cnt{{0, 1}};
+ auto dfs = [&](this auto&& dfs, TreeNode* root, long long s) -> int {
if (!root) {
return 0;
}
@@ -26,4 +25,4 @@ class Solution {
};
return dfs(root, 0);
}
-};
\ No newline at end of file
+};
diff --git a/lcci/04.12.Paths with Sum/Solution.py b/lcci/04.12.Paths with Sum/Solution.py
index 096e03e07552b..25e2179fe9ddc 100644
--- a/lcci/04.12.Paths with Sum/Solution.py
+++ b/lcci/04.12.Paths with Sum/Solution.py
@@ -1,14 +1,12 @@
# Definition for a binary tree node.
# class TreeNode:
-# def __init__(self, x):
-# self.val = x
-# self.left = None
-# self.right = None
-
-
+# def __init__(self, val=0, left=None, right=None):
+# self.val = val
+# self.left = left
+# self.right = right
class Solution:
- def pathSum(self, root: TreeNode, sum: int) -> int:
- def dfs(root: TreeNode, s: int):
+ def pathSum(self, root: Optional[TreeNode], sum: int) -> int:
+ def dfs(root: Optional[TreeNode], s: int) -> int:
if root is None:
return 0
s += root.val
diff --git a/lcci/04.12.Paths with Sum/Solution.swift b/lcci/04.12.Paths with Sum/Solution.swift
index cf1cbe9a5b95e..ad6fb0b161171 100644
--- a/lcci/04.12.Paths with Sum/Solution.swift
+++ b/lcci/04.12.Paths with Sum/Solution.swift
@@ -1,38 +1,36 @@
-/* class TreeNode {
-* var val: Int
-* var left: TreeNode?
-* var right: TreeNode?
-*
-* init(_ val: Int, _ left: TreeNode? = nil, _ right: TreeNode? = nil) {
-* self.val = val
-* self.left = left
-* self.right = right
-* }
-* }
-*/
-
+/**
+ * Definition for a binary tree node.
+ * public class TreeNode {
+ * public var val: Int
+ * public var left: TreeNode?
+ * public var right: TreeNode?
+ * public init() { self.val = 0; self.left = nil; self.right = nil; }
+ * public init(_ val: Int) { self.val = val; self.left = nil; self.right = nil; }
+ * public init(_ val: Int, _ left: TreeNode?, _ right: TreeNode?) {
+ * self.val = val
+ * self.left = left
+ * self.right = right
+ * }
+ * }
+ */
class Solution {
- private var cnt: [Int: Int] = [:]
- private var target: Int = 0
-
func pathSum(_ root: TreeNode?, _ sum: Int) -> Int {
- cnt[0] = 1
- target = sum
- return dfs(root, 0)
- }
+ var cnt: [Int: Int] = [0: 1]
+
+ func dfs(_ root: TreeNode?, _ s: Int) -> Int {
+ guard let root = root else { return 0 }
- private func dfs(_ root: TreeNode?, _ s: Int) -> Int {
- guard let root = root else {
- return 0
+ var s = s + root.val
+ var ans = cnt[s - sum, default: 0]
+
+ cnt[s, default: 0] += 1
+ ans += dfs(root.left, s)
+ ans += dfs(root.right, s)
+ cnt[s, default: 0] -= 1
+
+ return ans
}
- let newSum = s + root.val
- let ans = cnt[newSum - target, default: 0]
-
- cnt[newSum, default: 0] += 1
- let leftPaths = dfs(root.left, newSum)
- let rightPaths = dfs(root.right, newSum)
- cnt[newSum, default: 0] -= 1
-
- return ans + leftPaths + rightPaths
+
+ return dfs(root, 0)
}
-}
\ No newline at end of file
+}
diff --git a/lcci/05.03.Reverse Bits/README.md b/lcci/05.03.Reverse Bits/README.md
index cbec7bc2a611d..fad13598cbc1b 100644
--- a/lcci/05.03.Reverse Bits/README.md
+++ b/lcci/05.03.Reverse Bits/README.md
@@ -134,13 +134,13 @@ function reverseBits(num: number): number {
class Solution {
func reverseBits(_ num: Int) -> Int {
var ans = 0
- var countZeros = 0
+ var cnt = 0
var j = 0
for i in 0..<32 {
- countZeros += (num >> i & 1 ^ 1)
- while countZeros > 1 {
- countZeros -= (num >> j & 1 ^ 1)
+ cnt += (num >> i & 1 ^ 1)
+ while cnt > 1 {
+ cnt -= (num >> j & 1 ^ 1)
j += 1
}
ans = max(ans, i - j + 1)
diff --git a/lcci/05.03.Reverse Bits/README_EN.md b/lcci/05.03.Reverse Bits/README_EN.md
index eb68a91114262..a9c88a668e959 100644
--- a/lcci/05.03.Reverse Bits/README_EN.md
+++ b/lcci/05.03.Reverse Bits/README_EN.md
@@ -142,13 +142,13 @@ function reverseBits(num: number): number {
class Solution {
func reverseBits(_ num: Int) -> Int {
var ans = 0
- var countZeros = 0
+ var cnt = 0
var j = 0
for i in 0..<32 {
- countZeros += (num >> i & 1 ^ 1)
- while countZeros > 1 {
- countZeros -= (num >> j & 1 ^ 1)
+ cnt += (num >> i & 1 ^ 1)
+ while cnt > 1 {
+ cnt -= (num >> j & 1 ^ 1)
j += 1
}
ans = max(ans, i - j + 1)
diff --git a/lcci/05.03.Reverse Bits/Solution.swift b/lcci/05.03.Reverse Bits/Solution.swift
index da1c7fe2acf4a..0bd69d4893f51 100644
--- a/lcci/05.03.Reverse Bits/Solution.swift
+++ b/lcci/05.03.Reverse Bits/Solution.swift
@@ -1,13 +1,13 @@
class Solution {
func reverseBits(_ num: Int) -> Int {
var ans = 0
- var countZeros = 0
+ var cnt = 0
var j = 0
for i in 0..<32 {
- countZeros += (num >> i & 1 ^ 1)
- while countZeros > 1 {
- countZeros -= (num >> j & 1 ^ 1)
+ cnt += (num >> i & 1 ^ 1)
+ while cnt > 1 {
+ cnt -= (num >> j & 1 ^ 1)
j += 1
}
ans = max(ans, i - j + 1)
diff --git a/lcci/08.01.Three Steps Problem/README.md b/lcci/08.01.Three Steps Problem/README.md
index 421461ed9d774..93eacbfea15f8 100644
--- a/lcci/08.01.Three Steps Problem/README.md
+++ b/lcci/08.01.Three Steps Problem/README.md
@@ -19,7 +19,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.01.Three%20Steps%2
示例1:
- 输入:n = 3
+ 输入:n = 3
输出:4
说明: 有四种走法
@@ -226,37 +226,6 @@ $$
#### Python3
-```python
-class Solution:
- def waysToStep(self, n: int) -> int:
- mod = 10**9 + 7
-
- def mul(a: List[List[int]], b: List[List[int]]) -> List[List[int]]:
- m, n = len(a), len(b[0])
- c = [[0] * n for _ in range(m)]
- for i in range(m):
- for j in range(n):
- for k in range(len(a[0])):
- c[i][j] = (c[i][j] + a[i][k] * b[k][j] % mod) % mod
- return c
-
- def pow(a: List[List[int]], n: int) -> List[List[int]]:
- res = [[4, 2, 1]]
- while n:
- if n & 1:
- res = mul(res, a)
- n >>= 1
- a = mul(a, a)
- return res
-
- if n < 4:
- return 2 ** (n - 1)
- a = [[1, 1, 0], [1, 0, 1], [1, 0, 0]]
- return sum(pow(a, n - 4)[0]) % mod
-```
-
-#### Python3
-
```python
import numpy as np
@@ -266,8 +235,8 @@ class Solution:
if n < 4:
return 2 ** (n - 1)
mod = 10**9 + 7
- factor = np.mat([(1, 1, 0), (1, 0, 1), (1, 0, 0)], np.dtype("O"))
- res = np.mat([(4, 2, 1)], np.dtype("O"))
+ factor = np.asmatrix([(1, 1, 0), (1, 0, 1), (1, 0, 0)], np.dtype("O"))
+ res = np.asmatrix([(4, 2, 1)], np.dtype("O"))
n -= 4
while n:
if n & 1:
diff --git a/lcci/08.01.Three Steps Problem/README_EN.md b/lcci/08.01.Three Steps Problem/README_EN.md
index 93d93478b8bef..1f10f60a219b3 100644
--- a/lcci/08.01.Three Steps Problem/README_EN.md
+++ b/lcci/08.01.Three Steps Problem/README_EN.md
@@ -20,7 +20,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.01.Three%20Steps%2
- Input: n = 3
+ Input: n = 3
Output: 4
@@ -229,37 +229,6 @@ The time complexity is $O(\log n)$, and the space complexity is $O(1)$.
#### Python3
-```python
-class Solution:
- def waysToStep(self, n: int) -> int:
- mod = 10**9 + 7
-
- def mul(a: List[List[int]], b: List[List[int]]) -> List[List[int]]:
- m, n = len(a), len(b[0])
- c = [[0] * n for _ in range(m)]
- for i in range(m):
- for j in range(n):
- for k in range(len(a[0])):
- c[i][j] = (c[i][j] + a[i][k] * b[k][j] % mod) % mod
- return c
-
- def pow(a: List[List[int]], n: int) -> List[List[int]]:
- res = [[4, 2, 1]]
- while n:
- if n & 1:
- res = mul(res, a)
- n >>= 1
- a = mul(a, a)
- return res
-
- if n < 4:
- return 2 ** (n - 1)
- a = [[1, 1, 0], [1, 0, 1], [1, 0, 0]]
- return sum(pow(a, n - 4)[0]) % mod
-```
-
-#### Python3
-
```python
import numpy as np
@@ -269,8 +238,8 @@ class Solution:
if n < 4:
return 2 ** (n - 1)
mod = 10**9 + 7
- factor = np.mat([(1, 1, 0), (1, 0, 1), (1, 0, 0)], np.dtype("O"))
- res = np.mat([(4, 2, 1)], np.dtype("O"))
+ factor = np.asmatrix([(1, 1, 0), (1, 0, 1), (1, 0, 0)], np.dtype("O"))
+ res = np.asmatrix([(4, 2, 1)], np.dtype("O"))
n -= 4
while n:
if n & 1:
diff --git a/lcci/08.01.Three Steps Problem/Solution2.py b/lcci/08.01.Three Steps Problem/Solution2.py
index 47973c3c6b40d..ce8bd1b06daa3 100644
--- a/lcci/08.01.Three Steps Problem/Solution2.py
+++ b/lcci/08.01.Three Steps Problem/Solution2.py
@@ -1,26 +1,17 @@
-class Solution:
- def waysToStep(self, n: int) -> int:
- mod = 10**9 + 7
-
- def mul(a: List[List[int]], b: List[List[int]]) -> List[List[int]]:
- m, n = len(a), len(b[0])
- c = [[0] * n for _ in range(m)]
- for i in range(m):
- for j in range(n):
- for k in range(len(a[0])):
- c[i][j] = (c[i][j] + a[i][k] * b[k][j] % mod) % mod
- return c
+import numpy as np
- def pow(a: List[List[int]], n: int) -> List[List[int]]:
- res = [[4, 2, 1]]
- while n:
- if n & 1:
- res = mul(res, a)
- n >>= 1
- a = mul(a, a)
- return res
+class Solution:
+ def waysToStep(self, n: int) -> int:
if n < 4:
return 2 ** (n - 1)
- a = [[1, 1, 0], [1, 0, 1], [1, 0, 0]]
- return sum(pow(a, n - 4)[0]) % mod
+ mod = 10**9 + 7
+ factor = np.asmatrix([(1, 1, 0), (1, 0, 1), (1, 0, 0)], np.dtype("O"))
+ res = np.asmatrix([(4, 2, 1)], np.dtype("O"))
+ n -= 4
+ while n:
+ if n & 1:
+ res = res * factor % mod
+ factor = factor * factor % mod
+ n >>= 1
+ return res.sum() % mod
diff --git a/lcci/08.01.Three Steps Problem/Solution3.py b/lcci/08.01.Three Steps Problem/Solution3.py
deleted file mode 100644
index 7ee79117fbbaa..0000000000000
--- a/lcci/08.01.Three Steps Problem/Solution3.py
+++ /dev/null
@@ -1,17 +0,0 @@
-import numpy as np
-
-
-class Solution:
- def waysToStep(self, n: int) -> int:
- if n < 4:
- return 2 ** (n - 1)
- mod = 10**9 + 7
- factor = np.mat([(1, 1, 0), (1, 0, 1), (1, 0, 0)], np.dtype("O"))
- res = np.mat([(4, 2, 1)], np.dtype("O"))
- n -= 4
- while n:
- if n & 1:
- res = res * factor % mod
- factor = factor * factor % mod
- n >>= 1
- return res.sum() % mod
diff --git a/lcci/08.02.Robot in a Grid/README.md b/lcci/08.02.Robot in a Grid/README.md
index cc1bf9f600664..8b60835e73b0c 100644
--- a/lcci/08.02.Robot in a Grid/README.md
+++ b/lcci/08.02.Robot in a Grid/README.md
@@ -15,7 +15,9 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.02.Robot%20in%20a%
设想有个机器人坐在一个网格的左上角,网格 r 行 c 列。机器人只能向下或向右移动,但不能走到一些被禁止的网格(有障碍物)。设计一种算法,寻找机器人从左上角移动到右下角的路径。
+
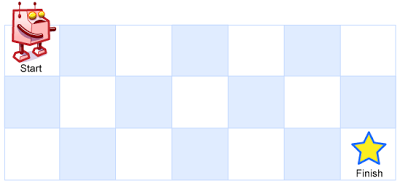
+
网格中的障碍物和空位置分别用 1
和 0
来表示。
返回一条可行的路径,路径由经过的网格的行号和列号组成。左上角为 0 行 0 列。
示例 1:
@@ -26,7 +28,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.02.Robot%20in%20a%
[0,0,0]
]
输出: [[0,0],[0,1],[0,2],[1,2],[2,2]]
-解释:
+解释:
输入中标粗的位置即为输出表示的路径,即
0行0列(左上角) -> 0行1列 -> 0行2列 -> 1行2列 -> 2行2列(右下角)
说明:r 和 c 的值均不超过 100。
@@ -107,7 +109,7 @@ public:
int m = obstacleGrid.size();
int n = obstacleGrid[0].size();
vector> ans;
- function dfs = [&](int i, int j) -> bool {
+ auto dfs = [&](this auto&& dfs, int i, int j) -> bool {
if (i >= m || j >= n || obstacleGrid[i][j] == 1) {
return false;
}
diff --git a/lcci/08.02.Robot in a Grid/README_EN.md b/lcci/08.02.Robot in a Grid/README_EN.md
index 3ba14cfdcd585..bb37b1b1bf1d9 100644
--- a/lcci/08.02.Robot in a Grid/README_EN.md
+++ b/lcci/08.02.Robot in a Grid/README_EN.md
@@ -15,7 +15,9 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.02.Robot%20in%20a%
Imagine a robot sitting on the upper left corner of grid with r rows and c columns. The robot can only move in two directions, right and down, but certain cells are "off limits" such that the robot cannot step on them. Design an algorithm to find a path for the robot from the top left to the bottom right.
+
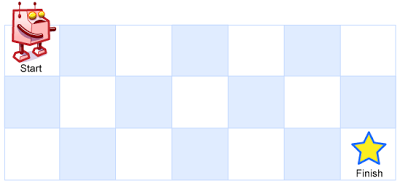
+
"off limits" and empty grid are represented by 1
and 0
respectively.
Return a valid path, consisting of row number and column number of grids in the path.
Example 1:
@@ -116,7 +118,7 @@ public:
int m = obstacleGrid.size();
int n = obstacleGrid[0].size();
vector> ans;
- function dfs = [&](int i, int j) -> bool {
+ auto dfs = [&](this auto&& dfs, int i, int j) -> bool {
if (i >= m || j >= n || obstacleGrid[i][j] == 1) {
return false;
}
diff --git a/lcci/08.02.Robot in a Grid/Solution.cpp b/lcci/08.02.Robot in a Grid/Solution.cpp
index e9657e05ef757..f7daef341d833 100644
--- a/lcci/08.02.Robot in a Grid/Solution.cpp
+++ b/lcci/08.02.Robot in a Grid/Solution.cpp
@@ -4,7 +4,7 @@ class Solution {
int m = obstacleGrid.size();
int n = obstacleGrid[0].size();
vector> ans;
- function dfs = [&](int i, int j) -> bool {
+ auto dfs = [&](this auto&& dfs, int i, int j) -> bool {
if (i >= m || j >= n || obstacleGrid[i][j] == 1) {
return false;
}
@@ -18,4 +18,4 @@ class Solution {
};
return dfs(0, 0) ? ans : vector>();
}
-};
\ No newline at end of file
+};
diff --git a/lcci/08.06.Hanota/README.md b/lcci/08.06.Hanota/README.md
index 04efe8940d384..b5dc3d3966adc 100644
--- a/lcci/08.06.Hanota/README.md
+++ b/lcci/08.06.Hanota/README.md
@@ -91,7 +91,7 @@ class Solution {
class Solution {
public:
void hanota(vector& A, vector& B, vector& C) {
- function&, vector&, vector&)> dfs = [&](int n, vector& a, vector& b, vector& c) {
+ auto dfs = [&](this auto&& dfs, int n, vector& a, vector& b, vector& c) {
if (n == 1) {
c.push_back(a.back());
a.pop_back();
diff --git a/lcci/08.06.Hanota/README_EN.md b/lcci/08.06.Hanota/README_EN.md
index 6d4243950efb0..3f52664c584e4 100644
--- a/lcci/08.06.Hanota/README_EN.md
+++ b/lcci/08.06.Hanota/README_EN.md
@@ -98,7 +98,7 @@ class Solution {
class Solution {
public:
void hanota(vector& A, vector& B, vector& C) {
- function&, vector&, vector&)> dfs = [&](int n, vector& a, vector& b, vector& c) {
+ auto dfs = [&](this auto&& dfs, int n, vector& a, vector& b, vector& c) {
if (n == 1) {
c.push_back(a.back());
a.pop_back();
diff --git a/lcci/08.06.Hanota/Solution.cpp b/lcci/08.06.Hanota/Solution.cpp
index 7cf7ad9647324..3b5b8d483ce8b 100644
--- a/lcci/08.06.Hanota/Solution.cpp
+++ b/lcci/08.06.Hanota/Solution.cpp
@@ -1,7 +1,7 @@
class Solution {
public:
void hanota(vector& A, vector& B, vector& C) {
- function&, vector&, vector&)> dfs = [&](int n, vector& a, vector& b, vector& c) {
+ auto dfs = [&](this auto&& dfs, int n, vector& a, vector& b, vector& c) {
if (n == 1) {
c.push_back(a.back());
a.pop_back();
@@ -14,4 +14,4 @@ class Solution {
};
dfs(A.size(), A, B, C);
}
-};
\ No newline at end of file
+};
diff --git a/lcci/08.07.Permutation I/README.md b/lcci/08.07.Permutation I/README.md
index 34a783fde5bac..9d3e78c2da0d4 100644
--- a/lcci/08.07.Permutation I/README.md
+++ b/lcci/08.07.Permutation I/README.md
@@ -45,7 +45,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.07.Permutation%20I
### 方法一:DFS(回溯)
-我们设计一个函数 $dfs(i)$ 表示已经填完了前 $i$ 个位置,现在需要填第 $i+1$ 个位置。枚举所有可能的字符,如果这个字符没有被填过,就填入这个字符,然后继续填下一个位置,直到填完所有的位置。
+我们设计一个函数 $\textit{dfs}(i)$ 表示已经填完了前 $i$ 个位置,现在需要填第 $i+1$ 个位置。枚举所有可能的字符,如果这个字符没有被填过,就填入这个字符,然后继续填下一个位置,直到填完所有的位置。
时间复杂度 $O(n \times n!)$,其中 $n$ 是字符串的长度。一共有 $n!$ 个排列,每个排列需要 $O(n)$ 的时间来构造。
@@ -57,22 +57,20 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.07.Permutation%20I
class Solution:
def permutation(self, S: str) -> List[str]:
def dfs(i: int):
- if i == n:
+ if i >= n:
ans.append("".join(t))
return
for j, c in enumerate(S):
- if vis[j]:
- continue
- vis[j] = True
- t.append(c)
- dfs(i + 1)
- t.pop()
- vis[j] = False
+ if not vis[j]:
+ vis[j] = True
+ t[i] = c
+ dfs(i + 1)
+ vis[j] = False
+ ans = []
n = len(S)
vis = [False] * n
- ans = []
- t = []
+ t = list(S)
dfs(0)
return ans
```
@@ -82,30 +80,31 @@ class Solution:
```java
class Solution {
private char[] s;
- private boolean[] vis = new boolean['z' + 1];
+ private char[] t;
+ private boolean[] vis;
private List ans = new ArrayList<>();
- private StringBuilder t = new StringBuilder();
public String[] permutation(String S) {
s = S.toCharArray();
+ int n = s.length;
+ vis = new boolean[n];
+ t = new char[n];
dfs(0);
return ans.toArray(new String[0]);
}
private void dfs(int i) {
- if (i == s.length) {
- ans.add(t.toString());
+ if (i >= s.length) {
+ ans.add(new String(t));
return;
}
- for (char c : s) {
- if (vis[c]) {
- continue;
+ for (int j = 0; j < s.length; ++j) {
+ if (!vis[j]) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[c] = true;
- t.append(c);
- dfs(i + 1);
- t.deleteCharAt(t.length() - 1);
- vis[c] = false;
}
}
}
@@ -119,51 +118,49 @@ public:
vector permutation(string S) {
int n = S.size();
vector vis(n);
+ string t = S;
vector ans;
- string t;
- function dfs = [&](int i) {
+ auto dfs = [&](this auto&& dfs, int i) {
if (i >= n) {
- ans.push_back(t);
+ ans.emplace_back(t);
return;
}
for (int j = 0; j < n; ++j) {
- if (vis[j]) {
- continue;
+ if (!vis[j]) {
+ vis[j] = true;
+ t[i] = S[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push_back(S[j]);
- dfs(i + 1);
- t.pop_back();
- vis[j] = false;
}
};
dfs(0);
return ans;
}
};
+
```
#### Go
```go
func permutation(S string) (ans []string) {
- t := []byte{}
- vis := make([]bool, len(S))
+ t := []byte(S)
+ n := len(t)
+ vis := make([]bool, n)
var dfs func(int)
dfs = func(i int) {
- if i >= len(S) {
+ if i >= n {
ans = append(ans, string(t))
return
}
for j := range S {
- if vis[j] {
- continue
+ if !vis[j] {
+ vis[j] = true
+ t[i] = S[j]
+ dfs(i + 1)
+ vis[j] = false
}
- vis[j] = true
- t = append(t, S[j])
- dfs(i + 1)
- t = t[:len(t)-1]
- vis[j] = false
}
}
dfs(0)
@@ -178,7 +175,7 @@ function permutation(S: string): string[] {
const n = S.length;
const vis: boolean[] = Array(n).fill(false);
const ans: string[] = [];
- const t: string[] = [];
+ const t: string[] = Array(n).fill('');
const dfs = (i: number) => {
if (i >= n) {
ans.push(t.join(''));
@@ -189,9 +186,8 @@ function permutation(S: string): string[] {
continue;
}
vis[j] = true;
- t.push(S[j]);
+ t[i] = S[j];
dfs(i + 1);
- t.pop();
vis[j] = false;
}
};
@@ -211,7 +207,7 @@ var permutation = function (S) {
const n = S.length;
const vis = Array(n).fill(false);
const ans = [];
- const t = [];
+ const t = Array(n).fill('');
const dfs = i => {
if (i >= n) {
ans.push(t.join(''));
@@ -222,9 +218,8 @@ var permutation = function (S) {
continue;
}
vis[j] = true;
- t.push(S[j]);
+ t[i] = S[j];
dfs(i + 1);
- t.pop();
vis[j] = false;
}
};
@@ -237,33 +232,30 @@ var permutation = function (S) {
```swift
class Solution {
- private var s: [Character] = []
- private var vis: [Bool] = Array(repeating: false, count: 128)
- private var ans: [String] = []
- private var t: String = ""
-
func permutation(_ S: String) -> [String] {
- s = Array(S)
- dfs(0)
- return ans
- }
-
- private func dfs(_ i: Int) {
- if i == s.count {
- ans.append(t)
- return
- }
- for c in s {
- let index = Int(c.asciiValue!)
- if vis[index] {
- continue
+ var ans: [String] = []
+ let s = Array(S)
+ var t = s
+ var vis = Array(repeating: false, count: s.count)
+ let n = s.count
+
+ func dfs(_ i: Int) {
+ if i >= n {
+ ans.append(String(t))
+ return
+ }
+ for j in 0.. List[str]:
def dfs(i: int):
- if i == n:
+ if i >= n:
ans.append("".join(t))
return
for j, c in enumerate(S):
- if vis[j]:
- continue
- vis[j] = True
- t.append(c)
- dfs(i + 1)
- t.pop()
- vis[j] = False
+ if not vis[j]:
+ vis[j] = True
+ t[i] = c
+ dfs(i + 1)
+ vis[j] = False
+ ans = []
n = len(S)
vis = [False] * n
- ans = []
- t = []
+ t = list(S)
dfs(0)
return ans
```
@@ -88,30 +86,31 @@ class Solution:
```java
class Solution {
private char[] s;
- private boolean[] vis = new boolean['z' + 1];
+ private char[] t;
+ private boolean[] vis;
private List ans = new ArrayList<>();
- private StringBuilder t = new StringBuilder();
public String[] permutation(String S) {
s = S.toCharArray();
+ int n = s.length;
+ vis = new boolean[n];
+ t = new char[n];
dfs(0);
return ans.toArray(new String[0]);
}
private void dfs(int i) {
- if (i == s.length) {
- ans.add(t.toString());
+ if (i >= s.length) {
+ ans.add(new String(t));
return;
}
- for (char c : s) {
- if (vis[c]) {
- continue;
+ for (int j = 0; j < s.length; ++j) {
+ if (!vis[j]) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[c] = true;
- t.append(c);
- dfs(i + 1);
- t.deleteCharAt(t.length() - 1);
- vis[c] = false;
}
}
}
@@ -125,22 +124,20 @@ public:
vector permutation(string S) {
int n = S.size();
vector vis(n);
+ string t = S;
vector ans;
- string t;
- function dfs = [&](int i) {
+ auto dfs = [&](this auto&& dfs, int i) {
if (i >= n) {
- ans.push_back(t);
+ ans.emplace_back(t);
return;
}
for (int j = 0; j < n; ++j) {
- if (vis[j]) {
- continue;
+ if (!vis[j]) {
+ vis[j] = true;
+ t[i] = S[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push_back(S[j]);
- dfs(i + 1);
- t.pop_back();
- vis[j] = false;
}
};
dfs(0);
@@ -153,23 +150,22 @@ public:
```go
func permutation(S string) (ans []string) {
- t := []byte{}
- vis := make([]bool, len(S))
+ t := []byte(S)
+ n := len(t)
+ vis := make([]bool, n)
var dfs func(int)
dfs = func(i int) {
- if i >= len(S) {
+ if i >= n {
ans = append(ans, string(t))
return
}
for j := range S {
- if vis[j] {
- continue
+ if !vis[j] {
+ vis[j] = true
+ t[i] = S[j]
+ dfs(i + 1)
+ vis[j] = false
}
- vis[j] = true
- t = append(t, S[j])
- dfs(i + 1)
- t = t[:len(t)-1]
- vis[j] = false
}
}
dfs(0)
@@ -184,7 +180,7 @@ function permutation(S: string): string[] {
const n = S.length;
const vis: boolean[] = Array(n).fill(false);
const ans: string[] = [];
- const t: string[] = [];
+ const t: string[] = Array(n).fill('');
const dfs = (i: number) => {
if (i >= n) {
ans.push(t.join(''));
@@ -195,9 +191,8 @@ function permutation(S: string): string[] {
continue;
}
vis[j] = true;
- t.push(S[j]);
+ t[i] = S[j];
dfs(i + 1);
- t.pop();
vis[j] = false;
}
};
@@ -217,7 +212,7 @@ var permutation = function (S) {
const n = S.length;
const vis = Array(n).fill(false);
const ans = [];
- const t = [];
+ const t = Array(n).fill('');
const dfs = i => {
if (i >= n) {
ans.push(t.join(''));
@@ -228,9 +223,8 @@ var permutation = function (S) {
continue;
}
vis[j] = true;
- t.push(S[j]);
+ t[i] = S[j];
dfs(i + 1);
- t.pop();
vis[j] = false;
}
};
@@ -243,33 +237,30 @@ var permutation = function (S) {
```swift
class Solution {
- private var s: [Character] = []
- private var vis: [Bool] = Array(repeating: false, count: 128)
- private var ans: [String] = []
- private var t: String = ""
-
func permutation(_ S: String) -> [String] {
- s = Array(S)
- dfs(0)
- return ans
- }
-
- private func dfs(_ i: Int) {
- if i == s.count {
- ans.append(t)
- return
- }
- for c in s {
- let index = Int(c.asciiValue!)
- if vis[index] {
- continue
+ var ans: [String] = []
+ let s = Array(S)
+ var t = s
+ var vis = Array(repeating: false, count: s.count)
+ let n = s.count
+
+ func dfs(_ i: Int) {
+ if i >= n {
+ ans.append(String(t))
+ return
+ }
+ for j in 0.. permutation(string S) {
int n = S.size();
vector vis(n);
+ string t = S;
vector ans;
- string t;
- function dfs = [&](int i) {
+ auto dfs = [&](this auto&& dfs, int i) {
if (i >= n) {
- ans.push_back(t);
+ ans.emplace_back(t);
return;
}
for (int j = 0; j < n; ++j) {
- if (vis[j]) {
- continue;
+ if (!vis[j]) {
+ vis[j] = true;
+ t[i] = S[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push_back(S[j]);
- dfs(i + 1);
- t.pop_back();
- vis[j] = false;
}
};
dfs(0);
return ans;
}
-};
\ No newline at end of file
+};
diff --git a/lcci/08.07.Permutation I/Solution.go b/lcci/08.07.Permutation I/Solution.go
index 20d5c55fded5f..1f84fb692481e 100644
--- a/lcci/08.07.Permutation I/Solution.go
+++ b/lcci/08.07.Permutation I/Solution.go
@@ -1,23 +1,22 @@
func permutation(S string) (ans []string) {
- t := []byte{}
- vis := make([]bool, len(S))
+ t := []byte(S)
+ n := len(t)
+ vis := make([]bool, n)
var dfs func(int)
dfs = func(i int) {
- if i >= len(S) {
+ if i >= n {
ans = append(ans, string(t))
return
}
for j := range S {
- if vis[j] {
- continue
+ if !vis[j] {
+ vis[j] = true
+ t[i] = S[j]
+ dfs(i + 1)
+ vis[j] = false
}
- vis[j] = true
- t = append(t, S[j])
- dfs(i + 1)
- t = t[:len(t)-1]
- vis[j] = false
}
}
dfs(0)
return
-}
\ No newline at end of file
+}
diff --git a/lcci/08.07.Permutation I/Solution.java b/lcci/08.07.Permutation I/Solution.java
index 896ed99f9d1ea..f65456f945759 100644
--- a/lcci/08.07.Permutation I/Solution.java
+++ b/lcci/08.07.Permutation I/Solution.java
@@ -1,29 +1,30 @@
class Solution {
private char[] s;
- private boolean[] vis = new boolean['z' + 1];
+ private char[] t;
+ private boolean[] vis;
private List ans = new ArrayList<>();
- private StringBuilder t = new StringBuilder();
public String[] permutation(String S) {
s = S.toCharArray();
+ int n = s.length;
+ vis = new boolean[n];
+ t = new char[n];
dfs(0);
return ans.toArray(new String[0]);
}
private void dfs(int i) {
- if (i == s.length) {
- ans.add(t.toString());
+ if (i >= s.length) {
+ ans.add(new String(t));
return;
}
- for (char c : s) {
- if (vis[c]) {
- continue;
+ for (int j = 0; j < s.length; ++j) {
+ if (!vis[j]) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[c] = true;
- t.append(c);
- dfs(i + 1);
- t.deleteCharAt(t.length() - 1);
- vis[c] = false;
}
}
-}
\ No newline at end of file
+}
diff --git a/lcci/08.07.Permutation I/Solution.js b/lcci/08.07.Permutation I/Solution.js
index f1dcb5d98f69f..c18ebd79c017d 100644
--- a/lcci/08.07.Permutation I/Solution.js
+++ b/lcci/08.07.Permutation I/Solution.js
@@ -6,7 +6,7 @@ var permutation = function (S) {
const n = S.length;
const vis = Array(n).fill(false);
const ans = [];
- const t = [];
+ const t = Array(n).fill('');
const dfs = i => {
if (i >= n) {
ans.push(t.join(''));
@@ -17,9 +17,8 @@ var permutation = function (S) {
continue;
}
vis[j] = true;
- t.push(S[j]);
+ t[i] = S[j];
dfs(i + 1);
- t.pop();
vis[j] = false;
}
};
diff --git a/lcci/08.07.Permutation I/Solution.py b/lcci/08.07.Permutation I/Solution.py
index 537c2f0484bb7..7e64e799f8691 100644
--- a/lcci/08.07.Permutation I/Solution.py
+++ b/lcci/08.07.Permutation I/Solution.py
@@ -1,21 +1,19 @@
class Solution:
def permutation(self, S: str) -> List[str]:
def dfs(i: int):
- if i == n:
+ if i >= n:
ans.append("".join(t))
return
for j, c in enumerate(S):
- if vis[j]:
- continue
- vis[j] = True
- t.append(c)
- dfs(i + 1)
- t.pop()
- vis[j] = False
+ if not vis[j]:
+ vis[j] = True
+ t[i] = c
+ dfs(i + 1)
+ vis[j] = False
+ ans = []
n = len(S)
vis = [False] * n
- ans = []
- t = []
+ t = list(S)
dfs(0)
return ans
diff --git a/lcci/08.07.Permutation I/Solution.swift b/lcci/08.07.Permutation I/Solution.swift
index 48803e420ab09..33a987fc012d9 100644
--- a/lcci/08.07.Permutation I/Solution.swift
+++ b/lcci/08.07.Permutation I/Solution.swift
@@ -1,30 +1,27 @@
class Solution {
- private var s: [Character] = []
- private var vis: [Bool] = Array(repeating: false, count: 128)
- private var ans: [String] = []
- private var t: String = ""
-
func permutation(_ S: String) -> [String] {
- s = Array(S)
- dfs(0)
- return ans
- }
+ var ans: [String] = []
+ let s = Array(S)
+ var t = s
+ var vis = Array(repeating: false, count: s.count)
+ let n = s.count
- private func dfs(_ i: Int) {
- if i == s.count {
- ans.append(t)
- return
- }
- for c in s {
- let index = Int(c.asciiValue!)
