|
15 | 15 | > 输出:`1`
|
16 | 16 | >
|
17 | 17 | > 解释:4 个主题空间中,只有 1 个不与走廊相邻,面积为 1。
|
18 |
| -> 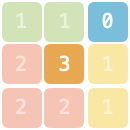 |
| 18 | +> 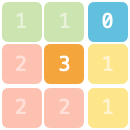 |
19 | 19 |
|
20 | 20 | **示例 2:**
|
21 | 21 |
|
|
24 | 24 | > 输出:`3`
|
25 | 25 | >
|
26 | 26 | > 解释:8 个主题空间中,有 5 个不与走廊相邻,面积分别为 3、1、1、1、2,最大面积为 3。
|
27 |
| ->  |
| 27 | +> 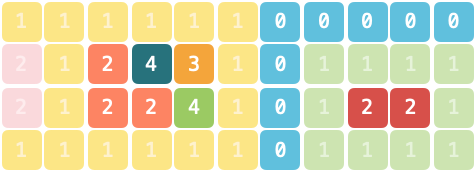 |
28 | 28 |
|
29 | 29 | **提示:**
|
30 | 30 |
|
|
36 | 36 |
|
37 | 37 | <!-- 这里可写通用的实现逻辑 -->
|
38 | 38 |
|
| 39 | +并查集。 |
| 40 | + |
| 41 | +并查集模板: |
| 42 | + |
| 43 | +模板 1——朴素并查集: |
| 44 | + |
| 45 | +```python |
| 46 | +# 初始化,p存储每个点的父节点 |
| 47 | +p = list(range(n)) |
| 48 | + |
| 49 | +# 返回x的祖宗节点 |
| 50 | +def find(x): |
| 51 | + if p[x] != x: |
| 52 | + # 路径压缩 |
| 53 | + p[x] = find(p[x]) |
| 54 | + return p[x] |
| 55 | + |
| 56 | +# 合并a和b所在的两个集合 |
| 57 | +p[find(a)] = find(b) |
| 58 | +``` |
| 59 | + |
| 60 | +模板 2——维护 size 的并查集: |
| 61 | + |
| 62 | +```python |
| 63 | +# 初始化,p存储每个点的父节点,size只有当节点是祖宗节点时才有意义,表示祖宗节点所在集合中,点的数量 |
| 64 | +p = list(range(n)) |
| 65 | +size = [1] * n |
| 66 | + |
| 67 | +# 返回x的祖宗节点 |
| 68 | +def find(x): |
| 69 | + if p[x] != x: |
| 70 | + # 路径压缩 |
| 71 | + p[x] = find(p[x]) |
| 72 | + return p[x] |
| 73 | + |
| 74 | +# 合并a和b所在的两个集合 |
| 75 | +if find(a) != find(b): |
| 76 | + size[find(b)] += size[find(a)] |
| 77 | + p[find(a)] = find(b) |
| 78 | +``` |
| 79 | + |
| 80 | +模板 3——维护到祖宗节点距离的并查集: |
| 81 | + |
| 82 | +```python |
| 83 | +# 初始化,p存储每个点的父节点,d[x]存储x到p[x]的距离 |
| 84 | +p = list(range(n)) |
| 85 | +d = [0] * n |
| 86 | + |
| 87 | +# 返回x的祖宗节点 |
| 88 | +def find(x): |
| 89 | + if p[x] != x: |
| 90 | + t = find(p[x]) |
| 91 | + d[x] += d[p[x]] |
| 92 | + p[x] = t |
| 93 | + return p[x] |
| 94 | + |
| 95 | +# 合并a和b所在的两个集合 |
| 96 | +p[find(a)] = find(b) |
| 97 | +d[find(a)] = distance |
| 98 | +``` |
| 99 | + |
39 | 100 | <!-- tabs:start -->
|
40 | 101 |
|
41 | 102 | ### **Python3**
|
42 | 103 |
|
43 | 104 | <!-- 这里可写当前语言的特殊实现逻辑 -->
|
44 | 105 |
|
45 | 106 | ```python
|
46 |
| - |
| 107 | +class Solution: |
| 108 | + def largestArea(self, grid: List[str]) -> int: |
| 109 | + m, n = len(grid), len(grid[0]) |
| 110 | + p = list(range(m * n + 1)) |
| 111 | + |
| 112 | + def find(x): |
| 113 | + if p[x] != x: |
| 114 | + p[x] = find(p[x]) |
| 115 | + return p[x] |
| 116 | + |
| 117 | + for i in range(m): |
| 118 | + for j in range(n): |
