|
| 1 | +## Functions - arguments and parameters |
| 2 | + |
| 3 | +- Parameters are like _placeholders_ for data that will be passed to a function. |
| 4 | + |
| 5 | +- Arguments are the actual values passed to a function while calling it |
| 6 | + |
| 7 | + ```javascript |
| 8 | + function calculateBill(billAmount, taxRate) { // here billAmount, taxRate are parameters const total = billAmount + billAmount * taxRate return total; } |
| 9 | + |
| 10 | + calculateBill(100, 0.13); // here 100, 0.13 are arguments |
| 11 | + ``` |
| 12 | +
|
| 13 | +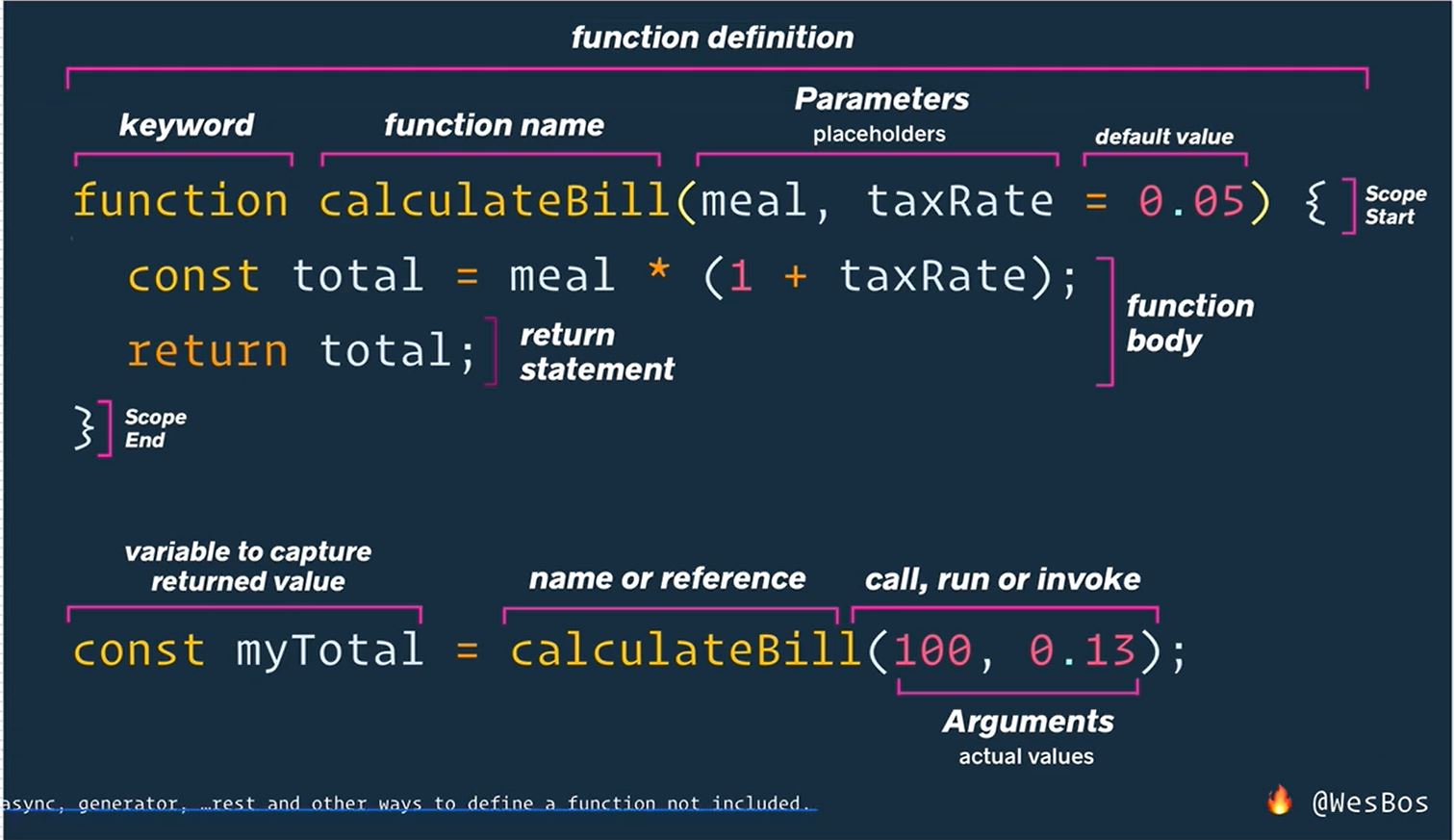 |
| 14 | +
|
| 15 | +- Parameters are variables local to the function; available only inside the function. |
| 16 | +
|
| 17 | +- You can also pass variables as arguments during a function call. |
| 18 | +
|
| 19 | +- We can also pass _expressions_ as arguments to a function. |
| 20 | +
|
| 21 | + ``` |
| 22 | + myTotal3 = calculateBill(20+20+30, 0.3); |
| 23 | + |
| 24 | + ``` |
| 25 | +
|
| 26 | +- So, we can either pass direct value or variables holding value or expressions resulting in a value to a function as arguments. |
| 27 | +
|
| 28 | +- **Passing functions as arguments:** |
| 29 | +
|
| 30 | + ```javascript |
| 31 | + function doctorize(name) { |
| 32 | + return `Dr. ${name}`; |
| 33 | + } |
| 34 | + |
| 35 | + function yell(name) { |
| 36 | + return `HEY ${name.toUpperCase()}`; |
| 37 | + } |
| 38 | + |
| 39 | + // We can pass a function inside another |
| 40 | + yell(doctorize("Soumya")); // HEY DR. SOUMYA |
| 41 | + // Above, returned value of doctorize function is passed to yell function |
| 42 | + ``` |
| 43 | +
|
| 44 | + - Default values: |
| 45 | +
|
| 46 | + ```javascript |
| 47 | + function yell(name = "Silly Goose") { |
| 48 | + return `HEY ${name.toUpperCase()}`; |
| 49 | + } |
| 50 | + |
| 51 | + yell("Soumya"); // HEY SOUMYA |
| 52 | + yell(); // HEY SILLY GOOSE |
| 53 | + |
| 54 | + // Above, if we don't pass any argument to yell function, then it takes the default value in function definition, |
| 55 | + // here Silly Goose, else it takes whatever we pass as argument. |
| 56 | + ``` |
| 57 | +
|
| 58 | + - **Important gotcha:** |
| 59 | +
|
| 60 | + ```javascript |
| 61 | + function calculateBill(billAmount, taxRate = 0.13, tipRate = 0.15) { |
| 62 | + console.log("Running Calculate Bill!!"); |
| 63 | + const total = billAmount + billAmount * taxRate + billAmount * tipRate; |
| 64 | + return total; |
| 65 | + } |
| 66 | + |
| 67 | + // Suppose above, we want to pass the tipRate but not the taxRate and want taxRate to be default, |
| 68 | + // then the only thing we can do is: |
| 69 | + |
| 70 | + calculateBill(100, undefined, 0.66); // here the taxRate will default to 0.13 as |
| 71 | + // we have passed undefined to it and the tipRate will be 0.66 as passed |
| 72 | + ``` |
0 commit comments