This repository was archived by the owner on Nov 1, 2021. It is now read-only.
Commit 5b4b6e9
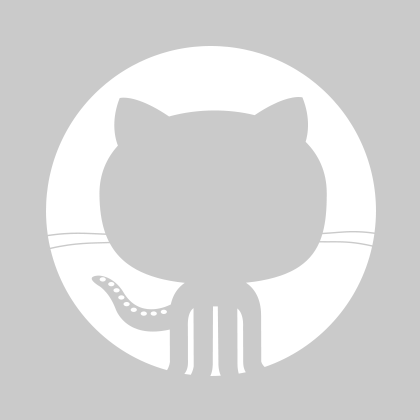
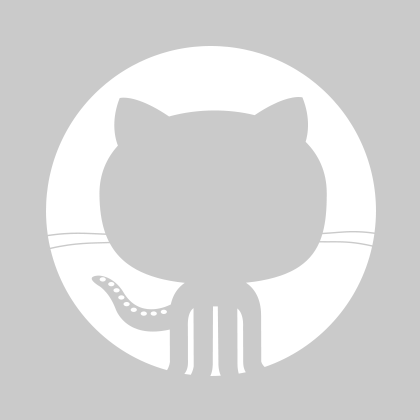
Artyom Skrobov
Artyom Skrobov
1 parent 0a07c05 commit 5b4b6e9
File tree
5 files changed
+149
-117
lines changed- include/clang/Analysis/Analyses
- lib/Analysis
5 files changed
+149
-117
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
15 | 16 |
| |
16 | 17 |
| |
17 | 18 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
17 |
| - | |
| 17 | + | |
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
22 | 21 |
| |
23 | 22 |
| |
24 | 23 |
| |
25 | 24 |
| |
26 | 25 |
| |
27 | 26 |
| |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 | 27 |
| |
82 | 28 |
| |
83 | 29 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
15 | 15 |
| |
16 | 16 |
| |
17 | 17 |
| |
18 |
| - | |
| 18 | + | |
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
| |||
198 | 198 |
| |
199 | 199 |
| |
200 | 200 |
| |
201 |
| - | |
202 |
| - | |
203 |
| - | |
204 |
| - | |
205 |
| - | |
206 |
| - | |
207 |
| - | |
208 |
| - | |
209 |
| - | |
210 |
| - | |
211 |
| - | |
212 |
| - | |
213 |
| - | |
214 |
| - | |
215 |
| - | |
216 |
| - | |
217 |
| - | |
218 |
| - | |
219 |
| - | |
220 |
| - | |
221 |
| - | |
222 |
| - | |
223 |
| - | |
224 |
| - | |
225 |
| - | |
226 |
| - | |
227 |
| - | |
228 |
| - | |
229 |
| - | |
230 |
| - | |
231 |
| - | |
232 |
| - | |
233 |
| - | |
234 |
| - | |
235 |
| - | |
236 |
| - | |
237 |
| - | |
238 |
| - | |
239 |
| - | |
240 |
| - | |
241 |
| - | |
242 |
| - | |
243 |
| - | |
244 |
| - | |
245 |
| - | |
246 |
| - | |
247 |
| - | |
248 |
| - | |
249 |
| - | |
250 |
| - | |
251 |
| - | |
252 |
| - | |
253 |
| - | |
254 |
| - | |
255 |
| - | |
256 |
| - | |
257 |
| - | |
258 |
| - | |
259 |
| - | |
260 |
| - | |
261 | 201 |
| |
262 | 202 |
| |
263 | 203 |
| |
| |||
831 | 771 |
| |
832 | 772 |
| |
833 | 773 |
| |
834 |
| - | |
| 774 | + | |
835 | 775 |
| |
836 | 776 |
| |
837 | 777 |
| |
|
0 commit comments