入一个null,标记当前层已经结束,这样每当出队的元素为null,则深度就可加一,附加判断当前节点是否是叶子节点即可。
+
+## 代码
+
+[这里](../src/one/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\344\275\277\347\224\250\346\217\222\345\205\245\346\216\222\345\272\217\345\257\271\351\223\276\350\241\250\350\277\233\350\241\214\346\216\222\345\272\217.md" "b/LeetCode/Doc/\344\275\277\347\224\250\346\217\222\345\205\245\346\216\222\345\272\217\345\257\271\351\223\276\350\241\250\350\277\233\350\241\214\346\216\222\345\272\217.md"
new file mode 100644
index 0000000..d51cac8
--- /dev/null
+++ "b/LeetCode/Doc/\344\275\277\347\224\250\346\217\222\345\205\245\346\216\222\345\272\217\345\257\271\351\223\276\350\241\250\350\277\233\350\241\214\346\216\222\345\272\217.md"
@@ -0,0 +1,30 @@
+# insertion-sort-list(使用插入排序对链表进行排序)
+
+知识点:排序,链表
+
+
+## 题目描述
+
+Sort a linked list using insertion sort.
+
+## 解题思路
+
+首先明确什么是数组的插入排序:
+首先将第二个数与第一个数进行对比,如果第二个数比第一个数小,则将第二个数插入到第一个数之前,这样保证前两个数是有序的;
+接下来将第三个数与前两个数对比,发现有比第三个数大的数即将第三个数插入到对应数的前面,这样一次插入可保证前三个数是有序的;
+以此类推,将后面的i个数分别其前面的i-1个数进行对比,并将其插入到第一个比其大的数前面,最后即可完成排序。
+
+链表与数组相比唯一的不同在于链表不能通过下标访问元素,而且链表不能像数组那样通过下标减1的方式访问到上一个元素,针对这种情况我们将上面的算法做出如下改进:
+
+ 使用curNode、nextNode两个指针,curNode初始指向head,nextNode初始指向curNode.next,curNode的意思是从head节点开始到curNode节点之间的所有元素均为有序的,这在初始时时成立的(初始时curNode=head,所以当然成立),nextNode指向的是curNode的下一个元素,我们使用这个nextNode指针的目的是判断已经确定的有序链表[head,curNode]之外的下一个节点与当前有序链表的关系,具体的:
+
+- 如果nextNode.val>=curNode.val,那么说明[head,curNode]+nextNode构成的链表也是有序的,所以应该讲curNode向后移,即curNode=curNode.next,同时nextNode=curNode.next.
+- 如果nextNode.val知识点:树
+
+
+## 题目描述
+
+Given a binary tree, return the postorder traversal of its nodes' values.
+
+For example:
+Given binary tree{1,#,2,3},
+
+
+
+return[1,2,3].
+
+Note: Recursive solution is trivial, could you do it iteratively?
+
+
+给定一个二叉树,返回其先序遍历结果。
+
+提示:递归解决方案是不值一提的,你选择迭代。
+
+
+## 解题思路
+
+首先明确什么是先序遍历:对于二叉树访问顺序为根-左-右,即为先序遍历。
+
+递归方式很简单,这里说一下迭代的思路,考虑如下二叉树:
+
+
+使用栈,入栈顺序为根、左,右。
+
+
+## 代码
+
+[这里](../src/seven/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\207\272\347\216\260\344\270\200\346\254\241\347\232\204\346\225\260.md" "b/LeetCode/Doc/\345\207\272\347\216\260\344\270\200\346\254\241\347\232\204\346\225\260.md"
new file mode 100644
index 0000000..55c92bd
--- /dev/null
+++ "b/LeetCode/Doc/\345\207\272\347\216\260\344\270\200\346\254\241\347\232\204\346\225\260.md"
@@ -0,0 +1,24 @@
+# single-number(出现一次的数)
+
+知识点:复杂度
+
+
+## 题目描述
+Given an array of integers, every element appears twice except for one. Find that single one.
+
+Note:
+Your algorithm should have a linear runtime complexity. Could you implement it without using extra memory?
+
+给定一个数组,除了某一个元素之外的其他元素都出现了两次,找出那个只出现了一次的数。
+
+注意:你的算法应该在线性事件复杂度内完成,不应该使用额外空间。
+
+
+## 解题思路
+
+使用异或操作,由于异或操作有"相同得0,不同得1"的特性,所以讲所有元素顺序异或完之后结果即为那个只出现了一次的数。
+
+
+## 代码
+
+[这里](../src/fourteen/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\207\272\347\216\260\344\270\200\346\254\241\347\232\204\346\225\2602.md" "b/LeetCode/Doc/\345\207\272\347\216\260\344\270\200\346\254\241\347\232\204\346\225\2602.md"
new file mode 100644
index 0000000..a62701f
--- /dev/null
+++ "b/LeetCode/Doc/\345\207\272\347\216\260\344\270\200\346\254\241\347\232\204\346\225\2602.md"
@@ -0,0 +1,26 @@
+# single-number-ii(出现一次的数2)
+
+知识点:复杂度
+
+
+## 题目描述
+Given an array of integers, every element appears three times except for one. Find that single one.
+Note:
+Your algorithm should have a linear runtime complexity. Could you implement it without using extra memory?
+
+给定一个数组,除了某一个元素之外的其他元素都出现了三次,找出那个只出现了一次的数。
+
+注意:你的算法应该在线性事件复杂度内完成,不应该使用额外空间。
+
+## 解题思路
+
+这道题是对single-number的升级,首先回想一下single-number是如何解的,它是用异或运算异或了所有数组中的值最后返回结果,其本质是使用异或计算出所有数中对应bit位只出现了一次的数,如果某一个位有两个数都出现过(两个数一样的情况下),那么那个位就位0,如果某一位只出现过一次,那么那一位就为1。按照这个思路,我们这里只要找出所有数中bit位只出现过1次的就可以,出现过三次的、两次的,都应该是0,那么我们使用三个变量:a ,b,c。
+
+a记录bit位出现过1次1的数值,b记录bit位出现过2次1的数值,c记录bit位出现过3次的位(c=b&a),然后将c中bit值为1的清空为0的就可以了。
+
+
+
+
+## 代码
+
+[这里](../src/fifteen/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\210\244\346\226\255\351\223\276\350\241\250\344\270\255\346\230\257\345\220\246\345\255\230\345\234\250\347\216\257.md" "b/LeetCode/Doc/\345\210\244\346\226\255\351\223\276\350\241\250\344\270\255\346\230\257\345\220\246\345\255\230\345\234\250\347\216\257.md"
new file mode 100644
index 0000000..9ca82a9
--- /dev/null
+++ "b/LeetCode/Doc/\345\210\244\346\226\255\351\223\276\350\241\250\344\270\255\346\230\257\345\220\246\345\255\230\345\234\250\347\216\257.md"
@@ -0,0 +1,25 @@
+# linked-list-cycle(判断链表中是否存在环)
+
+知识点:链表
+
+
+## 题目描述
+
+Given a linked list, determine if it has a cycle in it.
+
+Follow up:
+Can you solve it without using extra space?
+
+给定一个链表,判断其中是否存在环。
+
+额外要求:
+尽量不要使用额外空间
+
+
+## 解题思路
+
+使用快慢指针即可。
+
+## 代码
+
+[这里](../src/ten/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\212\240\346\262\271\347\253\231\351\227\256\351\242\230.md" "b/LeetCode/Doc/\345\212\240\346\262\271\347\253\231\351\227\256\351\242\230.md"
new file mode 100644
index 0000000..ae0cb47
--- /dev/null
+++ "b/LeetCode/Doc/\345\212\240\346\262\271\347\253\231\351\227\256\351\242\230.md"
@@ -0,0 +1,90 @@
+# 加油站问题(gas-station)
+
+知识点:贪心
+## 题目描述
+
+There are N gas stations along a circular route, where the amount of gas at station i is gas[i].
+
+You have a car with an unlimited gas tank and it costs cost[i] of gas to travel from station i to its next station (i+1). You begin the journey with an empty tank at one of the gas stations.
+
+Return the starting gas station's index if you can travel around the circuit once, otherwise return -1.
+
+Note:
+The solution is guaranteed to be unique.
+
+在一条循环路线上有N个加油站,索引为i的加油站具有的油量为gas[i]。
+你有一辆具有不限容量油箱的小汽车,并且这辆车从第i个加油站到第i+1个加油站耗费的油量是cost[i],初始的时候你的小汽车的油箱里面没有油,你需要寻找一个站点作为起点。
+如果从某个起点出发可以保证你开着你的车绕着赛道走一圈,则返回起点的加油站下标,否则返回-1
+返回起点加油站的下标。
+
+比如下图:
+
+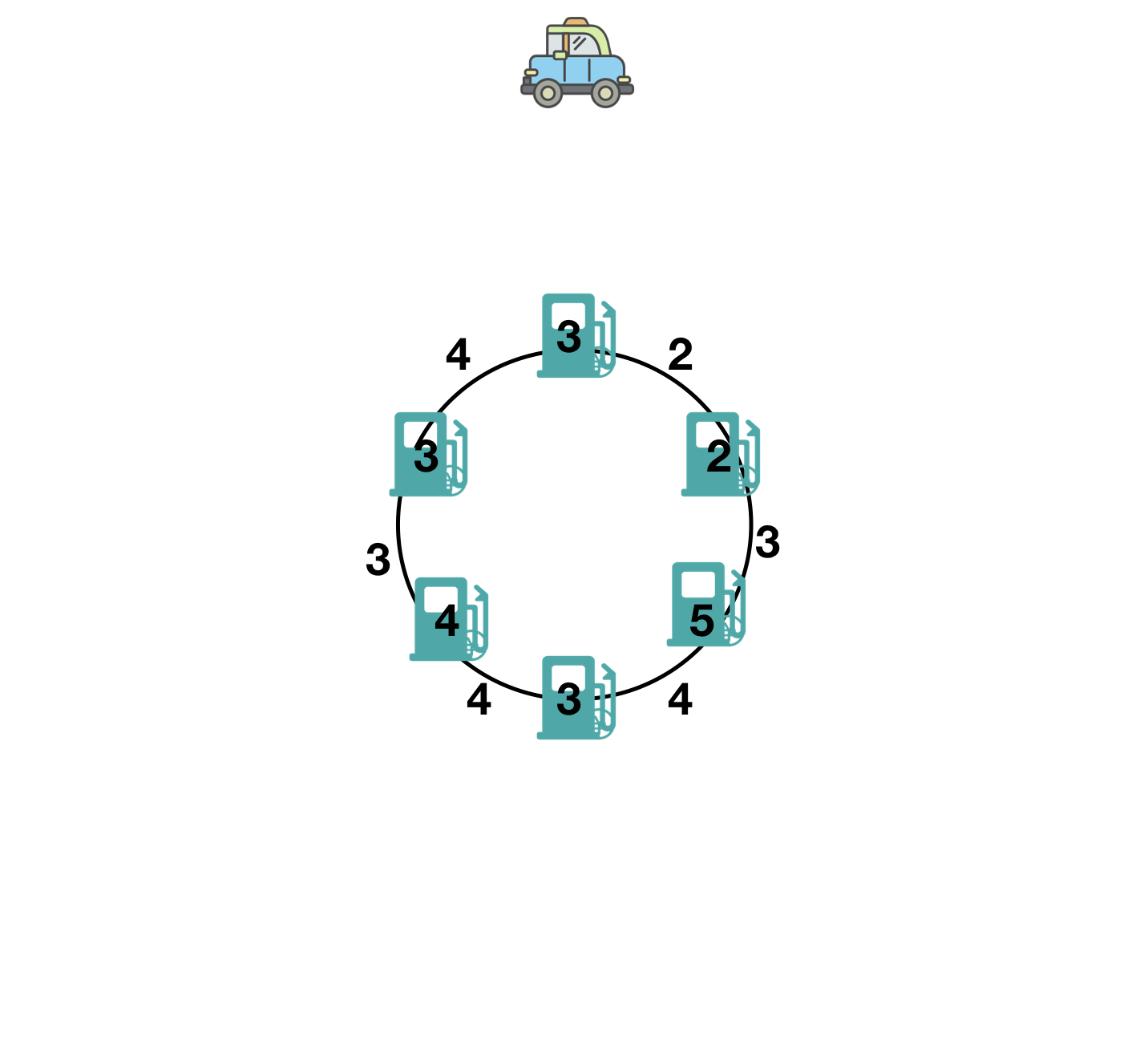
+
+加油站上的数字代表该加油站具有的油量,圆圈上的数字代表该路段需要耗费的油量,那么小车该从哪个加油站出发才能保证顺利走一圈呢?