- if vis[index] {
- continue
+ func dfs(_ i: Int) {
+ if i >= n {
+ ans.append(String(t))
+ return
+ }
+ for j in 0.. {
if (i >= n) {
ans.push(t.join(''));
@@ -13,9 +13,8 @@ function permutation(S: string): string[] {
continue;
}
vis[j] = true;
- t.push(S[j]);
+ t[i] = S[j];
dfs(i + 1);
- t.pop();
vis[j] = false;
}
};
diff --git a/lcci/08.08.Permutation II/README.md b/lcci/08.08.Permutation II/README.md
index 90b72d44fb2f1..936fb1b9d90cf 100644
--- a/lcci/08.08.Permutation II/README.md
+++ b/lcci/08.08.Permutation II/README.md
@@ -39,12 +39,12 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.08.Permutation%20I
我们可以先对字符串按照字符进行排序,这样就可以将重复的字符放在一起,方便我们进行去重。
-然后,我们设计一个函数 $dfs(i)$,表示当前需要填写第 $i$ 个位置的字符。函数的具体实现如下:
+然后,我们设计一个函数 $\textit{dfs}(i)$,表示当前需要填写第 $i$ 个位置的字符。函数的具体实现如下:
- 如果 $i = n$,说明我们已经填写完毕,将当前排列加入答案数组中,然后返回。
-- 否则,我们枚举第 $i$ 个位置的字符 $s[j]$,其中 $j$ 的范围是 $[0, n - 1]$。我们需要保证 $s[j]$ 没有被使用过,并且与前面枚举的字符不同,这样才能保证当前排列不重复。如果满足条件,我们就可以填写 $s[j]$,并继续递归地填写下一个位置,即调用 $dfs(i + 1)$。在递归调用结束后,我们需要将 $s[j]$ 标记为未使用,以便于进行后面的枚举。
+- 否则,我们枚举第 $i$ 个位置的字符 $\textit{s}[j]$,其中 $j$ 的范围是 $[0, n - 1]$。我们需要保证 $\textit{s}[j]$ 没有被使用过,并且与前面枚举的字符不同,这样才能保证当前排列不重复。如果满足条件,我们就可以填写 $\textit{s}[j]$,并继续递归地填写下一个位置,即调用 $\textit{dfs}(i + 1)$。在递归调用结束后,我们需要将 $\textit{s}[j]$ 标记为未使用,以便于进行后面的枚举。
-在主函数中,我们首先对字符串进行排序,然后调用 $dfs(0)$,即从第 $0$ 个位置开始填写,最终返回答案数组即可。
+在主函数中,我们首先对字符串进行排序,然后调用 $\textit{dfs}(0)$,即从第 $0$ 个位置开始填写,最终返回答案数组即可。
时间复杂度 $O(n \times n!)$,空间复杂度 $O(n)$。其中 $n$ 是字符串 $s$ 的长度。需要进行 $n!$ 次枚举,每次枚举需要 $O(n)$ 的时间来判断是否重复。另外,我们需要一个标记数组来标记每个位置是否被使用过,因此空间复杂度为 $O(n)$。
@@ -56,21 +56,20 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/08.08.Permutation%20I
class Solution:
def permutation(self, S: str) -> List[str]:
def dfs(i: int):
- if i == n:
+ if i >= n:
ans.append("".join(t))
return
- for j in range(n):
- if vis[j] or (j and cs[j] == cs[j - 1] and not vis[j - 1]):
- continue
- t[i] = cs[j]
- vis[j] = True
- dfs(i + 1)
- vis[j] = False
-
- cs = sorted(S)
- n = len(cs)
+ for j, c in enumerate(s):
+ if not vis[j] and (j == 0 or s[j] != s[j - 1] or vis[j - 1]):
+ vis[j] = True
+ t[i] = c
+ dfs(i + 1)
+ vis[j] = False
+
+ s = sorted(S)
ans = []
- t = [None] * n
+ t = s[:]
+ n = len(s)
vis = [False] * n
dfs(0)
return ans
@@ -80,35 +79,33 @@ class Solution:
```java
class Solution {
- private int n;
- private char[] cs;
- private List ans = new ArrayList<>();
+ private char[] s;
+ private char[] t;
private boolean[] vis;
- private StringBuilder t = new StringBuilder();
+ private List ans = new ArrayList<>();
public String[] permutation(String S) {
- cs = S.toCharArray();
- n = cs.length;
- Arrays.sort(cs);
+ int n = S.length();
+ s = S.toCharArray();
+ Arrays.sort(s);
+ t = new char[n];
vis = new boolean[n];
dfs(0);
return ans.toArray(new String[0]);
}
private void dfs(int i) {
- if (i == n) {
- ans.add(t.toString());
+ if (i >= s.length) {
+ ans.add(new String(t));
return;
}
- for (int j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] == cs[j - 1])) {
- continue;
+ for (int j = 0; j < s.length; ++j) {
+ if (!vis[j] && (j == 0 || s[j] != s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.append(cs[j]);
- dfs(i + 1);
- t.deleteCharAt(t.length() - 1);
- vis[j] = false;
}
}
}
@@ -120,26 +117,23 @@ class Solution {
class Solution {
public:
vector permutation(string S) {
- vector cs(S.begin(), S.end());
- sort(cs.begin(), cs.end());
- int n = cs.size();
- vector ans;
+ ranges::sort(S);
+ string t = S;
+ int n = t.size();
vector vis(n);
- string t;
- function dfs = [&](int i) {
- if (i == n) {
- ans.push_back(t);
+ vector ans;
+ auto dfs = [&](this auto&& dfs, int i) {
+ if (i >= n) {
+ ans.emplace_back(t);
return;
}
for (int j = 0; j < n; ++j) {
- if (vis[j] || (j && !vis[j - 1] && cs[j] == cs[j - 1])) {
- continue;
+ if (!vis[j] && (j == 0 || S[j] != S[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = S[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push_back(cs[j]);
- dfs(i + 1);
- t.pop_back();
- vis[j] = false;
}
};
dfs(0);
@@ -152,26 +146,23 @@ public:
```go
func permutation(S string) (ans []string) {
- cs := []byte(S)
- sort.Slice(cs, func(i, j int) bool { return cs[i] < cs[j] })
- t := []byte{}
- n := len(cs)
- vis := make([]bool, n)
+ s := []byte(S)
+ sort.Slice(s, func(i, j int) bool { return s[i] < s[j] })
+ t := slices.Clone(s)
+ vis := make([]bool, len(s))
var dfs func(int)
dfs = func(i int) {
- if i == n {
+ if i >= len(s) {
ans = append(ans, string(t))
return
}
- for j := 0; j < n; j++ {
- if vis[j] || (j > 0 && !vis[j-1] && cs[j] == cs[j-1]) {
- continue
+ for j := range s {
+ if !vis[j] && (j == 0 || s[j] != s[j-1] || vis[j-1]) {
+ vis[j] = true
+ t[i] = s[j]
+ dfs(i + 1)
+ vis[j] = false
}
- vis[j] = true
- t = append(t, cs[j])
- dfs(i + 1)
- t = t[:len(t)-1]
- vis[j] = false
}
}
dfs(0)
@@ -183,25 +174,23 @@ func permutation(S string) (ans []string) {
```ts
function permutation(S: string): string[] {
- const cs: string[] = S.split('').sort();
- const ans: string[] = [];
- const n = cs.length;
+ const s: string[] = S.split('').sort();
+ const n = s.length;
+ const t = Array(n).fill('');
const vis: boolean[] = Array(n).fill(false);
- const t: string[] = [];
+ const ans: string[] = [];
const dfs = (i: number) => {
- if (i === n) {
+ if (i >= n) {
ans.push(t.join(''));
return;
}
for (let j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] === cs[j - 1])) {
- continue;
+ if (!vis[j] && (j === 0 || s[j] !== s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push(cs[j]);
- dfs(i + 1);
- t.pop();
- vis[j] = false;
}
};
dfs(0);
@@ -217,25 +206,23 @@ function permutation(S: string): string[] {
* @return {string[]}
*/
var permutation = function (S) {
- const cs = S.split('').sort();
- const ans = [];
- const n = cs.length;
+ const s = S.split('').sort();
+ const n = s.length;
+ const t = Array(n).fill('');
const vis = Array(n).fill(false);
- const t = [];
+ const ans = [];
const dfs = i => {
- if (i === n) {
+ if (i >= n) {
ans.push(t.join(''));
return;
}
for (let j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] === cs[j - 1])) {
- continue;
+ if (!vis[j] && (j === 0 || s[j] !== s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push(cs[j]);
- dfs(i + 1);
- t.pop();
- vis[j] = false;
}
};
dfs(0);
@@ -247,36 +234,30 @@ var permutation = function (S) {
```swift
class Solution {
- private var n: Int = 0
- private var cs: [Character] = []
- private var ans: [String] = []
- private var vis: [Bool] = []
- private var t: String = ""
-
func permutation(_ S: String) -> [String] {
- cs = Array(S)
- n = cs.count
- cs.sort()
- vis = Array(repeating: false, count: n)
- dfs(0)
- return ans
- }
-
- private func dfs(_ i: Int) {
- if i == n {
- ans.append(t)
- return
- }
- for j in 0.. 0 && !vis[j - 1] && cs[j] == cs[j - 1]) {
- continue
+ var ans: [String] = []
+ var s: [Character] = Array(S).sorted()
+ var t: [Character] = Array(repeating: " ", count: s.count)
+ var vis: [Bool] = Array(repeating: false, count: s.count)
+ let n = s.count
+
+ func dfs(_ i: Int) {
+ if i >= n {
+ ans.append(String(t))
+ return
+ }
+ for j in 0..
@@ -64,21 +64,20 @@ The time complexity is $O(n \times n!)$, and the space complexity is $O(n)$. Her
class Solution:
def permutation(self, S: str) -> List[str]:
def dfs(i: int):
- if i == n:
+ if i >= n:
ans.append("".join(t))
return
- for j in range(n):
- if vis[j] or (j and cs[j] == cs[j - 1] and not vis[j - 1]):
- continue
- t[i] = cs[j]
- vis[j] = True
- dfs(i + 1)
- vis[j] = False
-
- cs = sorted(S)
- n = len(cs)
+ for j, c in enumerate(s):
+ if not vis[j] and (j == 0 or s[j] != s[j - 1] or vis[j - 1]):
+ vis[j] = True
+ t[i] = c
+ dfs(i + 1)
+ vis[j] = False
+
+ s = sorted(S)
ans = []
- t = [None] * n
+ t = s[:]
+ n = len(s)
vis = [False] * n
dfs(0)
return ans
@@ -88,35 +87,33 @@ class Solution:
```java
class Solution {
- private int n;
- private char[] cs;
- private List ans = new ArrayList<>();
+ private char[] s;
+ private char[] t;
private boolean[] vis;
- private StringBuilder t = new StringBuilder();
+ private List ans = new ArrayList<>();
public String[] permutation(String S) {
- cs = S.toCharArray();
- n = cs.length;
- Arrays.sort(cs);
+ int n = S.length();
+ s = S.toCharArray();
+ Arrays.sort(s);
+ t = new char[n];
vis = new boolean[n];
dfs(0);
return ans.toArray(new String[0]);
}
private void dfs(int i) {
- if (i == n) {
- ans.add(t.toString());
+ if (i >= s.length) {
+ ans.add(new String(t));
return;
}
- for (int j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] == cs[j - 1])) {
- continue;
+ for (int j = 0; j < s.length; ++j) {
+ if (!vis[j] && (j == 0 || s[j] != s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.append(cs[j]);
- dfs(i + 1);
- t.deleteCharAt(t.length() - 1);
- vis[j] = false;
}
}
}
@@ -128,26 +125,23 @@ class Solution {
class Solution {
public:
vector permutation(string S) {
- vector cs(S.begin(), S.end());
- sort(cs.begin(), cs.end());
- int n = cs.size();
- vector ans;
+ ranges::sort(S);
+ string t = S;
+ int n = t.size();
vector vis(n);
- string t;
- function dfs = [&](int i) {
- if (i == n) {
- ans.push_back(t);
+ vector ans;
+ auto dfs = [&](this auto&& dfs, int i) {
+ if (i >= n) {
+ ans.emplace_back(t);
return;
}
for (int j = 0; j < n; ++j) {
- if (vis[j] || (j && !vis[j - 1] && cs[j] == cs[j - 1])) {
- continue;
+ if (!vis[j] && (j == 0 || S[j] != S[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = S[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push_back(cs[j]);
- dfs(i + 1);
- t.pop_back();
- vis[j] = false;
}
};
dfs(0);
@@ -160,26 +154,23 @@ public:
```go
func permutation(S string) (ans []string) {
- cs := []byte(S)
- sort.Slice(cs, func(i, j int) bool { return cs[i] < cs[j] })
- t := []byte{}
- n := len(cs)
- vis := make([]bool, n)
+ s := []byte(S)
+ sort.Slice(s, func(i, j int) bool { return s[i] < s[j] })
+ t := slices.Clone(s)
+ vis := make([]bool, len(s))
var dfs func(int)
dfs = func(i int) {
- if i == n {
+ if i >= len(s) {
ans = append(ans, string(t))
return
}
- for j := 0; j < n; j++ {
- if vis[j] || (j > 0 && !vis[j-1] && cs[j] == cs[j-1]) {
- continue
+ for j := range s {
+ if !vis[j] && (j == 0 || s[j] != s[j-1] || vis[j-1]) {
+ vis[j] = true
+ t[i] = s[j]
+ dfs(i + 1)
+ vis[j] = false
}
- vis[j] = true
- t = append(t, cs[j])
- dfs(i + 1)
- t = t[:len(t)-1]
- vis[j] = false
}
}
dfs(0)
@@ -191,25 +182,23 @@ func permutation(S string) (ans []string) {
```ts
function permutation(S: string): string[] {
- const cs: string[] = S.split('').sort();
- const ans: string[] = [];
- const n = cs.length;
+ const s: string[] = S.split('').sort();
+ const n = s.length;
+ const t = Array(n).fill('');
const vis: boolean[] = Array(n).fill(false);
- const t: string[] = [];
+ const ans: string[] = [];
const dfs = (i: number) => {
- if (i === n) {
+ if (i >= n) {
ans.push(t.join(''));
return;
}
for (let j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] === cs[j - 1])) {
- continue;
+ if (!vis[j] && (j === 0 || s[j] !== s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push(cs[j]);
- dfs(i + 1);
- t.pop();
- vis[j] = false;
}
};
dfs(0);
@@ -225,25 +214,23 @@ function permutation(S: string): string[] {
* @return {string[]}
*/
var permutation = function (S) {
- const cs = S.split('').sort();
- const ans = [];
- const n = cs.length;
+ const s = S.split('').sort();
+ const n = s.length;
+ const t = Array(n).fill('');
const vis = Array(n).fill(false);
- const t = [];
+ const ans = [];
const dfs = i => {
- if (i === n) {
+ if (i >= n) {
ans.push(t.join(''));
return;
}
for (let j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] === cs[j - 1])) {
- continue;
+ if (!vis[j] && (j === 0 || s[j] !== s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push(cs[j]);
- dfs(i + 1);
- t.pop();
- vis[j] = false;
}
};
dfs(0);
@@ -255,36 +242,30 @@ var permutation = function (S) {
```swift
class Solution {
- private var n: Int = 0
- private var cs: [Character] = []
- private var ans: [String] = []
- private var vis: [Bool] = []
- private var t: String = ""
-
func permutation(_ S: String) -> [String] {
- cs = Array(S)
- n = cs.count
- cs.sort()
- vis = Array(repeating: false, count: n)
- dfs(0)
- return ans
- }
-
- private func dfs(_ i: Int) {
- if i == n {
- ans.append(t)
- return
- }
- for j in 0.. 0 && !vis[j - 1] && cs[j] == cs[j - 1]) {
- continue
+ var ans: [String] = []
+ var s: [Character] = Array(S).sorted()
+ var t: [Character] = Array(repeating: " ", count: s.count)
+ var vis: [Bool] = Array(repeating: false, count: s.count)
+ let n = s.count
+
+ func dfs(_ i: Int) {
+ if i >= n {
+ ans.append(String(t))
+ return
+ }
+ for j in 0.. permutation(string S) {
- vector cs(S.begin(), S.end());
- sort(cs.begin(), cs.end());
- int n = cs.size();
- vector ans;
+ ranges::sort(S);
+ string t = S;
+ int n = t.size();
vector vis(n);
- string t;
- function dfs = [&](int i) {
- if (i == n) {
- ans.push_back(t);
+ vector ans;
+ auto dfs = [&](this auto&& dfs, int i) {
+ if (i >= n) {
+ ans.emplace_back(t);
return;
}
for (int j = 0; j < n; ++j) {
- if (vis[j] || (j && !vis[j - 1] && cs[j] == cs[j - 1])) {
- continue;
+ if (!vis[j] && (j == 0 || S[j] != S[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = S[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push_back(cs[j]);
- dfs(i + 1);
- t.pop_back();
- vis[j] = false;
}
};
dfs(0);
return ans;
}
-};
\ No newline at end of file
+};
diff --git a/lcci/08.08.Permutation II/Solution.go b/lcci/08.08.Permutation II/Solution.go
index bdb449b7c9389..ce2be679b7676 100644
--- a/lcci/08.08.Permutation II/Solution.go
+++ b/lcci/08.08.Permutation II/Solution.go
@@ -1,26 +1,23 @@
func permutation(S string) (ans []string) {
- cs := []byte(S)
- sort.Slice(cs, func(i, j int) bool { return cs[i] < cs[j] })
- t := []byte{}
- n := len(cs)
- vis := make([]bool, n)
+ s := []byte(S)
+ sort.Slice(s, func(i, j int) bool { return s[i] < s[j] })
+ t := slices.Clone(s)
+ vis := make([]bool, len(s))
var dfs func(int)
dfs = func(i int) {
- if i == n {
+ if i >= len(s) {
ans = append(ans, string(t))
return
}
- for j := 0; j < n; j++ {
- if vis[j] || (j > 0 && !vis[j-1] && cs[j] == cs[j-1]) {
- continue
+ for j := range s {
+ if !vis[j] && (j == 0 || s[j] != s[j-1] || vis[j-1]) {
+ vis[j] = true
+ t[i] = s[j]
+ dfs(i + 1)
+ vis[j] = false
}
- vis[j] = true
- t = append(t, cs[j])
- dfs(i + 1)
- t = t[:len(t)-1]
- vis[j] = false
}
}
dfs(0)
return
-}
\ No newline at end of file
+}
diff --git a/lcci/08.08.Permutation II/Solution.java b/lcci/08.08.Permutation II/Solution.java
index e0dffd2d0cfae..b03452a42cd0d 100644
--- a/lcci/08.08.Permutation II/Solution.java
+++ b/lcci/08.08.Permutation II/Solution.java
@@ -1,33 +1,31 @@
class Solution {
- private int n;
- private char[] cs;
- private List ans = new ArrayList<>();
+ private char[] s;
+ private char[] t;
private boolean[] vis;
- private StringBuilder t = new StringBuilder();
+ private List ans = new ArrayList<>();
public String[] permutation(String S) {
- cs = S.toCharArray();
- n = cs.length;
- Arrays.sort(cs);
+ int n = S.length();
+ s = S.toCharArray();
+ Arrays.sort(s);
+ t = new char[n];
vis = new boolean[n];
dfs(0);
return ans.toArray(new String[0]);
}
private void dfs(int i) {
- if (i == n) {
- ans.add(t.toString());
+ if (i >= s.length) {
+ ans.add(new String(t));
return;
}
- for (int j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] == cs[j - 1])) {
- continue;
+ for (int j = 0; j < s.length; ++j) {
+ if (!vis[j] && (j == 0 || s[j] != s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.append(cs[j]);
- dfs(i + 1);
- t.deleteCharAt(t.length() - 1);
- vis[j] = false;
}
}
-}
\ No newline at end of file
+}
diff --git a/lcci/08.08.Permutation II/Solution.js b/lcci/08.08.Permutation II/Solution.js
index 44aed97be109c..4bb6b59170d9f 100644
--- a/lcci/08.08.Permutation II/Solution.js
+++ b/lcci/08.08.Permutation II/Solution.js
@@ -3,25 +3,23 @@
* @return {string[]}
*/
var permutation = function (S) {
- const cs = S.split('').sort();
- const ans = [];
- const n = cs.length;
+ const s = S.split('').sort();
+ const n = s.length;
+ const t = Array(n).fill('');
const vis = Array(n).fill(false);
- const t = [];
+ const ans = [];
const dfs = i => {
- if (i === n) {
+ if (i >= n) {
ans.push(t.join(''));
return;
}
for (let j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] === cs[j - 1])) {
- continue;
+ if (!vis[j] && (j === 0 || s[j] !== s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push(cs[j]);
- dfs(i + 1);
- t.pop();
- vis[j] = false;
}
};
dfs(0);
diff --git a/lcci/08.08.Permutation II/Solution.py b/lcci/08.08.Permutation II/Solution.py
index e6b19ccba395a..e39d27633f10d 100644
--- a/lcci/08.08.Permutation II/Solution.py
+++ b/lcci/08.08.Permutation II/Solution.py
@@ -1,21 +1,20 @@
class Solution:
def permutation(self, S: str) -> List[str]:
def dfs(i: int):
- if i == n:
+ if i >= n:
ans.append("".join(t))
return
- for j in range(n):
- if vis[j] or (j and cs[j] == cs[j - 1] and not vis[j - 1]):
- continue
- t[i] = cs[j]
- vis[j] = True
- dfs(i + 1)
- vis[j] = False
+ for j, c in enumerate(s):
+ if not vis[j] and (j == 0 or s[j] != s[j - 1] or vis[j - 1]):
+ vis[j] = True
+ t[i] = c
+ dfs(i + 1)
+ vis[j] = False
- cs = sorted(S)
- n = len(cs)
+ s = sorted(S)
ans = []
- t = [None] * n
+ t = s[:]
+ n = len(s)
vis = [False] * n
dfs(0)
return ans
diff --git a/lcci/08.08.Permutation II/Solution.swift b/lcci/08.08.Permutation II/Solution.swift
index a00ac743ff082..84151d360dc86 100644
--- a/lcci/08.08.Permutation II/Solution.swift
+++ b/lcci/08.08.Permutation II/Solution.swift
@@ -1,33 +1,27 @@
class Solution {
- private var n: Int = 0
- private var cs: [Character] = []
- private var ans: [String] = []
- private var vis: [Bool] = []
- private var t: String = ""
-
func permutation(_ S: String) -> [String] {
- cs = Array(S)
- n = cs.count
- cs.sort()
- vis = Array(repeating: false, count: n)
- dfs(0)
- return ans
- }
+ var ans: [String] = []
+ var s: [Character] = Array(S).sorted()
+ var t: [Character] = Array(repeating: " ", count: s.count)
+ var vis: [Bool] = Array(repeating: false, count: s.count)
+ let n = s.count
- private func dfs(_ i: Int) {
- if i == n {
- ans.append(t)
- return
- }
- for j in 0.. 0 && !vis[j - 1] && cs[j] == cs[j - 1]) {
- continue
+ func dfs(_ i: Int) {
+ if i >= n {
+ ans.append(String(t))
+ return
+ }
+ for j in 0.. {
- if (i === n) {
+ if (i >= n) {
ans.push(t.join(''));
return;
}
for (let j = 0; j < n; ++j) {
- if (vis[j] || (j > 0 && !vis[j - 1] && cs[j] === cs[j - 1])) {
- continue;
+ if (!vis[j] && (j === 0 || s[j] !== s[j - 1] || vis[j - 1])) {
+ vis[j] = true;
+ t[i] = s[j];
+ dfs(i + 1);
+ vis[j] = false;
}
- vis[j] = true;
- t.push(cs[j]);
- dfs(i + 1);
- t.pop();
- vis[j] = false;
}
};
dfs(0);
diff --git a/lcci/08.09.Bracket/README.md b/lcci/08.09.Bracket/README.md
index 6e4a46e5769b9..7272118acce4c 100644
--- a/lcci/08.09.Bracket/README.md
+++ b/lcci/08.09.Bracket/README.md
@@ -104,8 +104,7 @@ class Solution {
public:
vector generateParenthesis(int n) {
vector ans;
- function dfs;
- dfs = [&](int l, int r, string t) {
+ auto dfs = [&](this auto&& dfs, int l, int r, string t) {
if (l > n || r > n || l < r) return;
if (l == n && r == n) {
ans.push_back(t);
diff --git a/lcci/08.09.Bracket/README_EN.md b/lcci/08.09.Bracket/README_EN.md
index 295bd24efe68f..36ee966e887ab 100644
--- a/lcci/08.09.Bracket/README_EN.md
+++ b/lcci/08.09.Bracket/README_EN.md
@@ -112,8 +112,7 @@ class Solution {
public:
vector generateParenthesis(int n) {
vector ans;
- function dfs;
- dfs = [&](int l, int r, string t) {
+ auto dfs = [&](this auto&& dfs, int l, int r, string t) {
if (l > n || r > n || l < r) return;
if (l == n && r == n) {
ans.push_back(t);
diff --git a/lcci/08.09.Bracket/Solution.cpp b/lcci/08.09.Bracket/Solution.cpp
index 4c9a371b251c4..d386301341d2c 100644
--- a/lcci/08.09.Bracket/Solution.cpp
+++ b/lcci/08.09.Bracket/Solution.cpp
@@ -2,8 +2,7 @@ class Solution {
public:
vector generateParenthesis(int n) {
vector ans;
- function dfs;
- dfs = [&](int l, int r, string t) {
+ auto dfs = [&](this auto&& dfs, int l, int r, string t) {
if (l > n || r > n || l < r) return;
if (l == n && r == n) {
ans.push_back(t);
@@ -15,4 +14,4 @@ class Solution {
dfs(0, 0, "");
return ans;
}
-};
\ No newline at end of file
+};
diff --git a/lcci/17.15.Longest Word/README.md b/lcci/17.15.Longest Word/README.md
index a9840705c9c6a..0f6dffe5c3aa8 100644
--- a/lcci/17.15.Longest Word/README.md
+++ b/lcci/17.15.Longest Word/README.md
@@ -32,117 +32,79 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/17.15.Longest%20Word/
-### 方法一:前缀树 + DFS
+### 方法一:哈希表 + 排序 + DFS
+
+注意,题目中,每个单词实际上允许重复使用。
+
+我们可以用一个哈希表 $\textit{s}$ 存储所有单词,然后对单词按照长度降序排序,如果长度相同,按照字典序升序排序。
+
+接下来,我们遍历排序后的单词列表,对于每个单词 $\textit{w}$,我们先将其从哈希表 $\textit{s}$ 中移除,然后使用深度优先搜索 $\textit{dfs}$ 判断 $\textit{w}$ 是否可以由其他单词组成,如果可以,返回 $\textit{w}$。
+
+函数 $\textit{dfs}$ 的执行逻辑如下:
+
+- 如果 $\textit{w}$ 为空,返回 $\text{true}$;
+- 遍历 $\textit{w}$ 的所有前缀,如果前缀在哈希表 $\textit{s}$ 中且 $\textit{dfs}$ 返回 $\text{true}$,则返回 $\text{true}$;
+- 如果没有符合条件的前缀,返回 $\text{false}$。
+
+如果没有找到符合条件的单词,返回空字符串。
+
+时间复杂度 $O(m \times n \times \log n + n \times 2^M)$,空间复杂度 $O(m \times n)$。其中 $n$ 和 $m$ 分别为单词列表的长度和单词的平均长度,而 $M$ 为最长单词的长度。
#### Python3
```python
-class Trie:
- def __init__(self):
- self.children = [None] * 26
- self.is_end = False
-
- def insert(self, word):
- node = self
- for c in word:
- idx = ord(c) - ord('a')
- if node.children[idx] is None:
- node.children[idx] = Trie()
- node = node.children[idx]
- node.is_end = True
-
- def search(self, word):
- node = self
- for c in word:
- idx = ord(c) - ord('a')
- if node.children[idx] is None:
- return False
- node = node.children[idx]
- return node.is_end
-
-
class Solution:
def longestWord(self, words: List[str]) -> str:
- def cmp(a, b):
- if len(a) != len(b):
- return len(a) - len(b)
- return -1 if a > b else 1
-
- def dfs(w):
- return not w or any(
- trie.search(w[:i]) and dfs(w[i:]) for i in range(1, len(w) + 1)
- )
-
- words.sort(key=cmp_to_key(cmp))
- trie = Trie()
- ans = ""
+ def dfs(w: str) -> bool:
+ if not w:
+ return True
+ for k in range(1, len(w) + 1):
+ if w[:k] in s and dfs(w[k:]):
+ return True
+ return False
+
+ s = set(words)
+ words.sort(key=lambda x: (-len(x), x))
for w in words:
+ s.remove(w)
if dfs(w):
- ans = w
- trie.insert(w)
- return ans
+ return w
+ return ""
```
#### Java
```java
-class Trie {
- Trie[] children = new Trie[26];
- boolean isEnd;
-
- void insert(String word) {
- Trie node = this;
- for (char c : word.toCharArray()) {
- c -= 'a';
- if (node.children[c] == null) {
- node.children[c] = new Trie();
- }
- node = node.children[c];
- }
- node.isEnd = true;
- }
-
- boolean search(String word) {
- Trie node = this;
- for (char c : word.toCharArray()) {
- c -= 'a';
- if (node.children[c] == null) {
- return false;
- }
- node = node.children[c];
- }
- return node.isEnd;
- }
-}
-
class Solution {
- private Trie trie = new Trie();
+ private Set s = new HashSet<>();
public String longestWord(String[] words) {
+ for (String w : words) {
+ s.add(w);
+ }
Arrays.sort(words, (a, b) -> {
if (a.length() != b.length()) {
- return a.length() - b.length();
+ return b.length() - a.length();
}
- return b.compareTo(a);
+ return a.compareTo(b);
});
- String ans = "";
for (String w : words) {
+ s.remove(w);
if (dfs(w)) {
- ans = w;
+ return w;
}
- trie.insert(w);
}
- return ans;
+ return "";
}
private boolean dfs(String w) {
- if ("".equals(w)) {
+ if (w.length() == 0) {
return true;
}
- for (int i = 1; i <= w.length(); ++i) {
- if (trie.search(w.substring(0, i)) && dfs(w.substring(i))) {
+ for (int k = 1; k <= w.length(); ++k) {
+ if (s.contains(w.substring(0, k)) && dfs(w.substring(k))) {
return true;
}
}
@@ -151,131 +113,171 @@ class Solution {
}
```
-#### Go
-
-```go
-type Trie struct {
- children [26]*Trie
- isEnd bool
-}
+#### C++
-func newTrie() *Trie {
- return &Trie{}
-}
-func (this *Trie) insert(word string) {
- node := this
- for _, c := range word {
- c -= 'a'
- if node.children[c] == nil {
- node.children[c] = newTrie()
- }
- node = node.children[c]
- }
- node.isEnd = true
-}
+```cpp
+class Solution {
+public:
+ string longestWord(vector& words) {
+ unordered_set s(words.begin(), words.end());
+ ranges::sort(words, [&](const string& a, const string& b) {
+ return a.size() > b.size() || (a.size() == b.size() && a < b);
+ });
+ auto dfs = [&](this auto&& dfs, string w) -> bool {
+ if (w.empty()) {
+ return true;
+ }
+ for (int k = 1; k <= w.size(); ++k) {
+ if (s.contains(w.substr(0, k)) && dfs(w.substr(k))) {
+ return true;
+ }
+ }
+ return false;
+ };
+ for (const string& w : words) {
+ s.erase(w);
+ if (dfs(w)) {
+ return w;
+ }
+ }
+ return "";
+ }
+};
+```
-func (this *Trie) search(word string) bool {
- node := this
- for _, c := range word {
- c -= 'a'
- if node.children[c] == nil {
- return false
- }
- node = node.children[c]
- }
- return node.isEnd
-}
+#### Go
+```go
func longestWord(words []string) string {
+ s := map[string]bool{}
+ for _, w := range words {
+ s[w] = true
+ }
sort.Slice(words, func(i, j int) bool {
- a, b := words[i], words[j]
- if len(a) != len(b) {
- return len(a) < len(b)
- }
- return a > b
+ return len(words[i]) > len(words[j]) || (len(words[i]) == len(words[j]) && words[i] < words[j])
})
- trie := newTrie()
var dfs func(string) bool
dfs = func(w string) bool {
if len(w) == 0 {
return true
}
- for i := 1; i <= len(w); i++ {
- if trie.search(w[:i]) && dfs(w[i:]) {
+ for k := 1; k <= len(w); k++ {
+ if s[w[:k]] && dfs(w[k:]) {
return true
}
}
return false
}
- ans := ""
for _, w := range words {
+ s[w] = false
if dfs(w) {
- ans = w
+ return w
}
- trie.insert(w)
}
- return ans
+ return ""
}
```
-#### Swift
+#### TypeScript
-```swift
-class Trie {
- var children = [Trie?](repeating: nil, count: 26)
- var isEnd = false
-
- func insert(_ word: String) {
- var node = self
- for ch in word {
- let index = Int(ch.asciiValue! - Character("a").asciiValue!)
