|
| 1 | +# [面试题36. 二叉搜索树与双向链表](https://leetcode-cn.com/problems/er-cha-sou-suo-shu-yu-shuang-xiang-lian-biao-lcof/) |
| 2 | + |
| 3 | +## 题目描述 |
| 4 | +<!-- 这里写题目描述 --> |
| 5 | +输入一棵二叉搜索树,将该二叉搜索树转换成一个排序的循环双向链表。要求不能创建任何新的节点,只能调整树中节点指针的指向。 |
| 6 | + |
| 7 | +为了让您更好地理解问题,以下面的二叉搜索树为例: |
| 8 | + |
| 9 | +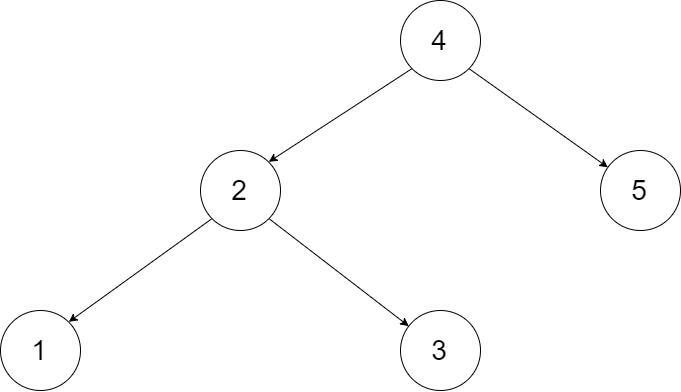 |
| 10 | + |
| 11 | +我们希望将这个二叉搜索树转化为双向循环链表。链表中的每个节点都有一个前驱和后继指针。对于双向循环链表,第一个节点的前驱是最后一个节点,最后一个节点的后继是第一个节点。 |
| 12 | + |
| 13 | +下图展示了上面的二叉搜索树转化成的链表。“head” 表示指向链表中有最小元素的节点。 |
| 14 | + |
| 15 | +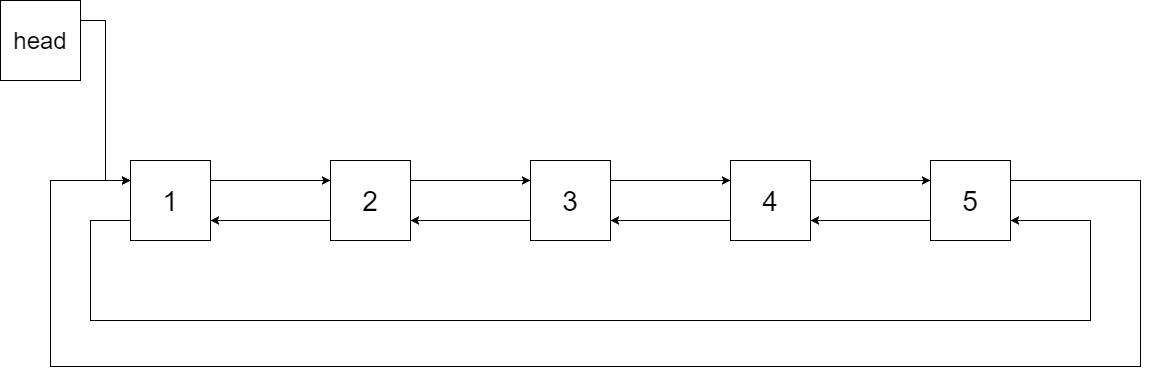 |
| 16 | + |
| 17 | +特别地,我们希望可以就地完成转换操作。当转化完成以后,树中节点的左指针需要指向前驱,树中节点的右指针需要指向后继。还需要返回链表中的第一个节点的指针。 |
| 18 | + |
| 19 | +**注意**:本题与主站 426 题相同:https://leetcode-cn.com/problems/convert-binary-search-tree-to-sorted-doubly-linked-list/ |
| 20 | + |
| 21 | +**注意**:此题对比原题有改动。 |
| 22 | + |
| 23 | +## 解法 |
| 24 | +<!-- 这里可写通用的实现逻辑 --> |
| 25 | +二叉搜索树中序遍历得到有序序列;根结点指向左子树最后一个结点,根结点也指向右子树第一个结点。 |
| 26 | + |
| 27 | +利用虚拟头结点递归遍历求解。 |
| 28 | + |
| 29 | +### Python3 |
| 30 | +<!-- 这里可写当前语言的特殊实现逻辑 --> |
| 31 | + |
| 32 | +```python |
| 33 | +""" |
| 34 | +# Definition for a Node. |
| 35 | +class Node: |
| 36 | + def __init__(self, val, left=None, right=None): |
| 37 | + self.val = val |
| 38 | + self.left = left |
| 39 | + self.right = right |
| 40 | +""" |
| 41 | + |
| 42 | + |
| 43 | +class Solution: |
| 44 | + def treeToDoublyList(self, root: 'Node') -> 'Node': |
| 45 | + def dfs(cur): |
| 46 | + if not cur: |
| 47 | + return |
| 48 | + dfs(cur.left) |
| 49 | + cur.left = self.lastNode |
| 50 | + if self.lastNode: |
| 51 | + self.lastNode.right = cur |
| 52 | + self.lastNode = cur |
| 53 | + dfs(cur.right) |
| 54 | + |
| 55 | + if not root: |
| 56 | + return root |
| 57 | + self.lastNode = head = Node(-1) |
| 58 | + dfs(root) |
| 59 | + head = head.right |
| 60 | + head.left = self.lastNode |
| 61 | + self.lastNode.right = head |
| 62 | + return head |
| 63 | + |
| 64 | +``` |
| 65 | + |
| 66 | +### Java |
| 67 | +<!-- 这里可写当前语言的特殊实现逻辑 --> |
| 68 | + |
| 69 | +```java |
| 70 | +/* |
| 71 | +// Definition for a Node. |
| 72 | +class Node { |
| 73 | + public int val; |
| 74 | + public Node left; |
| 75 | + public Node right; |
| 76 | +
|
| 77 | + public Node() {} |
| 78 | +
|
| 79 | + public Node(int _val) { |
| 80 | + val = _val; |
| 81 | + } |
| 82 | +
|
| 83 | + public Node(int _val,Node _left,Node _right) { |
| 84 | + val = _val; |
| 85 | + left = _left; |
| 86 | + right = _right; |
| 87 | + } |
| 88 | +}; |
| 89 | +*/ |
| 90 | +class Solution { |
| 91 | + private Node lastNode; |
| 92 | + public Node treeToDoublyList(Node root) { |
| 93 | + if (root == null) { |
| 94 | + return root; |
| 95 | + } |
| 96 | + lastNode = new Node(-1); |
| 97 | + Node head = lastNode; |
| 98 | + dfs(root); |
| 99 | + head = head.right; |
| 100 | + head.left = lastNode; |
| 101 | + lastNode.right = head; |
| 102 | + return head; |
| 103 | + } |
| 104 | + |
| 105 | + private void dfs(Node cur) { |
| 106 | + if (cur == null) { |
| 107 | + return; |
| 108 | + } |
| 109 | + dfs(cur.left); |
| 110 | + cur.left = lastNode; |
| 111 | + if (lastNode != null) { |
| 112 | + lastNode.right = cur; |
| 113 | + } |
| 114 | + lastNode = cur; |
| 115 | + dfs(cur.right); |
| 116 | + } |
| 117 | +} |
| 118 | +``` |
| 119 | + |
| 120 | +### ... |
| 121 | +``` |
| 122 | +
|
| 123 | +``` |
0 commit comments