+
+注意:给出的实例可以保证解决方案是唯一的。
+
+## 解题思路
+
+简单地想,我们当然是想从第1个加油站出发,到达下一个加油站时车里剩余的油尽可能多,这样车里剩下的油还可以继续后面的路程,所以,采取贪心的思路,首先计算从每一站出发,到达下一站后车里还能剩余的油,然后按照剩余油的多少进行排序,从可以剩余油最多的站出发,进行模拟行程,看最后可否走一圈,如果不可以,则换下一个起点。
+
+
+## 代码
+核心代码:
+```java
+public int canCompleteCircuit(int[] gas, int[] cost) {
+
+ //remaining为二维数组,remaing[index][0]代表车从第index出发到达index+1站后里面可以剩余的油,
+ // remaing[index][1]=index,因为后面要对remaing按照remaing[index][0]即剩余的油量排序,
+ // 所以要把原始的索引记录下来,排完序后直接从remaing[index][0]最大的值对应的remain[index][1]处开始开车即可
+ int[][] remaining = new int[gas.length][2];
+
+ //gas[i]---->gas[i+1] cost[i],计算remaining
+ for (int index = 0; index < gas.length; index++) {
+ remaining[index][0] = gas[index] - cost[index];
+ remaining[index][1] = index;
+ }
+
+ //按照remaining[index][0]对remaing进行排序
+ QuickSort(remaining, gas.length);
+
+ //由于上面排完序是降序,所以这里从后往前遍历
+ for (int index = gas.length - 1; index >= 0; index--) {
+ //如果剩余的油大于等于0,说明油足够到达下一站
+ if (remaining[index][0] >= 0) {
+ if (helper(gas, cost, remaining[index][1])) {
+ return remaining[index][1];
+ }
+ } else {
+ return -1;
+ }
+ }
+
+
+ return -1;
+ }
+
+ //模拟从第startIndex站出发,看能不能走完全程
+ boolean helper(int[] gas, int[] cost, int startIndex) {
+ int count = 0;
+ int curGas = 0;
+ for (int index = startIndex; count <= gas.length; count++, index = (index + 1) % gas.length) {
+ curGas += gas[index];
+ if (curGas >= cost[index]) {
+ curGas -= cost[index];
+ } else {
+ return false;
+ }
+ }
+
+ return true;
+ }
+
+```
+
+可以求得上例中的答案为0,即从第0号加油站出发,就像下面这样:
+
+
+
+[完整代码](../src/seventeen/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\215\225\350\257\215\345\210\207\345\210\206.md" "b/LeetCode/Doc/\345\215\225\350\257\215\345\210\207\345\210\206.md"
new file mode 100644
index 0000000..21d60e8
--- /dev/null
+++ "b/LeetCode/Doc/\345\215\225\350\257\215\345\210\207\345\210\206.md"
@@ -0,0 +1,42 @@
+# word-break(单词切分)
+
+知识点:动态规划
+
+
+## 题目描述
+
+Given a string s and a dictionary of words dict, determine if s can be segmented into a space-separated sequence of one or more dictionary words.
+
+For example, given
+s ="leetcode",
+dict =["leet", "code"].
+
+Return true because"leetcode"can be segmented as"leet code".
+
+-----
+
+给定一个字符串s和一个词典,判断s是否可以被切割成一个或者多个词典中的单词。
+
+比如,给定s="leetcode",dict=["leet","code"],应该返回true,因为"leetcode"可以被切分成"leet"和"code"两个片段。
+
+## 解题思路
+
+使用动态规划,假设$f(j)$的真值表示$s[0,j]$是否可以被分词,则:
+$f(n)=f(i)\&\&f(i+1,n)$
+那么如果我们要求$f(n)$是否可以被分词,我们首先需要求出$f(i)$,然后再对$s[i+1,n]$的字符串判断是否可以分词,要求$f(i)$,又要让$j$从0开始到$i-1$,判断$f(j)$为真且$f(j,i)$为真…
+
+伪代码如下:
+
+```
+假设s的长度为length,使用array[length+1]代表f(i)到f(n)的真值,则:
+初始array[0]=true:
+for i in 0 to length:
+ for j in 0 to i:
+ if array[j]&&dict.contains(s[j:i]):
+ array[i]=true;
+return array[length]
+```
+
+## 代码
+
+[这里](../src/eleven/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\215\225\350\257\215\345\210\207\345\210\2062.md" "b/LeetCode/Doc/\345\215\225\350\257\215\345\210\207\345\210\2062.md"
new file mode 100644
index 0000000..a92178a
--- /dev/null
+++ "b/LeetCode/Doc/\345\215\225\350\257\215\345\210\207\345\210\2062.md"
@@ -0,0 +1,62 @@
+# word-break-ii(单词切分2)
+
+知识点:动态规划
+
+
+## 题目描述
+Given a string s and a dictionary of words dict, add spaces in s to construct a sentence where each word is a valid dictionary word.
+
+Return all such possible sentences.
+
+For example, given
+s ="catsanddog",
+dict =["cat", "cats", "and", "sand", "dog"].
+
+A solution is["cats and dog", "cat sand dog"].
+
+-----
+
+给定字符串s和单词字典dict,在s中添加空格以构造一个句子,其中每个单词都是有效的字典单词。
+
+返回所有这些可能的句子。
+
+比如:
+s ="catsanddog",
+dict =["cat", "cats", "and", "sand", "dog"].
+
+应该返回["cats and dog", "cat sand dog"].
+
+## 解题思路
+
+我们从某一个位置`index`将`s`切分为前后两个部分,分别记为`s1`和`s2`,如果`s1`在`dict`中,我们去计算`s2`计算的结果,然后将`s2`切分的结果与`s1`合并即可,如果`s2`不能切割,我们就从`index+1`继续对`s`进行切割,实际是一种`dfs`的思想,核心思想如下:
+
+```java
+ArrayList wordBreak(String s, Set dict){
+ ArrayList res;
+ for(int index=1;index list=wordBreak(s[index:],dict);
+ if(list==null){
+ continue;
+ }else{
+ res+=merge(s[0:index],list);
+ }
+ }
+ }
+ return res;
+}
+```
+
+这样虽然可以解决问题,但是有点小瑕疵,考虑:`s="abcdefg"`,`dict={"bc","def","g"}`,那么:
+
+- index=1 s1=a s2=bcdefg ,切割bcdefg
+- Index=2 s1=ab s2=cdefg,切割cdefg
+- index=3 s1=abc s2=defg,切割defg
+- ...
+
+注意,第一步切割`bcdefg`的时候递归调用了`wordBreak`,在切割`bcdefg`的时候也会切割`cdefg`、`defg`、`efg`、`fg`、`g`,然后下面几步也是类似的操作,所以说下面几步实际上做了一部分重复的工作,为了避免这种重复的工作,我们可以使用`Map`,将`s`与其切分的结果存下来,这样下次再遇到`s`的时候直接从`map`中取之前分好的结果就行了,不用做重复操作。
+
+
+## 代码
+
+[这里](../src/twelve/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\220\214\344\270\200\346\235\241\347\233\264\347\272\277\344\270\212\347\232\204\346\234\200\345\244\232\347\232\204\347\202\271\347\232\204\346\225\260\351\207\217.md" "b/LeetCode/Doc/\345\220\214\344\270\200\346\235\241\347\233\264\347\272\277\344\270\212\347\232\204\346\234\200\345\244\232\347\232\204\347\202\271\347\232\204\346\225\260\351\207\217.md"
new file mode 100644
index 0000000..c234c5c
--- /dev/null
+++ "b/LeetCode/Doc/\345\220\214\344\270\200\346\235\241\347\233\264\347\272\277\344\270\212\347\232\204\346\234\200\345\244\232\347\232\204\347\202\271\347\232\204\346\225\260\351\207\217.md"
@@ -0,0 +1,123 @@
+# max-points-on-a-line(同一条直线上的最多的点的数量)
+
+知识点:穷举
+
+
+## 题目描述
+Given n points on a 2D plane, find the maximum number of points that lie on the same straight line.