- if node.children[index] == nil {
- node.children[index] = Trie()
+```ts
+function longestWord(words: string[]): string {
+ const s = new Set(words);
+
+ words.sort((a, b) => (a.length === b.length ? a.localeCompare(b) : b.length - a.length));
+
+ const dfs = (w: string): boolean => {
+ if (w === '') {
+ return true;
+ }
+ for (let k = 1; k <= w.length; ++k) {
+ if (s.has(w.substring(0, k)) && dfs(w.substring(k))) {
+ return true;
}
- node = node.children[index]!
}
- node.isEnd = true
+ return false;
+ };
+
+ for (const w of words) {
+ s.delete(w);
+ if (dfs(w)) {
+ return w;
+ }
}
- func search(_ word: String) -> Bool {
- var node = self
- for ch in word {
- let index = Int(ch.asciiValue! - Character("a").asciiValue!)
- if node.children[index] == nil {
- return false
+ return '';
+}
+```
+
+#### Rust
+
+```rust
+use std::collections::HashSet;
+
+impl Solution {
+ pub fn longest_word(words: Vec) -> String {
+ let mut s: HashSet = words.iter().cloned().collect();
+ let mut words = words;
+ words.sort_by(|a, b| b.len().cmp(&a.len()).then(a.cmp(b)));
+
+ fn dfs(w: String, s: &mut HashSet) -> bool {
+ if w.is_empty() {
+ return true;
}
- node = node.children[index]!
+ for k in 1..=w.len() {
+ if s.contains(&w[0..k]) && dfs(w[k..].to_string(), s) {
+ return true;
+ }
+ }
+ false
}
- return node.isEnd
+ for w in words {
+ s.remove(&w);
+ if dfs(w.clone(), &mut s) {
+ return w;
+ }
+ }
+ String::new()
}
}
+```
+
+#### Swift
+```swift
class Solution {
func longestWord(_ words: [String]) -> String {
- var words = words.sorted(by: { $0.count < $1.count || ($0.count == $1.count && $0 > $1) })
- let trie = Trie()
+ var s: Set = Set(words)
+ var words = words
+ words.sort { (a, b) -> Bool in
+ if a.count == b.count {
+ return a < b
+ } else {
+ return a.count > b.count
+ }
+ }
- var dfs: ((String) -> Bool)!
- dfs = { w in
+ func dfs(_ w: String) -> Bool {
if w.isEmpty {
return true
}
- for i in 1...w.count {
- if trie.search(String(w.prefix(i))) && dfs(String(w.suffix(w.count - i))) {
+ for k in 1...w.count {
+ let prefix = String(w.prefix(k))
+ if s.contains(prefix) && dfs(String(w.dropFirst(k))) {
return true
}
}
return false
}
- var ans = ""
for w in words {
+ s.remove(w)
if dfs(w) {
- ans = w
+ return w
}
- trie.insert(w)
}
- return ans
+
+ return ""
}
}
```
diff --git a/lcci/17.15.Longest Word/README_EN.md b/lcci/17.15.Longest Word/README_EN.md
index 7d5dcf7f14dec..131326adf233f 100644
--- a/lcci/17.15.Longest Word/README_EN.md
+++ b/lcci/17.15.Longest Word/README_EN.md
@@ -41,117 +41,79 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcci/17.15.Longest%20Word/
-### Solution 1
+### Solution 1: Hash Table + Sorting + DFS
+
+Note that in the problem, each word can actually be reused.
+
+We can use a hash table $\textit{s}$ to store all the words, then sort the words in descending order of length, and if the lengths are the same, sort them in ascending lexicographical order.
+
+Next, we iterate through the sorted list of words. For each word $\textit{w}$, we first remove it from the hash table $\textit{s}$, then use depth-first search $\textit{dfs}$ to determine if $\textit{w}$ can be composed of other words. If it can, we return $\textit{w}$.
+
+The execution logic of the function $\textit{dfs}$ is as follows:
+
+- If $\textit{w}$ is empty, return $\text{true}$;
+- Iterate through all prefixes of $\textit{w}$. If a prefix is in the hash table $\textit{s}$ and $\textit{dfs}$ returns $\text{true}$, then return $\text{true}$;
+- If no prefix meets the condition, return $\text{false}$.
+
+If no word meets the condition, return an empty string.
+
+The time complexity is $O(m \times n \times \log n + n \times 2^M)$, and the space complexity is $O(m \times n)$. Here, $n$ and $m$ are the length of the word list and the average length of the words, respectively, and $M$ is the length of the longest word.
#### Python3
```python
-class Trie:
- def __init__(self):
- self.children = [None] * 26
- self.is_end = False
-
- def insert(self, word):
- node = self
- for c in word:
- idx = ord(c) - ord('a')
- if node.children[idx] is None:
- node.children[idx] = Trie()
- node = node.children[idx]
- node.is_end = True
-
- def search(self, word):
- node = self
- for c in word:
- idx = ord(c) - ord('a')
- if node.children[idx] is None:
- return False
- node = node.children[idx]
- return node.is_end
-
-
class Solution:
def longestWord(self, words: List[str]) -> str:
- def cmp(a, b):
- if len(a) != len(b):
- return len(a) - len(b)
- return -1 if a > b else 1
-
- def dfs(w):
- return not w or any(
- trie.search(w[:i]) and dfs(w[i:]) for i in range(1, len(w) + 1)
- )
-
- words.sort(key=cmp_to_key(cmp))
- trie = Trie()
- ans = ""
+ def dfs(w: str) -> bool:
+ if not w:
+ return True
+ for k in range(1, len(w) + 1):
+ if w[:k] in s and dfs(w[k:]):
+ return True
+ return False
+
+ s = set(words)
+ words.sort(key=lambda x: (-len(x), x))
for w in words:
+ s.remove(w)
if dfs(w):
- ans = w
- trie.insert(w)
- return ans
+ return w
+ return ""
```
#### Java
```java
-class Trie {
- Trie[] children = new Trie[26];
- boolean isEnd;
-
- void insert(String word) {
- Trie node = this;
- for (char c : word.toCharArray()) {
- c -= 'a';
- if (node.children[c] == null) {
- node.children[c] = new Trie();
- }
- node = node.children[c];
- }
- node.isEnd = true;
- }
-
- boolean search(String word) {
- Trie node = this;
- for (char c : word.toCharArray()) {
- c -= 'a';
- if (node.children[c] == null) {
- return false;
- }
- node = node.children[c];
- }
- return node.isEnd;
- }
-}
-
class Solution {
- private Trie trie = new Trie();
+ private Set s = new HashSet<>();
public String longestWord(String[] words) {
+ for (String w : words) {
+ s.add(w);
+ }
Arrays.sort(words, (a, b) -> {
if (a.length() != b.length()) {
- return a.length() - b.length();
+ return b.length() - a.length();
}
- return b.compareTo(a);
+ return a.compareTo(b);
});
- String ans = "";
for (String w : words) {
+ s.remove(w);
if (dfs(w)) {
- ans = w;
+ return w;
}
- trie.insert(w);
}
- return ans;
+ return "";
}
private boolean dfs(String w) {
- if ("".equals(w)) {
+ if (w.length() == 0) {
return true;
}
- for (int i = 1; i <= w.length(); ++i) {
- if (trie.search(w.substring(0, i)) && dfs(w.substring(i))) {
+ for (int k = 1; k <= w.length(); ++k) {
+ if (s.contains(w.substring(0, k)) && dfs(w.substring(k))) {
return true;
}
}
@@ -160,131 +122,171 @@ class Solution {
}
```
-#### Go
-
-```go
-type Trie struct {
- children [26]*Trie
- isEnd bool
-}
+#### C++
-func newTrie() *Trie {
- return &Trie{}
-}
-func (this *Trie) insert(word string) {
- node := this
- for _, c := range word {
- c -= 'a'
- if node.children[c] == nil {
- node.children[c] = newTrie()
- }
- node = node.children[c]
- }
- node.isEnd = true
-}
+```cpp
+class Solution {
+public:
+ string longestWord(vector& words) {
+ unordered_set s(words.begin(), words.end());
+ ranges::sort(words, [&](const string& a, const string& b) {
+ return a.size() > b.size() || (a.size() == b.size() && a < b);
+ });
+ auto dfs = [&](this auto&& dfs, string w) -> bool {
+ if (w.empty()) {
+ return true;
+ }
+ for (int k = 1; k <= w.size(); ++k) {
+ if (s.contains(w.substr(0, k)) && dfs(w.substr(k))) {
+ return true;
+ }
+ }
+ return false;
+ };
+ for (const string& w : words) {
+ s.erase(w);
+ if (dfs(w)) {
+ return w;
+ }
+ }
+ return "";
+ }
+};
+```
-func (this *Trie) search(word string) bool {
- node := this
- for _, c := range word {
- c -= 'a'
- if node.children[c] == nil {
- return false
- }
- node = node.children[c]
- }
- return node.isEnd
-}
+#### Go
+```go
func longestWord(words []string) string {
+ s := map[string]bool{}
+ for _, w := range words {
+ s[w] = true
+ }
sort.Slice(words, func(i, j int) bool {
- a, b := words[i], words[j]
- if len(a) != len(b) {
- return len(a) < len(b)
- }
- return a > b
+ return len(words[i]) > len(words[j]) || (len(words[i]) == len(words[j]) && words[i] < words[j])
})
- trie := newTrie()
var dfs func(string) bool
dfs = func(w string) bool {
if len(w) == 0 {
return true
}
- for i := 1; i <= len(w); i++ {
- if trie.search(w[:i]) && dfs(w[i:]) {
+ for k := 1; k <= len(w); k++ {
+ if s[w[:k]] && dfs(w[k:]) {
return true
}
}
return false
}
- ans := ""
for _, w := range words {
+ s[w] = false
if dfs(w) {
- ans = w
+ return w
}
- trie.insert(w)
}
- return ans
+ return ""
}
```
-#### Swift
+#### TypeScript
-```swift
-class Trie {
- var children = [Trie?](repeating: nil, count: 26)
- var isEnd = false
-
- func insert(_ word: String) {
- var node = self
- for ch in word {
- let index = Int(ch.asciiValue! - Character("a").asciiValue!)
- if node.children[index] == nil {
- node.children[index] = Trie()
+```ts
+function longestWord(words: string[]): string {
+ const s = new Set(words);
+
+ words.sort((a, b) => (a.length === b.length ? a.localeCompare(b) : b.length - a.length));
+
+ const dfs = (w: string): boolean => {
+ if (w === '') {
+ return true;
+ }
+ for (let k = 1; k <= w.length; ++k) {
+ if (s.has(w.substring(0, k)) && dfs(w.substring(k))) {
+ return true;
}
- node = node.children[index]!
}
- node.isEnd = true
+ return false;
+ };
+
+ for (const w of words) {
+ s.delete(w);
+ if (dfs(w)) {
+ return w;
+ }
}
- func search(_ word: String) -> Bool {
- var node = self
- for ch in word {
- let index = Int(ch.asciiValue! - Character("a").asciiValue!)
- if node.children[index] == nil {
- return false
+ return '';
+}
+```
+
+#### Rust
+
+```rust
+use std::collections::HashSet;
+
+impl Solution {
+ pub fn longest_word(words: Vec) -> String {
+ let mut s: HashSet = words.iter().cloned().collect();
+ let mut words = words;
+ words.sort_by(|a, b| b.len().cmp(&a.len()).then(a.cmp(b)));
+
+ fn dfs(w: String, s: &mut HashSet) -> bool {
+ if w.is_empty() {
+ return true;
}
- node = node.children[index]!
+ for k in 1..=w.len() {
+ if s.contains(&w[0..k]) && dfs(w[k..].to_string(), s) {
+ return true;
+ }
+ }
+ false
}
- return node.isEnd
+ for w in words {
+ s.remove(&w);
+ if dfs(w.clone(), &mut s) {
+ return w;
+ }
+ }
+ String::new()
}
}
+```
+
+#### Swift
+```swift
class Solution {
func longestWord(_ words: [String]) -> String {
- var words = words.sorted(by: { $0.count < $1.count || ($0.count == $1.count && $0 > $1) })
- let trie = Trie()
+ var s: Set = Set(words)
+ var words = words
+ words.sort { (a, b) -> Bool in
+ if a.count == b.count {
+ return a < b
+ } else {
+ return a.count > b.count
+ }
+ }
- var dfs: ((String) -> Bool)!
- dfs = { w in
+ func dfs(_ w: String) -> Bool {
if w.isEmpty {
return true
}
- for i in 1...w.count {
- if trie.search(String(w.prefix(i))) && dfs(String(w.suffix(w.count - i))) {
+ for k in 1...w.count {
+ let prefix = String(w.prefix(k))
+ if s.contains(prefix) && dfs(String(w.dropFirst(k))) {
return true
}
}
return false
}
- var ans = ""
for w in words {
+ s.remove(w)
if dfs(w) {
- ans = w
+ return w
}
- trie.insert(w)
}
- return ans
+
+ return ""
}
}
```
diff --git a/lcci/17.15.Longest Word/Solution.cpp b/lcci/17.15.Longest Word/Solution.cpp
new file mode 100644
index 0000000000000..19b158b023c7d
--- /dev/null
+++ b/lcci/17.15.Longest Word/Solution.cpp
@@ -0,0 +1,27 @@
+class Solution {
+public:
+ string longestWord(vector& words) {
+ unordered_set s(words.begin(), words.end());
+ ranges::sort(words, [&](const string& a, const string& b) {
+ return a.size() > b.size() || (a.size() == b.size() && a < b);
+ });
+ auto dfs = [&](this auto&& dfs, string w) -> bool {
+ if (w.empty()) {
+ return true;
+ }
+ for (int k = 1; k <= w.size(); ++k) {
+ if (s.contains(w.substr(0, k)) && dfs(w.substr(k))) {
+ return true;
+ }
+ }
+ return false;
+ };
+ for (const string& w : words) {
+ s.erase(w);
+ if (dfs(w)) {
+ return w;
+ }
+ }
+ return "";
+ }
+};
diff --git a/lcci/17.15.Longest Word/Solution.go b/lcci/17.15.Longest Word/Solution.go
index 2a6dbd07cf5a4..321fb05d318ae 100644
--- a/lcci/17.15.Longest Word/Solution.go
+++ b/lcci/17.15.Longest Word/Solution.go
@@ -1,62 +1,28 @@
-type Trie struct {
- children [26]*Trie
- isEnd bool
-}
-
-func newTrie() *Trie {
- return &Trie{}
-}
-func (this *Trie) insert(word string) {
- node := this
- for _, c := range word {
- c -= 'a'
- if node.children[c] == nil {
- node.children[c] = newTrie()
- }
- node = node.children[c]
- }
- node.isEnd = true
-}
-
-func (this *Trie) search(word string) bool {
- node := this
- for _, c := range word {
- c -= 'a'
- if node.children[c] == nil {
- return false
- }
- node = node.children[c]
- }
- return node.isEnd
-}
-
func longestWord(words []string) string {
+ s := map[string]bool{}
+ for _, w := range words {
+ s[w] = true
+ }
sort.Slice(words, func(i, j int) bool {
- a, b := words[i], words[j]
- if len(a) != len(b) {
- return len(a) < len(b)
- }
- return a > b
+ return len(words[i]) > len(words[j]) || (len(words[i]) == len(words[j]) && words[i] < words[j])
})
- trie := newTrie()
var dfs func(string) bool
dfs = func(w string) bool {
if len(w) == 0 {
return true
}
- for i := 1; i <= len(w); i++ {
- if trie.search(w[:i]) && dfs(w[i:]) {
+ for k := 1; k <= len(w); k++ {
+ if s[w[:k]] && dfs(w[k:]) {
return true
}
}
return false
}
- ans := ""
for _, w := range words {
+ s[w] = false
if dfs(w) {
- ans = w
+ return w
}
- trie.insert(w)
}
- return ans
-}
\ No newline at end of file
+ return ""
+}
diff --git a/lcci/17.15.Longest Word/Solution.java b/lcci/17.15.Longest Word/Solution.java
index 2d6e2d40bd4c3..e7bbcc380a592 100644
--- a/lcci/17.15.Longest Word/Solution.java
+++ b/lcci/17.15.Longest Word/Solution.java
@@ -1,61 +1,34 @@
-class Trie {
- Trie[] children = new Trie[26];
- boolean isEnd;
-
- void insert(String word) {
- Trie node = this;
- for (char c : word.toCharArray()) {
- c -= 'a';
- if (node.children[c] == null) {
- node.children[c] = new Trie();
- }
- node = node.children[c];
- }
- node.isEnd = true;
- }
-
- boolean search(String word) {
- Trie node = this;
- for (char c : word.toCharArray()) {
- c -= 'a';
- if (node.children[c] == null) {
- return false;
- }
- node = node.children[c];
- }
- return node.isEnd;
- }
-}
-
class Solution {
- private Trie trie = new Trie();
+ private Set s = new HashSet<>();
public String longestWord(String[] words) {
+ for (String w : words) {
+ s.add(w);
+ }
Arrays.sort(words, (a, b) -> {
if (a.length() != b.length()) {
- return a.length() - b.length();
+ return b.length() - a.length();
}
- return b.compareTo(a);
+ return a.compareTo(b);
});
- String ans = "";
for (String w : words) {
+ s.remove(w);
if (dfs(w)) {
- ans = w;
+ return w;
}
- trie.insert(w);
}
- return ans;
+ return "";
}
private boolean dfs(String w) {
- if ("".equals(w)) {
+ if (w.length() == 0) {
return true;
}
- for (int i = 1; i <= w.length(); ++i) {
- if (trie.search(w.substring(0, i)) && dfs(w.substring(i))) {
+ for (int k = 1; k <= w.length(); ++k) {
+ if (s.contains(w.substring(0, k)) && dfs(w.substring(k))) {
return true;
}
}
return false;
}
-}
\ No newline at end of file
+}
diff --git a/lcci/17.15.Longest Word/Solution.py b/lcci/17.15.Longest Word/Solution.py
index 5a5b69545a1a2..c2dd7b8387c09 100644
--- a/lcci/17.15.Longest Word/Solution.py
+++ b/lcci/17.15.Longest Word/Solution.py
@@ -1,44 +1,17 @@
-class Trie:
- def __init__(self):
- self.children = [None] * 26
- self.is_end = False
-
- def insert(self, word):
- node = self
- for c in word:
- idx = ord(c) - ord('a')
- if node.children[idx] is None:
- node.children[idx] = Trie()
- node = node.children[idx]
- node.is_end = True
-
- def search(self, word):
- node = self
- for c in word:
- idx = ord(c) - ord('a')
- if node.children[idx] is None:
- return False
- node = node.children[idx]
- return node.is_end
-
-
class Solution:
def longestWord(self, words: List[str]) -> str:
- def cmp(a, b):
- if len(a) != len(b):
- return len(a) - len(b)
- return -1 if a > b else 1
-
- def dfs(w):
- return not w or any(
- trie.search(w[:i]) and dfs(w[i:]) for i in range(1, len(w) + 1)
- )
+ def dfs(w: str) -> bool:
+ if not w:
+ return True
+ for k in range(1, len(w) + 1):
+ if w[:k] in s and dfs(w[k:]):
+ return True
+ return False
- words.sort(key=cmp_to_key(cmp))
- trie = Trie()
- ans = ""
+ s = set(words)
+ words.sort(key=lambda x: (-len(x), x))
for w in words:
+ s.remove(w)
if dfs(w):
- ans = w
- trie.insert(w)
- return ans
+ return w
+ return ""
diff --git a/lcci/17.15.Longest Word/Solution.rs b/lcci/17.15.Longest Word/Solution.rs
new file mode 100644
index 0000000000000..068124b25c26a
--- /dev/null
+++ b/lcci/17.15.Longest Word/Solution.rs
@@ -0,0 +1,28 @@
+use std::collections::HashSet;
+
+impl Solution {
+ pub fn longest_word(words: Vec) -> String {
+ let mut s: HashSet = words.iter().cloned().collect();
+ let mut words = words;
+ words.sort_by(|a, b| b.len().cmp(&a.len()).then(a.cmp(b)));
+
+ fn dfs(w: String, s: &mut HashSet) -> bool {
+ if w.is_empty() {
+ return true;
+ }
+ for k in 1..=w.len() {
+ if s.contains(&w[0..k]) && dfs(w[k..].to_string(), s) {
+ return true;
+ }
+ }
+ false
+ }
+ for w in words {
+ s.remove(&w);
+ if dfs(w.clone(), &mut s) {
+ return w;
+ }
+ }
+ String::new()
+ }
+}
diff --git a/lcci/17.15.Longest Word/Solution.swift b/lcci/17.15.Longest Word/Solution.swift
index f05b1fc22864c..008d82149d0be 100644
--- a/lcci/17.15.Longest Word/Solution.swift
+++ b/lcci/17.15.Longest Word/Solution.swift
@@ -1,57 +1,35 @@
-class Trie {
- var children = [Trie?](repeating: nil, count: 26)
- var isEnd = false
-
- func insert(_ word: String) {
- var node = self
- for ch in word {
- let index = Int(ch.asciiValue! - Character("a").asciiValue!)
- if node.children[index] == nil {
- node.children[index] = Trie()
+class Solution {
+ func longestWord(_ words: [String]) -> String {
+ var s: Set = Set(words)
+ var words = words
+ words.sort { (a, b) -> Bool in
+ if a.count == b.count {
+ return a < b
+ } else {
+ return a.count > b.count
}
- node = node.children[index]!
}
- node.isEnd = true
- }
- func search(_ word: String) -> Bool {
- var node = self
- for ch in word {
- let index = Int(ch.asciiValue! - Character("a").asciiValue!)
- if node.children[index] == nil {
- return false
- }
- node = node.children[index]!
- }
- return node.isEnd
- }
-}
-
-class Solution {
- func longestWord(_ words: [String]) -> String {
- var words = words.sorted(by: { $0.count < $1.count || ($0.count == $1.count && $0 > $1) })
- let trie = Trie()
-
- var dfs: ((String) -> Bool)!