| 119 | + if i == 0 or i == m - 1 or j == 0 or j == n - 1 or grid[i][j] == '0': |
| 120 | + p[find(i * n + j)] = find(m * n) |
| 121 | + else: |
| 122 | + for x, y in [(-1, 0), (1, 0), (0, -1), (0, 1)]: |
| 123 | + if grid[i + x][j + y] == '0' or grid[i][j] == grid[i + x][j + y]: |
| 124 | + p[find(i * n + j)] = find((i + x) * n + j + y) |
| 125 | + |
| 126 | + mp = collections.defaultdict(int) |
| 127 | + res = 0 |
| 128 | + for i in range(m): |
| 129 | + for j in range(n): |
| 130 | + root = find(i * n + j) |
| 131 | + if root != find(m * n): |
| 132 | + mp[root] += 1 |
| 133 | + res = max(res, mp[root]) |
| 134 | + return res |
47 | 135 | ```
|
48 | 136 |
|
49 | 137 | ### **Java**
|
50 | 138 |
|
51 | 139 | <!-- 这里可写当前语言的特殊实现逻辑 -->
|
52 | 140 |
|
53 | 141 | ```java
|
54 |
| - |
| 142 | +class Solution { |
| 143 | + private int[] p; |
| 144 | + private int[][] dirs = new int[][]{{0, -1}, {0, 1}, {1, 0}, {-1, 0}}; |
| 145 | + |
| 146 | + public int largestArea(String[] grid) { |
| 147 | + int m = grid.length, n = grid[0].length(); |
| 148 | + p = new int[m * n + 1]; |
| 149 | + for (int i = 0; i < p.length; ++i) { |
| 150 | + p[i] = i; |
| 151 | + } |
| 152 | + for (int i = 0; i < m; ++i) { |
| 153 | + for (int j = 0; j < n; ++j) { |
| 154 | + if (i == 0 || i == m - 1 || j == 0 || j == n - 1 || grid[i].charAt(j) == '0') { |
| 155 | + p[find(i * n + j)] = find(m * n); |
| 156 | + } else { |
| 157 | + for (int[] e : dirs) { |
| 158 | + if (grid[i + e[0]].charAt(j + e[1]) == '0' || grid[i].charAt(j) == grid[i + e[0]].charAt(j + e[1])) { |
| 159 | + p[find(i * n + j)] = find((i + e[0]) * n + j + e[1]); |
| 160 | + } |
| 161 | + } |
| 162 | + } |
| 163 | + } |
| 164 | + } |
| 165 | + Map<Integer, Integer> mp = new HashMap<>(); |
| 166 | + int res = 0; |
| 167 | + for (int i = 0; i < m; ++i) { |
| 168 | + for (int j = 0; j < n; ++j) { |
| 169 | + int root = find(i * n + j); |
| 170 | + if (root != find(m * n)) { |
| 171 | + mp.put(root, mp.getOrDefault(root, 0) + 1); |
| 172 | + res = Math.max(res, mp.get(root)); |
| 173 | + } |
| 174 | + } |
| 175 | + } |
| 176 | + return res; |
| 177 | + } |
| 178 | + |
| 179 | + private int find(int x) { |
| 180 | + if (p[x] != x) { |
| 181 | + p[x] = find(p[x]); |
| 182 | + } |
| 183 | + return p[x]; |
| 184 | + } |
| 185 | +} |
55 | 186 | ```
|
56 | 187 |
|
57 | 188 | ### **...**
|
|
0 commit comments