+
+
+给定2D平面上的n个点,找到位于同一直线上的最大点的数量。
+
+
+## 解题思路
+
+首先明确如何量化"直线"这一概念,最简单的,我们可以固定一个起始点S(Start),然后遍历剩余的所有点,记做E(End),如果有两个E(记做E1和E2),满足$(E1.x-S.x)/(E1.y-S.y)=(E2.x-S.x)/(E2.y-S.y)$,那么就可以确定E2在S与E1确定的直线上,而$(E1.x-S.x)/(E1.y-S.y)$刚好表示的是以E1和S构成的直线的斜率。所以我们就可以得出结论:要确定三个点S、E1、E2是否在同一条直线上即E2在S、E1构成的唯一直线上,只要满足S-E1直线的斜率与S-E2直线的斜率相等即可。
+
+明确了如何判断三个点是否在一条直线之后我们再回过头来看这道题,n个点,找出点数最多的直线上的点的数量。注意,题目中没有说n个点两两都不相同,所以可能出现多个点的x、y相等,这时候应该多计算一个点(重复的点也是点)。我们可以使用两个循环,第一个循环从第一个点开始遍历所有点作为起始点S,第二个循环从起始点的下一个点遍历后面所有的点作为重点E,构建Map存储当前S对应的所有斜率下的最大点值,逻辑如下:
+
+```
+maxCount=0;
+for indexS from 0 to Length:
+ Map<斜率,点数量> map
+ //以indexS对应点为起点的所有直线中点数量的最大值
+ tempMaxCount=1
+ //与当前indexS对应点重合的点的数量
+ commonCount=0
+ for indexE from indexS+1 to Length:
+ if point[indexS]==point[indexE]:
+ //如果两个点重合
+ commonCount+=1
+ else :
+ 斜率=求斜率的函数(point[indexS],point[indexE])
+ //如果当前斜率之前已经被加入Map中,则更新最大点数为原来的点数+1
+ if(map.containsKey(斜率)){
+ tempMaxCount=max(tempMaxCount,map.get(斜率)+1)
+ map.put(斜率,map.get(斜率)+1)
+ }else{
+ tempMaxCount=2
+ map.put(斜率,2)
+ }
+ maxCount=max(maxCount,tempMaxCount+commonCount)
+return maxCount
+
+```
+
+以上就是完整的逻辑,有几个细节需要注意:
+
+- 斜率的精度
+
+ > 不管使用float还是double都会涉及斜率精度取舍的问题,所以这里建议使用字符串保存精度,方法是将两个点x距离和y距离的比值化为最简分数,然后转化为字符串表示
+
+- x距离或y距离为0
+
+ > 由于存在两个点在同一条横线或同一条竖线的可能,这是如果还是和化普通分数那样就不可取,比如0/4和0/9化为字符串后不一样,但是其表示的值是一样的,所以这种情况应该单独考虑
+
+完整逻辑请看详细代码。
+
+```java
+public int maxPoints(Point[] points) {
+
+ int length = points.length;
+ int maxPointCount = 0;
+ for (int startIndex = 0; startIndex < length; startIndex++) {
+ Map maxPointMap = new HashMap<>();
+ int commonPointCount = 0;
+ int tempMaxCount = 1;
+ for (int endIndex = startIndex + 1; endIndex < length; endIndex++) {
+ int distanceX = points[startIndex].x - points[endIndex].x;
+ int distanceY = points[startIndex].y - points[endIndex].y;
+
+
+ if (distanceX == 0 && distanceY == 0) {
+ commonPointCount += 1;
+ } else {
+ String curStrDis;
+ if (distanceX == 0) {
+ curStrDis = "distanceX";
+ } else if (distanceY == 0) {
+ curStrDis = "distanceY";
+ } else {
+ int maxCommonDivisor = commonDivisor(distanceX, distanceY);
+ if (maxCommonDivisor != 0) {
+ distanceX = distanceX / maxCommonDivisor;
+ distanceY = distanceY / maxCommonDivisor;
+ }
+
+ int flag = 0;
+
+ if (distanceX < 0) {
+ distanceX = -distanceX;
+ flag++;
+ }
+ if (distanceY < 0) {
+ distanceY = -distanceY;
+ flag++;
+ }
+
+ curStrDis = String.valueOf(distanceX) + distanceY;
+ if (flag == 1) {
+ curStrDis = "-" + curStrDis;
+ }
+ }
+
+ if (maxPointMap.containsKey(curStrDis)) {
+ tempMaxCount = Math.max(tempMaxCount, maxPointMap.get(curStrDis) + 1);
+ maxPointMap.put(curStrDis, maxPointMap.get(curStrDis) + 1);
+ } else {
+ //初始时put进去2,一个点是起始点,另一个点是当前点
+ maxPointMap.put(curStrDis, 2);
+ tempMaxCount = Math.max(tempMaxCount, 2);
+ }
+ }
+ }
+ maxPointCount = Math.max(maxPointCount, tempMaxCount + commonPointCount);
+ }
+
+ return maxPointCount;
+ }
+```
+
+## 代码
+
+[这里](../src/three/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\220\216\345\272\217\351\201\215\345\216\206\344\272\214\345\217\211\346\240\221.md" "b/LeetCode/Doc/\345\220\216\345\272\217\351\201\215\345\216\206\344\272\214\345\217\211\346\240\221.md"
new file mode 100644
index 0000000..3f7cca5
--- /dev/null
+++ "b/LeetCode/Doc/\345\220\216\345\272\217\351\201\215\345\216\206\344\272\214\345\217\211\346\240\221.md"
@@ -0,0 +1,59 @@
+# binary-tree-postorder-traversal(后序遍历二叉树)
+
+知识点:树
+
+
+## 题目描述
+
+Given a binary tree, return the postorder traversal of its nodes' values.
+
+For example:
+Given binary tree{1,#,2,3},
+
+
+
+return[3,2,1].
+
+**Note:** Recursive solution is trivial, could you do it iteratively?
+
+给定一个二叉树,返回其后序遍历结果。
+
+提示:递归解决方案是不值一提的,你选择迭代。
+
+
+## 解题思路
+
+首先明确什么是后序遍历:对于二叉树访问顺序为左-右-根,即为后序遍历。
+
+递归方式很简单,这里说一下迭代的思路,考虑如下二叉树:
+
+
+
+使用栈来解决非递归的后序遍历实现,重点在于以何种顺序入栈,由于是出栈的时候去遍历,所以按照后序遍历的顺序推导出入栈顺序应该是curNode、curNode.right、curNode.left,这样出栈的时候就能保证按照后序遍历的顺序了,另外需要使用一个preNode保存前一个遍历的节点,这样就能确定前一个被遍历的节点是否是当前节点的左/右子节点,如果是,说明其儿子已经被遍历了,这时就可以遍历自己了,否则不能遍历当前节点,需要先将当前节点的子节点入栈,最后的逻辑是:
+
+```
+初始:curNode=null,preNode=null,stack=new Stack(),stack.push(root)
+while(stack不为空):
+ if curNode==null:
+ curNode=stack.peek()
+ if curNode 是叶子节点:
+ print curNode.val
+ preNode=curNode
+ stack.pop()
+ curNode=null
+ else if preNode是curNode的直接子节点:
+ print curNode.val
+ preNode=curNode
+ stack.pop()
+ curNode=null
+ else:
+ stack.push(curNode.right)
+ stack.push(curNode.left)
+ curNode=curNode.left
+```
+
+
+
+## 代码
+
+[这里](../src/six/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\345\233\276\347\232\204\345\244\215\345\210\266.md" "b/LeetCode/Doc/\345\233\276\347\232\204\345\244\215\345\210\266.md"
new file mode 100644
index 0000000..6fcca10
--- /dev/null
+++ "b/LeetCode/Doc/\345\233\276\347\232\204\345\244\215\345\210\266.md"
@@ -0,0 +1,44 @@
+# clone-graph(图的复制)
+
+知识点:图
+
+## 题目描述
+Clone an undirected graph. Each node in the graph contains a label and a list of its neighbors.
+
+
+OJ's undirected graph serialization:
+Nodes are labeled uniquely.
+
+We use # as a separator for each node, and,as a separator for node label and each neighbor of the node.
+
+As an example, consider the serialized graph{0,1,2# 1,2# 2,2}.
+
+The graph has a total of three nodes, and therefore contains three parts as separated by#.
+
+First node is labeled as 0. Connect node 0 to both node s1 and 2.
+Second node is labeled as 1. Connect node 1 to node 2.
+Third node is labeled as 2. Connect node 2 to node2(itself), thus forming a self-cycle.
+
+Visually, the graph looks like the following:
+
+复制一个无向图,图中的每一个节点都有一个标签,以及其若干个邻居节点。
+OJ的无向图序列:
+节点被唯一标志
+我们使用#作为每一个节点的分隔符以及节点和其邻居节点的分隔符
+比如,考虑一个{0,1,2# 1,2# 2,2}序列
+该图一共有3个节点,被#分隔为3个部分
+第一个节点的标志是0,连接节点1和节点2
+第二个节点是1,连接节点1和节点2
+第三个节点是2,连接节点2和节点2(自己),即形成了一个自环
+将上图可视化出来就是这样的:
+
+
+
+
+## 解题思路
+
+
+
+## 代码
+
+[这里](../src/n/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\346\211\276\345\207\272\351\223\276\350\241\250\344\270\255\347\216\257\347\232\204\345\205\245\345\217\243\350\212\202\347\202\271.md" "b/LeetCode/Doc/\346\211\276\345\207\272\351\223\276\350\241\250\344\270\255\347\216\257\347\232\204\345\205\245\345\217\243\350\212\202\347\202\271.md"
new file mode 100644
index 0000000..237a744
--- /dev/null
+++ "b/LeetCode/Doc/\346\211\276\345\207\272\351\223\276\350\241\250\344\270\255\347\216\257\347\232\204\345\205\245\345\217\243\350\212\202\347\202\271.md"
@@ -0,0 +1,41 @@
+# linked-list-cycle-ii(找出链表中环的入口节点)
+
+知识点:链表
+
+
+## 题目描述
+Given a linked list, return the node where the cycle begins. If there is no cycle, returnnull.
+
+Follow up:
+Can you solve it without using extra space?
+
+
+给定一个链表,返回其中环的入口节点,如果没有环,返回null
+提示:尽量不要使用额外空间。
+
+
+## 解题思路
+
+参考:https://www.nowcoder.com/questionTerminal/6e630519bf86480296d0f1c868d425ad
+
+思路:
+
+ 1)使用快慢指针方法,判定是否存在环,并记录两指针相遇位置(Z);
+
+ 2)将两指针分别放在链表头(X)和相遇位置(Z),并改为相同速度推进,则两指针在环开始位置相遇(Y)。
+
+ 证明如下:
+
+ 如下图所示,X,Y,Z分别为链表起始位置,环开始位置和两指针相遇位置,则根据快指针速度为慢指针速度的两倍,当两指针相遇时快指针一定比慢指针多走了n圈,可以得出:
+
+ $2*(a + b) = a + b + n * (b + c)$;
+
+即 $a=(n - 1) * b + n * c = (n - 1)(b + c) +c$;
+
+
+
+ 注意到b+c恰好为环的长度,这时如果可以再让快指针走c步(只要是整数圈多c部即可)即可找出入口点了,所以我们再用两个指针,第一个指针p1指向起点X,第二个指针p2指向刚才相遇的点Z,然后让p1和p2一次走一步,当p1走了a步也就是$(n - 1)(b + c) +c$步时,p2也走了$(n - 1)(b + c) +c$步,注意p2起点距离圆的入口点多了b步,那么此时p2应该相对于圆的入口点走了$(n-1)(b+c)+c+b=n(b+c)$步,也就是到了圆的入口点,而p1走了a步自然也到了圆的入口点,所以此时p1与p2会相遇,那么反向推倒,当p1与p2相遇时刚好就在圆的入口点。
+
+## 代码
+
+[这里](../src/nine/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\346\213\267\350\264\235\345\205\267\346\234\211\351\232\217\346\234\272\346\214\207\351\222\210\347\232\204\351\223\276\350\241\250.md" "b/LeetCode/Doc/\346\213\267\350\264\235\345\205\267\346\234\211\351\232\217\346\234\272\346\214\207\351\222\210\347\232\204\351\223\276\350\241\250.md"
new file mode 100644
index 0000000..ca23577
--- /dev/null
+++ "b/LeetCode/Doc/\346\213\267\350\264\235\345\205\267\346\234\211\351\232\217\346\234\272\346\214\207\351\222\210\347\232\204\351\223\276\350\241\250.md"
@@ -0,0 +1,60 @@
+# copy-list-with-random-pointer(拷贝具有随机指针的链表)
+
+知识点:链表
+
+
+## 题目描述
+
+A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.
+
+Return a deep copy of the list.
+
+
+给出链表,每个节点包含一个额外的随机指针,该指针可以指向列表中的任何节点或为空。
+
+返回列表的深拷贝。
+
+
+## 解题思路
+
+
+
+上图为原始链表,黑色箭头代表next指针,绿色箭头代表random指针。
+
+
+
+第一步,复制每一个节点并将其插入到原来的链表中:
+
+
+
+红色的节点即为复制的节点
+
+第二步,根据原来节点的random指针更新复制的节点的random:
+
+```python
+currentNode = pHead
+ while currentNode is not None:
+ node = currentNode.next
+ if currentNode.random is not None:
+ node.random = currentNode.random.next
+ currentNode = node.next
+```
+
+第三步:分离出复制的节点们构成复制的链表:
+
+```python
+ cloneP = pHead.next
+
+ while currentNode.next is not None:
+ temp = currentNode.next
+ currentNode.next = temp.next
+ currentNode = temp
+```
+
+
+
+
+
+## 代码
+
+[这里](../src/thirteen/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\346\216\222\345\272\217List.md" "b/LeetCode/Doc/\346\216\222\345\272\217List.md"
new file mode 100644
index 0000000..6ff723a
--- /dev/null
+++ "b/LeetCode/Doc/\346\216\222\345\272\217List.md"
@@ -0,0 +1,59 @@
+# sort-list(排序List)
+
+知识点:排序,链表
+
+
+## 题目描述
+
+Sort a linked list in O(n log n) time using constant space complexity.
