|
| 1 | +周末的时候分享了一个技术session,讲到了@RestController 和 @Controller,当时没有太讲清楚,因为 team 里很多同事之前不是做 Java的,所以对这两个东西不太熟悉,于是写了篇文章整理了一下。 |
| 2 | + |
| 3 | +## @RestController vs @Controller |
| 4 | + |
| 5 | +### Controller 返回一个页面 |
| 6 | + |
| 7 | +单独使用 `@Controller` 不加 `@ResponseBody`的话一般使用在要返回一个视图的情况,这种情况属于比较传统的Spring MVC 的应用,对应于前后端不分离的情况。 |
| 8 | + |
| 9 | + |
| 10 | + |
| 11 | +### @RestController 返回JSON 或 XML 形式数据 |
| 12 | + |
| 13 | +但`@RestController`只返回对象,对象数据直接以 JSON 或 XML 形式写入 HTTP 响应(Response)中,这种情况属于 RESTful Web服务,这也是目前日常开发所接触的最常用的情况(前后端分离)。 |
| 14 | + |
| 15 | +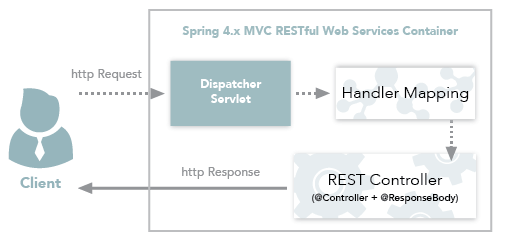 |
| 16 | + |
| 17 | +### @Controller +@ResponseBody 返回JSON 或 XML 形式数据 |
| 18 | + |
| 19 | +如果你需要在Spring4之前开发 RESTful Web服务的话,你需要使用`@Controller` 并结合`@ResponseBody`注解,也就是说`@Controller` +`@ResponseBody`= `@RestController`(Spring 4 之后新加的注解)。 |
| 20 | + |
| 21 | +> `@ResponseBody` 注解的作用是将 `Controller` 的方法返回的对象通过适当的转换器转换为指定的格式之后,写入到HTTP 响应(Response)对象的 body 中,通常用来返回 JSON 或者 XML 数据,返回 JSON 数据的情况比较多。 |
| 22 | +
|
| 23 | + |
| 24 | + |
| 25 | +Reference: |
| 26 | + |
| 27 | +- https://dzone.com/articles/spring-framework-restcontroller-vs-controller(图片来源) |
| 28 | +- https://javarevisited.blogspot.com/2017/08/difference-between-restcontroller-and-controller-annotations-spring-mvc-rest.html?m=1 |
| 29 | + |
| 30 | +### 示例1: @Controller 返回一个页面 |
| 31 | + |
| 32 | +当我们需要直接在后端返回一个页面的时候,Spring 推荐使用 Thymeleaf 模板引擎。Spring MVC中`@Controller`中的方法可以直接返回模板名称,接下来 Thymeleaf 模板引擎会自动进行渲染,模板中的表达式支持Spring表达式语言(Spring EL)。**如果需要用到 Thymeleaf 模板引擎,注意添加依赖!不然会报错。** |
| 33 | + |
| 34 | +Gradle: |
| 35 | + |
| 36 | +```groovy |
| 37 | + compile 'org.springframework.boot:spring-boot-starter-thymeleaf' |
| 38 | +``` |
| 39 | + |
| 40 | +Maven: |
| 41 | + |
| 42 | +```xml |
| 43 | +<dependency> |
| 44 | + <groupId>org.springframework.boot</groupId> |
| 45 | + <artifactId>spring-boot-starter-thymeleaf</artifactId> |
| 46 | +</dependency> |
| 47 | +``` |
| 48 | + |
| 49 | +`src/main/java/com/example/demo/controller/HelloController.java` |
| 50 | + |
| 51 | +```java |
| 52 | +@Controller |
| 53 | +public class HelloController { |
| 54 | + @GetMapping("/hello") |
| 55 | + public String greeting(@RequestParam(name = "name", required = false, defaultValue = "World") String name, Model model) { |
| 56 | + model.addAttribute("name", name); |
| 57 | + return "hello"; |
| 58 | + } |
| 59 | +} |
| 60 | +``` |
| 61 | +`src/main/resources/templates/hello.html` |
| 62 | + |
| 63 | +Spring 会去 resources 目录下 templates 目录下找,所以建议把页面放在 resources/templates 目录下 |
| 64 | + |
| 65 | +```html |
| 66 | +<!DOCTYPE HTML> |
| 67 | +<html xmlns:th="http://www.thymeleaf.org"> |
| 68 | +<head> |
| 69 | + <title>Getting Started: Serving Web Content</title> |
| 70 | + <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"/> |
| 71 | +</head> |
| 72 | +<body> |
| 73 | +<p th:text="'Hello, ' + ${name} + '!'"/> |
| 74 | +</body> |
| 75 | +</html> |
| 76 | +``` |
| 77 | + |
| 78 | +访问:http://localhost:8999/hello?name=team-c ,你将看到下面的内容 |
| 79 | + |
| 80 | +``` |
| 81 | +Hello, team-c! |
| 82 | +``` |
| 83 | + |
| 84 | +如果要对页面在templates目录下的hello文件夹中的话,返回页面的时候像下面这样写就可以了。 |
| 85 | + |
| 86 | +`src/main/resources/templates/hello/hello.html` |
| 87 | + |
| 88 | +```java |
| 89 | + return "hello/hello"; |
| 90 | +``` |
| 91 | + |
| 92 | +### 示例2: @Controller+@ResponseBody 返回 JSON 格式数据 |
| 93 | + |
| 94 | +**SpringBoot 默认集成了 jackson ,对于此需求你不需要添加任何相关依赖。** |
| 95 | + |
| 96 | +`src/main/java/com/example/demo/controller/Person.java` |
| 97 | + |
| 98 | +```java |
| 99 | +public class Person { |
| 100 | + private String name; |
| 101 | + private Integer age; |
| 102 | + ...... |
| 103 | + 省略getter/setter ,有参和无参的construtor方法 |
| 104 | +} |
| 105 | + |
| 106 | +``` |
| 107 | + |
| 108 | +`src/main/java/com/example/demo/controller/HelloController.java` |
| 109 | + |
| 110 | +```java |
| 111 | +@Controller |
| 112 | +public class HelloController { |
| 113 | + @PostMapping("/hello") |
| 114 | + @ResponseBody |
| 115 | + public Person greeting(@RequestBody Person person) { |
| 116 | + return person; |
| 117 | + } |
| 118 | + |
| 119 | +} |
| 120 | +``` |
| 121 | + |
| 122 | +使用 post 请求访问 http://localhost:8080/hello ,body 中附带以下参数,后端会以json 格式将 person 对象返回。 |
| 123 | + |
| 124 | +```json |
| 125 | +{ |
| 126 | + "name": "teamc", |
| 127 | + "age": 1 |
| 128 | +} |
| 129 | +``` |
| 130 | + |
| 131 | +### 示例3: @RestController 返回 JSON 格式数据 |
| 132 | + |
| 133 | +只需要将`HelloController`改为如下形式: |
| 134 | + |
| 135 | +```java |
| 136 | +@RestController |
| 137 | +public class HelloController { |
| 138 | + @PostMapping("/hello") |
| 139 | + public Person greeting(@RequestBody Person person) { |
| 140 | + return person; |
| 141 | + } |
| 142 | + |
| 143 | +} |
| 144 | +``` |
| 145 | + |
0 commit comments