|
| 1 | +### 新建 Spring Boot 项目常用的两种方式 |
| 2 | + |
| 3 | +你可以通过 https://start.spring.io/ 这个网站来生成一个 Spring Boot 的项目。 |
| 4 | + |
| 5 | +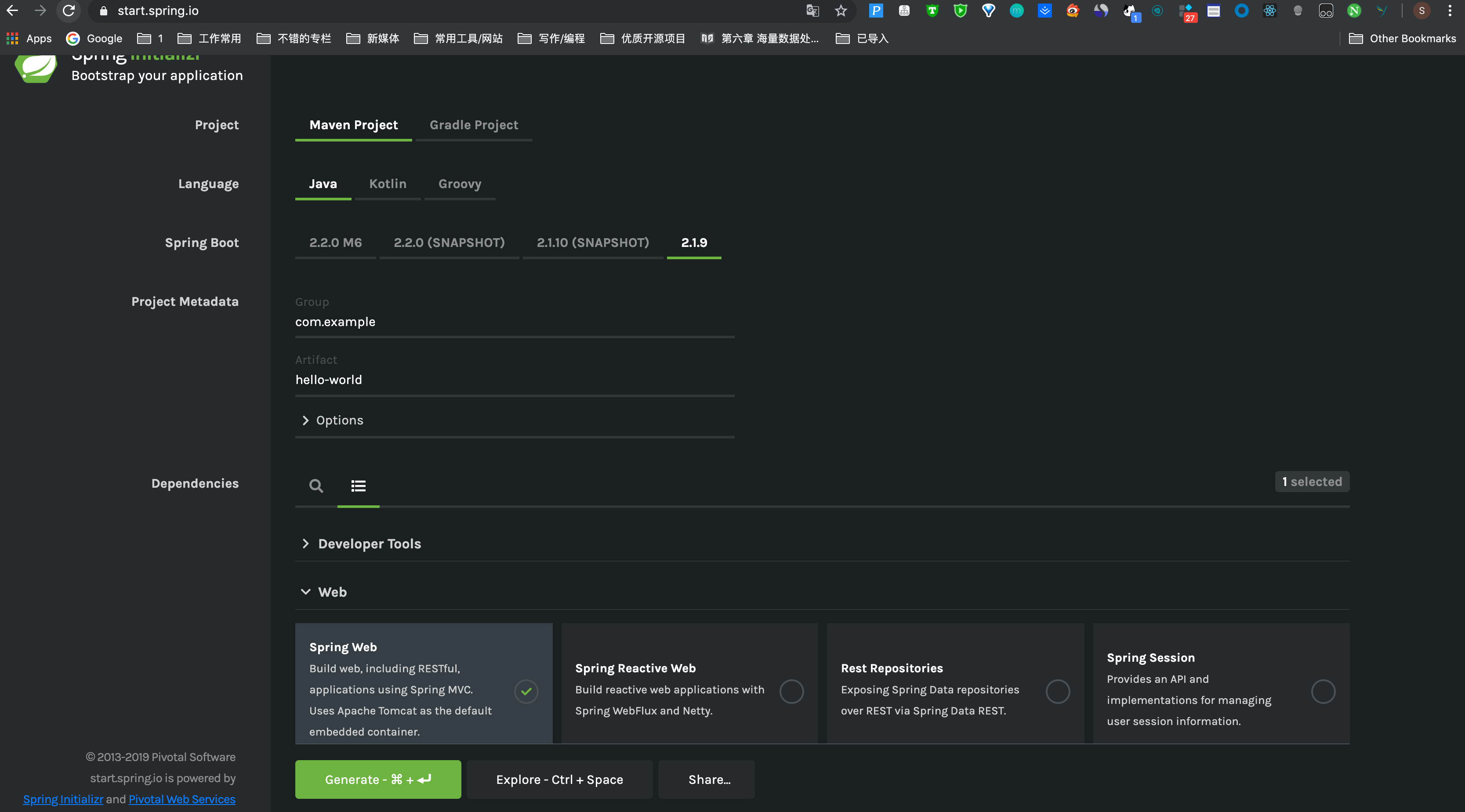 |
| 6 | + |
| 7 | +注意勾选上 Spring Web 这个模块,这是我们所必需的一个依赖。当所有选项都勾选完毕之后,点击下方的按钮 Generate 下载这个 Spring Boot 的项目。下载完成并解压之后,我们直接使用 IDEA 打开即可。 |
| 8 | + |
| 9 | +当然你也可以直接通过 IDEA 来生成一个 Spring Boot 的项目,具体方法和上面类似:`File->New->Project->Spring Initializr`。 |
| 10 | + |
| 11 | +### Spring Boot 项目结构分析 |
| 12 | + |
| 13 | +成功打开项目之后,项目长下面这个样子: |
| 14 | + |
| 15 | +<img src="https://my-blog-to-use.oss-cn-beijing.aliyuncs.com/2019-7/springboot-hellowold-structure.png" style="zoom:50%;" /> |
| 16 | + |
| 17 | + |
| 18 | + |
| 19 | +以 Application为后缀名的 Java 类一般就是 Spring Boot 的启动类,比如本项目的启动项目就是`HelloWorldApplication` 。我们直接像运行普通 Java 程序一样运行它,由于 Spring Boot 本身就嵌入servlet容器的缘故,我们的 web 项目就运行成功了, 非常方便。 |
| 20 | + |
| 21 | +需要注意的一点是 **Spring Boot 的启动类是需要最外层的,不然可能导致一些类无法被正确扫描到,导致一些奇怪的问题。** 一般情况下 Spring Boot 项目结构类似下面这样 |
| 22 | + |
| 23 | +``` |
| 24 | +com |
| 25 | + +- example |
| 26 | + +- myproject |
| 27 | + +- Application.java |
| 28 | + | |
| 29 | + +- domain |
| 30 | + | +- Customer.java |
| 31 | + | +- CustomerRepository.java |
| 32 | + | |
| 33 | + +- service |
| 34 | + | +- CustomerService.java |
| 35 | + | |
| 36 | + +- controller |
| 37 | + | +- CustomerController.java |
| 38 | + | |
| 39 | + | +- config |
| 40 | + | +- swagerConfig.java |
| 41 | + | |
| 42 | +``` |
| 43 | + |
| 44 | +1. ` Application.java`是项目的启动类 |
| 45 | +2. domain目录主要用于实体(Entity)与数据访问层(Repository) |
| 46 | +3. service 层主要是业务类代码 |
| 47 | +4. controller 负责页面访问控制 |
| 48 | +5. config 目录主要放一些配置类 |
| 49 | + |
| 50 | +### @SpringBootApplication 注解分析 |
| 51 | + |
| 52 | +`HelloWorldApplication` |
| 53 | + |
| 54 | +```java |
| 55 | +@SpringBootApplication |
| 56 | +public class HelloWorldApplication { |
| 57 | + |
| 58 | + public static void main(String[] args) { |
| 59 | + SpringApplication.run(HelloWorldApplication.class, args); |
| 60 | + } |
| 61 | + |
| 62 | +} |
| 63 | +``` |
| 64 | + |
| 65 | +说到 Spring Boot 启动类就不得不介绍一下 **`@SpringBootApplication` 注解**了,这个注解的相关代码如下: |
| 66 | + |
| 67 | +```java |
| 68 | +package org.springframework.boot.autoconfigure; |
| 69 | +@Target(ElementType.TYPE) |
| 70 | +@Retention(RetentionPolicy.RUNTIME) |
| 71 | +@Documented |
| 72 | +@Inherited |
| 73 | +@SpringBootConfiguration |
| 74 | +@EnableAutoConfiguration |