+
+使用常量空间在O(n log n)时间复杂度内对链表进行排序。
+
+## 解题思路
+
+首先思考常见排序算法的事件复杂度及空间复杂度(参考:[八大常见排序算法介绍](https://ai-exception.blog.csdn.net/article/details/79053814)):
+
+| 排序方法 | 时间复杂度 | 空间复杂度 |
+| ------------ | ------------------------------------- | ---------------- |
+| 直接插入排序 | $T(n)=O(n^2)$ | $S(n)=O(1)$ |
+| 希尔排序 | $T(n)=O(n^{1.5})$ | $S(n)=O(1)$ |
+| 冒泡排序 | $T(n)=O(n^2)$ | $S(n)=O(1)$ |
+| 快速排序 | $T(n)=O(nlog_2n)$ | $S(n)=O(log_2n)$ |
+| 选择排序 | $S(n)=O(1)$ | $T(n)=O(n^2)$ |
+| 堆排序 | $T(n)=O(nlog_2n)$ | $S(n)=O(1)$ |
+| 归并排序 | $T(n)=O(nlog_2n)$ | $S(n)=O(n)$ |
+| 基数排序 | $T(n)=O(d*n)$ d为排序数中最大数的位数 | $S(n)=O(n)$ |
+
+从上表中可以看出,对数组而言,时间复杂度为$O(nlogn)$的排序算法有:快速排序、堆排序、归并排序。
+
+快速排序和堆排序都对数组中下标的使用较为依赖,相比之下,归并排序更适合链表,再考虑到数组中归并排序的空间复杂度之所以为$O(n)$是因为合并的时候需要用一个辅助数组记录合并后的值以保证原数组中的数字排序不被破坏,而链表可以使用指针保证这一点而不需要额外辅助数组,所以空间复杂度会降为$O(1)$.
+
+综上,使用归并排序可以很好地解决本问题。
+
+大致逻辑:
+
+```
+fun listSort(head):
+ centerNode=head链表的中间节点
+ //分而治之的思想,分别排序后一半链表和前一半链表
+ left=listSort(centerNode.next)
+ //将centerNode的next置位null,保证同样是head为头结点,现在的链表长度为原来的一半
+ right=centerNode.next=null
+ listSort(head)
+ //合并两个子链表
+ return merge(left,right)
+```
+
+这里涉及到找链表中间节点的问题,方法是使用两个指针p、q,初始时p指向head,q指向head.next,当q不为null时:
+
+```
+p=p.next
+q=q.next.next
+```
+
+利用两个指针行走速度快慢的不同最终实现找出链表的中间节点,注意处理好空指针异常即可,这个算法称为快慢指针算法。
+
+
+## 代码
+
+[这里](../src/four/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\347\263\226\346\236\234\351\227\256\351\242\230.md" "b/LeetCode/Doc/\347\263\226\346\236\234\351\227\256\351\242\230.md"
new file mode 100644
index 0000000..1fbc76f
--- /dev/null
+++ "b/LeetCode/Doc/\347\263\226\346\236\234\351\227\256\351\242\230.md"
@@ -0,0 +1,68 @@
+# candy(糖果问题)
+
+知识点:动态规划
+
+
+## 题目描述
+There are N children standing in a line. Each child is assigned a rating value.
+
+You are giving candies to these children subjected to the following requirements:
+
+Each child must have at least one candy.
+Children with a higher rating get more candies than their neighbors.
+What is the minimum candies you must give?
+
+有n个小孩站成了一行,每一个孩子都有一个等级值,你现在要给这些孩子按照以下原则分糖果:
+
+每一个小孩必须最少分到一个糖果;
+拥有更高等级值的孩子必须比他相邻的孩子分的糖果多。
+
+请问你最少需要多少糖果?
+
+
+## 解题思路
+rating={1,2,5,3,9,2}
+candyArray={1,1,1,1,1,1}
+采用只加不减的策略,扫描2遍:
+第一遍从左向右扫描,保证右边孩子如果优先级比左边孩子大就让右边孩子的糖果数比左边孩子多1,这样可以保证从左到右孩子的糖果分布式符合要求的;
+第二标从右向左扫描,保证左边孩子如果优先级比右边孩子大就让左边孩子的糖果数比左边孩子多1,这样可以保证从右到左孩子的糖果分布式符合要求的;
+走完上面两步之后,可以保证不管是从左往右走还是从右往左走,孩子们的糖果分配都是符合要求的,最后将candyArray求和返回即可。
+
+
+## 代码
+
+核心代码:
+```java
+public int candy(int[] ratings) {
+
+
+ int n = ratings.length;
+ int[] candyArray = new int[n];
+ //第0个孩子初始分配1个糖果
+ candyArray[0] = 1;
+ for (int index = 1; index < ratings.length; index++) {
+
+ //如果右边孩子的优先级大于左边孩子的优先级
+ if (ratings[index] > ratings[index - 1]) {
+ //右边孩子分配的糖果数更新为左边孩子分配的糖果数加1
+ candyArray[index] = candyArray[index - 1] + 1;
+ } else {
+ //如果右边孩子的优先级不大于左边孩子的优先级,将右边孩子的糖果数分配为1
+ candyArray[index] = 1;
+ }
+ }
+
+ int sum = candyArray[ratings.length - 1];
+ for (int index = ratings.length - 2; index >= 0; index--) {
+ //如果左边孩子的优先级大于右边孩子的优先级且左边孩子分配的糖果小于等于右边孩子分配的糖果
+ if (ratings[index] > ratings[index + 1] && candyArray[index] <= candyArray[index + 1]) {
+ //左边孩子分配的糖果数更新为右边孩子的糖果数加1
+ candyArray[index] = candyArray[index + 1] + 1;
+ }
+ sum += candyArray[index];
+ }
+ return sum;
+ }
+
+```
+[这里](../src/sixteen/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\350\256\241\347\256\227\351\200\206\346\263\242\345\205\260\345\274\217\347\232\204\345\200\274.md" "b/LeetCode/Doc/\350\256\241\347\256\227\351\200\206\346\263\242\345\205\260\345\274\217\347\232\204\345\200\274.md"
new file mode 100644
index 0000000..2274886
--- /dev/null
+++ "b/LeetCode/Doc/\350\256\241\347\256\227\351\200\206\346\263\242\345\205\260\345\274\217\347\232\204\345\200\274.md"
@@ -0,0 +1,29 @@
+# 计算逆波兰式的值(evaluate-reverse-polish-notation)
+
+知识点:栈
+
+
+## 题目描述
+
+Evaluate the value of an arithmetic expression in Reverse Polish Notation.
+
+Valid operators are+,-,*,/. Each operand may be an integer or another expression.
+
+Some examples:
+
+计算逆波兰式的值,给定式子中合法的操作符有+、-、*、/,操作数可能是整型或者另一个表达式,比如:
+
+ > ["2", "1", "+", "3", "*"] -> ((2 + 1) * 3) -> 9
+ > ["4", "13", "5", "/", "+"] -> (4 + (13 / 5)) -> 6
+
+## 解题思路
+
+从头遍历元素,如果当前元素不是+、0、*、/之一,即为数字,则将该字符串转化为数字后入栈,如果当前元素时运算符,则从栈中弹出连续的两个元素作为操作数,然后结合当前元素(操作符)计算当前式子的结果,然后将结果再次入栈,直至遍历完成。
+
+需要注意的一个细节是弹出的两个元素作为二元操作符两边时的顺序,举例:
+["2", "1", "/", "3", "*"]
+入栈后:[2,1]
+出栈时op1=1,op2=2,所以结果应该是op2/op1而不是op1/op2.
+## 代码
+
+[这里](../src/two/Solution.java)
\ No newline at end of file
diff --git "a/LeetCode/Doc/\351\223\276\350\241\250\351\207\215\346\216\222\345\272\217.md" "b/LeetCode/Doc/\351\223\276\350\241\250\351\207\215\346\216\222\345\272\217.md"
new file mode 100644
index 0000000..3f216b0
--- /dev/null
+++ "b/LeetCode/Doc/\351\223\276\350\241\250\351\207\215\346\216\222\345\272\217.md"
@@ -0,0 +1,34 @@
+# reorder-list(链表重排序)
+
+知识点:链表
+
+
+## 题目描述
+
+Given a singly linked list$ L: L_0→L_1→…→L{n-1}→L_n$,
+reorder it to: $L_0→L_n →L_1→L_{n-1}→L_2→L_{n-2}→…$
+
+You must do this in-place without altering the nodes' values.
+
+For example,
+Given{1,2,3,4}, reorder it to{1,4,2,3}.