- dfs = { w in
+ func dfs(_ w: String) -> Bool {
if w.isEmpty {
return true
}
- for i in 1...w.count {
- if trie.search(String(w.prefix(i))) && dfs(String(w.suffix(w.count - i))) {
+ for k in 1...w.count {
+ let prefix = String(w.prefix(k))
+ if s.contains(prefix) && dfs(String(w.dropFirst(k))) {
return true
}
}
return false
}
-
- var ans = ""
+
for w in words {
+ s.remove(w)
if dfs(w) {
- ans = w
+ return w
}
- trie.insert(w)
}
- return ans
+
+ return ""
}
}
diff --git a/lcci/17.15.Longest Word/Solution.ts b/lcci/17.15.Longest Word/Solution.ts
new file mode 100644
index 0000000000000..1dc4862412162
--- /dev/null
+++ b/lcci/17.15.Longest Word/Solution.ts
@@ -0,0 +1,26 @@
+function longestWord(words: string[]): string {
+ const s = new Set(words);
+
+ words.sort((a, b) => (a.length === b.length ? a.localeCompare(b) : b.length - a.length));
+
+ const dfs = (w: string): boolean => {
+ if (w === '') {
+ return true;
+ }
+ for (let k = 1; k <= w.length; ++k) {
+ if (s.has(w.substring(0, k)) && dfs(w.substring(k))) {
+ return true;
+ }
+ }
+ return false;
+ };
+
+ for (const w of words) {
+ s.delete(w);
+ if (dfs(w)) {
+ return w;
+ }
+ }
+
+ return '';
+}
diff --git "a/lcof/\351\235\242\350\257\225\351\242\23011. \346\227\213\350\275\254\346\225\260\347\273\204\347\232\204\346\234\200\345\260\217\346\225\260\345\255\227/README.md" "b/lcof/\351\235\242\350\257\225\351\242\23011. \346\227\213\350\275\254\346\225\260\347\273\204\347\232\204\346\234\200\345\260\217\346\225\260\345\255\227/README.md"
index ad4c6421ed926..f238a9a8c0e5a 100644
--- "a/lcof/\351\235\242\350\257\225\351\242\23011. \346\227\213\350\275\254\346\225\260\347\273\204\347\232\204\346\234\200\345\260\217\346\225\260\345\255\227/README.md"
+++ "b/lcof/\351\235\242\350\257\225\351\242\23011. \346\227\213\350\275\254\346\225\260\347\273\204\347\232\204\346\234\200\345\260\217\346\225\260\345\255\227/README.md"
@@ -46,7 +46,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof/%E9%9D%A2%E8%AF%95%E9
- `numbers[mid] > numbers[r]`:中间元素一定不是最小值,因此 $l = mid + 1$;
- `numbers[mid] < numbers[r]`:中间元素可能是最小值,因此 $r = mid$;
-- `numbers[mid] == numbers[r]`:中间元素一定不是最小值,因此 $r = r - 1$。
+- `numbers[mid] == numbers[r]`:无法确定最小值的位置,但可以简单地缩小搜索范围,因此 $r = r - 1$。
循环结束时,指针 $l$ 和 $r$ 指向同一个元素,即为最小值。
diff --git "a/lcof/\351\235\242\350\257\225\351\242\23020. \350\241\250\347\244\272\346\225\260\345\200\274\347\232\204\345\255\227\347\254\246\344\270\262/README.md" "b/lcof/\351\235\242\350\257\225\351\242\23020. \350\241\250\347\244\272\346\225\260\345\200\274\347\232\204\345\255\227\347\254\246\344\270\262/README.md"
index f2eb26a4d88c1..539be1dbe3691 100644
--- "a/lcof/\351\235\242\350\257\225\351\242\23020. \350\241\250\347\244\272\346\225\260\345\200\274\347\232\204\345\255\227\347\254\246\344\270\262/README.md"
+++ "b/lcof/\351\235\242\350\257\225\351\242\23020. \350\241\250\347\244\272\346\225\260\345\200\274\347\232\204\345\255\227\347\254\246\344\270\262/README.md"
@@ -111,7 +111,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof/%E9%9D%A2%E8%AF%95%E9
遍历 $s[i,..j]$ 范围内的每个字符,根据字符的类型进行分类讨论:
-- 如果当前字符是 `+` 或者 `-`,那么该字符的前一个字符必须是 `e` 或者 `E`,或者空格,否则返回 `false`。
+- 如果当前字符是 `+` 或者 `-`,那么该字符必须是第一个有效字符(即空格后的第一个非空字符),或者该字符的前一个字符必须是 `e` 或者 `E`,否则返回 `false`。
- 如果当前字符是数字,那么我们将 `digit` 置为 `true`。
- 如果当前字符是 `.`,那么该字符之前不能出现过 `.` 或者 `e`/`E`,否则返回 `false`,否则我们将 `dot` 置为 `true`。
- 如果当前字符是 `e` 或者 `E`,那么该字符之前不能出现过 `e`/`E`,并且必须出现过数字,否则返回 `false`,否则我们将 `e` 置为 `true`,并且将 `digit` 置为 `false`,表示 `e` 之后必须出现数字。
diff --git "a/lcof/\351\235\242\350\257\225\351\242\23026. \346\240\221\347\232\204\345\255\220\347\273\223\346\236\204/README.md" "b/lcof/\351\235\242\350\257\225\351\242\23026. \346\240\221\347\232\204\345\255\220\347\273\223\346\236\204/README.md"
index d82afb7073ebb..357589c016e2d 100644
--- "a/lcof/\351\235\242\350\257\225\351\242\23026. \346\240\221\347\232\204\345\255\220\347\273\223\346\236\204/README.md"
+++ "b/lcof/\351\235\242\350\257\225\351\242\23026. \346\240\221\347\232\204\345\255\220\347\273\223\346\236\204/README.md"
@@ -60,7 +60,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof/%E9%9D%A2%E8%AF%95%E9
1. 如果树 B 为空,则树 B 是树 A 的子结构,返回 `true`;
2. 如果树 A 为空,或者树 A 的根节点的值不等于树 B 的根节点的值,则树 B 不是树 A 的子结构,返回 `false`;
-3. 判断树 A 的左子树是否包含树 B,即调用 $\textit{dfs}(A.left, B)$,并且判断树 A 的右子树是否包含树 B,即调用 $\textit{dfs}(A.right, B)$。如果其中有一个函数返回 `false`,则树 B 不是树 A 的子结构,返回 `false`;否则,返回 `true`。
+3. 判断树 A 的左子树是否包含树 B 的左子树,即调用 $\textit{dfs}(A.left, B.left)$,并且判断树 A 的右子树是否包含树 B 的右子树,即调用 $\textit{dfs}(A.right, B.right)$。如果其中有一个函数返回 `false`,则树 B 不是树 A 的子结构,返回 `false`;否则,返回 `true`。
在函数 `isSubStructure` 中,我们首先判断树 A 和树 B 是否为空,如果其中有一个为空,则树 B 不是树 A 的子结构,返回 `false`。然后,我们调用 $\textit{dfs}(A, B)$,判断树 A 是否包含树 B。如果是,则返回 `true`;否则,递归判断树 A 的左子树是否包含树 B,以及树 A 的右子树是否包含树 B。如果其中有一个返回 `true`,则树 B 是树 A 的子结构,返回 `true`;否则,返回 `false`。
diff --git "a/lcof/\351\235\242\350\257\225\351\242\23044. \346\225\260\345\255\227\345\272\217\345\210\227\344\270\255\346\237\220\344\270\200\344\275\215\347\232\204\346\225\260\345\255\227/README.md" "b/lcof/\351\235\242\350\257\225\351\242\23044. \346\225\260\345\255\227\345\272\217\345\210\227\344\270\255\346\237\220\344\270\200\344\275\215\347\232\204\346\225\260\345\255\227/README.md"
index 9305b97a146e7..3b8e96c4f1e5e 100644
--- "a/lcof/\351\235\242\350\257\225\351\242\23044. \346\225\260\345\255\227\345\272\217\345\210\227\344\270\255\346\237\220\344\270\200\344\275\215\347\232\204\346\225\260\345\255\227/README.md"
+++ "b/lcof/\351\235\242\350\257\225\351\242\23044. \346\225\260\345\255\227\345\272\217\345\210\227\344\270\255\346\237\220\344\270\200\344\275\215\347\232\204\346\225\260\345\255\227/README.md"
@@ -12,7 +12,7 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof/%E9%9D%A2%E8%AF%95%E9
-数字以0123456789101112131415…的格式序列化到一个字符序列中。在这个序列中,第5位(从下标0开始计数)是5,第13位是1,第19位是4,等等。
+数字以123456789101112131415…的格式序列化到一个字符序列中。在这个序列中,第5位是5,第13位是1,第19位是4,等等。
请写一个函数,求任意第n位对应的数字。
diff --git "a/lcof/\351\235\242\350\257\225\351\242\23046. \346\212\212\346\225\260\345\255\227\347\277\273\350\257\221\346\210\220\345\255\227\347\254\246\344\270\262/README.md" "b/lcof/\351\235\242\350\257\225\351\242\23046. \346\212\212\346\225\260\345\255\227\347\277\273\350\257\221\346\210\220\345\255\227\347\254\246\344\270\262/README.md"
index 98773024f9f8a..f0442ed4c037a 100644
--- "a/lcof/\351\235\242\350\257\225\351\242\23046. \346\212\212\346\225\260\345\255\227\347\277\273\350\257\221\346\210\220\345\255\227\347\254\246\344\270\262/README.md"
+++ "b/lcof/\351\235\242\350\257\225\351\242\23046. \346\212\212\346\225\260\345\255\227\347\277\273\350\257\221\346\210\220\345\255\227\347\254\246\344\270\262/README.md"
@@ -40,12 +40,12 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof/%E9%9D%A2%E8%AF%95%E9
我们先将数字 `num` 转为字符串 $s$,字符串 $s$ 的长度记为 $n$。
-然后我们设计一个函数 $dfs(i)$,表示从第 $i$ 个数字开始的不同翻译的数目。那么答案就是 $dfs(0)$。
+然后我们设计一个函数 $dfs(i)$,表示从索引为 $i$ 的数字开始的不同翻译的数目。那么答案就是 $dfs(0)$。
函数 $dfs(i)$ 的计算如下:
-- 如果 $i \ge n - 1$,说明已经翻译到最后一个数字,只有一种翻译方法,返回 $1$;
-- 否则,我们可以选择翻译第 $i$ 个数字,此时翻译方法数目为 $dfs(i + 1)$;如果第 $i$ 个数字和第 $i + 1$ 个数字可以组成一个有效的字符(即 $s[i] == 1$ 或者 $s[i] == 2$ 且 $s[i + 1] \lt 6$),那么我们还可以选择翻译第 $i$ 和第 $i + 1$ 个数字,此时翻译方法数目为 $dfs(i + 2)$。因此 $dfs(i) = dfs(i+1) + dfs(i+2)$。
+- 如果 $i \ge n - 1$,说明已经翻译到最后一个数字或者越过最后一个字符,均只有一种翻译方法,返回 $1$;
+- 否则,我们可以选择翻译索引为 $i$ 的数字,此时翻译方法数目为 $dfs(i + 1)$;如果索引为 $i$ 的数字和索引为 $i + 1$ 的数字可以组成一个有效的字符(即 $s[i] == 1$ 或者 $s[i] == 2$ 且 $s[i + 1] \lt 6$),那么我们还可以选择翻译索引为 $i$ 和索引为 $i + 1$ 的数字,此时翻译方法数目为 $dfs(i + 2)$。因此 $dfs(i) = dfs(i+1) + dfs(i+2)$。
过程中我们可以使用记忆化搜索,将已经计算过的 $dfs(i)$ 的值存储起来,避免重复计算。
diff --git "a/lcof/\351\235\242\350\257\225\351\242\23067. \346\212\212\345\255\227\347\254\246\344\270\262\350\275\254\346\215\242\346\210\220\346\225\264\346\225\260/README.md" "b/lcof/\351\235\242\350\257\225\351\242\23067. \346\212\212\345\255\227\347\254\246\344\270\262\350\275\254\346\215\242\346\210\220\346\225\264\346\225\260/README.md"
index ebe212c8fb7b0..1edfd50f307a8 100644
--- "a/lcof/\351\235\242\350\257\225\351\242\23067. \346\212\212\345\255\227\347\254\246\344\270\262\350\275\254\346\215\242\346\210\220\346\225\264\346\225\260/README.md"
+++ "b/lcof/\351\235\242\350\257\225\351\242\23067. \346\212\212\345\255\227\347\254\246\344\270\262\350\275\254\346\215\242\346\210\220\346\225\264\346\225\260/README.md"
@@ -143,6 +143,46 @@ class Solution {
}
```
+#### C++
+
+```cpp
+class Solution {
+public:
+ int strToInt(string str) {
+ int res = 0, bndry = INT_MAX / 10;
+ int i = 0, sign = 1, length = str.size();
+ if (length == 0) {
+ return 0;
+ }
+ // 删除首部空格
+ while (str[i] == ' ') {
+ if (++i == length) {
+ return 0;
+ }
+ }
+ // 若有负号则标识符号位
+ if (str[i] == '-') {
+ sign = -1;
+ }
+ if (str[i] == '-' || str[i] == '+') {
+ i++;
+ }
+ for (int j = i; j < length; j++) {
+ if (str[j] < '0' || str[j] > '9') {
+ break;
+ }
+ // res>214748364越界;res=214748364且str[j] > '7'越界
+ if (res > bndry || res == bndry && str[j] > '7') {
+ return sign == 1 ? INT_MAX : INT_MIN;
+ }
+ // 从左向右遍历数字并更新结果
+ res = res * 10 + (str[j] - '0');
+ }
+ return sign * res;
+ }
+};
+```
+
#### Go
```go
diff --git "a/lcof/\351\235\242\350\257\225\351\242\23067. \346\212\212\345\255\227\347\254\246\344\270\262\350\275\254\346\215\242\346\210\220\346\225\264\346\225\260/Solution.cpp" "b/lcof/\351\235\242\350\257\225\351\242\23067. \346\212\212\345\255\227\347\254\246\344\270\262\350\275\254\346\215\242\346\210\220\346\225\264\346\225\260/Solution.cpp"
new file mode 100644
index 0000000000000..c392b7ddcbd1f
--- /dev/null
+++ "b/lcof/\351\235\242\350\257\225\351\242\23067. \346\212\212\345\255\227\347\254\246\344\270\262\350\275\254\346\215\242\346\210\220\346\225\264\346\225\260/Solution.cpp"
@@ -0,0 +1,35 @@
+class Solution {
+public:
+ int strToInt(string str) {
+ int res = 0, bndry = INT_MAX / 10;
+ int i = 0, sign = 1, length = str.size();
+ if (length == 0) {
+ return 0;
+ }
+ // 删除首部空格
+ while (str[i] == ' ') {
+ if (++i == length) {
+ return 0;
+ }
+ }
+ // 若有负号则标识符号位
+ if (str[i] == '-') {
+ sign = -1;
+ }
+ if (str[i] == '-' || str[i] == '+') {
+ i++;
+ }
+ for (int j = i; j < length; j++) {
+ if (str[j] < '0' || str[j] > '9') {
+ break;
+ }
+ // res>214748364越界;res=214748364且str[j] > '7'越界
+ if (res > bndry || res == bndry && str[j] > '7') {
+ return sign == 1 ? INT_MAX : INT_MIN;
+ }
+ // 从左向右遍历数字并更新结果
+ res = res * 10 + (str[j] - '0');
+ }
+ return sign * res;
+ }
+};
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/README.md"
index 0a7445246a6a8..dd18ee74ba2eb 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/README.md"
@@ -69,9 +69,6 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof2/%E5%89%91%E6%8C%87%2
#### Python3
```python
-from sortedcontainers import SortedSet
-
-
class Solution:
def containsNearbyAlmostDuplicate(self, nums: List[int], k: int, t: int) -> bool:
s = SortedSet()
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/Solution.py" "b/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/Solution.py"
index f7ec481ecdd5d..b0910923928e3 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/Solution.py"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 057. \345\200\274\345\222\214\344\270\213\346\240\207\344\271\213\345\267\256\351\203\275\345\234\250\347\273\231\345\256\232\347\232\204\350\214\203\345\233\264\345\206\205/Solution.py"
@@ -1,6 +1,3 @@
-from sortedcontainers import SortedSet
-
-
class Solution:
def containsNearbyAlmostDuplicate(self, nums: List[int], k: int, t: int) -> bool:
s = SortedSet()
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/README.md"
index a3933de14e03c..54acea6311fdc 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/README.md"
@@ -65,9 +65,6 @@ MyCalendar.book(20, 30); // returns true ,第三个日程安排可以添加到
#### Python3
```python
-from sortedcontainers import SortedDict
-
-
class MyCalendar:
def __init__(self):
self.sd = SortedDict()
@@ -89,9 +86,6 @@ class MyCalendar:
#### Java
```java
-import java.util.Map;
-import java.util.TreeMap;
-
class MyCalendar {
private final TreeMap tm = new TreeMap<>();
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.java" "b/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.java"
index 3d04816dc4b2e..1bb41cffcc07f 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.java"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.java"
@@ -1,6 +1,3 @@
-import java.util.Map;
-import java.util.TreeMap;
-
class MyCalendar {
private final TreeMap tm = new TreeMap<>();
@@ -25,4 +22,4 @@ public boolean book(int start, int end) {
/**
* Your MyCalendar object will be instantiated and called as such: MyCalendar
* obj = new MyCalendar(); boolean param_1 = obj.book(start,end);
- */
\ No newline at end of file
+ */
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.py" "b/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.py"
index a17617ab776e9..c04195aeb8688 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.py"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 058. \346\227\245\347\250\213\350\241\250/Solution.py"
@@ -1,6 +1,3 @@
-from sortedcontainers import SortedDict
-
-
class MyCalendar:
def __init__(self):
self.sd = SortedDict()
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/README.md"
index 979fe1952d23a..b0debe2acef44 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/README.md"
@@ -50,7 +50,11 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof2/%E5%89%91%E6%8C%87%2
-### 方法一
+### 方法一:自定义排序
+
+我们先用哈希表 $pos$ 记录数组 $arr2$ 中每个元素的位置。然后,我们将数组 $arr1$ 中的每个元素映射成一个二元组 $(pos.get(x, 1000 + x), x)$,并对二元组进行排序。最后我们取出所有二元组的第二个元素并返回即可。
+
+时间复杂度 $O(n \times \log n + m)$,空间复杂度 $O(n + m)$。其中 $n$ 和 $m$ 分别是数组 $arr1$ 和 $arr2$ 的长度。
@@ -59,9 +63,8 @@ edit_url: https://github.com/doocs/leetcode/edit/main/lcof2/%E5%89%91%E6%8C%87%2
```python
class Solution:
def relativeSortArray(self, arr1: List[int], arr2: List[int]) -> List[int]:
- mp = {num: i for i, num in enumerate(arr2)}
- arr1.sort(key=lambda x: (mp.get(x, 10000), x))
- return arr1
+ pos = {x: i for i, x in enumerate(arr2)}
+ return sorted(arr1, key=lambda x: pos.get(x, 1000 + x))
```
#### Java
@@ -69,20 +72,17 @@ class Solution:
```java
class Solution {
public int[] relativeSortArray(int[] arr1, int[] arr2) {
- int[] mp = new int[1001];
- for (int x : arr1) {
- ++mp[x];
+ Map pos = new HashMap<>(arr2.length);
+ for (int i = 0; i < arr2.length; ++i) {
+ pos.put(arr2[i], i);
}
- int i = 0;
- for (int x : arr2) {
- while (mp[x]-- > 0) {
- arr1[i++] = x;
- }
+ int[][] arr = new int[arr1.length][0];
+ for (int i = 0; i < arr.length; ++i) {
+ arr[i] = new int[] {arr1[i], pos.getOrDefault(arr1[i], arr2.length + arr1[i])};
}
- for (int j = 0; j < mp.length; ++j) {
- while (mp[j]-- > 0) {
- arr1[i++] = j;
- }
+ Arrays.sort(arr, (a, b) -> a[1] - b[1]);
+ for (int i = 0; i < arr.length; ++i) {
+ arr1[i] = arr[i][0];
}
return arr1;
}
@@ -95,14 +95,18 @@ class Solution {
class Solution {
public:
vector relativeSortArray(vector& arr1, vector& arr2) {
- vector mp(1001);
- for (int x : arr1) ++mp[x];
- int i = 0;
- for (int x : arr2) {
- while (mp[x]-- > 0) arr1[i++] = x;
+ unordered_map pos;
+ for (int i = 0; i < arr2.size(); ++i) {
+ pos[arr2[i]] = i;
}
- for (int j = 0; j < mp.size(); ++j) {
- while (mp[j]-- > 0) arr1[i++] = j;
+ vector> arr;
+ for (int i = 0; i < arr1.size(); ++i) {
+ int j = pos.count(arr1[i]) ? pos[arr1[i]] : arr2.size();
+ arr.emplace_back(j, arr1[i]);
+ }
+ sort(arr.begin(), arr.end());
+ for (int i = 0; i < arr1.size(); ++i) {
+ arr1[i] = arr[i].second;
}
return arr1;
}
@@ -113,58 +117,250 @@ public:
```go
func relativeSortArray(arr1 []int, arr2 []int) []int {
- mp := make([]int, 1001)
- for _, x := range arr1 {
- mp[x]++
+ pos := map[int]int{}
+ for i, x := range arr2 {
+ pos[x] = i
}
- i := 0
- for _, x := range arr2 {
- for mp[x] > 0 {
- arr1[i] = x
- mp[x]--
- i++
+ arr := make([][2]int, len(arr1))
+ for i, x := range arr1 {
+ if p, ok := pos[x]; ok {
+ arr[i] = [2]int{p, x}
+ } else {
+ arr[i] = [2]int{len(arr2), x}
}
}
- for j, cnt := range mp {
- for cnt > 0 {
- arr1[i] = j
- i++
- cnt--
- }
+ sort.Slice(arr, func(i, j int) bool {
+ return arr[i][0] < arr[j][0] || arr[i][0] == arr[j][0] && arr[i][1] < arr[j][1]
+ })
+ for i, x := range arr {
+ arr1[i] = x[1]
}
return arr1
}
```
+#### TypeScript
+
+```ts
+function relativeSortArray(arr1: number[], arr2: number[]): number[] {
+ const pos: Map = new Map();
+ for (let i = 0; i < arr2.length; ++i) {
+ pos.set(arr2[i], i);
+ }
+ const arr: number[][] = [];
+ for (const x of arr1) {
+ const j = pos.get(x) ?? arr2.length;
+ arr.push([j, x]);
+ }
+ arr.sort((a, b) => a[0] - b[0] || a[1] - b[1]);
+ return arr.map(a => a[1]);
+}
+```
+
+#### Swift
+
+```swift
+class Solution {
+ func relativeSortArray(_ arr1: [Int], _ arr2: [Int]) -> [Int] {
+ var pos = [Int: Int]()
+ for (i, x) in arr2.enumerated() {
+ pos[x] = i
+ }
+ var arr = [(Int, Int)]()
+ for x in arr1 {
+ let j = pos[x] ?? arr2.count
+ arr.append((j, x))
+ }
+ arr.sort { $0.0 < $1.0 || ($0.0 == $1.0 && $0.1 < $1.1) }
+ return arr.map { $0.1 }
+ }
+}
+```
+
-
+
-### 方法二
+### 方法二:计数排序
-
+我们可以使用计数排序的思想,首先统计数组 $arr1$ 中每个元素的出现次数,然后按照数组 $arr2$ 中的顺序,将 $arr1$ 中的元素按照出现次数放入答案数组 $ans$ 中。最后,我们遍历 $arr1$ 中的所有元素,将未在 $arr2$ 中出现的元素按照升序放入答案数组 $ans$ 的末尾。
+
+时间复杂度 $O(n + m)$,空间复杂度 $O(n)$。其中 $n$ 和 $m$ 分别是数组 $arr1$ 和 $arr2$ 的长度。
+
+
#### Python3
```python
class Solution:
def relativeSortArray(self, arr1: List[int], arr2: List[int]) -> List[int]:
- mp = [0] * 1001
- for x in arr1:
- mp[x] += 1
- i = 0
+ cnt = Counter(arr1)
+ ans = []
for x in arr2:
- while mp[x] > 0:
- arr1[i] = x
- mp[x] -= 1
- i += 1
- for x, cnt in enumerate(mp):
- for _ in range(cnt):
- arr1[i] = x
- i += 1
- return arr1
+ ans.extend([x] * cnt[x])
+ cnt.pop(x)
+ mi, mx = min(arr1), max(arr1)
+ for x in range(mi, mx + 1):
+ ans.extend([x] * cnt[x])
+ return ans
+```
+
+#### Java
+
+```java
+class Solution {
+ public int[] relativeSortArray(int[] arr1, int[] arr2) {
+ int[] cnt = new int[1001];
+ int mi = 1001, mx = 0;
+ for (int x : arr1) {
+ ++cnt[x];
+ mi = Math.min(mi, x);
+ mx = Math.max(mx, x);
+ }
+ int m = arr1.length;
+ int[] ans = new int[m];
+ int i = 0;
+ for (int x : arr2) {
+ while (cnt[x] > 0) {
+ --cnt[x];
+ ans[i++] = x;
+ }
+ }
+ for (int x = mi; x <= mx; ++x) {
+ while (cnt[x] > 0) {
+ --cnt[x];
+ ans[i++] = x;
+ }
+ }
+ return ans;
+ }
+}
+```
+
+#### C++
+
+```cpp
+class Solution {
+public:
+ vector relativeSortArray(vector& arr1, vector& arr2) {
+ vector cnt(1001);
+ for (int x : arr1) {
+ ++cnt[x];
+ }
+ auto [mi, mx] = minmax_element(arr1.begin(), arr1.end());
+ vector ans;
+ for (int x : arr2) {
+ while (cnt[x]) {
+ ans.push_back(x);
+ --cnt[x];
+ }
+ }
+ for (int x = *mi; x <= *mx; ++x) {
+ while (cnt[x]) {
+ ans.push_back(x);
+ --cnt[x];
+ }
+ }
+ return ans;
+ }
+};
+```
+
+#### Go
+
+```go
+func relativeSortArray(arr1 []int, arr2 []int) []int {
+ cnt := make([]int, 1001)
+ mi, mx := 1001, 0
+ for _, x := range arr1 {
+ cnt[x]++
+ mi = min(mi, x)
+ mx = max(mx, x)
+ }
+ ans := make([]int, 0, len(arr1))
+ for _, x := range arr2 {
+ for cnt[x] > 0 {
+ ans = append(ans, x)
+ cnt[x]--
+ }
+ }
+ for x := mi; x <= mx; x++ {
+ for cnt[x] > 0 {
+ ans = append(ans, x)
+ cnt[x]--
+ }
+ }
+ return ans
+}
+```
+
+#### TypeScript
+
+```ts
+function relativeSortArray(arr1: number[], arr2: number[]): number[] {
+ const cnt = Array(1001).fill(0);
+ let mi = Number.POSITIVE_INFINITY;
+ let mx = Number.NEGATIVE_INFINITY;
+
+ for (const x of arr1) {
+ cnt[x]++;
+ mi = Math.min(mi, x);
+ mx = Math.max(mx, x);
+ }
+
+ const ans: number[] = [];
+ for (const x of arr2) {
+ while (cnt[x]) {
+ cnt[x]--;
+ ans.push(x);
+ }
+ }
+
+ for (let i = mi; i <= mx; i++) {
+ while (cnt[i]) {
+ cnt[i]--;
+ ans.push(i);
+ }
+ }
+
+ return ans;
+}
+```
+
+#### Swift
+
+```swift
+class Solution {
+ func relativeSortArray(_ arr1: [Int], _ arr2: [Int]) -> [Int] {
+ var cnt = [Int](repeating: 0, count: 1001)
+ for x in arr1 {
+ cnt[x] += 1
+ }
+
+ guard let mi = arr1.min(), let mx = arr1.max() else {
+ return []
+ }
+
+ var ans = [Int]()
+ for x in arr2 {
+ while cnt[x] > 0 {
+ ans.append(x)
+ cnt[x] -= 1
+ }
+ }
+
+ for x in mi...mx {
+ while cnt[x] > 0 {
+ ans.append(x)
+ cnt[x] -= 1
+ }
+ }
+
+ return ans
+ }
+}
```
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.cpp" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.cpp"
index 6b3c5fdd5b2c8..fcf60d27382a1 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.cpp"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.cpp"
@@ -1,14 +1,18 @@
class Solution {
public:
vector relativeSortArray(vector& arr1, vector& arr2) {
- vector mp(1001);
- for (int x : arr1) ++mp[x];
- int i = 0;
- for (int x : arr2) {
- while (mp[x]-- > 0) arr1[i++] = x;
+ unordered_map pos;
+ for (int i = 0; i < arr2.size(); ++i) {
+ pos[arr2[i]] = i;
}
- for (int j = 0; j < mp.size(); ++j) {
- while (mp[j]-- > 0) arr1[i++] = j;
+ vector> arr;
+ for (int i = 0; i < arr1.size(); ++i) {
+ int j = pos.count(arr1[i]) ? pos[arr1[i]] : arr2.size();
+ arr.emplace_back(j, arr1[i]);
+ }
+ sort(arr.begin(), arr.end());
+ for (int i = 0; i < arr1.size(); ++i) {
+ arr1[i] = arr[i].second;
}
return arr1;
}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.go" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.go"
index b20033dee2491..9c9cdd1191d98 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.go"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.go"
@@ -1,22 +1,21 @@
func relativeSortArray(arr1 []int, arr2 []int) []int {
- mp := make([]int, 1001)
- for _, x := range arr1 {
- mp[x]++
+ pos := map[int]int{}
+ for i, x := range arr2 {
+ pos[x] = i
}
- i := 0
- for _, x := range arr2 {
- for mp[x] > 0 {
- arr1[i] = x
- mp[x]--
- i++
+ arr := make([][2]int, len(arr1))
+ for i, x := range arr1 {
+ if p, ok := pos[x]; ok {
+ arr[i] = [2]int{p, x}
+ } else {
+ arr[i] = [2]int{len(arr2), x}
}
}
- for j, cnt := range mp {
- for cnt > 0 {
- arr1[i] = j
- i++
- cnt--
- }
+ sort.Slice(arr, func(i, j int) bool {
+ return arr[i][0] < arr[j][0] || arr[i][0] == arr[j][0] && arr[i][1] < arr[j][1]
+ })
+ for i, x := range arr {
+ arr1[i] = x[1]
}
return arr1
}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.java" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.java"
index 544cc51b93962..23afacc4be6ac 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.java"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.java"
@@ -1,19 +1,16 @@
class Solution {
public int[] relativeSortArray(int[] arr1, int[] arr2) {
- int[] mp = new int[1001];
- for (int x : arr1) {
- ++mp[x];
+ Map pos = new HashMap<>(arr2.length);
+ for (int i = 0; i < arr2.length; ++i) {
+ pos.put(arr2[i], i);
}
- int i = 0;
- for (int x : arr2) {
- while (mp[x]-- > 0) {
- arr1[i++] = x;
- }
+ int[][] arr = new int[arr1.length][0];
+ for (int i = 0; i < arr.length; ++i) {
+ arr[i] = new int[] {arr1[i], pos.getOrDefault(arr1[i], arr2.length + arr1[i])};
}
- for (int j = 0; j < mp.length; ++j) {
- while (mp[j]-- > 0) {
- arr1[i++] = j;
- }
+ Arrays.sort(arr, (a, b) -> a[1] - b[1]);
+ for (int i = 0; i < arr.length; ++i) {
+ arr1[i] = arr[i][0];
}
return arr1;
}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.py" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.py"
index 2d57297cd3d65..67a4ab1154ad1 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.py"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.py"
@@ -1,5 +1,4 @@
class Solution:
def relativeSortArray(self, arr1: List[int], arr2: List[int]) -> List[int]:
- mp = {num: i for i, num in enumerate(arr2)}
- arr1.sort(key=lambda x: (mp.get(x, 10000), x))
- return arr1
+ pos = {x: i for i, x in enumerate(arr2)}
+ return sorted(arr1, key=lambda x: pos.get(x, 1000 + x))
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.swift"
new file mode 100644
index 0000000000000..af048342c47b6
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.swift"
@@ -0,0 +1,15 @@
+class Solution {
+ func relativeSortArray(_ arr1: [Int], _ arr2: [Int]) -> [Int] {
+ var pos = [Int: Int]()
+ for (i, x) in arr2.enumerated() {
+ pos[x] = i
+ }
+ var arr = [(Int, Int)]()
+ for x in arr1 {
+ let j = pos[x] ?? arr2.count
+ arr.append((j, x))
+ }
+ arr.sort { $0.0 < $1.0 || ($0.0 == $1.0 && $0.1 < $1.1) }
+ return arr.map { $0.1 }
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.ts" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.ts"
new file mode 100644
index 0000000000000..c31bb85520964
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution.ts"
@@ -0,0 +1,13 @@
+function relativeSortArray(arr1: number[], arr2: number[]): number[] {
+ const pos: Map = new Map();
+ for (let i = 0; i < arr2.length; ++i) {
+ pos.set(arr2[i], i);
+ }
+ const arr: number[][] = [];
+ for (const x of arr1) {
+ const j = pos.get(x) ?? arr2.length;
+ arr.push([j, x]);
+ }
+ arr.sort((a, b) => a[0] - b[0] || a[1] - b[1]);
+ return arr.map(a => a[1]);
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.cpp" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.cpp"
new file mode 100644
index 0000000000000..8ceeb19fee19a
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.cpp"
@@ -0,0 +1,24 @@
+class Solution {
+public:
+ vector relativeSortArray(vector& arr1, vector& arr2) {
+ vector cnt(1001);
+ for (int x : arr1) {
+ ++cnt[x];
+ }
+ auto [mi, mx] = minmax_element(arr1.begin(), arr1.end());
+ vector ans;
+ for (int x : arr2) {
+ while (cnt[x]) {
+ ans.push_back(x);
+ --cnt[x];
+ }
+ }
+ for (int x = *mi; x <= *mx; ++x) {
+ while (cnt[x]) {
+ ans.push_back(x);
+ --cnt[x];
+ }
+ }
+ return ans;
+ }
+};
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.go" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.go"
new file mode 100644
index 0000000000000..cafbc511113b8
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.go"
@@ -0,0 +1,23 @@
+func relativeSortArray(arr1 []int, arr2 []int) []int {
+ cnt := make([]int, 1001)
+ mi, mx := 1001, 0
+ for _, x := range arr1 {
+ cnt[x]++
+ mi = min(mi, x)
+ mx = max(mx, x)
+ }
+ ans := make([]int, 0, len(arr1))
+ for _, x := range arr2 {
+ for cnt[x] > 0 {
+ ans = append(ans, x)
+ cnt[x]--
+ }
+ }
+ for x := mi; x <= mx; x++ {
+ for cnt[x] > 0 {
+ ans = append(ans, x)
+ cnt[x]--
+ }
+ }
+ return ans
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.java" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.java"
new file mode 100644
index 0000000000000..b7c284041e15c
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.java"
@@ -0,0 +1,27 @@
+class Solution {
+ public int[] relativeSortArray(int[] arr1, int[] arr2) {
+ int[] cnt = new int[1001];
+ int mi = 1001, mx = 0;
+ for (int x : arr1) {
+ ++cnt[x];
+ mi = Math.min(mi, x);
+ mx = Math.max(mx, x);
+ }
+ int m = arr1.length;
+ int[] ans = new int[m];
+ int i = 0;
+ for (int x : arr2) {
+ while (cnt[x] > 0) {
+ --cnt[x];
+ ans[i++] = x;
+ }
+ }
+ for (int x = mi; x <= mx; ++x) {
+ while (cnt[x] > 0) {
+ --cnt[x];
+ ans[i++] = x;
+ }
+ }
+ return ans;
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.py" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.py"
index fc44d1f6ea545..389ef3393ec94 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.py"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.py"
@@ -1,16 +1,11 @@
class Solution:
def relativeSortArray(self, arr1: List[int], arr2: List[int]) -> List[int]:
- mp = [0] * 1001
- for x in arr1:
- mp[x] += 1
- i = 0
+ cnt = Counter(arr1)
+ ans = []
for x in arr2:
- while mp[x] > 0:
- arr1[i] = x
- mp[x] -= 1
- i += 1
- for x, cnt in enumerate(mp):
- for _ in range(cnt):
- arr1[i] = x
- i += 1
- return arr1
+ ans.extend([x] * cnt[x])
+ cnt.pop(x)
+ mi, mx = min(arr1), max(arr1)
+ for x in range(mi, mx + 1):
+ ans.extend([x] * cnt[x])
+ return ans
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.swift"
new file mode 100644
index 0000000000000..c737ce8d1f4ba
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.swift"
@@ -0,0 +1,29 @@
+class Solution {
+ func relativeSortArray(_ arr1: [Int], _ arr2: [Int]) -> [Int] {
+ var cnt = [Int](repeating: 0, count: 1001)
+ for x in arr1 {
+ cnt[x] += 1
+ }
+
+ guard let mi = arr1.min(), let mx = arr1.max() else {
+ return []
+ }
+
+ var ans = [Int]()
+ for x in arr2 {
+ while cnt[x] > 0 {
+ ans.append(x)
+ cnt[x] -= 1
+ }
+ }
+
+ for x in mi...mx {
+ while cnt[x] > 0 {
+ ans.append(x)
+ cnt[x] -= 1
+ }
+ }
+
+ return ans
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.ts" "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.ts"
new file mode 100644
index 0000000000000..ce90520b6bcf8
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 075. \346\225\260\347\273\204\347\233\270\345\257\271\346\216\222\345\272\217/Solution2.ts"
@@ -0,0 +1,28 @@
+function relativeSortArray(arr1: number[], arr2: number[]): number[] {
+ const cnt = Array(1001).fill(0);
+ let mi = Number.POSITIVE_INFINITY;
+ let mx = Number.NEGATIVE_INFINITY;
+
+ for (const x of arr1) {
+ cnt[x]++;
+ mi = Math.min(mi, x);
+ mx = Math.max(mx, x);
+ }
+
+ const ans: number[] = [];
+ for (const x of arr2) {
+ while (cnt[x]) {
+ cnt[x]--;
+ ans.push(x);
+ }
+ }
+
+ for (let i = mi; i <= mx; i++) {
+ while (cnt[i]) {
+ cnt[i]--;
+ ans.push(i);
+ }
+ }
+
+ return ans;
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 076. \346\225\260\347\273\204\344\270\255\347\232\204\347\254\254 k \345\244\247\347\232\204\346\225\260\345\255\227/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 076. \346\225\260\347\273\204\344\270\255\347\232\204\347\254\254 k \345\244\247\347\232\204\346\225\260\345\255\227/README.md"
index 3832f7087e47a..7b9be98b5e3da 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 076. \346\225\260\347\273\204\344\270\255\347\232\204\347\254\254 k \345\244\247\347\232\204\346\225\260\345\255\227/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 076. \346\225\260\347\273\204\344\270\255\347\232\204\347\254\254 k \345\244\247\347\232\204\346\225\260\345\255\227/README.md"
@@ -180,6 +180,41 @@ func quickSort(nums []int, left, right, k int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func findKthLargest(_ nums: [Int], _ k: Int) -> Int {
+ var nums = nums
+ let n = nums.count
+ return quickSelect(&nums, 0, n - 1, n - k)
+ }
+
+ private func quickSelect(_ nums: inout [Int], _ left: Int, _ right: Int, _ k: Int) -> Int {
+ if left == right {
+ return nums[left]
+ }
+
+ var i = left - 1
+ var j = right + 1
+ let pivot = nums[(left + right) / 2]
+
+ while i < j {
+ repeat { i += 1 } while nums[i] < pivot
+ repeat { j -= 1 } while nums[j] > pivot
+ if i < j {
+ nums.swapAt(i, j)
+ }
+ }
+
+ if j < k {
+ return quickSelect(&nums, j + 1, right, k)
+ }
+ return quickSelect(&nums, left, j, k)
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 076. \346\225\260\347\273\204\344\270\255\347\232\204\347\254\254 k \345\244\247\347\232\204\346\225\260\345\255\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 076. \346\225\260\347\273\204\344\270\255\347\232\204\347\254\254 k \345\244\247\347\232\204\346\225\260\345\255\227/Solution.swift"
new file mode 100644
index 0000000000000..357311f343c0e
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 076. \346\225\260\347\273\204\344\270\255\347\232\204\347\254\254 k \345\244\247\347\232\204\346\225\260\345\255\227/Solution.swift"
@@ -0,0 +1,30 @@
+class Solution {
+ func findKthLargest(_ nums: [Int], _ k: Int) -> Int {
+ var nums = nums
+ let n = nums.count
+ return quickSelect(&nums, 0, n - 1, n - k)
+ }
+
+ private func quickSelect(_ nums: inout [Int], _ left: Int, _ right: Int, _ k: Int) -> Int {
+ if left == right {
+ return nums[left]
+ }
+
+ var i = left - 1
+ var j = right + 1
+ let pivot = nums[(left + right) / 2]
+
+ while i < j {
+ repeat { i += 1 } while nums[i] < pivot
+ repeat { j -= 1 } while nums[j] > pivot
+ if i < j {
+ nums.swapAt(i, j)
+ }
+ }
+
+ if j < k {
+ return quickSelect(&nums, j + 1, right, k)
+ }
+ return quickSelect(&nums, left, j, k)
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 077. \351\223\276\350\241\250\346\216\222\345\272\217/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 077. \351\223\276\350\241\250\346\216\222\345\272\217/README.md"
index 2cf786ba9d063..77c1c6ed37991 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 077. \351\223\276\350\241\250\346\216\222\345\272\217/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 077. \351\223\276\350\241\250\346\216\222\345\272\217/README.md"
@@ -377,6 +377,64 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+
+/** class ListNode {
+* var val: Int
+* var next: ListNode?