| 75 | +@ComponentScan(excludeFilters = { |
| 76 | + @Filter(type = FilterType.CUSTOM, classes = TypeExcludeFilter.class), |
| 77 | + @Filter(type = FilterType.CUSTOM, classes = AutoConfigurationExcludeFilter.class) }) |
| 78 | +public @interface SpringBootApplication { |
| 79 | + ...... |
| 80 | +} |
| 81 | +``` |
| 82 | + |
| 83 | +```java |
| 84 | +package org.springframework.boot; |
| 85 | +@Target(ElementType.TYPE) |
| 86 | +@Retention(RetentionPolicy.RUNTIME) |
| 87 | +@Documented |
| 88 | +@Configuration |
| 89 | +public @interface SpringBootConfiguration { |
| 90 | + |
| 91 | +} |
| 92 | +``` |
| 93 | + |
| 94 | +可以看出大概可以把 `@SpringBootApplication `看作是 `@Configuration`、`@EnableAutoConfiguration`、`@ComponentScan ` 注解的集合。根据 SpringBoot官网,这三个注解的作用分别是: |
| 95 | + |
| 96 | +- `@EnableAutoConfiguration`:启用 SpringBoot 的自动配置机制 |
| 97 | +- `@ComponentScan`: 扫描被`@Component` (`@Service`,`@Controller`)注解的bean,注解默认会扫描该类所在的包下所有的类。 |
| 98 | +- `@Configuration`:允许在上下文中注册额外的bean或导入其他配置类。 |
| 99 | + |
| 100 | +所以说 `@SpringBootApplication `就是几个重要的注解的组合,为什么要有它?当然是为了省事,避免了我们每次开发 Spring Boot 项目都要写一些必备的注解。这一点在我们平时开发中也经常用到,比如我们通常会提一个测试基类,这个基类包含了我们写测试所需要的一些基本的注解和一些依赖。 |
| 101 | + |
| 102 | +### 新建一个 Controller |
| 103 | + |
| 104 | +上面说了这么多,我们现在正式开始写 Spring Boot 版的 “Hello World” 吧。 |
| 105 | + |
| 106 | +新建一个 controller 文件夹,并在这个文件夹下新建一个名字叫做 `HelloWorldController` 的类。 |
| 107 | + |
| 108 | +`com.example.helloworld.controller` |
| 109 | + |
| 110 | +```java |
| 111 | +import org.springframework.web.bind.annotation.GetMapping; |
| 112 | +import org.springframework.web.bind.annotation.RequestMapping; |
| 113 | +import org.springframework.web.bind.annotation.RestController; |
| 114 | + |
| 115 | +@RestController |
| 116 | +@RequestMapping("test") |
| 117 | +public class HelloWorldController { |
| 118 | + @GetMapping("hello") |
| 119 | + public String sayHello() { |
| 120 | + return "Hello World"; |
| 121 | + } |
| 122 | +} |
| 123 | +``` |
| 124 | + |
| 125 | +默认情况下,Spring Boot 项目会使用 8080 作为项目的端口号。如果我们修改端口号的话,非常简单,直接修改 |
| 126 | + |
| 127 | +`application.properties`配置文件即可。 |
| 128 | + |
| 129 | +`src/main/resources/application.properties` |
| 130 | + |
| 131 | +```properties |
| 132 | +server.port=8333 |
| 133 | +``` |
| 134 | + |
| 135 | +### 大功告成,运行项目 |
| 136 | + |
| 137 | + |
| 138 | + |
| 139 | + |
| 140 | + |
| 141 | +- [ ] Spring Boot 启动流程分析 |
| 142 | + |
| 143 | + |
0 commit comments