+
+给定一个链表$ L: L_0→L_1→…→L{n-1}→L_n$,
+将其重排序为: $L_0→L_n →L_1→L_{n-1}→L_2→L_{n-2}→…$
+你必须在不改变节点值的情况下完成。
+比如:
+给定{1,2,3,4},重排序为:{1,4,2,3}
+
+## 解题思路
+
+比如:$ L: L_0→L_1→…→L{n-1}→L_n$,首先利用快慢指针将该链表分为两个部分:
+
+$L_a:L_0→L_1→…L_{\frac{n}{2}}$,
+
+$L_b:L_{\frac{n}{2}+1}→L_{\frac{n}{2}+2}→…L_{n}$
+
+然后将$L_b$翻转,然后合并$L_a$和$L_b$即可。
+
+## 代码
+
+[这里](../src/eight/Solution.java)
\ No newline at end of file
diff --git a/LeetCode/LeetCode.iml b/LeetCode/LeetCode.iml
index c90834f..bb6b39c 100644
--- a/LeetCode/LeetCode.iml
+++ b/LeetCode/LeetCode.iml
@@ -7,5 +7,15 @@
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/LeetCode/out/production/LeetCode/eight/ListNode.class b/LeetCode/out/production/LeetCode/eight/ListNode.class
new file mode 100644
index 0000000..332128c
Binary files /dev/null and b/LeetCode/out/production/LeetCode/eight/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/eight/Solution.class b/LeetCode/out/production/LeetCode/eight/Solution.class
new file mode 100644
index 0000000..841bad0
Binary files /dev/null and b/LeetCode/out/production/LeetCode/eight/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/eleven/Solution.class b/LeetCode/out/production/LeetCode/eleven/Solution.class
new file mode 100644
index 0000000..df5b41d
Binary files /dev/null and b/LeetCode/out/production/LeetCode/eleven/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/fifteen/Solution.class b/LeetCode/out/production/LeetCode/fifteen/Solution.class
new file mode 100644
index 0000000..c1ef9d7
Binary files /dev/null and b/LeetCode/out/production/LeetCode/fifteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/five/ListNode.class b/LeetCode/out/production/LeetCode/five/ListNode.class
new file mode 100644
index 0000000..4916f51
Binary files /dev/null and b/LeetCode/out/production/LeetCode/five/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/five/Solution.class b/LeetCode/out/production/LeetCode/five/Solution.class
new file mode 100644
index 0000000..882323c
Binary files /dev/null and b/LeetCode/out/production/LeetCode/five/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/four/ListNode.class b/LeetCode/out/production/LeetCode/four/ListNode.class
new file mode 100644
index 0000000..18aad38
Binary files /dev/null and b/LeetCode/out/production/LeetCode/four/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/four/Solution.class b/LeetCode/out/production/LeetCode/four/Solution.class
new file mode 100644
index 0000000..565bd80
Binary files /dev/null and b/LeetCode/out/production/LeetCode/four/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/fourteen/Solution.class b/LeetCode/out/production/LeetCode/fourteen/Solution.class
new file mode 100644
index 0000000..be2bb26
Binary files /dev/null and b/LeetCode/out/production/LeetCode/fourteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/nine/ListNode.class b/LeetCode/out/production/LeetCode/nine/ListNode.class
new file mode 100644
index 0000000..05f6cb4
Binary files /dev/null and b/LeetCode/out/production/LeetCode/nine/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/nine/Solution.class b/LeetCode/out/production/LeetCode/nine/Solution.class
new file mode 100644
index 0000000..56cb064
Binary files /dev/null and b/LeetCode/out/production/LeetCode/nine/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/one/Solution.class b/LeetCode/out/production/LeetCode/one/Solution.class
new file mode 100644
index 0000000..6f19ddd
Binary files /dev/null and b/LeetCode/out/production/LeetCode/one/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/one/TreeNode.class b/LeetCode/out/production/LeetCode/one/TreeNode.class
new file mode 100644
index 0000000..31e1261
Binary files /dev/null and b/LeetCode/out/production/LeetCode/one/TreeNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/eight/ListNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/eight/ListNode.class
new file mode 100644
index 0000000..6d2a93e
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/eight/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/eight/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/eight/Solution.class
new file mode 100644
index 0000000..a712098
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/eight/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/eleven/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/eleven/Solution.class
new file mode 100644
index 0000000..5efaf9b
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/eleven/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/fifteen/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/fifteen/Solution.class
new file mode 100644
index 0000000..68b6e53
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/fifteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/five/ListNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/five/ListNode.class
new file mode 100644
index 0000000..23a6999
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/five/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/five/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/five/Solution.class
new file mode 100644
index 0000000..828cd69
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/five/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/four/ListNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/four/ListNode.class
new file mode 100644
index 0000000..c73b577
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/four/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/four/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/four/Solution.class
new file mode 100644
index 0000000..8eb34b6
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/four/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/fourteen/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/fourteen/Solution.class
new file mode 100644
index 0000000..eaef3a2
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/fourteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/nine/ListNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/nine/ListNode.class
new file mode 100644
index 0000000..c5340ad
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/nine/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/nine/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/nine/Solution.class
new file mode 100644
index 0000000..3802afe
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/nine/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/one/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/one/Solution.class
new file mode 100644
index 0000000..e2afaf0
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/one/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/one/TreeNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/one/TreeNode.class
new file mode 100644
index 0000000..5a5535e
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/one/TreeNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/samplepackage/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/samplepackage/Solution.class
new file mode 100644
index 0000000..7015aac
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/samplepackage/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/seven/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/seven/Solution.class
new file mode 100644
index 0000000..f6cd089
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/seven/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/seven/TreeNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/seven/TreeNode.class
new file mode 100644
index 0000000..991e7b0
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/seven/TreeNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/seventeen/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/seventeen/Solution.class
new file mode 100644
index 0000000..f99cb0b
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/seventeen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/six/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/six/Solution.class
new file mode 100644
index 0000000..bd883f7
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/six/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/six/TreeNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/six/TreeNode.class
new file mode 100644
index 0000000..f0dfb19
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/six/TreeNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/sixteen/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/sixteen/Solution.class
new file mode 100644
index 0000000..41eeb6e
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/sixteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/ten/ListNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/ten/ListNode.class
new file mode 100644
index 0000000..94064ed
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/ten/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/ten/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/ten/Solution.class
new file mode 100644
index 0000000..f51489a
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/ten/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/thirteen/RandomListNode.class b/LeetCode/out/production/LeetCode/production/LeetCode/thirteen/RandomListNode.class
new file mode 100644
index 0000000..21a7b05
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/thirteen/RandomListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/thirteen/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/thirteen/Solution.class
new file mode 100644
index 0000000..15af792
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/thirteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/three/Point.class b/LeetCode/out/production/LeetCode/production/LeetCode/three/Point.class
new file mode 100644
index 0000000..95dd29d
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/three/Point.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/three/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/three/Solution.class
new file mode 100644
index 0000000..e8833f2
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/three/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/twelve/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/twelve/Solution.class
new file mode 100644
index 0000000..e7a2e34
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/twelve/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/production/LeetCode/two/Solution.class b/LeetCode/out/production/LeetCode/production/LeetCode/two/Solution.class
new file mode 100644
index 0000000..3880397
Binary files /dev/null and b/LeetCode/out/production/LeetCode/production/LeetCode/two/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/samplepackage/Solution.class b/LeetCode/out/production/LeetCode/samplepackage/Solution.class
new file mode 100644
index 0000000..8d986fa
Binary files /dev/null and b/LeetCode/out/production/LeetCode/samplepackage/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/seven/Solution.class b/LeetCode/out/production/LeetCode/seven/Solution.class
new file mode 100644
index 0000000..4c2d4e6
Binary files /dev/null and b/LeetCode/out/production/LeetCode/seven/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/seven/TreeNode.class b/LeetCode/out/production/LeetCode/seven/TreeNode.class
new file mode 100644
index 0000000..93e9323
Binary files /dev/null and b/LeetCode/out/production/LeetCode/seven/TreeNode.class differ
diff --git a/LeetCode/out/production/LeetCode/seventeen/Solution.class b/LeetCode/out/production/LeetCode/seventeen/Solution.class
new file mode 100644
index 0000000..caa71be
Binary files /dev/null and b/LeetCode/out/production/LeetCode/seventeen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/six/Solution.class b/LeetCode/out/production/LeetCode/six/Solution.class
new file mode 100644
index 0000000..d629a31
Binary files /dev/null and b/LeetCode/out/production/LeetCode/six/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/six/TreeNode.class b/LeetCode/out/production/LeetCode/six/TreeNode.class
new file mode 100644
index 0000000..b69e801
Binary files /dev/null and b/LeetCode/out/production/LeetCode/six/TreeNode.class differ
diff --git a/LeetCode/out/production/LeetCode/sixteen/Solution.class b/LeetCode/out/production/LeetCode/sixteen/Solution.class
new file mode 100644
index 0000000..bc830e9
Binary files /dev/null and b/LeetCode/out/production/LeetCode/sixteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/ten/ListNode.class b/LeetCode/out/production/LeetCode/ten/ListNode.class
new file mode 100644
index 0000000..3337b50
Binary files /dev/null and b/LeetCode/out/production/LeetCode/ten/ListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/ten/Solution.class b/LeetCode/out/production/LeetCode/ten/Solution.class
new file mode 100644
index 0000000..c50b548
Binary files /dev/null and b/LeetCode/out/production/LeetCode/ten/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/thirteen/RandomListNode.class b/LeetCode/out/production/LeetCode/thirteen/RandomListNode.class
new file mode 100644
index 0000000..b555ee9
Binary files /dev/null and b/LeetCode/out/production/LeetCode/thirteen/RandomListNode.class differ
diff --git a/LeetCode/out/production/LeetCode/thirteen/Solution.class b/LeetCode/out/production/LeetCode/thirteen/Solution.class
new file mode 100644
index 0000000..cc15554
Binary files /dev/null and b/LeetCode/out/production/LeetCode/thirteen/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/three/Point.class b/LeetCode/out/production/LeetCode/three/Point.class
new file mode 100644
index 0000000..85de9ba
Binary files /dev/null and b/LeetCode/out/production/LeetCode/three/Point.class differ
diff --git a/LeetCode/out/production/LeetCode/three/Solution.class b/LeetCode/out/production/LeetCode/three/Solution.class
new file mode 100644
index 0000000..5a343d0
Binary files /dev/null and b/LeetCode/out/production/LeetCode/three/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/twelve/Solution.class b/LeetCode/out/production/LeetCode/twelve/Solution.class
new file mode 100644
index 0000000..171652a
Binary files /dev/null and b/LeetCode/out/production/LeetCode/twelve/Solution.class differ
diff --git a/LeetCode/out/production/LeetCode/two/Solution.class b/LeetCode/out/production/LeetCode/two/Solution.class
new file mode 100644
index 0000000..4c3d94a
Binary files /dev/null and b/LeetCode/out/production/LeetCode/two/Solution.class differ
diff --git a/LeetCode/src/eight/Solution.java b/LeetCode/src/eight/Solution.java
new file mode 100644
index 0000000..ec55f31
--- /dev/null
+++ b/LeetCode/src/eight/Solution.java
@@ -0,0 +1,76 @@
+package eight;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+
+/**
+ * Definition for singly-linked list.