+* init() { self.val = 0; self.next = nil }
+* init(_ val: Int) { self.val = val; self.next = nil }
+* init(_ val: Int, _ next: ListNode?) { self.val = val; self.next = next }
+* }
+*/
+
+class Solution {
+ func sortList(_ head: ListNode?) -> ListNode? {
+ guard let head = head, head.next != nil else {
+ return head
+ }
+
+ var slow: ListNode? = head
+ var fast: ListNode? = head.next
+
+ while fast != nil && fast?.next != nil {
+ slow = slow?.next
+ fast = fast?.next?.next
+ }
+
+ let mid = slow?.next
+ slow?.next = nil
+
+ let left = sortList(head)
+ let right = sortList(mid)
+
+ return merge(left, right)
+ }
+
+ private func merge(_ l1: ListNode?, _ l2: ListNode?) -> ListNode? {
+ let dummy = ListNode()
+ var cur = dummy
+ var l1 = l1, l2 = l2
+
+ while let node1 = l1, let node2 = l2 {
+ if node1.val <= node2.val {
+ cur.next = node1
+ l1 = node1.next
+ } else {
+ cur.next = node2
+ l2 = node2.next
+ }
+ cur = cur.next!
+ }
+
+ cur.next = l1 ?? l2
+ return dummy.next
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 077. \351\223\276\350\241\250\346\216\222\345\272\217/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 077. \351\223\276\350\241\250\346\216\222\345\272\217/Solution.swift"
new file mode 100644
index 0000000000000..9ab81ab9303af
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 077. \351\223\276\350\241\250\346\216\222\345\272\217/Solution.swift"
@@ -0,0 +1,53 @@
+
+/** class ListNode {
+* var val: Int
+* var next: ListNode?
+* init() { self.val = 0; self.next = nil }
+* init(_ val: Int) { self.val = val; self.next = nil }
+* init(_ val: Int, _ next: ListNode?) { self.val = val; self.next = next }
+* }
+*/
+
+class Solution {
+ func sortList(_ head: ListNode?) -> ListNode? {
+ guard let head = head, head.next != nil else {
+ return head
+ }
+
+ var slow: ListNode? = head
+ var fast: ListNode? = head.next
+
+ while fast != nil && fast?.next != nil {
+ slow = slow?.next
+ fast = fast?.next?.next
+ }
+
+ let mid = slow?.next
+ slow?.next = nil
+
+ let left = sortList(head)
+ let right = sortList(mid)
+
+ return merge(left, right)
+ }
+
+ private func merge(_ l1: ListNode?, _ l2: ListNode?) -> ListNode? {
+ let dummy = ListNode()
+ var cur = dummy
+ var l1 = l1, l2 = l2
+
+ while let node1 = l1, let node2 = l2 {
+ if node1.val <= node2.val {
+ cur.next = node1
+ l1 = node1.next
+ } else {
+ cur.next = node2
+ l2 = node2.next
+ }
+ cur = cur.next!
+ }
+
+ cur.next = l1 ?? l2
+ return dummy.next
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 078. \345\220\210\345\271\266\346\216\222\345\272\217\351\223\276\350\241\250/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 078. \345\220\210\345\271\266\346\216\222\345\272\217\351\223\276\350\241\250/README.md"
index 46dda02934b66..4515d22dd972f 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 078. \345\220\210\345\271\266\346\216\222\345\272\217\351\223\276\350\241\250/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 078. \345\220\210\345\271\266\346\216\222\345\272\217\351\223\276\350\241\250/README.md"
@@ -363,6 +363,54 @@ def merge_two_lists(l1, l2)
end
```
+#### Swift
+
+```swift
+/** class ListNode {
+* var val: Int
+* var next: ListNode?
+* init() { self.val = 0; self.next = nil }
+* init(_ val: Int) { self.val = val; self.next = nil }
+* init(_ val: Int, _ next: ListNode?) { self.val = val; self.next = next }
+* }
+*/
+
+class Solution {
+ func mergeKLists(_ lists: [ListNode?]) -> ListNode? {
+ let n = lists.count
+ if n == 0 {
+ return nil
+ }
+
+ var mergedList: ListNode? = lists[0]
+ for i in 1.. ListNode? {
+ let dummy = ListNode()
+ var cur = dummy
+ var l1 = l1
+ var l2 = l2
+
+ while let node1 = l1, let node2 = l2 {
+ if node1.val <= node2.val {
+ cur.next = node1
+ l1 = node1.next
+ } else {
+ cur.next = node2
+ l2 = node2.next
+ }
+ cur = cur.next!
+ }
+ cur.next = l1 ?? l2
+ return dummy.next
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 078. \345\220\210\345\271\266\346\216\222\345\272\217\351\223\276\350\241\250/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 078. \345\220\210\345\271\266\346\216\222\345\272\217\351\223\276\350\241\250/Solution.swift"
new file mode 100644
index 0000000000000..d6c00ecb4c6b9
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 078. \345\220\210\345\271\266\346\216\222\345\272\217\351\223\276\350\241\250/Solution.swift"
@@ -0,0 +1,43 @@
+/** class ListNode {
+* var val: Int
+* var next: ListNode?
+* init() { self.val = 0; self.next = nil }
+* init(_ val: Int) { self.val = val; self.next = nil }
+* init(_ val: Int, _ next: ListNode?) { self.val = val; self.next = next }
+* }
+*/
+
+class Solution {
+ func mergeKLists(_ lists: [ListNode?]) -> ListNode? {
+ let n = lists.count
+ if n == 0 {
+ return nil
+ }
+
+ var mergedList: ListNode? = lists[0]
+ for i in 1.. ListNode? {
+ let dummy = ListNode()
+ var cur = dummy
+ var l1 = l1
+ var l2 = l2
+
+ while let node1 = l1, let node2 = l2 {
+ if node1.val <= node2.val {
+ cur.next = node1
+ l1 = node1.next
+ } else {
+ cur.next = node2
+ l2 = node2.next
+ }
+ cur = cur.next!
+ }
+ cur.next = l1 ?? l2
+ return dummy.next
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 079. \346\211\200\346\234\211\345\255\220\351\233\206/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 079. \346\211\200\346\234\211\345\255\220\351\233\206/README.md"
index f923f68cfd386..2a1e44460234b 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 079. \346\211\200\346\234\211\345\255\220\351\233\206/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 079. \346\211\200\346\234\211\345\255\220\351\233\206/README.md"
@@ -188,6 +188,27 @@ impl Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func subsets(_ nums: [Int]) -> [[Int]] {
+ var res = [[Int]]()
+ dfs(0, nums, [], &res)
+ return res
+ }
+
+ private func dfs(_ i: Int, _ nums: [Int], _ current: [Int], _ res: inout [[Int]]) {
+ res.append(current)
+ for j in i..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 079. \346\211\200\346\234\211\345\255\220\351\233\206/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 079. \346\211\200\346\234\211\345\255\220\351\233\206/Solution.swift"
new file mode 100644
index 0000000000000..d2a72c648b14f
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 079. \346\211\200\346\234\211\345\255\220\351\233\206/Solution.swift"
@@ -0,0 +1,16 @@
+class Solution {
+ func subsets(_ nums: [Int]) -> [[Int]] {
+ var res = [[Int]]()
+ dfs(0, nums, [], &res)
+ return res
+ }
+
+ private func dfs(_ i: Int, _ nums: [Int], _ current: [Int], _ res: inout [[Int]]) {
+ res.append(current)
+ for j in i.. [[Int]] {
+ var res = [[Int]]()
+ dfs(1, n, k, [], &res)
+ return res
+ }
+
+ private func dfs(_ start: Int, _ n: Int, _ k: Int, _ current: [Int], _ res: inout [[Int]]) {
+ if current.count == k {
+ res.append(current)
+ return
+ }
+
+ if start > n {
+ return
+ }
+
+ for i in start...n {
+ var newCurrent = current
+ newCurrent.append(i)
+ dfs(i + 1, n, k, newCurrent, &res)
+ }
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 080. \345\220\253\346\234\211 k \344\270\252\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 080. \345\220\253\346\234\211 k \344\270\252\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/Solution.swift"
new file mode 100644
index 0000000000000..8fcd987d69701
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 080. \345\220\253\346\234\211 k \344\270\252\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/Solution.swift"
@@ -0,0 +1,24 @@
+class Solution {
+ func combine(_ n: Int, _ k: Int) -> [[Int]] {
+ var res = [[Int]]()
+ dfs(1, n, k, [], &res)
+ return res
+ }
+
+ private func dfs(_ start: Int, _ n: Int, _ k: Int, _ current: [Int], _ res: inout [[Int]]) {
+ if current.count == k {
+ res.append(current)
+ return
+ }
+
+ if start > n {
+ return
+ }
+
+ for i in start...n {
+ var newCurrent = current
+ newCurrent.append(i)
+ dfs(i + 1, n, k, newCurrent, &res)
+ }
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 081. \345\205\201\350\256\270\351\207\215\345\244\215\351\200\211\346\213\251\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 081. \345\205\201\350\256\270\351\207\215\345\244\215\351\200\211\346\213\251\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/README.md"
index 5ea16337e40df..5eecf3be5eff7 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 081. \345\205\201\350\256\270\351\207\215\345\244\215\351\200\211\346\213\251\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 081. \345\205\201\350\256\270\351\207\215\345\244\215\351\200\211\346\213\251\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/README.md"
@@ -258,6 +258,38 @@ public class Solution
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var ans: [[Int]] = []
+ private var target: Int = 0
+ private var candidates: [Int] = []
+
+ func combinationSum(_ candidates: [Int], _ target: Int) -> [[Int]] {
+ self.ans = []
+ self.target = target
+ self.candidates = candidates
+ dfs(0, 0, [])
+ return ans
+ }
+
+ private func dfs(_ sum: Int, _ index: Int, _ current: [Int]) {
+ if sum == target {
+ ans.append(current)
+ return
+ }
+ if sum > target {
+ return
+ }
+ for i in index..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 081. \345\205\201\350\256\270\351\207\215\345\244\215\351\200\211\346\213\251\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 081. \345\205\201\350\256\270\351\207\215\345\244\215\351\200\211\346\213\251\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/Solution.swift"
new file mode 100644
index 0000000000000..ad2150ec3f31c
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 081. \345\205\201\350\256\270\351\207\215\345\244\215\351\200\211\346\213\251\345\205\203\347\264\240\347\232\204\347\273\204\345\220\210/Solution.swift"
@@ -0,0 +1,27 @@
+class Solution {
+ private var ans: [[Int]] = []
+ private var target: Int = 0
+ private var candidates: [Int] = []
+
+ func combinationSum(_ candidates: [Int], _ target: Int) -> [[Int]] {
+ self.ans = []
+ self.target = target
+ self.candidates = candidates
+ dfs(0, 0, [])
+ return ans
+ }
+
+ private func dfs(_ sum: Int, _ index: Int, _ current: [Int]) {
+ if sum == target {
+ ans.append(current)
+ return
+ }
+ if sum > target {
+ return
+ }
+ for i in index..>
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var ans: [[Int]] = []
+ private var candidates: [Int] = []
+ private var target: Int = 0
+
+ func combinationSum2(_ candidates: [Int], _ target: Int) -> [[Int]] {
+ self.ans = []
+ self.target = target
+ self.candidates = candidates.sorted()
+ dfs(0, 0, [])
+ return ans
+ }
+
+ private func dfs(_ index: Int, _ sum: Int, _ current: [Int]) {
+ if sum > target {
+ return
+ }
+ if sum == target {
+ ans.append(current)
+ return
+ }
+ for i in index.. index && candidates[i] == candidates[i - 1] {
+ continue
+ }
+ dfs(i + 1, sum + candidates[i], current + [candidates[i]])
+ }
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 082. \345\220\253\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\347\273\204\345\220\210/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 082. \345\220\253\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\347\273\204\345\220\210/Solution.swift"
new file mode 100644
index 0000000000000..acbf254fa8b79
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 082. \345\220\253\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\347\273\204\345\220\210/Solution.swift"
@@ -0,0 +1,29 @@
+class Solution {
+ private var ans: [[Int]] = []
+ private var candidates: [Int] = []
+ private var target: Int = 0
+
+ func combinationSum2(_ candidates: [Int], _ target: Int) -> [[Int]] {
+ self.ans = []
+ self.target = target
+ self.candidates = candidates.sorted()
+ dfs(0, 0, [])
+ return ans
+ }
+
+ private func dfs(_ index: Int, _ sum: Int, _ current: [Int]) {
+ if sum > target {
+ return
+ }
+ if sum == target {
+ ans.append(current)
+ return
+ }
+ for i in index.. index && candidates[i] == candidates[i - 1] {
+ continue
+ }
+ dfs(i + 1, sum + candidates[i], current + [candidates[i]])
+ }
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 083. \346\262\241\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 083. \346\262\241\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/README.md"
index 2114fa3d9b8e6..319bba66e05ce 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 083. \346\262\241\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 083. \346\262\241\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/README.md"
@@ -247,6 +247,38 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func permute(_ nums: [Int]) -> [[Int]] {
+ var res = [[Int]]()
+ var path = [Int]()
+ var used = [Bool](repeating: false, count: nums.count)
+ dfs(0, nums.count, nums, &used, &path, &res)
+ return res
+ }
+
+ private func dfs(
+ _ u: Int, _ n: Int, _ nums: [Int], _ used: inout [Bool], _ path: inout [Int], _ res: inout [[Int]]
+ ) {
+ if u == n {
+ res.append(path)
+ return
+ }
+ for i in 0..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 083. \346\262\241\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 083. \346\262\241\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/Solution.swift"
new file mode 100644
index 0000000000000..6f72730e15f81
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 083. \346\262\241\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/Solution.swift"
@@ -0,0 +1,27 @@
+class Solution {
+ func permute(_ nums: [Int]) -> [[Int]] {
+ var res = [[Int]]()
+ var path = [Int]()
+ var used = [Bool](repeating: false, count: nums.count)
+ dfs(0, nums.count, nums, &used, &path, &res)
+ return res
+ }
+
+ private func dfs(
+ _ u: Int, _ n: Int, _ nums: [Int], _ used: inout [Bool], _ path: inout [Int], _ res: inout [[Int]]
+ ) {
+ if u == n {
+ res.append(path)
+ return
+ }
+ for i in 0.. [[Int]] {
+ var res = [[Int]]()
+ var path = [Int]()
+ var used = [Bool](repeating: false, count: nums.count)
+ let sortedNums = nums.sorted()
+ dfs(0, sortedNums.count, sortedNums, &used, &path, &res)
+ return res
+ }
+
+ private func dfs(
+ _ u: Int, _ n: Int, _ nums: [Int], _ used: inout [Bool], _ path: inout [Int], _ res: inout [[Int]]
+ ) {
+ if u == n {
+ res.append(path)
+ return
+ }
+ for i in 0.. 0 && nums[i] == nums[i - 1] && !used[i - 1]) {
+ continue
+ }
+ path.append(nums[i])
+ used[i] = true
+ dfs(u + 1, n, nums, &used, &path, &res)
+ used[i] = false
+ path.removeLast()
+ }
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 084. \345\220\253\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 084. \345\220\253\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/Solution.swift"
new file mode 100644
index 0000000000000..4e9d6b55b69d8
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 084. \345\220\253\346\234\211\351\207\215\345\244\215\345\205\203\347\264\240\351\233\206\345\220\210\347\232\204\345\205\250\346\216\222\345\210\227/Solution.swift"
@@ -0,0 +1,29 @@
+class Solution {
+ func permuteUnique(_ nums: [Int]) -> [[Int]] {
+ var res = [[Int]]()
+ var path = [Int]()
+ var used = [Bool](repeating: false, count: nums.count)
+ let sortedNums = nums.sorted()
+ dfs(0, sortedNums.count, sortedNums, &used, &path, &res)
+ return res
+ }
+
+ private func dfs(
+ _ u: Int, _ n: Int, _ nums: [Int], _ used: inout [Bool], _ path: inout [Int], _ res: inout [[Int]]
+ ) {
+ if u == n {
+ res.append(path)
+ return
+ }
+ for i in 0.. 0 && nums[i] == nums[i - 1] && !used[i - 1]) {
+ continue
+ }
+ path.append(nums[i])
+ used[i] = true
+ dfs(u + 1, n, nums, &used, &path, &res)
+ used[i] = false
+ path.removeLast()
+ }
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 085. \347\224\237\346\210\220\345\214\271\351\205\215\347\232\204\346\213\254\345\217\267/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 085. \347\224\237\346\210\220\345\214\271\351\205\215\347\232\204\346\213\254\345\217\267/README.md"
index 80e7c55cfd3ff..53f40338c844e 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 085. \347\224\237\346\210\220\345\214\271\351\205\215\347\232\204\346\213\254\345\217\267/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 085. \347\224\237\346\210\220\345\214\271\351\205\215\347\232\204\346\213\254\345\217\267/README.md"
@@ -188,6 +188,31 @@ var generateParenthesis = function (n) {
};
```
+#### Swift
+
+```swift
+class Solution {
+ func generateParenthesis(_ n: Int) -> [String] {
+ var ans = [String]()
+ dfs(0, 0, n, "", &ans)
+ return ans
+ }
+
+ private func dfs(_ left: Int, _ right: Int, _ n: Int, _ t: String, _ ans: inout [String]) {
+ if left == n && right == n {
+ ans.append(t)
+ return
+ }
+ if left < n {
+ dfs(left + 1, right, n, t + "(", &ans)
+ }
+ if right < left {
+ dfs(left, right + 1, n, t + ")", &ans)
+ }
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 085. \347\224\237\346\210\220\345\214\271\351\205\215\347\232\204\346\213\254\345\217\267/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 085. \347\224\237\346\210\220\345\214\271\351\205\215\347\232\204\346\213\254\345\217\267/Solution.swift"
new file mode 100644
index 0000000000000..ebda6543e2334
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 085. \347\224\237\346\210\220\345\214\271\351\205\215\347\232\204\346\213\254\345\217\267/Solution.swift"
@@ -0,0 +1,20 @@
+class Solution {
+ func generateParenthesis(_ n: Int) -> [String] {
+ var ans = [String]()
+ dfs(0, 0, n, "", &ans)
+ return ans
+ }
+
+ private func dfs(_ left: Int, _ right: Int, _ n: Int, _ t: String, _ ans: inout [String]) {
+ if left == n && right == n {
+ ans.append(t)
+ return
+ }
+ if left < n {
+ dfs(left + 1, right, n, t + "(", &ans)
+ }
+ if right < left {
+ dfs(left, right + 1, n, t + ")", &ans)
+ }
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 086. \345\210\206\345\211\262\345\233\236\346\226\207\345\255\220\345\255\227\347\254\246\344\270\262/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 086. \345\210\206\345\211\262\345\233\236\346\226\207\345\255\220\345\255\227\347\254\246\344\270\262/README.md"
index 29b5e9db2fcee..30e36f8da74c8 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 086. \345\210\206\345\211\262\345\233\236\346\226\207\345\255\220\345\255\227\347\254\246\344\270\262/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 086. \345\210\206\345\211\262\345\233\236\346\226\207\345\255\220\345\255\227\347\254\246\344\270\262/README.md"
@@ -285,6 +285,49 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var n: Int = 0
+ private var s: String = ""
+ private var f: [[Bool]] = []
+ private var t: [String] = []
+ private var ans: [[String]] = []
+
+ func partition(_ s: String) -> [[String]] {
+ n = s.count
+ self.s = s
+ f = Array(repeating: Array(repeating: true, count: n), count: n)
+
+ let chars = Array(s)
+
+ for i in stride(from: n - 1, through: 0, by: -1) {
+ for j in i + 1 ..< n {
+ f[i][j] = chars[i] == chars[j] && f[i + 1][j - 1]
+ }
+ }
+
+ dfs(0)
+ return ans
+ }
+
+ private func dfs(_ i: Int) {
+ if i == n {
+ ans.append(t)
+ return
+ }
+ for j in i ..< n {
+ if f[i][j] {
+ t.append(String(s[s.index(s.startIndex, offsetBy: i)...s.index(s.startIndex, offsetBy: j)]))
+ dfs(j + 1)
+ t.removeLast()
+ }
+ }
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 086. \345\210\206\345\211\262\345\233\236\346\226\207\345\255\220\345\255\227\347\254\246\344\270\262/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 086. \345\210\206\345\211\262\345\233\236\346\226\207\345\255\220\345\255\227\347\254\246\344\270\262/Solution.swift"
new file mode 100644
index 0000000000000..23a5d4200ef8d
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 086. \345\210\206\345\211\262\345\233\236\346\226\207\345\255\220\345\255\227\347\254\246\344\270\262/Solution.swift"
@@ -0,0 +1,38 @@
+class Solution {
+ private var n: Int = 0
+ private var s: String = ""
+ private var f: [[Bool]] = []
+ private var t: [String] = []
+ private var ans: [[String]] = []
+
+ func partition(_ s: String) -> [[String]] {
+ n = s.count
+ self.s = s
+ f = Array(repeating: Array(repeating: true, count: n), count: n)
+
+ let chars = Array(s)
+
+ for i in stride(from: n - 1, through: 0, by: -1) {
+ for j in i + 1 ..< n {
+ f[i][j] = chars[i] == chars[j] && f[i + 1][j - 1]
+ }
+ }
+
+ dfs(0)
+ return ans
+ }
+
+ private func dfs(_ i: Int) {
+ if i == n {
+ ans.append(t)
+ return
+ }
+ for j in i ..< n {
+ if f[i][j] {
+ t.append(String(s[s.index(s.startIndex, offsetBy: i)...s.index(s.startIndex, offsetBy: j)]))
+ dfs(j + 1)
+ t.removeLast()
+ }
+ }
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 087. \345\244\215\345\216\237 IP/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 087. \345\244\215\345\216\237 IP/README.md"
index 68621fb5d7e99..6d2ad9326d3dc 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 087. \345\244\215\345\216\237 IP/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 087. \345\244\215\345\216\237 IP/README.md"
@@ -288,6 +288,45 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var n: Int = 0
+ private var s: String = ""
+ private var ans: [String] = []
+ private var t: [String] = []
+
+ func restoreIpAddresses(_ s: String) -> [String] {
+ n = s.count
+ self.s = s
+ dfs(0)
+ return ans
+ }
+
+ private func dfs(_ i: Int) {
+ if i >= n && t.count == 4 {
+ ans.append(t.joined(separator: "."))
+ return
+ }
+ if i >= n || t.count >= 4 {
+ return
+ }
+ var x = 0
+ let chars = Array(s)
+ for j in i.. 255 || (chars[i] == "0" && i != j) {
+ break
+ }
+ t.append(String(chars[i...j]))
+ dfs(j + 1)
+ t.removeLast()
+ }
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 087. \345\244\215\345\216\237 IP/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 087. \345\244\215\345\216\237 IP/Solution.swift"
new file mode 100644
index 0000000000000..bd98392ad19e7
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 087. \345\244\215\345\216\237 IP/Solution.swift"
@@ -0,0 +1,34 @@
+class Solution {
+ private var n: Int = 0
+ private var s: String = ""
+ private var ans: [String] = []
+ private var t: [String] = []
+
+ func restoreIpAddresses(_ s: String) -> [String] {
+ n = s.count
+ self.s = s
+ dfs(0)
+ return ans
+ }
+
+ private func dfs(_ i: Int) {
+ if i >= n && t.count == 4 {
+ ans.append(t.joined(separator: "."))