+ */
+class ListNode {
+ int val;
+ ListNode next;
+
+ ListNode(int x) {
+ val = x;
+ next = null;
+ }
+}
+
+public class Solution {
+ public void reorderList(ListNode head) {
+ if (head != null && head.next != null) {
+ ListNode fast, slow;
+ fast = slow = head;
+ //快慢指针找出链表的中间节点
+ while (fast.next != null && fast.next.next != null) {
+ fast = fast.next.next;
+ slow = slow.next;
+ }
+
+ //拆分链表
+ ListNode preNode = slow.next;
+ slow.next = null;
+ ListNode afterNode = preNode.next;
+ preNode.next=null;
+ //翻转后一半链表
+ while (afterNode != null) {
+ ListNode tempNode = afterNode.next;
+ afterNode.next = preNode;
+ preNode = afterNode;
+ afterNode = tempNode;
+ }
+
+ //合并链表
+ while (preNode != null && head != null) {
+ ListNode tempNode1 = head.next;
+ ListNode tempNode2 = preNode.next;
+ head.next = preNode;
+ preNode.next = tempNode1;
+ preNode = tempNode2;
+ head = tempNode1;
+
+ }
+
+
+ }
+
+ }
+
+
+ public static void main(String[] args) {
+ ListNode head = new ListNode(1);
+ ListNode node2 = new ListNode(2);
+ ListNode node3 = new ListNode(3);
+ ListNode node4 = new ListNode(4);
+ ListNode node5 = new ListNode(5);
+
+ head.next = node2;
+ node2.next = node3;
+ node3.next = node4;
+ node4.next = node5;
+ Solution solution = new Solution();
+ solution.reorderList(head);
+ }
+}
\ No newline at end of file
diff --git a/LeetCode/src/eleven/Solution.java b/LeetCode/src/eleven/Solution.java
new file mode 100644
index 0000000..51c1e13
--- /dev/null
+++ b/LeetCode/src/eleven/Solution.java
@@ -0,0 +1,45 @@
+package eleven;
+
+import java.util.HashSet;
+import java.util.Set;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+public class Solution {
+ public boolean wordBreak(String s, Set dict) {
+ if (s == null) {
+ return false;
+ }
+
+ int sLength = s.length();
+ boolean[] array = new boolean[sLength + 1];
+ array[0] = true;
+
+
+ for (int index = 0; index <= sLength; index++) {
+ for (int index2 = 0; index2 < index; index2++) {
+ for (String dictItem : dict) {
+ if (s.substring(index2, index).equals(dictItem) && array[index2]) {
+ array[index] = true;
+ }
+ }
+ }
+
+ }
+
+
+ return array[sLength];
+ }
+
+ public static void main(String[] args) {
+ Set dict = new HashSet<>();
+ dict.add("aaa");
+ dict.add("aaaa");
+ Solution solution = new Solution();
+ System.out.println(solution.wordBreak("aaaaaaa", dict));
+ }
+}
+
+
diff --git a/LeetCode/src/fifteen/Solution.java b/LeetCode/src/fifteen/Solution.java
new file mode 100644
index 0000000..6f33128
--- /dev/null
+++ b/LeetCode/src/fifteen/Solution.java
@@ -0,0 +1,30 @@
+package fifteen;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+public class Solution {
+ public int singleNumber(int[] A) {
+
+ //只出现过1次的位
+ int a = 0;
+ //出现过2次的位
+ int b = 0;
+ //出现过三次的位
+ int c;
+ for (int index = 0; index < A.length; index++) {
+ //之前的b或上现在的出现了2次的位
+ b = b | (a & A[index]);
+ //只出现过1次的位
+ a = a ^ A[index];
+ c = a & b;
+ //抹去出现了3次的bits,~c将原来是1的都变为0,这样那些为与完之后结果一定是0,原来为0的变为1,这样与的结果由a决定
+ a = a & (~c);
+ b = b & (~c);
+
+ }
+ return a;
+ }
+
+}
diff --git a/LeetCode/src/five/Solution.java b/LeetCode/src/five/Solution.java
new file mode 100644
index 0000000..14390b2
--- /dev/null
+++ b/LeetCode/src/five/Solution.java
@@ -0,0 +1,70 @@
+package five;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+
+class ListNode {
+ int val;
+ ListNode next;
+
+ ListNode(int x) {
+ val = x;
+ next = null;
+ }
+}
+
+public class Solution {
+ public ListNode insertionSortList(ListNode head) {
+ if (head == null || head.next == null) {
+ return head;
+ }
+
+ ListNode tempHead = new ListNode(0);
+ tempHead.next = head;
+
+
+ ListNode curNode = head;
+ ListNode indexNode;
+
+ while (curNode != null) {
+
+ indexNode = curNode.next;
+ while (indexNode != null && indexNode.val >= curNode.val) {
+ indexNode = indexNode.next;
+ curNode = curNode.next;
+ }
+
+ if (indexNode == null) {
+ return tempHead.next;
+ }
+
+ ListNode copyOfTempHead = tempHead;
+ ListNode copyOfHead = tempHead.next;
+
+ while (copyOfHead.val < indexNode.val) {
+ copyOfTempHead = copyOfTempHead.next;
+ copyOfHead = copyOfHead.next;
+ }
+ copyOfTempHead.next = indexNode;
+ curNode.next = indexNode.next;
+ indexNode.next = copyOfHead;
+
+
+ }
+
+ return tempHead.next;
+ }
+
+ public static void main(String[] args) {
+ ListNode head = new ListNode(3);
+ ListNode node2 = new ListNode(2);
+ ListNode node1 = new ListNode(1);
+ head.next = node2;
+ node2.next = node1;
+
+ Solution solution = new Solution();
+ solution.insertionSortList(head);
+ }
+}
diff --git a/LeetCode/src/four/Solution.java b/LeetCode/src/four/Solution.java
new file mode 100644
index 0000000..aee80bf
--- /dev/null
+++ b/LeetCode/src/four/Solution.java
@@ -0,0 +1,90 @@
+package four;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+
+/**
+ * Definition for singly-linked list.
+ */
+
+class ListNode {
+ int val;
+ ListNode next;
+
+ ListNode(int x) {
+ val = x;
+ next = null;
+ }
+}
+
+public class Solution {
+ public ListNode sortList(ListNode head) {
+ if (head == null || head.next == null) {
+ return head;
+ }
+ ListNode startNode, endNode;
+ startNode = head;
+ endNode = head.next;
+
+ while (startNode != null && endNode != null && endNode.next != null) {
+ startNode = startNode.next;
+ endNode = endNode.next.next;
+ }
+
+
+ ListNode right = sortList(startNode.next);
+ startNode.next = null;
+ ListNode left = sortList(head);
+
+
+ return mergeList(left, right);
+ }
+
+ private ListNode mergeList(ListNode left, ListNode right) {
+ ListNode tempListNode = new ListNode(0);
+ ListNode p = tempListNode;
+
+ while (left != null && right != null) {
+ if (left.val < right.val) {
+ p.next = left;
+ p = p.next;
+ left = left.next;
+ } else {
+ p.next = right;
+ p = p.next;
+ right = right.next;
+ }
+ }
+ if (left != null) {
+ p.next = left;
+ }
+ if (right != null) {
+ p.next = right;
+ }
+
+
+ return tempListNode.next;
+ }
+
+ public static void main(String[] args) {
+ ListNode head = new ListNode(1);
+ ListNode node2 = new ListNode(2);
+ ListNode node3 = new ListNode(3);
+ ListNode node4 = new ListNode(4);
+ ListNode node5 = new ListNode(5);
+ ListNode node6 = new ListNode(6);
+ ListNode node7 = new ListNode(7);
+
+ head.next = node7;
+ node7.next = node3;
+ node3.next = node2;
+ node2.next = node4;
+ node4.next = node5;
+
+ Solution solution = new Solution();
+ head = solution.sortList(head);
+ int a = 0;
+ }
+}
diff --git a/LeetCode/src/fourteen/Solution.java b/LeetCode/src/fourteen/Solution.java
new file mode 100644
index 0000000..cb924e9
--- /dev/null
+++ b/LeetCode/src/fourteen/Solution.java
@@ -0,0 +1,23 @@
+package fourteen;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+public class Solution {
+ public int singleNumber(int[] A) {
+
+
+ int res = A[0];
+ for (int index = 1; index < A.length; index++) {
+ res = res ^ A[index];
+ }
+ return res;
+ }
+
+ public static void main(String[] args) {
+ int[] A = {1, 2, 3, 2, 1};
+ Solution solution = new Solution();
+ System.out.println(solution.singleNumber(A));
+ }
+}
diff --git a/LeetCode/src/nine/Solution.java b/LeetCode/src/nine/Solution.java
new file mode 100644
index 0000000..7d7afc1
--- /dev/null
+++ b/LeetCode/src/nine/Solution.java
@@ -0,0 +1,65 @@
+package nine;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+
+/**
+ * Definition for singly-linked list.
+ */
+
+class ListNode {
+ int val;
+ ListNode next;
+
+ ListNode(int x) {
+ val = x;
+ next = null;
+ }
+}
+
+public class Solution {
+ public ListNode detectCycle(ListNode head) {
+ if (head == null) {
+ return null;
+ }
+ ListNode fast, slow;
+ fast = slow = head;
+ while (fast.next != null && fast.next.next != null) {
+ fast = fast.next.next;
+ slow = slow.next;
+ if (fast == slow) {
+ break;
+ }
+ }
+
+ if (fast.next == null || fast.next.next == null) {
+ return null;
+ }
+
+ fast = head;
+ while (fast != null && slow != null) {
+ if (fast == slow) {
+ return fast;
+ }
+ fast = fast.next;
+ slow = slow.next;
+
+ }
+ return null;
+
+
+ }
+
+ public static void main(String[] args){
+ ListNode head=new ListNode(1);
+ ListNode node2=new ListNode(2);
+ head.next=node2;
+ node2.next=head;
+ Solution solution=new Solution();
+ ListNode res=solution.detectCycle(head);
+ System.out.println(res.val);
+
+ }
+}
\ No newline at end of file
diff --git a/LeetCode/src/one/Solution.java b/LeetCode/src/one/Solution.java
new file mode 100644
index 0000000..7113c6a
--- /dev/null
+++ b/LeetCode/src/one/Solution.java
@@ -0,0 +1,79 @@
+package one;
+
+import java.util.LinkedList;
+import java.util.Queue;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+class TreeNode {
+ int val;
+ TreeNode left;
+ TreeNode right;
+
+ TreeNode(int x) {
+ val = x;
+ }
+}
+
+public class Solution {
+ public int run(TreeNode root) {
+ if (root == null) {
+ return 0;
+ }
+
+ Queue treeNodeQueue = new LinkedList();
+ treeNodeQueue.offer(root);
+ treeNodeQueue.offer(null);
+
+
+ int minDepth = 1;
+
+ while (!treeNodeQueue.isEmpty()) {
+ TreeNode curNode = treeNodeQueue.poll();
+ if (curNode == null) {
+
+ if (treeNodeQueue.isEmpty()) {
+ return minDepth;
+ } else {
+ minDepth += 1;
+ treeNodeQueue.offer(null);
+ }
+ } else {
+ //判断当前节点是否是叶子节点
+ if (curNode.left == null && curNode.right == null) {
+ return minDepth;
+ }
+ if (curNode.left != null) {
+ treeNodeQueue.offer(curNode.left);
+ }
+ if (curNode.right != null) {
+ treeNodeQueue.offer(curNode.right);
+ }
+ }
+
+
+ }
+ return minDepth;
+ }
+
+
+
+
+ public static void main(String[] args) {
+ Solution solution = new Solution();
+ TreeNode root = new TreeNode(1);
+ TreeNode node2 = new TreeNode(2);
+ TreeNode node3 = new TreeNode(2);
+ TreeNode node4 = new TreeNode(2);
+ TreeNode node5 = new TreeNode(2);
+ root.left = node2;
+ root.right = node3;
+ node2.left = node4;
+ node2.right = node5;
+
+ System.out.println(solution.run(root));
+
+ }
+}
\ No newline at end of file
diff --git a/LeetCode/src/samplepackage/Solution.java b/LeetCode/src/samplepackage/Solution.java
new file mode 100644
index 0000000..62a9eb3
--- /dev/null
+++ b/LeetCode/src/samplepackage/Solution.java
@@ -0,0 +1,13 @@
+package samplepackage;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+public class Solution {
+
+ public static void main(String[] args){
+ Solution solution=new Solution();
+
+ }
+}
diff --git a/LeetCode/src/seven/Solution.java b/LeetCode/src/seven/Solution.java
new file mode 100644
index 0000000..9cfce3a
--- /dev/null
+++ b/LeetCode/src/seven/Solution.java
@@ -0,0 +1,83 @@
+package seven;
+
+/**
+ * @author dmrfcoder
+ * @date 2019-04-13
+ */
+
+import java.util.ArrayList;
+import java.util.Stack;
+
+/**
+ * Definition for binary tree
+ */
+
+class TreeNode {
+ int val;
+ TreeNode left;
+ TreeNode right;
+
+ TreeNode(int x) {
+ val = x;
+ }
+}
+
+public class Solution {
+ public ArrayList preorderTraversal(TreeNode root) {
+ Stack stack = new Stack<>();
+ ArrayList arrayList = new ArrayList<>();
+ if (root == null) {
+ return arrayList;
+ }
+
+
+ TreeNode curNode = root;
+ arrayList.add(root.val);
+ stack.push(root);
+
+ while (!stack.empty()) {
+ if (curNode == null) {
+ curNode = stack.pop();
+ if (curNode.right != null) {
+ arrayList.add(curNode.right.val);
+ stack.push(curNode.right);
+ curNode = curNode.right;
+ } else {
+ curNode = null;
+ }
+ continue;
+ } else if (curNode.left != null) {
+ arrayList.add(curNode.left.val);
+ stack.push(curNode.left);
+
+
+ }
+ curNode = curNode.left;
+ }
+
+ return arrayList;
+
+ }
+
+ public static void main(String args[]) {
+ TreeNode root = new TreeNode(1);
+ TreeNode node2 = new TreeNode(2);
+ TreeNode node3 = new TreeNode(3);
+ TreeNode node4 = new TreeNode(4);
+ TreeNode node5 = new TreeNode(5);
+ TreeNode node6 = new TreeNode(6);
+ TreeNode node7 = new TreeNode(7);
+
+ root.left = node2;
+// root.right = node3;
+// node2.left = node4;
+// node2.right = node5;
+// node3.left = node6;
+// node3.right = node7;
+
+ Solution solution = new Solution();
+ ArrayList arrayList = solution.preorderTraversal(root);
+ System.out.println(arrayList.toArray().toString());
+
+ }
+}
\ No newline at end of file
diff --git a/LeetCode/src/seventeen/Solution.java b/LeetCode/src/seventeen/Solution.java
new file mode 100644
index 0000000..f78572f
--- /dev/null
+++ b/LeetCode/src/seventeen/Solution.java
@@ -0,0 +1,103 @@
+package seventeen;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+public class Solution {
+ public int canCompleteCircuit(int[] gas, int[] cost) {
+
+ //remaining为二维数组,remaing[index][0]代表车从第index出发到达index+1站后里面可以剩余的油,
+ // remaing[index][1]=index,因为后面要对remaing按照remaing[index][0]即剩余的油量排序,
+ // 所以要把原始的索引记录下来,排完序后直接从remaing[index][0]最大的值对应的remain[index][1]处开始开车即可
+ int[][] remaining = new int[gas.length][2];
+
+ //gas[i]---->gas[i+1] cost[i],计算remaining
+ for (int index = 0; index < gas.length; index++) {
+ remaining[index][0] = gas[index] - cost[index];
+ remaining[index][1] = index;
+ }
+
+ //按照remaining[index][0]对remaing进行排序
+ QuickSort(remaining, gas.length);
+
+ //由于上面排完序是降序,所以这里从后往前遍历
+ for (int index = gas.length - 1; index >= 0; index--) {
+ //如果剩余的油大于等于0,说明油足够到达下一站
+ if (remaining[index][0] >= 0) {
+ if (helper(gas, cost, remaining[index][1])) {
+ return remaining[index][1];
+ }
+ } else {
+ return -1;
+ }
+ }
+
+
+ return -1;
+ }
+
+ //模拟从第startIndex站出发,看能不能走完全程
+ boolean helper(int[] gas, int[] cost, int startIndex) {
+ int count = 0;
+ int curGas = 0;
+ for (int index = startIndex; count <= gas.length; count++, index = (index + 1) % gas.length) {
+ curGas += gas[index];
+ if (curGas >= cost[index]) {
+ curGas -= cost[index];
+ } else {
+ return false;
+ }
+ }
+
+ return true;
+ }
+
+ void QuickSort(int[][] v, int length) {
+ QSort(v, 0, length - 1);
+ }
+
+ void QSort(int[][] v, int low, int high) {
+
+ if (low >= high) {
+ return;
+ }
+ int t = Partition(v, low, high);
+ QSort(v, low, t - 1);
+ QSort(v, t + 1, high);
+ }
+
+ int Partition(int[][] v, int low, int high) {
+ int pivotkey;
+ pivotkey = v[low][0];
+
+ while (low < high) {
+ while (low < high && v[high][0] >= pivotkey) {
+ --high;
+ }
+ int[] t;
+ t = v[low];
+ v[low] = v[high];
+ v[high] = t;
+
+ while (low < high && v[low][0] <= pivotkey) {
+ ++low;
+ }
+ t = v[low];
+ v[low] = v[high];
+ v[high] = t;
+ }
+
+ return low;
+ }
+
+
+ public static void main(String[] args) {
+ Solution solution = new Solution();
+ int[] gas = {3, 2, 5, 3, 4, 3};
+ int[] cost = {2, 3, 4, 4, 3, 4};
+ int index = solution.canCompleteCircuit(gas, cost);
+ System.out.println("startIndex is: " + index);
+
+ }
+}
diff --git a/LeetCode/src/six/Solution.java b/LeetCode/src/six/Solution.java
new file mode 100644
index 0000000..b5b0d4a
--- /dev/null
+++ b/LeetCode/src/six/Solution.java
@@ -0,0 +1,88 @@
+package six;
+
+import java.util.ArrayList;
+import java.util.Stack;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+
+
+class TreeNode {
+ int val;
+ TreeNode left;
+ TreeNode right;
+
+ TreeNode(int x) {
+ val = x;
+ }
+}
+
+public class Solution {
+ public ArrayList postorderTraversal(TreeNode root) {
+ Stack treeNodeStack = new Stack<>();
+ ArrayList arrayList = new ArrayList<>();
+ if (root == null) {
+ return arrayList;
+ }
+
+ TreeNode preNode = null;
+ TreeNode curNode = null;
+
+ treeNodeStack.push(root);
+
+ while (!treeNodeStack.isEmpty()) {
+ if (curNode == null) {
+ curNode = treeNodeStack.peek();
+
+ }
+
+ if (curNode.left == null && curNode.right == null) {
+ preNode = curNode;
+ arrayList.add(curNode.val);
+ treeNodeStack.pop();
+ curNode = null;
+ } else if ((preNode == curNode.left && curNode.left != null) || (preNode == curNode.right && curNode.right != null)) {
+ preNode = curNode;
+ arrayList.add(curNode.val);
+ treeNodeStack.pop();
+ curNode = null;
+ } else {
+ if (curNode.right != null) {
+ treeNodeStack.push(curNode.right);
+ }
+
+ if (curNode.left != null) {
+ treeNodeStack.push(curNode.left);
+ }
+ curNode = curNode.left;
+ }
+
+
+ }
+ return arrayList;
+ }
+
+ public static void main(String args[]) {
+ TreeNode root = new TreeNode(1);
+ TreeNode node2 = new TreeNode(2);
+ TreeNode node3 = new TreeNode(3);
+ TreeNode node4 = new TreeNode(4);
+ TreeNode node5 = new TreeNode(5);
+ TreeNode node6 = new TreeNode(6);
+ TreeNode node7 = new TreeNode(7);
+
+ root.left = node2;
+// root.right = node3;
+// node2.left = node4;
+// node2.right = node5;
+// node3.left = node6;
+// node3.right = node7;
+
+ Solution solution = new Solution();
+ ArrayList arrayList = solution.postorderTraversal(root);
+ System.out.println(arrayList.toArray().toString());
+
+ }
+}
\ No newline at end of file
diff --git a/LeetCode/src/sixteen/Solution.java b/LeetCode/src/sixteen/Solution.java
new file mode 100644
index 0000000..40f6413
--- /dev/null
+++ b/LeetCode/src/sixteen/Solution.java
@@ -0,0 +1,48 @@
+package sixteen;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+public class Solution {
+ public int candy(int[] ratings) {
+
+
+ int n = ratings.length;
+ int[] candyArray = new int[n];
+ //第0个孩子初始分配1个糖果
+ candyArray[0] = 1;
+ for (int index = 1; index < ratings.length; index++) {
+
+ //如果右边孩子的优先级大于左边孩子的优先级
+ if (ratings[index] > ratings[index - 1]) {
+ //右边孩子分配的糖果数更新为左边孩子分配的糖果数加1
+ candyArray[index] = candyArray[index - 1] + 1;
+ } else {
+ //如果右边孩子的优先级不大于左边孩子的优先级,将右边孩子的糖果数分配为1
+ candyArray[index] = 1;
+ }
+ }
+
+ int sum = candyArray[ratings.length - 1];
+ for (int index = ratings.length - 2; index >= 0; index--) {
+ //如果左边孩子的优先级大于右边孩子的优先级且左边孩子分配的糖果小于等于右边孩子分配的糖果
+ if (ratings[index] > ratings[index + 1] && candyArray[index] <= candyArray[index + 1]) {
+ //左边孩子分配的糖果数更新为右边孩子的糖果数加1
+ candyArray[index] = candyArray[index + 1] + 1;
+ }
+ sum += candyArray[index];
+ }
+ return sum;
+ }
+
+
+ public static void main(String[] args) {
+
+ int[] ratings = {1, 0, 2};
+
+ Solution solution = new Solution();
+ int a = solution.candy(ratings);
+ System.out.println(a);
+ }
+}
diff --git a/LeetCode/src/ten/Solution.java b/LeetCode/src/ten/Solution.java
new file mode 100644
index 0000000..62262f5
--- /dev/null
+++ b/LeetCode/src/ten/Solution.java
@@ -0,0 +1,40 @@
+package ten;
+
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+
+class ListNode {
+ int val;
+ ListNode next;
+
+ ListNode(int x) {
+ val = x;
+ next = null;
+ }
+}
+
+public class Solution {
+
+ public boolean hasCycle(ListNode head) {
+
+ if (head == null) {
+ return false;
+ }
+ ListNode fast, slow;
+ fast = slow = head;
+
+ while (fast.next != null && fast.next.next != null) {
+ fast = fast.next.next;
+ slow = slow.next;
+ if (fast == slow) {
+ return true;
+ }
+ }
+ return false;
+
+
+ }
+}
diff --git a/LeetCode/src/thirteen/Solution.java b/LeetCode/src/thirteen/Solution.java
new file mode 100644
index 0000000..0a7d6d0
--- /dev/null
+++ b/LeetCode/src/thirteen/Solution.java
@@ -0,0 +1,91 @@
+package thirteen;
+
+/**
+ * @author dmrfcoder
+ * @date 2019-04-19
+ */
+
+/**
+ * Definition for singly-linked list with a random pointer.