+ return
+ }
+ if i >= n || t.count >= 4 {
+ return
+ }
+ var x = 0
+ let chars = Array(s)
+ for j in i.. 255 || (chars[i] == "0" && i != j) {
+ break
+ }
+ t.append(String(chars[i...j]))
+ dfs(j + 1)
+ t.removeLast()
+ }
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/README.md"
index e4430696431d1..939f08d17f275 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/README.md"
@@ -141,6 +141,21 @@ function minCostClimbingStairs(cost: number[]): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minCostClimbingStairs(_ cost: [Int]) -> Int {
+ let n = cost.count
+ var dp = Array(repeating: 0, count: n + 1)
+ for i in 2...n {
+ dp[i] = min(dp[i - 1] + cost[i - 1], dp[i - 2] + cost[i - 2])
+ }
+ return dp[n]
+ }
+}
+```
+
@@ -220,6 +235,23 @@ function minCostClimbingStairs(cost: number[]): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minCostClimbingStairs(_ cost: [Int]) -> Int {
+ var a = 0
+ var b = 0
+ for i in 1..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/Solution.swift"
new file mode 100644
index 0000000000000..520656f71ffcb
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/Solution.swift"
@@ -0,0 +1,10 @@
+class Solution {
+ func minCostClimbingStairs(_ cost: [Int]) -> Int {
+ let n = cost.count
+ var dp = Array(repeating: 0, count: n + 1)
+ for i in 2...n {
+ dp[i] = min(dp[i - 1] + cost[i - 1], dp[i - 2] + cost[i - 2])
+ }
+ return dp[n]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/Solution2.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/Solution2.swift"
new file mode 100644
index 0000000000000..c8c37db6d9938
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 088. \347\210\254\346\245\274\346\242\257\347\232\204\346\234\200\345\260\221\346\210\220\346\234\254/Solution2.swift"
@@ -0,0 +1,12 @@
+class Solution {
+ func minCostClimbingStairs(_ cost: [Int]) -> Int {
+ var a = 0
+ var b = 0
+ for i in 1.. Int {
+ let n = nums.count
+ if n == 0 { return 0 }
+ if n == 1 { return nums[0] }
+
+ var f = Array(repeating: 0, count: n + 1)
+ f[1] = nums[0]
+
+ for i in 2...n {
+ f[i] = max(f[i - 1], f[i - 2] + nums[i - 1])
+ }
+
+ return f[n]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 089. \346\210\277\345\261\213\345\201\267\347\233\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 089. \346\210\277\345\261\213\345\201\267\347\233\227/Solution.swift"
new file mode 100644
index 0000000000000..fb5eb31d3781c
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 089. \346\210\277\345\261\213\345\201\267\347\233\227/Solution.swift"
@@ -0,0 +1,16 @@
+class Solution {
+ func rob(_ nums: [Int]) -> Int {
+ let n = nums.count
+ if n == 0 { return 0 }
+ if n == 1 { return nums[0] }
+
+ var f = Array(repeating: 0, count: n + 1)
+ f[1] = nums[0]
+
+ for i in 2...n {
+ f[i] = max(f[i - 1], f[i - 2] + nums[i - 1])
+ }
+
+ return f[n]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 090. \347\216\257\345\275\242\346\210\277\345\261\213\345\201\267\347\233\227/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 090. \347\216\257\345\275\242\346\210\277\345\261\213\345\201\267\347\233\227/README.md"
index cbdde236ac876..a5078e70925c3 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 090. \347\216\257\345\275\242\346\210\277\345\261\213\345\201\267\347\233\227/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 090. \347\216\257\345\275\242\346\210\277\345\261\213\345\201\267\347\233\227/README.md"
@@ -192,6 +192,30 @@ impl Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func rob(_ nums: [Int]) -> Int {
+ let n = nums.count
+ if n == 1 {
+ return nums[0]
+ }
+ return max(rob(nums, 0, n - 2), rob(nums, 1, n - 1))
+ }
+
+ private func rob(_ nums: [Int], _ l: Int, _ r: Int) -> Int {
+ var f = 0, g = 0
+ for i in l...r {
+ let temp = max(f, g)
+ g = f + nums[i]
+ f = temp
+ }
+ return max(f, g)
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 090. \347\216\257\345\275\242\346\210\277\345\261\213\345\201\267\347\233\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 090. \347\216\257\345\275\242\346\210\277\345\261\213\345\201\267\347\233\227/Solution.swift"
new file mode 100644
index 0000000000000..bdfbc1be01907
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 090. \347\216\257\345\275\242\346\210\277\345\261\213\345\201\267\347\233\227/Solution.swift"
@@ -0,0 +1,19 @@
+class Solution {
+ func rob(_ nums: [Int]) -> Int {
+ let n = nums.count
+ if n == 1 {
+ return nums[0]
+ }
+ return max(rob(nums, 0, n - 2), rob(nums, 1, n - 1))
+ }
+
+ private func rob(_ nums: [Int], _ l: Int, _ r: Int) -> Int {
+ var f = 0, g = 0
+ for i in l...r {
+ let temp = max(f, g)
+ g = f + nums[i]
+ f = temp
+ }
+ return max(f, g)
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 091. \347\262\211\345\210\267\346\210\277\345\255\220/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 091. \347\262\211\345\210\267\346\210\277\345\255\220/README.md"
index f47a979f234bd..18fc2689ec077 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 091. \347\262\211\345\210\267\346\210\277\345\255\220/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 091. \347\262\211\345\210\267\346\210\277\345\255\220/README.md"
@@ -156,6 +156,23 @@ impl Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minCost(_ costs: [[Int]]) -> Int {
+ var r = 0, g = 0, b = 0
+ for cost in costs {
+ let _r = r, _g = g, _b = b
+ r = min(_g, _b) + cost[0]
+ g = min(_r, _b) + cost[1]
+ b = min(_r, _g) + cost[2]
+ }
+ return min(r, min(g, b))
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 091. \347\262\211\345\210\267\346\210\277\345\255\220/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 091. \347\262\211\345\210\267\346\210\277\345\255\220/Solution.swift"
new file mode 100644
index 0000000000000..cd1286c1d41e0
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 091. \347\262\211\345\210\267\346\210\277\345\255\220/Solution.swift"
@@ -0,0 +1,12 @@
+class Solution {
+ func minCost(_ costs: [[Int]]) -> Int {
+ var r = 0, g = 0, b = 0
+ for cost in costs {
+ let _r = r, _g = g, _b = b
+ r = min(_g, _b) + cost[0]
+ g = min(_r, _b) + cost[1]
+ b = min(_r, _g) + cost[2]
+ }
+ return min(r, min(g, b))
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 092. \347\277\273\350\275\254\345\255\227\347\254\246/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 092. \347\277\273\350\275\254\345\255\227\347\254\246/README.md"
index 799a58460f4de..4b70101d608db 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 092. \347\277\273\350\275\254\345\255\227\347\254\246/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 092. \347\277\273\350\275\254\345\255\227\347\254\246/README.md"
@@ -180,6 +180,35 @@ function minFlipsMonoIncr(s: string): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minFlipsMonoIncr(_ s: String) -> Int {
+ let n = s.count
+ var left0 = 0, right0 = 0
+ let chars = Array(s)
+
+ for char in chars {
+ if char == "0" {
+ right0 += 1
+ }
+ }
+
+ var ans = min(right0, n - right0)
+
+ for i in 1...n {
+ let x = chars[i - 1] == "0" ? 0 : 1
+ right0 -= x ^ 1
+ left0 += x ^ 1
+ ans = min(ans, i - left0 + right0)
+ }
+
+ return ans
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 092. \347\277\273\350\275\254\345\255\227\347\254\246/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 092. \347\277\273\350\275\254\345\255\227\347\254\246/Solution.swift"
new file mode 100644
index 0000000000000..9a21105516927
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 092. \347\277\273\350\275\254\345\255\227\347\254\246/Solution.swift"
@@ -0,0 +1,24 @@
+class Solution {
+ func minFlipsMonoIncr(_ s: String) -> Int {
+ let n = s.count
+ var left0 = 0, right0 = 0
+ let chars = Array(s)
+
+ for char in chars {
+ if char == "0" {
+ right0 += 1
+ }
+ }
+
+ var ans = min(right0, n - right0)
+
+ for i in 1...n {
+ let x = chars[i - 1] == "0" ? 0 : 1
+ right0 -= x ^ 1
+ left0 += x ^ 1
+ ans = min(ans, i - left0 + right0)
+ }
+
+ return ans
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 093. \346\234\200\351\225\277\346\226\220\346\263\242\351\202\243\345\245\221\346\225\260\345\210\227/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 093. \346\234\200\351\225\277\346\226\220\346\263\242\351\202\243\345\245\221\346\225\260\345\210\227/README.md"
index 7bece72ba906c..feb0c3507b3d6 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 093. \346\234\200\351\225\277\346\226\220\346\263\242\351\202\243\345\245\221\346\225\260\345\210\227/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 093. \346\234\200\351\225\277\346\226\220\346\263\242\351\202\243\345\245\221\346\225\260\345\210\227/README.md"
@@ -188,6 +188,35 @@ func lenLongestFibSubseq(arr []int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func lenLongestFibSubseq(_ arr: [Int]) -> Int {
+ let n = arr.count
+ var mp = [Int: Int]()
+ for i in 0.. 2 ? ans : 0
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 093. \346\234\200\351\225\277\346\226\220\346\263\242\351\202\243\345\245\221\346\225\260\345\210\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 093. \346\234\200\351\225\277\346\226\220\346\263\242\351\202\243\345\245\221\346\225\260\345\210\227/Solution.swift"
new file mode 100644
index 0000000000000..4149dafe4b8c2
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 093. \346\234\200\351\225\277\346\226\220\346\263\242\351\202\243\345\245\221\346\225\260\345\210\227/Solution.swift"
@@ -0,0 +1,24 @@
+class Solution {
+ func lenLongestFibSubseq(_ arr: [Int]) -> Int {
+ let n = arr.count
+ var mp = [Int: Int]()
+ for i in 0.. 2 ? ans : 0
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 094. \346\234\200\345\260\221\345\233\236\346\226\207\345\210\206\345\211\262/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 094. \346\234\200\345\260\221\345\233\236\346\226\207\345\210\206\345\211\262/README.md"
index bb55fa65ac8e7..1e5468831a809 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 094. \346\234\200\345\260\221\345\233\236\346\226\207\345\210\206\345\211\262/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 094. \346\234\200\345\260\221\345\233\236\346\226\207\345\210\206\345\211\262/README.md"
@@ -249,6 +249,40 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minCut(_ s: String) -> Int {
+ let n = s.count
+ let sArray = Array(s)
+
+ var g = Array(repeating: Array(repeating: true, count: n), count: n)
+
+ for i in stride(from: n - 1, through: 0, by: -1) {
+ for j in i + 1.. 0 ? 1 + f[j - 1] : 0)
+ }
+ }
+ }
+
+ return f[n - 1]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 094. \346\234\200\345\260\221\345\233\236\346\226\207\345\210\206\345\211\262/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 094. \346\234\200\345\260\221\345\233\236\346\226\207\345\210\206\345\211\262/Solution.swift"
new file mode 100644
index 0000000000000..94f93ea9befa0
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 094. \346\234\200\345\260\221\345\233\236\346\226\207\345\210\206\345\211\262/Solution.swift"
@@ -0,0 +1,29 @@
+class Solution {
+ func minCut(_ s: String) -> Int {
+ let n = s.count
+ let sArray = Array(s)
+
+ var g = Array(repeating: Array(repeating: true, count: n), count: n)
+
+ for i in stride(from: n - 1, through: 0, by: -1) {
+ for j in i + 1.. 0 ? 1 + f[j - 1] : 0)
+ }
+ }
+ }
+
+ return f[n - 1]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 095. \346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 095. \346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227/README.md"
index eafbc0baaa62c..ed70055c0ae18 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 095. \346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 095. \346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227/README.md"
@@ -275,6 +275,30 @@ class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func longestCommonSubsequence(_ text1: String, _ text2: String) -> Int {
+ let m = text1.count, n = text2.count
+ let text1Array = Array(text1)
+ let text2Array = Array(text2)
+ var f = Array(repeating: Array(repeating: 0, count: n + 1), count: m + 1)
+
+ for i in 1...m {
+ for j in 1...n {
+ if text1Array[i - 1] == text2Array[j - 1] {
+ f[i][j] = f[i - 1][j - 1] + 1
+ } else {
+ f[i][j] = max(f[i - 1][j], f[i][j - 1])
+ }
+ }
+ }
+ return f[m][n]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 095. \346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 095. \346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227/Solution.swift"
new file mode 100644
index 0000000000000..33b58c9c221ab
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 095. \346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227/Solution.swift"
@@ -0,0 +1,19 @@
+class Solution {
+ func longestCommonSubsequence(_ text1: String, _ text2: String) -> Int {
+ let m = text1.count, n = text2.count
+ let text1Array = Array(text1)
+ let text2Array = Array(text2)
+ var f = Array(repeating: Array(repeating: 0, count: n + 1), count: m + 1)
+
+ for i in 1...m {
+ for j in 1...n {
+ if text1Array[i - 1] == text2Array[j - 1] {
+ f[i][j] = f[i - 1][j - 1] + 1
+ } else {
+ f[i][j] = max(f[i - 1][j], f[i][j - 1])
+ }
+ }
+ }
+ return f[m][n]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/README.md"
index 7303593e9a389..3bbc5e813d5bb 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/README.md"
@@ -290,6 +290,51 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var memo = [String: Bool]()
+ private var s1: [Character] = []
+ private var s2: [Character] = []
+ private var s3: [Character] = []
+ private var m = 0
+ private var n = 0
+
+ func isInterleave(_ s1: String, _ s2: String, _ s3: String) -> Bool {
+ m = s1.count
+ n = s2.count
+ if m + n != s3.count {
+ return false
+ }
+ self.s1 = Array(s1)
+ self.s2 = Array(s2)
+ self.s3 = Array(s3)
+ return dfs(0, 0)
+ }
+
+ private func dfs(_ i: Int, _ j: Int) -> Bool {
+ if i >= m && j >= n {
+ return true
+ }
+ let key = "\(i),\(j)"
+ if let cached = memo[key] {
+ return cached
+ }
+ let k = i + j
+ var ans = false
+ if i < m && s1[i] == s3[k] && dfs(i + 1, j) {
+ ans = true
+ }
+ if !ans && j < n && s2[j] == s3[k] && dfs(i, j + 1) {
+ ans = true
+ }
+ memo[key] = ans
+ return ans
+ }
+}
+```
+
@@ -640,6 +685,37 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func isInterleave(_ s1: String, _ s2: String, _ s3: String) -> Bool {
+ let m = s1.count, n = s2.count
+ if m + n != s3.count {
+ return false
+ }
+
+ let s1 = Array(s1), s2 = Array(s2), s3 = Array(s3)
+ var dp = Array(repeating: Array(repeating: false, count: n + 1), count: m + 1)
+ dp[0][0] = true
+
+ for i in 0...m {
+ for j in 0...n {
+ let k = i + j - 1
+ if i > 0 && s1[i - 1] == s3[k] {
+ dp[i][j] = dp[i][j] || dp[i - 1][j]
+ }
+ if j > 0 && s2[j - 1] == s3[k] {
+ dp[i][j] = dp[i][j] || dp[i][j - 1]
+ }
+ }
+ }
+
+ return dp[m][n]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/Solution.swift"
new file mode 100644
index 0000000000000..d6fca9ca354cb
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/Solution.swift"
@@ -0,0 +1,40 @@
+class Solution {
+ private var memo = [String: Bool]()
+ private var s1: [Character] = []
+ private var s2: [Character] = []
+ private var s3: [Character] = []
+ private var m = 0
+ private var n = 0
+
+ func isInterleave(_ s1: String, _ s2: String, _ s3: String) -> Bool {
+ m = s1.count
+ n = s2.count
+ if m + n != s3.count {
+ return false
+ }
+ self.s1 = Array(s1)
+ self.s2 = Array(s2)
+ self.s3 = Array(s3)
+ return dfs(0, 0)
+ }
+
+ private func dfs(_ i: Int, _ j: Int) -> Bool {
+ if i >= m && j >= n {
+ return true
+ }
+ let key = "\(i),\(j)"
+ if let cached = memo[key] {
+ return cached
+ }
+ let k = i + j
+ var ans = false
+ if i < m && s1[i] == s3[k] && dfs(i + 1, j) {
+ ans = true
+ }
+ if !ans && j < n && s2[j] == s3[k] && dfs(i, j + 1) {
+ ans = true
+ }
+ memo[key] = ans
+ return ans
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/Solution2.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/Solution2.swift"
new file mode 100644
index 0000000000000..4015d37c0b462
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 096. \345\255\227\347\254\246\344\270\262\344\272\244\347\273\207/Solution2.swift"
@@ -0,0 +1,26 @@
+class Solution {
+ func isInterleave(_ s1: String, _ s2: String, _ s3: String) -> Bool {
+ let m = s1.count, n = s2.count
+ if m + n != s3.count {
+ return false
+ }
+
+ let s1 = Array(s1), s2 = Array(s2), s3 = Array(s3)
+ var dp = Array(repeating: Array(repeating: false, count: n + 1), count: m + 1)
+ dp[0][0] = true
+
+ for i in 0...m {
+ for j in 0...n {
+ let k = i + j - 1
+ if i > 0 && s1[i - 1] == s3[k] {
+ dp[i][j] = dp[i][j] || dp[i - 1][j]
+ }
+ if j > 0 && s2[j - 1] == s3[k] {
+ dp[i][j] = dp[i][j] || dp[i][j - 1]
+ }
+ }
+ }
+
+ return dp[m][n]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 097. \345\255\220\345\272\217\345\210\227\347\232\204\346\225\260\347\233\256/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 097. \345\255\220\345\272\217\345\210\227\347\232\204\346\225\260\347\233\256/README.md"
index b0d69eacdb89f..d5daf6b21c940 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 097. \345\255\220\345\272\217\345\210\227\347\232\204\346\225\260\347\233\256/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 097. \345\255\220\345\272\217\345\210\227\347\232\204\346\225\260\347\233\256/README.md"
@@ -202,6 +202,36 @@ function numDistinct(s: string, t: string): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func numDistinct(_ s: String, _ t: String) -> Int {
+ let m = s.count, n = t.count
+ let sArray = Array(s)
+ let tArray = Array(t)
+
+ var dp = Array(repeating: Array(repeating: 0, count: n + 1), count: m + 1)
+
+
+ for i in 0...m {
+ dp[i][0] = 1
+ }
+
+ for i in 1...m {
+ for j in 1...n {
+ dp[i][j] = dp[i - 1][j]
+ if sArray[i - 1] == tArray[j - 1] {
+ dp[i][j] += dp[i - 1][j - 1]
+ }
+ }
+ }
+
+ return dp[m][n]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 097. \345\255\220\345\272\217\345\210\227\347\232\204\346\225\260\347\233\256/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 097. \345\255\220\345\272\217\345\210\227\347\232\204\346\225\260\347\233\256/Solution.swift"
new file mode 100644
index 0000000000000..7043361fd52ef
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 097. \345\255\220\345\272\217\345\210\227\347\232\204\346\225\260\347\233\256/Solution.swift"
@@ -0,0 +1,25 @@
+class Solution {
+ func numDistinct(_ s: String, _ t: String) -> Int {
+ let m = s.count, n = t.count
+ let sArray = Array(s)
+ let tArray = Array(t)
+
+ var dp = Array(repeating: Array(repeating: 0, count: n + 1), count: m + 1)
+
+
+ for i in 0...m {
+ dp[i][0] = 1
+ }
+
+ for i in 1...m {
+ for j in 1...n {
+ dp[i][j] = dp[i - 1][j]
+ if sArray[i - 1] == tArray[j - 1] {
+ dp[i][j] += dp[i - 1][j - 1]
+ }
+ }
+ }
+
+ return dp[m][n]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/README.md"
index cb03911942f59..403c34d0afcc8 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/README.md"
@@ -247,6 +247,30 @@ var uniquePaths = function (m, n) {
};
```
+#### Swift
+
+```swift
+class Solution {
+ func uniquePaths(_ m: Int, _ n: Int) -> Int {
+ var dp = Array(repeating: Array(repeating: 0, count: n), count: m)
+ dp[0][0] = 1
+
+ for i in 0.. 0 {
+ dp[i][j] += dp[i - 1][j]
+ }
+ if j > 0 {
+ dp[i][j] += dp[i][j - 1]
+ }
+ }
+ }
+
+ return dp[m - 1][n - 1]
+ }
+}
+```
+
@@ -362,6 +386,24 @@ var uniquePaths = function (m, n) {
};
```
+#### Swift
+
+```swift
+class Solution {
+ func uniquePaths(_ m: Int, _ n: Int) -> Int {
+ var dp = Array(repeating: Array(repeating: 1, count: n), count: m)
+
+ for i in 1..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/Solution.swift"
new file mode 100644
index 0000000000000..179dadb90bd5e
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/Solution.swift"
@@ -0,0 +1,19 @@
+class Solution {
+ func uniquePaths(_ m: Int, _ n: Int) -> Int {
+ var dp = Array(repeating: Array(repeating: 0, count: n), count: m)
+ dp[0][0] = 1
+
+ for i in 0.. 0 {
+ dp[i][j] += dp[i - 1][j]
+ }
+ if j > 0 {
+ dp[i][j] += dp[i][j - 1]
+ }
+ }
+ }
+
+ return dp[m - 1][n - 1]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/Solution2.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/Solution2.swift"
new file mode 100644
index 0000000000000..8f39c1cb87188
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 098. \350\267\257\345\276\204\347\232\204\346\225\260\347\233\256/Solution2.swift"
@@ -0,0 +1,13 @@
+class Solution {
+ func uniquePaths(_ m: Int, _ n: Int) -> Int {
+ var dp = Array(repeating: Array(repeating: 1, count: n), count: m)
+
+ for i in 1.. Int {
+ let m = grid.count
+ let n = grid[0].count
+ var dp = grid
+
+ for i in 1..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 099. \346\234\200\345\260\217\350\267\257\345\276\204\344\271\213\345\222\214/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 099. \346\234\200\345\260\217\350\267\257\345\276\204\344\271\213\345\222\214/Solution.swift"
new file mode 100644
index 0000000000000..34197f086e1a2
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 099. \346\234\200\345\260\217\350\267\257\345\276\204\344\271\213\345\222\214/Solution.swift"
@@ -0,0 +1,23 @@
+class Solution {
+ func minPathSum(_ grid: [[Int]]) -> Int {
+ let m = grid.count
+ let n = grid[0].count
+ var dp = grid
+
+ for i in 1.. Int {
+ let n = triangle.count
+ var dp = Array(repeating: 0, count: n + 1)
+
+ for i in (0..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 100. \344\270\211\350\247\222\345\275\242\344\270\255\346\234\200\345\260\217\350\267\257\345\276\204\344\271\213\345\222\214/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 100. \344\270\211\350\247\222\345\275\242\344\270\255\346\234\200\345\260\217\350\267\257\345\276\204\344\271\213\345\222\214/Solution.swift"
new file mode 100644
index 0000000000000..2aa79db08afb9
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 100. \344\270\211\350\247\222\345\275\242\344\270\255\346\234\200\345\260\217\350\267\257\345\276\204\344\271\213\345\222\214/Solution.swift"
@@ -0,0 +1,14 @@
+class Solution {
+ func minimumTotal(_ triangle: [[Int]]) -> Int {
+ let n = triangle.count
+ var dp = Array(repeating: 0, count: n + 1)
+
+ for i in (0.. Bool {
+ let s = nums.reduce(0, +)
+ if s % 2 != 0 { return false }
+ let target = s / 2
+ var dp = Array(repeating: false, count: target + 1)
+ dp[0] = true
+
+ for num in nums {
+ for j in stride(from: target, through: num, by: -1) {
+ dp[j] = dp[j] || dp[j - num]
+ }
+ }
+
+ return dp[target]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 101. \345\210\206\345\211\262\347\255\211\345\222\214\345\255\220\344\270\262/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 101. \345\210\206\345\211\262\347\255\211\345\222\214\345\255\220\344\270\262/Solution.swift"
new file mode 100644
index 0000000000000..b6eda6e15b417
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 101. \345\210\206\345\211\262\347\255\211\345\222\214\345\255\220\344\270\262/Solution.swift"
@@ -0,0 +1,17 @@
+class Solution {
+ func canPartition(_ nums: [Int]) -> Bool {
+ let s = nums.reduce(0, +)
+ if s % 2 != 0 { return false }
+ let target = s / 2
+ var dp = Array(repeating: false, count: target + 1)
+ dp[0] = true
+
+ for num in nums {
+ for j in stride(from: target, through: num, by: -1) {
+ dp[j] = dp[j] || dp[j - num]
+ }
+ }
+
+ return dp[target]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/README.md"
index 8c6e72e679a66..c9fd89e167210 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/README.md"
@@ -163,6 +163,35 @@ func findTargetSumWays(nums []int, target int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func findTargetSumWays(_ nums: [Int], _ target: Int) -> Int {
+ if target < -1000 || target > 1000 {
+ return 0
+ }
+
+ let n = nums.count
+ var dp = Array(repeating: Array(repeating: 0, count: 2001), count: n)
+
+ dp[0][nums[0] + 1000] += 1
+ dp[0][-nums[0] + 1000] += 1
+
+ for i in 1.. 0 {
+ dp[i][j + nums[i] + 1000] += dp[i - 1][j + 1000]
+ dp[i][j - nums[i] + 1000] += dp[i - 1][j + 1000]
+ }
+ }
+ }
+
+ return dp[n - 1][target + 1000]
+ }
+}
+```
+
@@ -241,6 +270,29 @@ func findTargetSumWays(nums []int, target int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func findTargetSumWays(_ nums: [Int], _ target: Int) -> Int {
+ let s = nums.reduce(0, +)
+ if s - target < 0 || (s - target) % 2 != 0 {
+ return 0
+ }
+ let target = (s - target) / 2
+ var dp = [Int](repeating: 0, count: target + 1)
+ dp[0] = 1
+
+ for num in nums {
+ for j in stride(from: target, through: num, by: -1) {
+ dp[j] += dp[j - num]
+ }
+ }
+ return dp[target]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/Solution.swift"
new file mode 100644
index 0000000000000..d6e91b605e4a9
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/Solution.swift"
@@ -0,0 +1,24 @@
+class Solution {
+ func findTargetSumWays(_ nums: [Int], _ target: Int) -> Int {
+ if target < -1000 || target > 1000 {
+ return 0
+ }
+
+ let n = nums.count
+ var dp = Array(repeating: Array(repeating: 0, count: 2001), count: n)
+
+ dp[0][nums[0] + 1000] += 1
+ dp[0][-nums[0] + 1000] += 1
+
+ for i in 1.. 0 {
+ dp[i][j + nums[i] + 1000] += dp[i - 1][j + 1000]
+ dp[i][j - nums[i] + 1000] += dp[i - 1][j + 1000]
+ }
+ }
+ }
+
+ return dp[n - 1][target + 1000]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/Solution2.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/Solution2.swift"
new file mode 100644
index 0000000000000..399bae61ffbb6
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 102. \345\212\240\345\207\217\347\232\204\347\233\256\346\240\207\345\200\274/Solution2.swift"
@@ -0,0 +1,18 @@
+class Solution {
+ func findTargetSumWays(_ nums: [Int], _ target: Int) -> Int {
+ let s = nums.reduce(0, +)
+ if s - target < 0 || (s - target) % 2 != 0 {
+ return 0
+ }
+ let target = (s - target) / 2
+ var dp = [Int](repeating: 0, count: target + 1)
+ dp[0] = 1
+
+ for num in nums {
+ for j in stride(from: target, through: num, by: -1) {
+ dp[j] += dp[j - num]
+ }
+ }
+ return dp[target]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 103. \346\234\200\345\260\221\347\232\204\347\241\254\345\270\201\346\225\260\347\233\256/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 103. \346\234\200\345\260\221\347\232\204\347\241\254\345\270\201\346\225\260\347\233\256/README.md"
index 96d02c1d78299..c7f06ff5fb2b5 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 103. \346\234\200\345\260\221\347\232\204\347\241\254\345\270\201\346\225\260\347\233\256/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 103. \346\234\200\345\260\221\347\232\204\347\241\254\345\270\201\346\225\260\347\233\256/README.md"
@@ -169,6 +169,26 @@ var coinChange = function (coins, amount) {
};
```
+#### Swift
+
+```swift
+class Solution {
+ func coinChange(_ coins: [Int], _ amount: Int) -> Int {
+ var dp = [Int](repeating: amount + 1, count: amount + 1)
+ dp[0] = 0
+
+ for coin in coins {
+ if coin > amount { continue }
+ for j in coin...amount {
+ dp[j] = min(dp[j], dp[j - coin] + 1)
+ }
+ }
+
+ return dp[amount] > amount ? -1 : dp[amount]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 103. \346\234\200\345\260\221\347\232\204\347\241\254\345\270\201\346\225\260\347\233\256/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 103. \346\234\200\345\260\221\347\232\204\347\241\254\345\270\201\346\225\260\347\233\256/Solution.swift"
new file mode 100644
index 0000000000000..2a8a532c972c1
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 103. \346\234\200\345\260\221\347\232\204\347\241\254\345\270\201\346\225\260\347\233\256/Solution.swift"
@@ -0,0 +1,15 @@
+class Solution {
+ func coinChange(_ coins: [Int], _ amount: Int) -> Int {
+ var dp = [Int](repeating: amount + 1, count: amount + 1)
+ dp[0] = 0
+
+ for coin in coins {
+ if coin > amount { continue }
+ for j in coin...amount {
+ dp[j] = min(dp[j], dp[j - coin] + 1)
+ }
+ }
+
+ return dp[amount] > amount ? -1 : dp[amount]
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 104. \346\216\222\345\210\227\347\232\204\346\225\260\347\233\256/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 104. \346\216\222\345\210\227\347\232\204\346\225\260\347\233\256/README.md"
index 5bae4765fa3e0..632d3565e7961 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 104. \346\216\222\345\210\227\347\232\204\346\225\260\347\233\256/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 104. \346\216\222\345\210\227\347\232\204\346\225\260\347\233\256/README.md"
@@ -141,6 +141,27 @@ func combinationSum4(nums []int, target int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func combinationSum4(_ nums: [Int], _ target: Int) -> Int {
+ var dp = [Int](repeating: 0, count: target + 1)
+ dp[0] = 1
+
+ for i in 1...target {
+ for num in nums {
+ if i >= num, dp[i] <= Int.max - dp[i - num] {
+ dp[i] += dp[i - num]
+ }
+ }
+ }
+
+ return dp[target]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 104. \346\216\222\345\210\227\347\232\204\346\225\260\347\233\256/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 104. \346\216\222\345\210\227\347\232\204\346\225\260\347\233\256/Solution.swift"
new file mode 100644
index 0000000000000..b6629dc05aa27
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 104. \346\216\222\345\210\227\347\232\204\346\225\260\347\233\256/Solution.swift"
@@ -0,0 +1,16 @@
+class Solution {
+ func combinationSum4(_ nums: [Int], _ target: Int) -> Int {
+ var dp = [Int](repeating: 0, count: target + 1)
+ dp[0] = 1
+
+ for i in 1...target {
+ for num in nums {
+ if i >= num, dp[i] <= Int.max - dp[i - num] {
+ dp[i] += dp[i - num]
+ }
+ }
+ }
+
+ return dp[target]
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 105. \345\262\233\345\261\277\347\232\204\346\234\200\345\244\247\351\235\242\347\247\257/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 105. \345\262\233\345\261\277\347\232\204\346\234\200\345\244\247\351\235\242\347\247\257/README.md"
index fec092604a769..cc606dc3f2369 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 105. \345\262\233\345\261\277\347\232\204\346\234\200\345\244\247\351\235\242\347\247\257/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 105. \345\262\233\345\261\277\347\232\204\346\234\200\345\244\247\351\235\242\347\247\257/README.md"
@@ -250,6 +250,50 @@ impl Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var m = 0
+ private var n = 0
+ private var grid: [[Int]] = []
+
+ func maxAreaOfIsland(_ grid: [[Int]]) -> Int {
+ self.m = grid.count
+ self.n = grid[0].count
+ self.grid = grid
+ var maxArea = 0
+
+ for i in 0.. Int {
+ if grid[i][j] == 0 {
+ return 0
+ }
+
+ var area = 1
+ grid[i][j] = 0
+ let dirs = [-1, 0, 1, 0, -1]
+
+ for k in 0..<4 {
+ let x = i + dirs[k], y = j + dirs[k + 1]
+ if x >= 0 && x < m && y >= 0 && y < n {
+ area += dfs(x, y)
+ }
+ }
+
+ return area
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 105. \345\262\233\345\261\277\347\232\204\346\234\200\345\244\247\351\235\242\347\247\257/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 105. \345\262\233\345\261\277\347\232\204\346\234\200\345\244\247\351\235\242\347\247\257/Solution.swift"
new file mode 100644
index 0000000000000..da64e873a66c1
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 105. \345\262\233\345\261\277\347\232\204\346\234\200\345\244\247\351\235\242\347\247\257/Solution.swift"
@@ -0,0 +1,39 @@
+class Solution {
+ private var m = 0
+ private var n = 0
+ private var grid: [[Int]] = []
+
+ func maxAreaOfIsland(_ grid: [[Int]]) -> Int {
+ self.m = grid.count
+ self.n = grid[0].count
+ self.grid = grid
+ var maxArea = 0
+
+ for i in 0.. Int {
+ if grid[i][j] == 0 {
+ return 0
+ }
+
+ var area = 1
+ grid[i][j] = 0
+ let dirs = [-1, 0, 1, 0, -1]
+
+ for k in 0..<4 {
+ let x = i + dirs[k], y = j + dirs[k + 1]
+ if x >= 0 && x < m && y >= 0 && y < n {
+ area += dfs(x, y)
+ }
+ }
+
+ return area
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 106. \344\272\214\345\210\206\345\233\276/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 106. \344\272\214\345\210\206\345\233\276/README.md"
index 710a738b34294..b0afc43b343c1 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 106. \344\272\214\345\210\206\345\233\276/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 106. \344\272\214\345\210\206\345\233\276/README.md"
@@ -183,6 +183,36 @@ func isBipartite(graph [][]int) bool {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var parent: [Int] = []
+
+ func isBipartite(_ graph: [[Int]]) -> Bool {
+ let n = graph.count
+ parent = Array(0.. Int {
+ if parent[x] != x {
+ parent[x] = find(parent[x])
+ }
+ return parent[x]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 106. \344\272\214\345\210\206\345\233\276/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 106. \344\272\214\345\210\206\345\233\276/Solution.swift"
new file mode 100644
index 0000000000000..9ebd6dd7071b7
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 106. \344\272\214\345\210\206\345\233\276/Solution.swift"
@@ -0,0 +1,25 @@
+class Solution {
+ private var parent: [Int] = []
+
+ func isBipartite(_ graph: [[Int]]) -> Bool {
+ let n = graph.count
+ parent = Array(0.. Int {
+ if parent[x] != x {
+ parent[x] = find(parent[x])
+ }
+ return parent[x]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 107. \347\237\251\351\230\265\344\270\255\347\232\204\350\267\235\347\246\273/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 107. \347\237\251\351\230\265\344\270\255\347\232\204\350\267\235\347\246\273/README.md"
index 7a1840e9e959b..d54780acac9b6 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 107. \347\237\251\351\230\265\344\270\255\347\232\204\350\267\235\347\246\273/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 107. \347\237\251\351\230\265\344\270\255\347\232\204\350\267\235\347\246\273/README.md"
@@ -200,6 +200,44 @@ func updateMatrix(mat [][]int) [][]int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func updateMatrix(_ mat: [[Int]]) -> [[Int]] {
+ let m = mat.count
+ let n = mat[0].count
+ var ans = Array(repeating: Array(repeating: -1, count: n), count: m)
+ var queue = [(Int, Int)]()
+
+ for i in 0..= 0 && x < m && y >= 0 && y < n && ans[x][y] == -1 {
+ ans[x][y] = ans[i][j] + 1
+ queue.append((x, y))
+ }
+ }
+ }
+
+ return ans
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 107. \347\237\251\351\230\265\344\270\255\347\232\204\350\267\235\347\246\273/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 107. \347\237\251\351\230\265\344\270\255\347\232\204\350\267\235\347\246\273/Solution.swift"
new file mode 100644
index 0000000000000..be98341a4a6cf
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 107. \347\237\251\351\230\265\344\270\255\347\232\204\350\267\235\347\246\273/Solution.swift"
@@ -0,0 +1,33 @@
+class Solution {
+ func updateMatrix(_ mat: [[Int]]) -> [[Int]] {
+ let m = mat.count
+ let n = mat[0].count
+ var ans = Array(repeating: Array(repeating: -1, count: n), count: m)
+ var queue = [(Int, Int)]()
+
+ for i in 0..= 0 && x < m && y >= 0 && y < n && ans[x][y] == -1 {
+ ans[x][y] = ans[i][j] + 1
+ queue.append((x, y))
+ }
+ }
+ }
+
+ return ans
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 108. \345\215\225\350\257\215\346\274\224\345\217\230/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 108. \345\215\225\350\257\215\346\274\224\345\217\230/README.md"
index 12309e85a5a3a..cfc23846b8909 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 108. \345\215\225\350\257\215\346\274\224\345\217\230/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 108. \345\215\225\350\257\215\346\274\224\345\217\230/README.md"
@@ -204,6 +204,43 @@ func ladderLength(beginWord string, endWord string, wordList []string) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func ladderLength(_ beginWord: String, _ endWord: String, _ wordList: [String]) -> Int {
+ var words = Set(wordList)
+ var queue = [beginWord]
+ var ans = 1
+
+ while !queue.isEmpty {
+ for _ in 0..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 108. \345\215\225\350\257\215\346\274\224\345\217\230/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 108. \345\215\225\350\257\215\346\274\224\345\217\230/Solution.swift"
new file mode 100644
index 0000000000000..3d1231ac00c9e
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 108. \345\215\225\350\257\215\346\274\224\345\217\230/Solution.swift"
@@ -0,0 +1,32 @@
+class Solution {
+ func ladderLength(_ beginWord: String, _ endWord: String, _ wordList: [String]) -> Int {
+ var words = Set(wordList)
+ var queue = [beginWord]
+ var ans = 1
+
+ while !queue.isEmpty {
+ for _ in 0.. 0 {
+ step++
+ size := len(q)
+ for i := 0; i < size; i++ {
+ cur := q[0]
+ q = q[1:]
+ for j := 0; j < 4; j++ {
+ for k := -1; k <= 1; k += 2 {
+ next := cur[:j] + string((cur[j]-'0'+byte(k)+10)%10+'0') + cur[j+1:]
+ if next == target {
+ return step
+ }
+ if !dead[next] && !visited[next] {
+ q = append(q, next)
+ visited[next] = true
+ }
+ }
+ }
+ }
+ }
+ return -1
+}
+```
+
+#### Swift
+
+```swift
+class Solution {
+ func openLock(_ deadends: [String], _ target: String) -> Int {
+ let deadSet = Set(deadends)
+ if deadSet.contains(target) || deadSet.contains("0000") {
+ return -1
+ }
+ if target == "0000" {
+ return 0
+ }
+
+ var visited = Set()
+ var queue = ["0000"]
+ visited.insert("0000")
+ var step = 0
+
+ while !queue.isEmpty {
+ step += 1
+ for _ in 0.. [String] {
+ var neighbors = [String]()
+ var chars = Array(lock)
+ for i in 0..<4 {
+ let original = chars[i]
+ chars[i] = prevChar(original)
+ neighbors.append(String(chars))
+ chars[i] = nextChar(original)
+ neighbors.append(String(chars))
+ chars[i] = original
+ }
+ return neighbors
+ }
+
+ private func prevChar(_ c: Character) -> Character {
+ return c == "0" ? "9" : Character(UnicodeScalar(c.asciiValue! - 1))
+ }
+
+ private func nextChar(_ c: Character) -> Character {
+ return c == "9" ? "0" : Character(UnicodeScalar(c.asciiValue! + 1))
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 109. \345\274\200\345\257\206\347\240\201\351\224\201/Solution.go" "b/lcof2/\345\211\221\346\214\207 Offer II 109. \345\274\200\345\257\206\347\240\201\351\224\201/Solution.go"
new file mode 100644
index 0000000000000..e150cc42225e8
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 109. \345\274\200\345\257\206\347\240\201\351\224\201/Solution.go"
@@ -0,0 +1,36 @@
+func openLock(deadends []string, target string) int {
+ dead := map[string]bool{}
+ for _, s := range deadends {
+ dead[s] = true
+ }
+ if dead["0000"] {
+ return -1
+ }
+ if target == "0000" {
+ return 0
+ }
+ q := []string{"0000"}
+ visited := map[string]bool{"0000": true}
+ step := 0
+ for len(q) > 0 {
+ step++
+ size := len(q)
+ for i := 0; i < size; i++ {
+ cur := q[0]
+ q = q[1:]
+ for j := 0; j < 4; j++ {
+ for k := -1; k <= 1; k += 2 {
+ next := cur[:j] + string((cur[j]-'0'+byte(k)+10)%10+'0') + cur[j+1:]
+ if next == target {
+ return step
+ }
+ if !dead[next] && !visited[next] {
+ q = append(q, next)
+ visited[next] = true
+ }
+ }
+ }
+ }
+ }
+ return -1
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 109. \345\274\200\345\257\206\347\240\201\351\224\201/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 109. \345\274\200\345\257\206\347\240\201\351\224\201/Solution.swift"
new file mode 100644
index 0000000000000..f50451503b7f7
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 109. \345\274\200\345\257\206\347\240\201\351\224\201/Solution.swift"
@@ -0,0 +1,57 @@
+class Solution {
+ func openLock(_ deadends: [String], _ target: String) -> Int {
+ let deadSet = Set(deadends)
+ if deadSet.contains(target) || deadSet.contains("0000") {
+ return -1
+ }
+ if target == "0000" {
+ return 0
+ }
+
+ var visited = Set()
+ var queue = ["0000"]
+ visited.insert("0000")
+ var step = 0
+
+ while !queue.isEmpty {
+ step += 1
+ for _ in 0.. [String] {
+ var neighbors = [String]()
+ var chars = Array(lock)
+ for i in 0..<4 {
+ let original = chars[i]
+ chars[i] = prevChar(original)
+ neighbors.append(String(chars))
+ chars[i] = nextChar(original)
+ neighbors.append(String(chars))
+ chars[i] = original
+ }
+ return neighbors
+ }
+
+ private func prevChar(_ c: Character) -> Character {
+ return c == "0" ? "9" : Character(UnicodeScalar(c.asciiValue! - 1))
+ }
+
+ private func nextChar(_ c: Character) -> Character {
+ return c == "9" ? "0" : Character(UnicodeScalar(c.asciiValue! + 1))
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 110. \346\211\200\346\234\211\350\267\257\345\276\204/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 110. \346\211\200\346\234\211\350\267\257\345\276\204/README.md"
index d836612be9356..cf5ad50bd1cd5 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 110. \346\211\200\346\234\211\350\267\257\345\276\204/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 110. \346\211\200\346\234\211\350\267\257\345\276\204/README.md"
@@ -189,6 +189,35 @@ func allPathsSourceTarget(graph [][]int) [][]int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var results = [[Int]]()
+ private var graph = [[Int]]()
+
+ func allPathsSourceTarget(_ graph: [[Int]]) -> [[Int]] {
+ self.graph = graph
+ var path = [0]
+ dfs(0, &path)
+ return results
+ }
+
+ private func dfs(_ node: Int, _ path: inout [Int]) {
+ if node == graph.count - 1 {
+ results.append(Array(path))
+ return
+ }
+
+ for next in graph[node] {
+ path.append(next)
+ dfs(next, &path)
+ path.removeLast()
+ }
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 110. \346\211\200\346\234\211\350\267\257\345\276\204/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 110. \346\211\200\346\234\211\350\267\257\345\276\204/Solution.swift"
new file mode 100644
index 0000000000000..687ea6eb06818
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 110. \346\211\200\346\234\211\350\267\257\345\276\204/Solution.swift"
@@ -0,0 +1,24 @@
+class Solution {
+ private var results = [[Int]]()
+ private var graph = [[Int]]()
+
+ func allPathsSourceTarget(_ graph: [[Int]]) -> [[Int]] {
+ self.graph = graph
+ var path = [0]
+ dfs(0, &path)
+ return results
+ }
+
+ private func dfs(_ node: Int, _ path: inout [Int]) {
+ if node == graph.count - 1 {
+ results.append(Array(path))
+ return
+ }
+
+ for next in graph[node] {
+ path.append(next)
+ dfs(next, &path)
+ path.removeLast()
+ }
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 111. \350\256\241\347\256\227\351\231\244\346\263\225/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 111. \350\256\241\347\256\227\351\231\244\346\263\225/README.md"
index 50e3b501799b6..bee586df396e5 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 111. \350\256\241\347\256\227\351\231\244\346\263\225/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 111. \350\256\241\347\256\227\351\231\244\346\263\225/README.md"
@@ -292,6 +292,72 @@ func find(x int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var parent = [Int]()
+ private var weight = [Double]()
+
+ func calcEquation(
+ _ equations: [[String]],
+ _ values: [Double],
+ _ queries: [[String]]
+ ) -> [Double] {
+ let n = equations.count
+ parent = Array(0..<(n * 2))
+ weight = Array(repeating: 1.0, count: n * 2)
+
+ var map = [String: Int]()
+ var index = 0
+
+ for i in 0.. Int {
+ if parent[x] != x {
+ let origin = parent[x]
+ parent[x] = find(parent[x])
+ weight[x] *= weight[origin]
+ }
+ return parent[x]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 111. \350\256\241\347\256\227\351\231\244\346\263\225/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 111. \350\256\241\347\256\227\351\231\244\346\263\225/Solution.swift"
new file mode 100644
index 0000000000000..084ab48d48fd5
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 111. \350\256\241\347\256\227\351\231\244\346\263\225/Solution.swift"
@@ -0,0 +1,61 @@
+class Solution {
+ private var parent = [Int]()
+ private var weight = [Double]()
+
+ func calcEquation(
+ _ equations: [[String]],
+ _ values: [Double],
+ _ queries: [[String]]
+ ) -> [Double] {
+ let n = equations.count
+ parent = Array(0..<(n * 2))
+ weight = Array(repeating: 1.0, count: n * 2)
+
+ var map = [String: Int]()
+ var index = 0
+
+ for i in 0.. Int {
+ if parent[x] != x {
+ let origin = parent[x]
+ parent[x] = find(parent[x])
+ weight[x] *= weight[origin]
+ }
+ return parent[x]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 112. \346\234\200\351\225\277\351\200\222\345\242\236\350\267\257\345\276\204/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 112. \346\234\200\351\225\277\351\200\222\345\242\236\350\267\257\345\276\204/README.md"
index 4e8337208369c..f878e8e0288b2 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 112. \346\234\200\351\225\277\351\200\222\345\242\236\350\267\257\345\276\204/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 112. \346\234\200\351\225\277\351\200\222\345\242\236\350\267\257\345\276\204/README.md"
@@ -205,6 +205,49 @@ func longestIncreasingPath(matrix [][]int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var memo: [[Int]] = []
+ private var matrix: [[Int]] = []
+ private var m: Int = 0
+ private var n: Int = 0
+
+ func longestIncreasingPath(_ matrix: [[Int]]) -> Int {
+ self.matrix = matrix
+ m = matrix.count
+ n = matrix[0].count
+ memo = Array(repeating: Array(repeating: -1, count: n), count: m)
+
+ var ans = 0
+ for i in 0.. Int {
+ if memo[i][j] != -1 {
+ return memo[i][j]
+ }
+ var ans = 1
+ let dirs = [(-1, 0), (1, 0), (0, -1), (0, 1)]
+
+ for (dx, dy) in dirs {
+ let x = i + dx, y = j + dy
+ if x >= 0, x < m, y >= 0, y < n, matrix[x][y] > matrix[i][j] {
+ ans = max(ans, dfs(x, y) + 1)
+ }
+ }
+ memo[i][j] = ans
+ return ans
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 112. \346\234\200\351\225\277\351\200\222\345\242\236\350\267\257\345\276\204/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 112. \346\234\200\351\225\277\351\200\222\345\242\236\350\267\257\345\276\204/Solution.swift"
new file mode 100644
index 0000000000000..a1bca0d1e6a02
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 112. \346\234\200\351\225\277\351\200\222\345\242\236\350\267\257\345\276\204/Solution.swift"
@@ -0,0 +1,38 @@
+class Solution {
+ private var memo: [[Int]] = []
+ private var matrix: [[Int]] = []
+ private var m: Int = 0
+ private var n: Int = 0
+
+ func longestIncreasingPath(_ matrix: [[Int]]) -> Int {
+ self.matrix = matrix
+ m = matrix.count
+ n = matrix[0].count
+ memo = Array(repeating: Array(repeating: -1, count: n), count: m)
+
+ var ans = 0
+ for i in 0.. Int {
+ if memo[i][j] != -1 {
+ return memo[i][j]
+ }
+ var ans = 1
+ let dirs = [(-1, 0), (1, 0), (0, -1), (0, 1)]
+
+ for (dx, dy) in dirs {
+ let x = i + dx, y = j + dy
+ if x >= 0, x < m, y >= 0, y < n, matrix[x][y] > matrix[i][j] {
+ ans = max(ans, dfs(x, y) + 1)
+ }
+ }
+ memo[i][j] = ans
+ return ans
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 113. \350\257\276\347\250\213\351\241\272\345\272\217/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 113. \350\257\276\347\250\213\351\241\272\345\272\217/README.md"
index 52e72ae62a670..8fc55cbe257d2 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 113. \350\257\276\347\250\213\351\241\272\345\272\217/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 113. \350\257\276\347\250\213\351\241\272\345\272\217/README.md"
@@ -269,6 +269,46 @@ public class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func findOrder(_ numCourses: Int, _ prerequisites: [[Int]]) -> [Int] {
+ var graph = Array(repeating: [Int](), count: numCourses)
+ var indegree = Array(repeating: 0, count: numCourses)
+
+ for prereq in prerequisites {
+ let course = prereq[0]
+ let prereqCourse = prereq[1]
+ graph[prereqCourse].append(course)
+ indegree[course] += 1
+ }
+
+ var queue = [Int]()
+ for i in 0..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 113. \350\257\276\347\250\213\351\241\272\345\272\217/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 113. \350\257\276\347\250\213\351\241\272\345\272\217/Solution.swift"
new file mode 100644
index 0000000000000..3bb5be6ec4b4d
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 113. \350\257\276\347\250\213\351\241\272\345\272\217/Solution.swift"
@@ -0,0 +1,35 @@
+class Solution {
+ func findOrder(_ numCourses: Int, _ prerequisites: [[Int]]) -> [Int] {
+ var graph = Array(repeating: [Int](), count: numCourses)
+ var indegree = Array(repeating: 0, count: numCourses)
+
+ for prereq in prerequisites {
+ let course = prereq[0]
+ let prereqCourse = prereq[1]
+ graph[prereqCourse].append(course)
+ indegree[course] += 1
+ }
+
+ var queue = [Int]()
+ for i in 0.. len(w2) {
+ return ""
+ }
+ }
+ queue := make([]byte, 0)
+ for k := range inDegree {
+ if inDegree[k] == 0 {
+ queue = append(queue, k)
+ }
+ }
+ res := make([]byte, 0)
+ for len(queue) > 0 {
+ node := queue[0]
+ queue = queue[1:]
+ res = append(res, node)
+ for _, next := range graph[node] {
+ inDegree[next]--
+ if inDegree[next] == 0 {
+ queue = append(queue, next)
+ }
+ }
+ }
+ if len(res) != len(inDegree) {
+ return ""
+ }
+ return string(res)
+}
+```
+
+#### Swift
+
+```swift
+class Solution {
+ func alienOrder(_ words: [String]) -> String {
+ var graph = Array(repeating: Set(), count: 26)
+ var indegree = Array(repeating: 0, count: 26)
+ var seen = Array(repeating: false, count: 26)
+ var letterCount = 0
+
+ for i in 0.. words[i + 1].count {
+ return ""
+ }
+ }
+ }
+
+ for char in words[words.count - 1] {
+ let index = Int(char.asciiValue! - Character("a").asciiValue!)
+ if !seen[index] {
+ seen[index] = true
+ letterCount += 1
+ }
+ }
+
+ var queue = [Int]()
+ for i in 0..<26 {
+ if seen[i] && indegree[i] == 0 {
+ queue.append(i)
+ }
+ }
+
+ var order = ""
+ while !queue.isEmpty {
+ let u = queue.removeFirst()
+ order += String(UnicodeScalar(u + Int(Character("a").asciiValue!))!)
+ for v in graph[u] {
+ indegree[v] -= 1
+ if indegree[v] == 0 {
+ queue.append(v)
+ }
+ }
+ }
+
+ return order.count == letterCount ? order : ""
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 114. \345\244\226\346\230\237\346\226\207\345\255\227\345\205\270/Solution.go" "b/lcof2/\345\211\221\346\214\207 Offer II 114. \345\244\226\346\230\237\346\226\207\345\255\227\345\205\270/Solution.go"
new file mode 100644
index 0000000000000..94dad68e38e6a
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 114. \345\244\226\346\230\237\346\226\207\345\255\227\345\205\270/Solution.go"
@@ -0,0 +1,57 @@
+func alienOrder(words []string) string {
+ n := len(words)
+ if n == 0 {
+ return ""
+ }
+ if n == 1 {
+ return words[0]
+ }
+ inDegree := make(map[byte]int)
+ graph := make(map[byte][]byte)
+ for _, word := range words {
+ for i := 0; i < len(word); i++ {
+ inDegree[word[i]] = 0
+ }
+ }
+ for i := 0; i < n-1; i++ {
+ w1, w2 := words[i], words[i+1]
+ minLen := len(w1)
+ if len(w2) < minLen {
+ minLen = len(w2)
+ }
+ foundDifference := false
+ for j := 0; j < minLen; j++ {
+ if w1[j] != w2[j] {
+ inDegree[w2[j]]++
+ graph[w1[j]] = append(graph[w1[j]], w2[j])
+ foundDifference = true
+ break
+ }
+ }
+ if !foundDifference && len(w1) > len(w2) {
+ return ""
+ }
+ }
+ queue := make([]byte, 0)
+ for k := range inDegree {
+ if inDegree[k] == 0 {
+ queue = append(queue, k)
+ }
+ }
+ res := make([]byte, 0)
+ for len(queue) > 0 {
+ node := queue[0]
+ queue = queue[1:]
+ res = append(res, node)
+ for _, next := range graph[node] {
+ inDegree[next]--
+ if inDegree[next] == 0 {
+ queue = append(queue, next)
+ }
+ }
+ }
+ if len(res) != len(inDegree) {
+ return ""
+ }
+ return string(res)
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 114. \345\244\226\346\230\237\346\226\207\345\255\227\345\205\270/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 114. \345\244\226\346\230\237\346\226\207\345\255\227\345\205\270/Solution.swift"
new file mode 100644
index 0000000000000..8f6ed449fed7f
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 114. \345\244\226\346\230\237\346\226\207\345\255\227\345\205\270/Solution.swift"
@@ -0,0 +1,67 @@
+class Solution {
+ func alienOrder(_ words: [String]) -> String {
+ var graph = Array(repeating: Set(), count: 26)
+ var indegree = Array(repeating: 0, count: 26)
+ var seen = Array(repeating: false, count: 26)
+ var letterCount = 0
+
+ for i in 0.. words[i + 1].count {
+ return ""
+ }
+ }
+ }
+
+ for char in words[words.count - 1] {
+ let index = Int(char.asciiValue! - Character("a").asciiValue!)
+ if !seen[index] {
+ seen[index] = true
+ letterCount += 1
+ }
+ }
+
+ var queue = [Int]()
+ for i in 0..<26 {
+ if seen[i] && indegree[i] == 0 {
+ queue.append(i)
+ }
+ }
+
+ var order = ""
+ while !queue.isEmpty {
+ let u = queue.removeFirst()
+ order += String(UnicodeScalar(u + Int(Character("a").asciiValue!))!)
+ for v in graph[u] {
+ indegree[v] -= 1
+ if indegree[v] == 0 {
+ queue.append(v)
+ }
+ }
+ }
+
+ return order.count == letterCount ? order : ""
+ }
+}
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 115. \351\207\215\345\273\272\345\272\217\345\210\227/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 115. \351\207\215\345\273\272\345\272\217\345\210\227/README.md"
index 3f1b34527a0af..2b1f18bc59a6a 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 115. \351\207\215\345\273\272\345\272\217\345\210\227/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 115. \351\207\215\345\273\272\345\272\217\345\210\227/README.md"
@@ -250,6 +250,46 @@ function sequenceReconstruction(nums: number[], sequences: number[][]): boolean
}
```
+#### Swift
+
+```swift
+class Solution {
+ func sequenceReconstruction(_ nums: [Int], _ sequences: [[Int]]) -> Bool {
+ let n = nums.count
+ var indegree = [Int](repeating: 0, count: n)
+ var graph = Array(repeating: [Int](), count: n)
+
+ for sequence in sequences {
+ for i in 1..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 115. \351\207\215\345\273\272\345\272\217\345\210\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 115. \351\207\215\345\273\272\345\272\217\345\210\227/Solution.swift"
new file mode 100644
index 0000000000000..16fc600079abb
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 115. \351\207\215\345\273\272\345\272\217\345\210\227/Solution.swift"
@@ -0,0 +1,35 @@
+class Solution {
+ func sequenceReconstruction(_ nums: [Int], _ sequences: [[Int]]) -> Bool {
+ let n = nums.count
+ var indegree = [Int](repeating: 0, count: n)
+ var graph = Array(repeating: [Int](), count: n)
+
+ for sequence in sequences {
+ for i in 1.. Int {
+ self.isConnected = isConnected
+ self.n = isConnected.count
+ self.visited = [Bool](repeating: false, count: n)
+ var numberOfCircles = 0
+
+ for i in 0..
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 116. \346\234\213\345\217\213\345\234\210/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 116. \346\234\213\345\217\213\345\234\210/Solution.swift"
new file mode 100644
index 0000000000000..b1e70c6aec534
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 116. \346\234\213\345\217\213\345\234\210/Solution.swift"
@@ -0,0 +1,29 @@
+class Solution {
+ private var isConnected: [[Int]] = []
+ private var visited: [Bool] = []
+ private var n: Int = 0
+
+ func findCircleNum(_ isConnected: [[Int]]) -> Int {
+ self.isConnected = isConnected
+ self.n = isConnected.count
+ self.visited = [Bool](repeating: false, count: n)
+ var numberOfCircles = 0
+
+ for i in 0.. Int {
+ let n = strs.count
+ parent = Array(0.. Bool {
+ let n = a.count
+ var count = 0
+ let arrA = Array(a), arrB = Array(b)
+
+ for i in 0.. 2 {
+ return false
+ }
+ }
+ return count <= 2
+ }
+
+ private func find(_ x: Int) -> Int {
+ if parent[x] != x {
+ parent[x] = find(parent[x])
+ }
+ return parent[x]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 117. \347\233\270\344\274\274\347\232\204\345\255\227\347\254\246\344\270\262/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 117. \347\233\270\344\274\274\347\232\204\345\255\227\347\254\246\344\270\262/Solution.swift"
new file mode 100644
index 0000000000000..ac1f651a34e6b
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 117. \347\233\270\344\274\274\347\232\204\345\255\227\347\254\246\344\270\262/Solution.swift"
@@ -0,0 +1,47 @@
+class Solution {
+ private var parent: [Int] = []
+
+ func numSimilarGroups(_ strs: [String]) -> Int {
+ let n = strs.count
+ parent = Array(0.. Bool {
+ let n = a.count
+ var count = 0
+ let arrA = Array(a), arrB = Array(b)
+
+ for i in 0.. 2 {
+ return false
+ }
+ }
+ return count <= 2
+ }
+
+ private func find(_ x: Int) -> Int {
+ if parent[x] != x {
+ parent[x] = find(parent[x])
+ }
+ return parent[x]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 118. \345\244\232\344\275\231\347\232\204\350\276\271/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 118. \345\244\232\344\275\231\347\232\204\350\276\271/README.md"
index b9dda603f6065..25fa6655da072 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 118. \345\244\232\344\275\231\347\232\204\350\276\271/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 118. \345\244\232\344\275\231\347\232\204\350\276\271/README.md"
@@ -164,6 +164,36 @@ func findRedundantConnection(edges [][]int) []int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ private var parent: [Int] = []
+
+ func findRedundantConnection(_ edges: [[Int]]) -> [Int] {
+ parent = Array(0..<1010)
+
+ for edge in edges {
+ let a = edge[0]
+ let b = edge[1]
+
+ if find(a) == find(b) {
+ return edge
+ }
+ parent[find(a)] = find(b)
+ }
+ return []
+ }
+
+ private func find(_ x: Int) -> Int {
+ if parent[x] != x {
+ parent[x] = find(parent[x])
+ }
+ return parent[x]
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 118. \345\244\232\344\275\231\347\232\204\350\276\271/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 118. \345\244\232\344\275\231\347\232\204\350\276\271/Solution.swift"
new file mode 100644
index 0000000000000..5987e53a2b151
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 118. \345\244\232\344\275\231\347\232\204\350\276\271/Solution.swift"
@@ -0,0 +1,25 @@
+class Solution {
+ private var parent: [Int] = []
+
+ func findRedundantConnection(_ edges: [[Int]]) -> [Int] {
+ parent = Array(0..<1010)
+
+ for edge in edges {
+ let a = edge[0]
+ let b = edge[1]
+
+ if find(a) == find(b) {
+ return edge
+ }
+ parent[find(a)] = find(b)
+ }
+ return []
+ }
+
+ private func find(_ x: Int) -> Int {
+ if parent[x] != x {
+ parent[x] = find(parent[x])
+ }
+ return parent[x]
+ }
+}
\ No newline at end of file
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 119. \346\234\200\351\225\277\350\277\236\347\273\255\345\272\217\345\210\227/README.md" "b/lcof2/\345\211\221\346\214\207 Offer II 119. \346\234\200\351\225\277\350\277\236\347\273\255\345\272\217\345\210\227/README.md"
index c38278c1a11ec..91a2164a9c7f8 100644
--- "a/lcof2/\345\211\221\346\214\207 Offer II 119. \346\234\200\351\225\277\350\277\236\347\273\255\345\272\217\345\210\227/README.md"
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 119. \346\234\200\351\225\277\350\277\236\347\273\255\345\272\217\345\210\227/README.md"
@@ -221,6 +221,34 @@ var longestConsecutive = function (nums) {
};
```
+#### Swift
+
+```swift
+class Solution {
+ func longestConsecutive(_ nums: [Int]) -> Int {
+ let n = nums.count
+ if n < 2 {
+ return n
+ }
+
+ let sortedNums = Array(Set(nums)).sorted()
+ var ans = 1
+ var currentStreak = 1
+
+ for i in 1..
@@ -360,6 +388,33 @@ var longestConsecutive = function (nums) {
};
```
+#### Swift
+
+```swift
+class Solution {
+ func longestConsecutive(_ nums: [Int]) -> Int {
+ let numSet: Set = Set(nums)
+ var longestStreak = 0
+
+ for num in nums {
+ if !numSet.contains(num - 1) {
+ var currentNum = num
+ var currentStreak = 1
+
+ while numSet.contains(currentNum + 1) {
+ currentNum += 1
+ currentStreak += 1
+ }
+
+ longestStreak = max(longestStreak, currentStreak)
+ }
+ }
+
+ return longestStreak
+ }
+}
+```
+
diff --git "a/lcof2/\345\211\221\346\214\207 Offer II 119. \346\234\200\351\225\277\350\277\236\347\273\255\345\272\217\345\210\227/Solution.swift" "b/lcof2/\345\211\221\346\214\207 Offer II 119. \346\234\200\351\225\277\350\277\236\347\273\255\345\272\217\345\210\227/Solution.swift"
new file mode 100644
index 0000000000000..ff42f1c5b1949
--- /dev/null
+++ "b/lcof2/\345\211\221\346\214\207 Offer II 119. \346\234\200\351\225\277\350\277\236\347\273\255\345\272\217\345\210\227/Solution.swift"
@@ -0,0 +1,23 @@
+class Solution {
+ func longestConsecutive(_ nums: [Int]) -> Int {
+ let n = nums.count
+ if n < 2 {
+ return n
+ }
+
+ let sortedNums = Array(Set(nums)).sorted()
+ var ans = 1
+ var currentStreak = 1
+
+ for i in 1.. Int {
+ let numSet: Set = Set(nums)
+ var longestStreak = 0
+
+ for num in nums {
+ if !numSet.contains(num - 1) {
+ var currentNum = num
+ var currentStreak = 1
+
+ while numSet.contains(currentNum + 1) {
+ currentNum += 1
+ currentStreak += 1
+ }
+
+ longestStreak = max(longestStreak, currentStreak)
+ }
+ }
+
+ return longestStreak
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 01. \347\214\234\346\225\260\345\255\227/README.md" "b/lcp/LCP 01. \347\214\234\346\225\260\345\255\227/README.md"
index 27eaab2822b7b..4ddf53357c988 100644
--- "a/lcp/LCP 01. \347\214\234\346\225\260\345\255\227/README.md"
+++ "b/lcp/LCP 01. \347\214\234\346\225\260\345\255\227/README.md"
@@ -155,6 +155,22 @@ int game(int* guess, int guessSize, int* answer, int answerSize) {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func game(_ guess: [Int], _ answer: [Int]) -> Int {
+ var correctGuesses = 0
+ for i in 0..<3 {
+ if guess[i] == answer[i] {
+ correctGuesses += 1
+ }
+ }
+ return correctGuesses
+ }
+}
+```
+
diff --git "a/lcp/LCP 01. \347\214\234\346\225\260\345\255\227/Solution.swift" "b/lcp/LCP 01. \347\214\234\346\225\260\345\255\227/Solution.swift"
new file mode 100644
index 0000000000000..23f55f3b0e31d
--- /dev/null
+++ "b/lcp/LCP 01. \347\214\234\346\225\260\345\255\227/Solution.swift"
@@ -0,0 +1,11 @@
+class Solution {
+ func game(_ guess: [Int], _ answer: [Int]) -> Int {
+ var correctGuesses = 0
+ for i in 0..<3 {
+ if guess[i] == answer[i] {
+ correctGuesses += 1
+ }
+ }
+ return correctGuesses
+ }
+}
diff --git "a/lcp/LCP 02. \345\210\206\345\274\217\345\214\226\347\256\200/README.md" "b/lcp/LCP 02. \345\210\206\345\274\217\345\214\226\347\256\200/README.md"
index f2c5e01372cdd..c819541cadd16 100644
--- "a/lcp/LCP 02. \345\210\206\345\274\217\345\214\226\347\256\200/README.md"
+++ "b/lcp/LCP 02. \345\210\206\345\274\217\345\214\226\347\256\200/README.md"
@@ -195,6 +195,36 @@ var fraction = function (cont) {
};
```
+#### Swift
+
+```swift
+class Solution {
+ private var cont: [Int] = []
+
+ func fraction(_ cont: [Int]) -> [Int] {
+ self.cont = cont
+ return dfs(0)
+ }
+
+ private func dfs(_ i: Int) -> [Int] {
+ if i == cont.count - 1 {
+ return [cont[i], 1]
+ }
+ let next = dfs(i + 1)
+ let a = next[0]
+ let b = next[1]
+ let x = a * cont[i] + b
+ let y = a
+ let g = gcd(x, y)
+ return [x / g, y / g]
+ }
+
+ private func gcd(_ a: Int, _ b: Int) -> Int {
+ return b == 0 ? a : gcd(b, a % b)
+ }
+}
+```
+
diff --git "a/lcp/LCP 02. \345\210\206\345\274\217\345\214\226\347\256\200/Solution.swift" "b/lcp/LCP 02. \345\210\206\345\274\217\345\214\226\347\256\200/Solution.swift"
new file mode 100644
index 0000000000000..90097779be77a
--- /dev/null
+++ "b/lcp/LCP 02. \345\210\206\345\274\217\345\214\226\347\256\200/Solution.swift"
@@ -0,0 +1,25 @@
+class Solution {
+ private var cont: [Int] = []
+
+ func fraction(_ cont: [Int]) -> [Int] {
+ self.cont = cont
+ return dfs(0)
+ }
+
+ private func dfs(_ i: Int) -> [Int] {
+ if i == cont.count - 1 {
+ return [cont[i], 1]
+ }
+ let next = dfs(i + 1)
+ let a = next[0]
+ let b = next[1]
+ let x = a * cont[i] + b
+ let y = a
+ let g = gcd(x, y)
+ return [x / g, y / g]
+ }
+
+ private func gcd(_ a: Int, _ b: Int) -> Int {
+ return b == 0 ? a : gcd(b, a % b)
+ }
+}
diff --git "a/lcp/LCP 03. \346\234\272\345\231\250\344\272\272\345\244\247\345\206\222\351\231\251/README.md" "b/lcp/LCP 03. \346\234\272\345\231\250\344\272\272\345\244\247\345\206\222\351\231\251/README.md"
index c086edd1b0eb7..4a10c79e9ade4 100644
--- "a/lcp/LCP 03. \346\234\272\345\231\250\344\272\272\345\244\247\345\206\222\351\231\251/README.md"
+++ "b/lcp/LCP 03. \346\234\272\345\231\250\344\272\272\345\244\247\345\206\222\351\231\251/README.md"
@@ -239,6 +239,49 @@ function robot(command: string, obstacles: number[][], x: number, y: number): bo
}
```
+#### Swift
+
+```swift
+class Solution {
+ func robot(_ command: String, _ obstacles: [[Int]], _ x: Int, _ y: Int) -> Bool {
+ var visited: Set<[Int]> = []
+ var i = 0, j = 0
+ visited.insert([i, j])
+
+ for c in command {
+ if c == "U" {
+ j += 1
+ } else {
+ i += 1
+ }
+ visited.insert([i, j])
+ }
+
+ func canReach(_ targetX: Int, _ targetY: Int) -> Bool {
+ let k = min(targetX / i, targetY / j)
+ return visited.contains([targetX - k * i, targetY - k * j])
+ }
+
+ if !canReach(x, y) {
+ return false
+ }
+
+ for obstacle in obstacles {
+ let obstacleX = obstacle[0]
+ let obstacleY = obstacle[1]
+ if obstacleX > x || obstacleY > y {
+ continue
+ }
+ if canReach(obstacleX, obstacleY) {
+ return false
+ }
+ }
+
+ return true
+ }
+}
+```
+
diff --git "a/lcp/LCP 03. \346\234\272\345\231\250\344\272\272\345\244\247\345\206\222\351\231\251/Solution.swift" "b/lcp/LCP 03. \346\234\272\345\231\250\344\272\272\345\244\247\345\206\222\351\231\251/Solution.swift"
new file mode 100644
index 0000000000000..7f8aebe2c5231
--- /dev/null
+++ "b/lcp/LCP 03. \346\234\272\345\231\250\344\272\272\345\244\247\345\206\222\351\231\251/Solution.swift"
@@ -0,0 +1,38 @@
+class Solution {
+ func robot(_ command: String, _ obstacles: [[Int]], _ x: Int, _ y: Int) -> Bool {
+ var visited: Set<[Int]> = []
+ var i = 0, j = 0
+ visited.insert([i, j])
+
+ for c in command {
+ if c == "U" {
+ j += 1
+ } else {
+ i += 1
+ }
+ visited.insert([i, j])
+ }
+
+ func canReach(_ targetX: Int, _ targetY: Int) -> Bool {
+ let k = min(targetX / i, targetY / j)
+ return visited.contains([targetX - k * i, targetY - k * j])
+ }
+
+ if !canReach(x, y) {
+ return false
+ }
+
+ for obstacle in obstacles {
+ let obstacleX = obstacle[0]
+ let obstacleY = obstacle[1]
+ if obstacleX > x || obstacleY > y {
+ continue
+ }
+ if canReach(obstacleX, obstacleY) {
+ return false
+ }
+ }
+
+ return true
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 05. \345\217\221 LeetCoin/README.md" "b/lcp/LCP 05. \345\217\221 LeetCoin/README.md"
index 6402769f54b27..fad40a20eceae 100644
--- "a/lcp/LCP 05. \345\217\221 LeetCoin/README.md"
+++ "b/lcp/LCP 05. \345\217\221 LeetCoin/README.md"
@@ -449,6 +449,144 @@ public:
};
```
+#### Swift
+
+```swift
+class Node {
+ var left: Node?
+ var right: Node?
+ let l: Int
+ let r: Int
+ let mid: Int
+ var v = 0
+ var add = 0
+
+ init(_ l: Int, _ r: Int) {
+ self.l = l
+ self.r = r
+ self.mid = (l + r) >> 1
+ }
+}
+
+class SegmentTree {
+ private var root: Node
+ private let MOD = 1_000_000_007
+
+ init(_ n: Int) {
+ root = Node(1, n)
+ }
+
+ func modify(_ l: Int, _ r: Int, _ v: Int) {
+ modify(l, r, v, root)
+ }
+
+ private func modify(_ l: Int, _ r: Int, _ v: Int, _ node: Node) {
+ if l > r {
+ return
+ }
+ if node.l >= l && node.r <= r {
+ node.v = (node.v + (node.r - node.l + 1) * v) % MOD
+ node.add = (node.add + v) % MOD
+ return
+ }
+ pushdown(node)
+ if l <= node.mid {
+ modify(l, r, v, node.left!)
+ }
+ if r > node.mid {
+ modify(l, r, v, node.right!)
+ }
+ pushup(node)
+ }
+
+ func query(_ l: Int, _ r: Int) -> Int {
+ return query(l, r, root)
+ }
+
+ private func query(_ l: Int, _ r: Int, _ node: Node) -> Int {
+ if l > r {
+ return 0
+ }
+ if node.l >= l && node.r <= r {
+ return node.v
+ }
+ pushdown(node)
+ var v = 0
+ if l <= node.mid {
+ v = (v + query(l, r, node.left!)) % MOD
+ }
+ if r > node.mid {
+ v = (v + query(l, r, node.right!)) % MOD
+ }
+ return v
+ }
+
+ private func pushup(_ node: Node) {
+ node.v = (node.left!.v + node.right!.v) % MOD
+ }
+
+ private func pushdown(_ node: Node) {
+ if node.left == nil {
+ node.left = Node(node.l, node.mid)
+ }
+ if node.right == nil {
+ node.right = Node(node.mid + 1, node.r)
+ }
+ if node.add != 0 {
+ let left = node.left!, right = node.right!
+ left.v = (left.v + (left.r - left.l + 1) * node.add) % MOD
+ right.v = (right.v + (right.r - right.l + 1) * node.add) % MOD
+ left.add = (left.add + node.add) % MOD
+ right.add = (right.add + node.add) % MOD
+ node.add = 0
+ }
+ }
+}
+
+class Solution {
+ private var g: [[Int]] = []
+ private var begin: [Int] = []
+ private var end: [Int] = []
+ private var idx = 1
+
+ func bonus(_ n: Int, _ leadership: [[Int]], _ operations: [[Int]]) -> [Int] {
+ g = Array(repeating: [], count: n + 1)
+ for l in leadership {
+ let (a, b) = (l[0], l[1])
+ g[a].append(b)
+ }
+
+ begin = Array(repeating: 0, count: n + 1)
+ end = Array(repeating: 0, count: n + 1)
+ idx = 1
+ dfs(1)
+
+ var ans: [Int] = []
+ let tree = SegmentTree(n)
+ for op in operations {
+ let (p, v) = (op[0], op[1])
+ if p == 1 {
+ tree.modify(begin[v], begin[v], op[2])
+ } else if p == 2 {
+ tree.modify(begin[v], end[v], op[2])
+ } else if p == 3 {
+ ans.append(tree.query(begin[v], end[v]))
+ }
+ }
+ return ans
+ }
+
+ private func dfs(_ u: Int) {
+ begin[u] = idx
+ for v in g[u] {
+ dfs(v)
+ }
+ end[u] = idx
+ idx += 1
+ }
+}
+```
+
diff --git "a/lcp/LCP 05. \345\217\221 LeetCoin/Solution.swift" "b/lcp/LCP 05. \345\217\221 LeetCoin/Solution.swift"
new file mode 100644
index 0000000000000..52937b2979ad8
--- /dev/null
+++ "b/lcp/LCP 05. \345\217\221 LeetCoin/Solution.swift"
@@ -0,0 +1,133 @@
+class Node {
+ var left: Node?
+ var right: Node?
+ let l: Int
+ let r: Int
+ let mid: Int
+ var v = 0
+ var add = 0
+
+ init(_ l: Int, _ r: Int) {
+ self.l = l
+ self.r = r
+ self.mid = (l + r) >> 1
+ }
+}
+
+class SegmentTree {
+ private var root: Node
+ private let MOD = 1_000_000_007
+
+ init(_ n: Int) {
+ root = Node(1, n)
+ }
+
+ func modify(_ l: Int, _ r: Int, _ v: Int) {
+ modify(l, r, v, root)
+ }
+
+ private func modify(_ l: Int, _ r: Int, _ v: Int, _ node: Node) {
+ if l > r {
+ return
+ }
+ if node.l >= l && node.r <= r {
+ node.v = (node.v + (node.r - node.l + 1) * v) % MOD
+ node.add = (node.add + v) % MOD
+ return
+ }
+ pushdown(node)
+ if l <= node.mid {
+ modify(l, r, v, node.left!)
+ }
+ if r > node.mid {
+ modify(l, r, v, node.right!)
+ }
+ pushup(node)
+ }
+
+ func query(_ l: Int, _ r: Int) -> Int {
+ return query(l, r, root)
+ }
+
+ private func query(_ l: Int, _ r: Int, _ node: Node) -> Int {
+ if l > r {
+ return 0
+ }
+ if node.l >= l && node.r <= r {
+ return node.v
+ }
+ pushdown(node)
+ var v = 0
+ if l <= node.mid {
+ v = (v + query(l, r, node.left!)) % MOD
+ }
+ if r > node.mid {
+ v = (v + query(l, r, node.right!)) % MOD
+ }
+ return v
+ }
+
+ private func pushup(_ node: Node) {
+ node.v = (node.left!.v + node.right!.v) % MOD
+ }
+
+ private func pushdown(_ node: Node) {
+ if node.left == nil {
+ node.left = Node(node.l, node.mid)
+ }
+ if node.right == nil {
+ node.right = Node(node.mid + 1, node.r)
+ }
+ if node.add != 0 {
+ let left = node.left!, right = node.right!
+ left.v = (left.v + (left.r - left.l + 1) * node.add) % MOD
+ right.v = (right.v + (right.r - right.l + 1) * node.add) % MOD
+ left.add = (left.add + node.add) % MOD
+ right.add = (right.add + node.add) % MOD
+ node.add = 0
+ }
+ }
+}
+
+class Solution {
+ private var g: [[Int]] = []
+ private var begin: [Int] = []
+ private var end: [Int] = []
+ private var idx = 1
+
+ func bonus(_ n: Int, _ leadership: [[Int]], _ operations: [[Int]]) -> [Int] {
+ g = Array(repeating: [], count: n + 1)
+ for l in leadership {
+ let (a, b) = (l[0], l[1])
+ g[a].append(b)
+ }
+
+ begin = Array(repeating: 0, count: n + 1)
+ end = Array(repeating: 0, count: n + 1)
+ idx = 1
+ dfs(1)
+
+ var ans: [Int] = []
+ let tree = SegmentTree(n)
+ for op in operations {
+ let (p, v) = (op[0], op[1])
+ if p == 1 {
+ tree.modify(begin[v], begin[v], op[2])
+ } else if p == 2 {
+ tree.modify(begin[v], end[v], op[2])
+ } else if p == 3 {
+ ans.append(tree.query(begin[v], end[v]))
+ }
+ }
+ return ans
+ }
+
+ private func dfs(_ u: Int) {
+ begin[u] = idx
+ for v in g[u] {
+ dfs(v)
+ }
+ end[u] = idx
+ idx += 1
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 06. \346\213\277\347\241\254\345\270\201/README.md" "b/lcp/LCP 06. \346\213\277\347\241\254\345\270\201/README.md"
index a13eeebc8f399..fd1f297a5456f 100644
--- "a/lcp/LCP 06. \346\213\277\347\241\254\345\270\201/README.md"
+++ "b/lcp/LCP 06. \346\213\277\347\241\254\345\270\201/README.md"
@@ -154,6 +154,20 @@ int minCount(int* coins, int coinsSize) {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minCount(_ coins: [Int]) -> Int {
+ var ans = 0
+ for x in coins {
+ ans += (x + 1) >> 1
+ }
+ return ans
+ }
+}
+```
+
diff --git "a/lcp/LCP 06. \346\213\277\347\241\254\345\270\201/Solution.swift" "b/lcp/LCP 06. \346\213\277\347\241\254\345\270\201/Solution.swift"
new file mode 100644
index 0000000000000..9b88a97b003b4
--- /dev/null
+++ "b/lcp/LCP 06. \346\213\277\347\241\254\345\270\201/Solution.swift"
@@ -0,0 +1,9 @@
+class Solution {
+ func minCount(_ coins: [Int]) -> Int {
+ var ans = 0
+ for x in coins {
+ ans += (x + 1) >> 1
+ }
+ return ans
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/README.md" "b/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/README.md"
index 1703fe246c239..f3a58cc62caa7 100644
--- "a/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/README.md"
+++ "b/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/README.md"
@@ -155,6 +155,25 @@ function numWays(n: number, relation: number[][], k: number): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func numWays(_ n: Int, _ relation: [[Int]], _ k: Int) -> Int {
+ var f = Array(repeating: Array(repeating: 0, count: n), count: k + 1)
+ f[0][0] = 1
+
+ for i in 1...k {
+ for r in relation {
+ let a = r[0], b = r[1]
+ f[i][b] += f[i - 1][a]
+ }
+ }
+ return f[k][n - 1]
+ }
+}
+```
+
@@ -255,6 +274,30 @@ function numWays(n: number, relation: number[][], k: number): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func numWays(_ n: Int, _ relation: [[Int]], _ k: Int) -> Int {
+ var f = Array(repeating: 0, count: n)
+ f[0] = 1
+ var steps = k
+
+ while steps > 0 {
+ var g = Array(repeating: 0, count: n)
+ for r in relation {
+ let a = r[0], b = r[1]
+ g[b] += f[a]
+ }
+ f = g
+ steps -= 1
+ }
+
+ return f[n - 1]
+ }
+}
+```
+
diff --git "a/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/Solution.swift" "b/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/Solution.swift"
new file mode 100644
index 0000000000000..c652759ac9adf
--- /dev/null
+++ "b/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/Solution.swift"
@@ -0,0 +1,14 @@
+class Solution {
+ func numWays(_ n: Int, _ relation: [[Int]], _ k: Int) -> Int {
+ var f = Array(repeating: Array(repeating: 0, count: n), count: k + 1)
+ f[0][0] = 1
+
+ for i in 1...k {
+ for r in relation {
+ let a = r[0], b = r[1]
+ f[i][b] += f[i - 1][a]
+ }
+ }
+ return f[k][n - 1]
+ }
+}
diff --git "a/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/Solution2.swift" "b/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/Solution2.swift"
new file mode 100644
index 0000000000000..b62995acf5a39
--- /dev/null
+++ "b/lcp/LCP 07. \344\274\240\351\200\222\344\277\241\346\201\257/Solution2.swift"
@@ -0,0 +1,19 @@
+class Solution {
+ func numWays(_ n: Int, _ relation: [[Int]], _ k: Int) -> Int {
+ var f = Array(repeating: 0, count: n)
+ f[0] = 1
+ var steps = k
+
+ while steps > 0 {
+ var g = Array(repeating: 0, count: n)
+ for r in relation {
+ let a = r[0], b = r[1]
+ g[b] += f[a]
+ }
+ f = g
+ steps -= 1
+ }
+
+ return f[n - 1]
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 08. \345\211\247\346\203\205\350\247\246\345\217\221\346\227\266\351\227\264/README.md" "b/lcp/LCP 08. \345\211\247\346\203\205\350\247\246\345\217\221\346\227\266\351\227\264/README.md"
index 7f3a754caf57c..f29879959c9dc 100644
--- "a/lcp/LCP 08. \345\211\247\346\203\205\350\247\246\345\217\221\346\227\266\351\227\264/README.md"
+++ "b/lcp/LCP 08. \345\211\247\346\203\205\350\247\246\345\217\221\346\227\266\351\227\264/README.md"
@@ -140,6 +140,47 @@ class Solution {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func getTriggerTime(_ increase: [[Int]], _ requirements: [[Int]]) -> [Int] {
+ let m = increase.count, n = requirements.count
+ var s = Array(repeating: [0, 0, 0], count: m + 1)
+
+ for i in 0.. Bool {
+ for i in 0..<3 {
+ if a[i] < b[i] {
+ return false
+ }
+ }
+ return true
+ }
+}
+```
+
diff --git "a/lcp/LCP 08. \345\211\247\346\203\205\350\247\246\345\217\221\346\227\266\351\227\264/Solution.swift" "b/lcp/LCP 08. \345\211\247\346\203\205\350\247\246\345\217\221\346\227\266\351\227\264/Solution.swift"
new file mode 100644
index 0000000000000..1b2606567fd06
--- /dev/null
+++ "b/lcp/LCP 08. \345\211\247\346\203\205\350\247\246\345\217\221\346\227\266\351\227\264/Solution.swift"
@@ -0,0 +1,36 @@
+class Solution {
+ func getTriggerTime(_ increase: [[Int]], _ requirements: [[Int]]) -> [Int] {
+ let m = increase.count, n = requirements.count
+ var s = Array(repeating: [0, 0, 0], count: m + 1)
+
+ for i in 0.. Bool {
+ for i in 0..<3 {
+ if a[i] < b[i] {
+ return false
+ }
+ }
+ return true
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 09. \346\234\200\345\260\217\350\267\263\350\267\203\346\254\241\346\225\260/README.md" "b/lcp/LCP 09. \346\234\200\345\260\217\350\267\263\350\267\203\346\254\241\346\225\260/README.md"
index 570853993406f..02c7840200690 100644
--- "a/lcp/LCP 09. \346\234\200\345\260\217\350\267\263\350\267\203\346\254\241\346\225\260/README.md"
+++ "b/lcp/LCP 09. \346\234\200\345\260\217\350\267\263\350\267\203\346\254\241\346\225\260/README.md"
@@ -178,6 +178,50 @@ func minJump(jump []int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minJump(_ jump: [Int]) -> Int {
+ let n = jump.count
+ var vis = Array(repeating: false, count: n)
+ var queue = [0]
+ vis[0] = true
+ var ans = 0
+ var maxReach = 1
+
+ while !queue.isEmpty {
+ ans += 1
+ let size = queue.count
+
+ for _ in 0..= n {
+ return ans
+ }
+
+ if !vis[forwardJump] {
+ queue.append(forwardJump)
+ vis[forwardJump] = true
+ }
+
+ while maxReach < i {
+ if !vis[maxReach] {
+ queue.append(maxReach)
+ vis[maxReach] = true
+ }
+ maxReach += 1
+ }
+ }
+ }
+
+ return -1
+ }
+}
+```
+
diff --git "a/lcp/LCP 09. \346\234\200\345\260\217\350\267\263\350\267\203\346\254\241\346\225\260/Solution.swift" "b/lcp/LCP 09. \346\234\200\345\260\217\350\267\263\350\267\203\346\254\241\346\225\260/Solution.swift"
new file mode 100644
index 0000000000000..cb4693fc17269
--- /dev/null
+++ "b/lcp/LCP 09. \346\234\200\345\260\217\350\267\263\350\267\203\346\254\241\346\225\260/Solution.swift"
@@ -0,0 +1,39 @@
+class Solution {
+ func minJump(_ jump: [Int]) -> Int {
+ let n = jump.count
+ var vis = Array(repeating: false, count: n)
+ var queue = [0]
+ vis[0] = true
+ var ans = 0
+ var maxReach = 1
+
+ while !queue.isEmpty {
+ ans += 1
+ let size = queue.count
+
+ for _ in 0..= n {
+ return ans
+ }
+
+ if !vis[forwardJump] {
+ queue.append(forwardJump)
+ vis[forwardJump] = true
+ }
+
+ while maxReach < i {
+ if !vis[maxReach] {
+ queue.append(maxReach)
+ vis[maxReach] = true
+ }
+ maxReach += 1
+ }
+ }
+ }
+
+ return -1
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 10. \344\272\214\345\217\211\346\240\221\344\273\273\345\212\241\350\260\203\345\272\246/README.md" "b/lcp/LCP 10. \344\272\214\345\217\211\346\240\221\344\273\273\345\212\241\350\260\203\345\272\246/README.md"
index 217b12783b14e..88a761b550252 100644
--- "a/lcp/LCP 10. \344\272\214\345\217\211\346\240\221\344\273\273\345\212\241\350\260\203\345\272\246/README.md"
+++ "b/lcp/LCP 10. \344\272\214\345\217\211\346\240\221\344\273\273\345\212\241\350\260\203\345\272\246/README.md"
@@ -204,6 +204,43 @@ function minimalExecTime(root: TreeNode | null): number {
}
```
+#### Swift
+
+```swift
+/**
+* public class TreeNode {
+* public var val: Int
+* public var left: TreeNode?
+* public var right: TreeNode?
+* public init() { self.val = 0; self.left = nil; self.right = nil; }
+* public init(_ val: Int) { self.val = val; self.left = nil; self.right = nil; }
+* public init(_ val: Int, _ left: TreeNode?, _ right: TreeNode?) {
+* self.val = val
+* self.left = left
+* self.right = right
+* }
+* }
+*/
+
+class Solution {
+ func minimalExecTime(_ root: TreeNode?) -> Double {
+ return dfs(root)[1]
+ }
+
+ private func dfs(_ root: TreeNode?) -> [Double] {
+ guard let root = root else { return [0.0, 0.0] }
+
+ let left = dfs(root.left)
+ let right = dfs(root.right)
+
+ let sum = left[0] + right[0] + Double(root.val)
+ let time = max(max(left[1], right[1]), (left[0] + right[0]) / 2) + Double(root.val)
+
+ return [sum, time]
+ }
+}
+```
+
diff --git "a/lcp/LCP 10. \344\272\214\345\217\211\346\240\221\344\273\273\345\212\241\350\260\203\345\272\246/Solution.swift" "b/lcp/LCP 10. \344\272\214\345\217\211\346\240\221\344\273\273\345\212\241\350\260\203\345\272\246/Solution.swift"
new file mode 100644
index 0000000000000..d1449cfd0909d
--- /dev/null
+++ "b/lcp/LCP 10. \344\272\214\345\217\211\346\240\221\344\273\273\345\212\241\350\260\203\345\272\246/Solution.swift"
@@ -0,0 +1,32 @@
+/**
+* public class TreeNode {
+* public var val: Int
+* public var left: TreeNode?
+* public var right: TreeNode?
+* public init() { self.val = 0; self.left = nil; self.right = nil; }
+* public init(_ val: Int) { self.val = val; self.left = nil; self.right = nil; }
+* public init(_ val: Int, _ left: TreeNode?, _ right: TreeNode?) {
+* self.val = val
+* self.left = left
+* self.right = right
+* }
+* }
+*/
+
+class Solution {
+ func minimalExecTime(_ root: TreeNode?) -> Double {
+ return dfs(root)[1]
+ }
+
+ private func dfs(_ root: TreeNode?) -> [Double] {
+ guard let root = root else { return [0.0, 0.0] }
+
+ let left = dfs(root.left)
+ let right = dfs(root.right)
+
+ let sum = left[0] + right[0] + Double(root.val)
+ let time = max(max(left[1], right[1]), (left[0] + right[0]) / 2) + Double(root.val)
+
+ return [sum, time]
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 11. \346\234\237\346\234\233\344\270\252\346\225\260\347\273\237\350\256\241/README.md" "b/lcp/LCP 11. \346\234\237\346\234\233\344\270\252\346\225\260\347\273\237\350\256\241/README.md"
index e9159c998fec7..62db68dcdb726 100644
--- "a/lcp/LCP 11. \346\234\237\346\234\233\344\270\252\346\225\260\347\273\237\350\256\241/README.md"
+++ "b/lcp/LCP 11. \346\234\237\346\234\233\344\270\252\346\225\260\347\273\237\350\256\241/README.md"
@@ -125,6 +125,17 @@ function expectNumber(scores: number[]): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func expectNumber(_ scores: [Int]) -> Int {
+ let uniqueScores = Set(scores)
+ return uniqueScores.count
+ }
+}
+```
+
diff --git "a/lcp/LCP 11. \346\234\237\346\234\233\344\270\252\346\225\260\347\273\237\350\256\241/Solution.swift" "b/lcp/LCP 11. \346\234\237\346\234\233\344\270\252\346\225\260\347\273\237\350\256\241/Solution.swift"
new file mode 100644
index 0000000000000..af188317abaf1
--- /dev/null
+++ "b/lcp/LCP 11. \346\234\237\346\234\233\344\270\252\346\225\260\347\273\237\350\256\241/Solution.swift"
@@ -0,0 +1,6 @@
+class Solution {
+ func expectNumber(_ scores: [Int]) -> Int {
+ let uniqueScores = Set(scores)
+ return uniqueScores.count
+ }
+}
diff --git "a/lcp/LCP 12. \345\260\217\345\274\240\345\210\267\351\242\230\350\256\241\345\210\222/README.md" "b/lcp/LCP 12. \345\260\217\345\274\240\345\210\267\351\242\230\350\256\241\345\210\222/README.md"
index 42804b7024b0b..a2da6fe9ec4ef 100644
--- "a/lcp/LCP 12. \345\260\217\345\274\240\345\210\267\351\242\230\350\256\241\345\210\222/README.md"
+++ "b/lcp/LCP 12. \345\260\217\345\274\240\345\210\267\351\242\230\350\256\241\345\210\222/README.md"
@@ -217,6 +217,44 @@ function minTime(time: number[], m: number): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minTime(_ time: [Int], _ m: Int) -> Int {
+ var left = 0
+ var right = time.reduce(0, +)
+
+ while left < right {
+ let mid = (left + right) / 2
+ if check(mid, time, m) {
+ right = mid
+ } else {
+ left = mid + 1
+ }
+ }
+ return left
+ }
+
+ private func check(_ t: Int, _ time: [Int], _ m: Int) -> Bool {
+ var sum = 0
+ var maxTime = 0
+ var days = 1
+
+ for x in time {
+ sum += x
+ maxTime = max(maxTime, x)
+ if sum - maxTime > t {
+ sum = x
+ maxTime = x
+ days += 1
+ }
+ }
+ return days <= m
+ }
+}
+```
+
diff --git "a/lcp/LCP 12. \345\260\217\345\274\240\345\210\267\351\242\230\350\256\241\345\210\222/Solution.swift" "b/lcp/LCP 12. \345\260\217\345\274\240\345\210\267\351\242\230\350\256\241\345\210\222/Solution.swift"
new file mode 100644
index 0000000000000..8213c97c09ac9
--- /dev/null
+++ "b/lcp/LCP 12. \345\260\217\345\274\240\345\210\267\351\242\230\350\256\241\345\210\222/Solution.swift"
@@ -0,0 +1,33 @@
+class Solution {
+ func minTime(_ time: [Int], _ m: Int) -> Int {
+ var left = 0
+ var right = time.reduce(0, +)
+
+ while left < right {
+ let mid = (left + right) / 2
+ if check(mid, time, m) {
+ right = mid
+ } else {
+ left = mid + 1
+ }
+ }
+ return left
+ }
+
+ private func check(_ t: Int, _ time: [Int], _ m: Int) -> Bool {
+ var sum = 0
+ var maxTime = 0
+ var days = 1
+
+ for x in time {
+ sum += x
+ maxTime = max(maxTime, x)
+ if sum - maxTime > t {
+ sum = x
+ maxTime = x
+ days += 1
+ }
+ }
+ return days <= m
+ }
+}
diff --git "a/lcp/LCP 17. \351\200\237\347\256\227\346\234\272\345\231\250\344\272\272/README.md" "b/lcp/LCP 17. \351\200\237\347\256\227\346\234\272\345\231\250\344\272\272/README.md"
index ef691e227f58a..7c8136ef52536 100644
--- "a/lcp/LCP 17. \351\200\237\347\256\227\346\234\272\345\231\250\344\272\272/README.md"
+++ "b/lcp/LCP 17. \351\200\237\347\256\227\346\234\272\345\231\250\344\272\272/README.md"
@@ -118,6 +118,25 @@ func calculate(s string) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func calculate(_ s: String) -> Int {
+ var x = 1
+ var y = 0
+ for c in s {
+ if c == "A" {
+ x = x * 2 + y
+ } else if c == "B" {
+ y = y * 2 + x
+ }
+ }
+ return x + y
+ }
+}
+```
+
diff --git "a/lcp/LCP 17. \351\200\237\347\256\227\346\234\272\345\231\250\344\272\272/Solution.swift" "b/lcp/LCP 17. \351\200\237\347\256\227\346\234\272\345\231\250\344\272\272/Solution.swift"
new file mode 100644
index 0000000000000..d8f69186b0f44
--- /dev/null
+++ "b/lcp/LCP 17. \351\200\237\347\256\227\346\234\272\345\231\250\344\272\272/Solution.swift"
@@ -0,0 +1,14 @@
+class Solution {
+ func calculate(_ s: String) -> Int {
+ var x = 1
+ var y = 0
+ for c in s {
+ if c == "A" {
+ x = x * 2 + y
+ } else if c == "B" {
+ y = y * 2 + x
+ }
+ }
+ return x + y
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 18. \346\227\251\351\244\220\347\273\204\345\220\210/README.md" "b/lcp/LCP 18. \346\227\251\351\244\220\347\273\204\345\220\210/README.md"
index c04eb85dcb523..f57903fb90c8f 100644
--- "a/lcp/LCP 18. \346\227\251\351\244\220\347\273\204\345\220\210/README.md"
+++ "b/lcp/LCP 18. \346\227\251\351\244\220\347\273\204\345\220\210/README.md"
@@ -187,6 +187,37 @@ func breakfastNumber(staple []int, drinks []int, x int) int {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func breakfastNumber(_ staple: [Int], _ drinks: [Int], _ x: Int) -> Int {
+ let mod = 1000000007
+ var result = 0
+ let sortedDrinks = drinks.sorted()
+
+ for s in staple {
+ let remaining = x - s
+ if remaining >= sortedDrinks.first ?? 0 {
+ var left = 0
+ var right = sortedDrinks.count - 1
+
+ while left < right {
+ let mid = (left + right + 1) / 2
+ if sortedDrinks[mid] <= remaining {
+ left = mid
+ } else {
+ right = mid - 1
+ }
+ }
+ result = (result + left + 1) % mod
+ }
+ }
+ return result
+ }
+}
+```
+
diff --git "a/lcp/LCP 18. \346\227\251\351\244\220\347\273\204\345\220\210/Solution.swift" "b/lcp/LCP 18. \346\227\251\351\244\220\347\273\204\345\220\210/Solution.swift"
new file mode 100644
index 0000000000000..f126c14fafca0
--- /dev/null
+++ "b/lcp/LCP 18. \346\227\251\351\244\220\347\273\204\345\220\210/Solution.swift"
@@ -0,0 +1,26 @@
+class Solution {
+ func breakfastNumber(_ staple: [Int], _ drinks: [Int], _ x: Int) -> Int {
+ let mod = 1000000007
+ var result = 0
+ let sortedDrinks = drinks.sorted()
+
+ for s in staple {
+ let remaining = x - s
+ if remaining >= sortedDrinks.first ?? 0 {
+ var left = 0
+ var right = sortedDrinks.count - 1
+
+ while left < right {
+ let mid = (left + right + 1) / 2
+ if sortedDrinks[mid] <= remaining {
+ left = mid
+ } else {
+ right = mid - 1
+ }
+ }
+ result = (result + left + 1) % mod
+ }
+ }
+ return result
+ }
+}
\ No newline at end of file
diff --git "a/lcp/LCP 19. \347\247\213\345\217\266\346\224\266\350\227\217\351\233\206/README.md" "b/lcp/LCP 19. \347\247\213\345\217\266\346\224\266\350\227\217\351\233\206/README.md"
index 5caceb7fe92ad..47753dabb0320 100644
--- "a/lcp/LCP 19. \347\247\213\345\217\266\346\224\266\350\227\217\351\233\206/README.md"
+++ "b/lcp/LCP 19. \347\247\213\345\217\266\346\224\266\350\227\217\351\233\206/README.md"
@@ -200,6 +200,35 @@ function minimumOperations(leaves: string): number {
}
```
+#### Swift
+
+```swift
+class Solution {
+ func minimumOperations(_ leaves: String) -> Int {
+ let n = leaves.count
+ let inf = Int.max / 2
+ var f = Array(repeating: [inf, inf, inf], count: n)
+ let leavesArray = Array(leaves)
+
+ f[0][0] = leavesArray[0] == "r" ? 0 : 1
+
+ for i in 1..
diff --git "a/lcp/LCP 19. \347\247\213\345\217\266\346\224\266\350\227\217\351\233\206/Solution.swift" "b/lcp/LCP 19. \347\247\213\345\217\266\346\224\266\350\227\217\351\233\206/Solution.swift"
new file mode 100644
index 0000000000000..18c6f753b5f39
--- /dev/null
+++ "b/lcp/LCP 19. \347\247\213\345\217\266\346\224\266\350\227\217\351\233\206/Solution.swift"
@@ -0,0 +1,24 @@
+class Solution {
+ func minimumOperations(_ leaves: String) -> Int {
+ let n = leaves.count
+ let inf = Int.max / 2
+ var f = Array(repeating: [inf, inf, inf], count: n)
+ let leavesArray = Array(leaves)
+
+ f[0][0] = leavesArray[0] == "r" ? 0 : 1
+
+ for i in 1.. Int {
+ if k == 0 || k == n * n {
+ return 1
+ }
+
+ func combination(_ n: Int, _ r: Int) -> Int {
+ guard r <= n else { return 0 }
+ if r == 0 || r == n { return 1 }
+ var result = 1
+ for i in 0..
diff --git "a/lcp/LCP 22. \351\273\221\347\231\275\346\226\271\346\240\274\347\224\273/Solution.swift" "b/lcp/LCP 22. \351\273\221\347\231\275\346\226\271\346\240\274\347\224\273/Solution.swift"
new file mode 100644
index 0000000000000..cce487d748515
--- /dev/null
+++ "b/lcp/LCP 22. \351\273\221\347\231\275\346\226\271\346\240\274\347\224\273/Solution.swift"
@@ -0,0 +1,30 @@
+class Solution {
+ func paintingPlan(_ n: Int, _ k: Int) -> Int {
+ if k == 0 || k == n * n {
+ return 1
+ }
+
+ func combination(_ n: Int, _ r: Int) -> Int {
+ guard r <= n else { return 0 }
+ if r == 0 || r == n { return 1 }
+ var result = 1
+ for i in 0..)
+ }
+
+
+ if lowerHeap.count > upperHeap.count + 1 {
+ if let maxLower = lowerHeap.first {
+ lowerHeap.removeFirst()
+ upperHeap.append(maxLower)
+ sumLower -= maxLower
+ sumUpper += maxLower
+ upperHeap.sort()
+ }
+ }
+ }
+
+ func findMedian() -> Int {
+ if lowerHeap.count > upperHeap.count {
+ return lowerHeap.first ?? 0
+ } else if let minUpper = upperHeap.first, let maxLower = lowerHeap.first {
+ return (minUpper + maxLower) / 2
+ }
+ return 0
+ }
+
+ func cal() -> Int {
+ let median = findMedian()
+ var result = (sumUpper - median * upperHeap.count) + (median * lowerHeap.count - sumLower)
+ result %= mod
+ if result < 0 {
+ result += mod
+ }
+ return Int(result)
+ }
+}
+
+class Solution {
+ func numsGame(_ nums: [Int]) -> [Int] {
+ let n = nums.count
+ var result = [Int]()
+ let finder = MedianFinder()
+
+ for i in 0..