+ */
+class RandomListNode {
+ int label;
+ RandomListNode next, random;
+
+ RandomListNode(int x) {
+ this.label = x;
+ }
+};
+
+public class Solution {
+ public RandomListNode copyRandomList(RandomListNode head) {
+
+ if (head == null) {
+ return null;
+ }
+ RandomListNode curNode = head;
+ RandomListNode curNodeNext;
+ while (curNode != null) {
+ RandomListNode copyOfCurNode = new RandomListNode(curNode.label);
+ curNodeNext = curNode.next;
+ curNode.next = copyOfCurNode;
+ copyOfCurNode.next = curNodeNext;
+ curNode = curNode.next.next;
+ }
+
+ curNode = head;
+
+ while (curNode != null) {
+ curNode.next.random = curNode.random;
+ curNode = curNode.next.next;
+ }
+
+ RandomListNode copyListHead = head.next;
+ RandomListNode curNewNode = head.next;
+ RandomListNode tempNode;
+
+ curNode = head;
+
+ while (curNode != null) {
+ tempNode = curNewNode.next;
+ curNode.next = tempNode;
+ if (tempNode != null) {
+ curNewNode.next = tempNode.next;
+ } else {
+ curNewNode.next = null;
+ }
+
+ curNode = tempNode;
+ if (curNode!=null){
+ curNewNode = curNode.next;
+ }
+
+
+ }
+
+ return copyListHead;
+ }
+
+ public static void main(String[] args) {
+ RandomListNode head = new RandomListNode(-1);
+ RandomListNode node2 = new RandomListNode(1);
+ RandomListNode node3 = new RandomListNode(3);
+ RandomListNode node4 = new RandomListNode(4);
+ RandomListNode node5 = new RandomListNode(5);
+ head.next = node2;
+ //node2.next = node3;
+// node3.next = node4;
+// node4.next = node5;
+//
+// head.random = node5;
+// node2.random = head;
+// node3.random = null;
+// node4.random = node3;
+// node5.random = node2;
+
+ Solution s = new Solution();
+ RandomListNode randomListNode = s.copyRandomList(head);
+
+ int a = 0;
+ }
+}
diff --git a/LeetCode/src/three/Solution.java b/LeetCode/src/three/Solution.java
new file mode 100644
index 0000000..b48b4dc
--- /dev/null
+++ b/LeetCode/src/three/Solution.java
@@ -0,0 +1,117 @@
+package three;
+
+import java.util.HashMap;
+import java.util.Map;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+class Point {
+ int x;
+ int y;
+
+ Point() {
+ x = 0;
+ y = 0;
+ }
+
+ Point(int a, int b) {
+ x = a;
+ y = b;
+ }
+}
+
+public class Solution {
+ public int maxPoints(Point[] points) {
+
+ int length = points.length;
+ int maxPointCount = 0;
+ for (int startIndex = 0; startIndex < length; startIndex++) {
+ Map maxPointMap = new HashMap<>();
+ int commonPointCount = 0;
+ int tempMaxCount = 1;
+ for (int endIndex = startIndex + 1; endIndex < length; endIndex++) {
+ int distanceX = points[startIndex].x - points[endIndex].x;
+ int distanceY = points[startIndex].y - points[endIndex].y;
+
+
+ if (distanceX == 0 && distanceY == 0) {
+ commonPointCount += 1;
+ } else {
+ String curStrDis;
+ if (distanceX == 0) {
+ curStrDis = "distanceX";
+ } else if (distanceY == 0) {
+ curStrDis = "distanceY";
+ } else {
+ int maxCommonDivisor = commonDivisor(distanceX, distanceY);
+ if (maxCommonDivisor != 0) {
+ distanceX = distanceX / maxCommonDivisor;
+ distanceY = distanceY / maxCommonDivisor;
+ }
+
+ int flag = 0;
+
+ if (distanceX < 0) {
+ distanceX = -distanceX;
+ flag++;
+ }
+ if (distanceY < 0) {
+ distanceY = -distanceY;
+ flag++;
+ }
+
+ curStrDis = String.valueOf(distanceX) + distanceY;
+ if (flag == 1) {
+ curStrDis = "-" + curStrDis;
+ }
+ }
+
+ if (maxPointMap.containsKey(curStrDis)) {
+ tempMaxCount = Math.max(tempMaxCount, maxPointMap.get(curStrDis) + 1);
+ maxPointMap.put(curStrDis, maxPointMap.get(curStrDis) + 1);
+ } else {
+ //初始时put进去2,一个点是起始点,另一个点是当前点
+ maxPointMap.put(curStrDis, 2);
+ tempMaxCount = Math.max(tempMaxCount, 2);
+ }
+ }
+ }
+ maxPointCount = Math.max(maxPointCount, tempMaxCount + commonPointCount);
+ }
+
+ return maxPointCount;
+ }
+
+ /**
+ * 求最大公约数
+ *
+ * @param a
+ * @param b
+ * @return
+ */
+ public static int commonDivisor(int a, int b) {
+ a = Math.abs(a);
+ b = Math.abs(b);
+ int count = 0;
+ for (int i = 1; i <= Math.min(a, b); i++) {
+ if (a % i == 0 && b % i == 0) {
+ count = i;
+ }
+ }
+ return count;
+ }
+
+ public static void main(String[] args) {
+
+ Point point1 = new Point(2, 3);
+ Point point2 = new Point(3, 3);
+ Point point3 = new Point(-5, 3);
+ Point point4 = new Point(9, -25);
+ Point[] points = {point1, point2, point3};
+ Solution solution = new Solution();
+ System.out.println(solution.maxPoints(points));
+ }
+
+}
diff --git a/LeetCode/src/twelve/Solution.java b/LeetCode/src/twelve/Solution.java
new file mode 100644
index 0000000..95732d2
--- /dev/null
+++ b/LeetCode/src/twelve/Solution.java
@@ -0,0 +1,74 @@
+package twelve;
+
+/**
+ * @author dmrfcoder
+ * @date 2019-04-18
+ */
+
+import java.util.*;
+
+public class Solution {
+ public ArrayList wordBreak(String s, Set dict) {
+ ArrayList dfs = dfs(s, dict, new HashMap<>());
+
+ return dfs;
+ }
+
+ private ArrayList dfs(String s, Set dict, Map> map) {
+
+ ArrayList arrayList = new ArrayList<>();
+ if ("".equals(s)) {
+ arrayList.add("");
+ return arrayList;
+ }
+ if (map.containsKey(s)) {
+ return map.get(s);
+ } else {
+ for (int index = s.length()-1; index >= 0; index--) {
+ if (dict.contains(s.substring(index))) {
+ ArrayList dfsResult = dfs(s.substring(0,index), dict, map);
+ if (dfsResult.size() == 0) {
+ continue;
+ } else {
+ String temp = s.substring(index);
+
+ for (String str : dfsResult) {
+ if ("".equals(str)) {
+ arrayList.add(temp);
+ } else {
+ StringBuilder stringBuilder = new StringBuilder();
+ stringBuilder.append(str).append(" ").append(temp);
+ arrayList.add(stringBuilder.toString());
+ }
+
+ }
+
+ }
+ }
+ }
+ }
+ map.put(s, arrayList);
+ return arrayList;
+
+ }
+
+ public static void main(String[] args) {
+ Set dict = new HashSet<>();
+ dict.add("cat");
+ dict.add("cats");
+ dict.add("and");
+ dict.add("sand");
+ dict.add("dog");
+ dict.add("aaaa");
+ dict.add("aa");
+ dict.add("a");
+ dict.add("b");
+ Solution solution = new Solution();
+ ArrayList list = solution.wordBreak("catsanddog", dict);
+ for (String s : list) {
+ System.out.println(s);
+ }
+
+ }
+
+}
\ No newline at end of file
diff --git a/LeetCode/src/two/Solution.java b/LeetCode/src/two/Solution.java
new file mode 100644
index 0000000..782cd32
--- /dev/null
+++ b/LeetCode/src/two/Solution.java
@@ -0,0 +1,59 @@
+package two;
+
+import java.util.Stack;
+
+/**
+ * @author dmrfcoder
+ * @date 2019/4/10
+ */
+public class Solution {
+ public int evalRPN(String[] tokens) {
+ Stack operateStack = new Stack<>();
+
+ int size = tokens.length;
+
+
+ for (int index = 0; index < size; index += 1) {
+ String itemToken = tokens[index];
+
+ if ("+".equals(itemToken) || "-".equals(itemToken) || "*".equals(itemToken) || "/".equals(itemToken)) {
+ Integer operate2 = operateStack.pop();
+ Integer operate1 = operateStack.pop();
+ Integer result = 0;
+ switch (itemToken) {
+ case "+":
+
+ result = operate1 + operate2;
+ break;
+ case "-":
+
+ result = operate1 - operate2;
+ break;
+ case "*":
+ result = operate1 * operate2;
+ break;
+ case "/":
+ result = (Integer) operate1 / operate2;
+ break;
+ default:
+ break;
+ }
+ operateStack.push(result);
+
+ } else {
+ operateStack.push(Integer.valueOf(itemToken));
+ }
+
+
+ }
+
+
+ return operateStack.pop();
+ }
+
+ public static void main(String[] args) {
+ String[] token = {"2","1","+","3","*"};
+ Solution solution = new Solution();
+ System.out.println(solution.evalRPN(token));
+ }
+}
diff --git a/README.md b/README.md
index 7acad24..01f6626 100644
--- a/README.md
+++ b/README.md
@@ -140,3 +140,26 @@
+# LeetCode
+
+- [minimum-depth-of-binary-tree(求解二叉树的最小深度)](./LeetCode/Doc/二叉树的最小深度.md)
+- [evaluate-reverse-polish-notation(计算逆波兰式的值)](./LeetCode/Doc/计算逆波兰式的值.md)
+- [max-points-on-a-line(同一条直线上的最多的点的数量)](./LeetCode/Doc/同一条直线上的最多的点的数量.md)
+- [sort-list(排序List)](./LeetCode/Doc/排序List.md)
+- [insertion-sort-list(使用插入排序对链表进行排序)](./LeetCode/Doc/使用插入排序对链表进行排序.md)
+- [binary-tree-postorder-traversal(后序遍历二叉树)](./LeetCode/Doc/后序遍历二叉树.md)
+- [binary-tree-preorder-traversal(先序遍历二叉树)](./LeetCode/Doc/先序遍历二叉树.md)
+- [reorder-list(链表重排序)](./LeetCode/Doc/链表重排序.md)
+- [linked-list-cycle-ii(找出链表中环的入口节点)](./LeetCode/Doc/找出链表中环的入口节点.md)
+- [linked-list-cycle(判断链表中是否存在环)](./LeetCode/Doc/判断链表中是否存在环.md)
+- [word-break(单词切分)](./LeetCode/Doc/单词切分.md)
+- [word-break-ii(单词切分2)](./LeetCode/Doc/单词切分2.md)
+- [copy-list-with-random-pointer(拷贝具有随机指针的链表)](./LeetCode/Doc/拷贝具有随机指针的链表.md)
+- [single-number(出现一次的数)](./LeetCode/Doc/出现一次的数.md)
+- [**single-number-ii(出现一次的数2)**](./LeetCode/Doc/出现一次的数2.md)
+- [candy(糖果问题)](./LeetCode/Doc/糖果问题.md)
+- [Gas-station(加油站问题)](./LeetCode/Doc/加油站问题.md)
+- [clone-graph(图的复制)](./LeetCode/Doc/图的复制.md)
+
+
+
diff --git "a/SwordToOffer/Doc/\346\225\264\346\225\260\344\270\2551\345\207\272\347\216\260\347\232\204\346\254\241\346\225\260.md" "b/SwordToOffer/Doc/\346\225\264\346\225\260\344\270\2551\345\207\272\347\216\260\347\232\204\346\254\241\346\225\260.md"
index ca9c894..0854469 100644
--- "a/SwordToOffer/Doc/\346\225\264\346\225\260\344\270\2551\345\207\272\347\216\260\347\232\204\346\254\241\346\225\260.md"
+++ "b/SwordToOffer/Doc/\346\225\264\346\225\260\344\270\2551\345\207\272\347\216\260\347\232\204\346\254\241\346\225\260.md"
@@ -4,7 +4,7 @@
## 题目描述
-求出1~13的整数中1出现的次数,并算出100~1300的整数中1出现的次数?为此他特别数了一下1~13中包含1的数字有1、10、11、12、13因此共出现6次,但是对于后面问题他就没辙了。ACMer希望你们帮帮他,并把问题更加普遍化,可以很快的求出任意非负整数区间中1出现的次数(从1 到 n 中1出现的次数)。
+求出1 ~ 13的整数中1出现的次数,并算出100 ~ 1300的整数中1出现的次数?为此他特别数了一下1~13中包含1的数字有1、10、11、12、13因此共出现6次,但是对于后面问题他就没辙了。ACMer希望你们帮帮他,并把问题更加普遍化,可以很快的求出任意非负整数区间中1出现的次数(从1 到 n 中1出现的次数)。
## 解题思路
### 思路一
观察规律,以百位上出现1的次数为例说明,假设n大于等于100且百位上的数为k,则: