|
6 | 6 | #include "PowerManagement.h"
|
7 | 7 |
|
8 | 8 | Battery battery;
|
9 |
| -Board board; |
10 | 9 | Charger charger;
|
| 10 | +Board board; |
11 | 11 |
|
12 | 12 | void setup(){
|
| 13 | + battery.begin(); |
| 14 | + charger.begin(); |
| 15 | + board.begin(); |
13 | 16 | /* Rest of your setup() code */
|
14 | 17 | }
|
15 | 18 | ```
|
16 | 19 |
|
17 | 20 |
|
18 | 21 | ## Battery
|
19 |
| -The battery object contains methods to read battery usage and health metrics. You can get current and average values for voltage, percentage, current and time as well as an estimated of the time left to charge completely and time left to discharge. |
| 22 | +The Battery class in the PowerManagement library provides a comprehensive set of tools for monitoring and managing the health and usage of your battery. This includes real-time data on voltage, current, power, temperature, and overall battery capacity, enabling you to optimize your application for better energy efficiency and battery longevity. |
20 | 23 |
|
21 |
| -```cpp |
22 |
| -Serial.print("* Voltage: "); |
23 |
| -Serial.println(String(battery.readVoltageAvg()) + "mV"); |
| 24 | +### Voltage Monitoring |
| 25 | + |
| 26 | +| Method | Data Type | Description | |
| 27 | +|:-------------------------|:------------|:-------------------------------------------------| |
| 28 | +| battery.voltage() | float | Read the current voltage of the battery. | |
| 29 | +| battery.averageVoltage() | float | Get the average voltage. | |
| 30 | +| battery.minimumVoltage() | float | Access the minimum voltage since the last reset. | |
| 31 | +| battery.maximumVoltage() | float | Access the maximum voltage since the last reset. | |
| 32 | + |
| 33 | +### Current Monitoring |
| 34 | + |
| 35 | +| Method | Data Type | Description | |
| 36 | +|:-------------------------|:------------|:-------------------------------------------------| |
| 37 | +| battery.current() | int16_t | Measure the current flow from the battery. | |
| 38 | +| battery.averageCurrent() | int16_t | Obtain the average current. | |
| 39 | +| battery.minimumCurrent() | int16_t | Access the minimum current since the last reset. | |
| 40 | +| battery.maximumCurrent() | int16_t | Access the maximum current since the last reset. | |
| 41 | + |
| 42 | +### Power Monitoring |
| 43 | + |
| 44 | +| Method | Data Type | Description | |
| 45 | +|:-----------------------|:------------|:------------------------------------------------------| |
| 46 | +| battery.power() | int16_t | Calculate the current power usage in milliwatts (mW). | |
| 47 | +| battery.averagePower() | int16_t | Get the average power usage in milliwatts (mW). | |
24 | 48 |
|
25 |
| -Serial.print("* Current: "); |
26 |
| -Serial.println(String(battery.readCurrent()) + "mA"); |
| 49 | +### Temperature Monitoring |
27 | 50 |
|
28 |
| -Serial.print("* Percentage: "); |
29 |
| -Serial.println(String(battery.readPercentage()) + "%"); |
| 51 | +| Method | Data Type | Description | |
| 52 | +|:-------------------------------------|:------------|:---------------------------------------------------------| |
| 53 | +| battery.internalTemperature() | uint8_t | Read the internal temperature of the battery gauge chip. | |
| 54 | +| battery.averageInternalTemperature() | uint8_t | Obtain the average internal temperature. | |
30 | 55 |
|
31 |
| -Serial.print("* Remaining Capacity: "); |
32 |
| -Serial.println(String(battery.readRemainingCapacity()) + "mAh"); |
| 56 | +### Capacity and State of Charge |
33 | 57 |
|
34 |
| -Serial.print("* Temperature: "); |
35 |
| -Serial.println(String(battery.readTempAvg())); |
| 58 | +| Method | Data Type | Description | |
| 59 | +|:----------------------------|:------------|:------------------------------------------------------------| |
| 60 | +| battery.remainingCapacity() | uint16_t | Monitor the battery's remaining capacity in mAh. | |
| 61 | +| battery.percentage() | uint8_t | Get the battery's state of charge as a percentage (0-100%). | |
36 | 62 |
|
37 |
| -Serial.print("* Time-to-full: "); |
38 |
| -Serial.println(String(battery.readTimeToFull()) + "s"); |
| 63 | +### Time Estimates |
39 | 64 |
|
40 |
| -Serial.print("* Time-to-empty: "); |
41 |
| -Serial.println(String(battery.readTimeToEmpty()) + "s"); |
| 65 | +| Method | Data Type | Description | |
| 66 | +|:----------------------|:------------|:------------------------------------------------------| |
| 67 | +| battery.timeToEmpty() | int32_t | Estimate the time until the battery is empty. | |
| 68 | +| battery.timeToFull() | int32_t | Estimate the time until the battery is fully charged. | |
42 | 69 |
|
| 70 | +### Configuring Battery Characteristics |
| 71 | + |
| 72 | +To ensure accurate readings and effective battery management, you can configure the `BatteryCharacteristics` struct to match the specific attributes of your battery: |
| 73 | + |
| 74 | +```cpp |
| 75 | +BatteryCharacteristics characteristics = BatteryCharacteristics(); |
| 76 | + |
| 77 | +characteristics.capacity = 200; // Set the battery's capacity in mAh |
| 78 | +characteristics.emptyVoltage = 3.3f; // Voltage at which the battery is considered empty |
| 79 | +characteristics.chargeVoltage = 4.2f; // Voltage at which the battery is charged |
| 80 | +characteristics.endOfChargeCurrent = 50; // End of charge current in mA |
| 81 | +characteristics.ntcResistor = NTCResistor::Resistor10K; // Set NTC resistor value (10K or 100K Ohm) |
| 82 | +characteristics.recoveryVoltage = 3.88f; // Voltage to reset empty detection |
43 | 83 | ```
|
44 | 84 |
|
| 85 | +This configuration ensures that the Battery class operates with parameters that match your battery’s specifications, providing more accurate and reliable monitoring and management. |
| 86 | + |
| 87 | + |
45 | 88 | ## Charger
|
46 | 89 | Charging a LiPo battery is done in three stages. This library allows you to monitor what charging stage we are in as well as control some of the chagring parameters.
|
47 | 90 |
|
@@ -242,9 +285,8 @@ Here's an overview of the reduction in power usage that you can expect from this
|
242 | 285 | 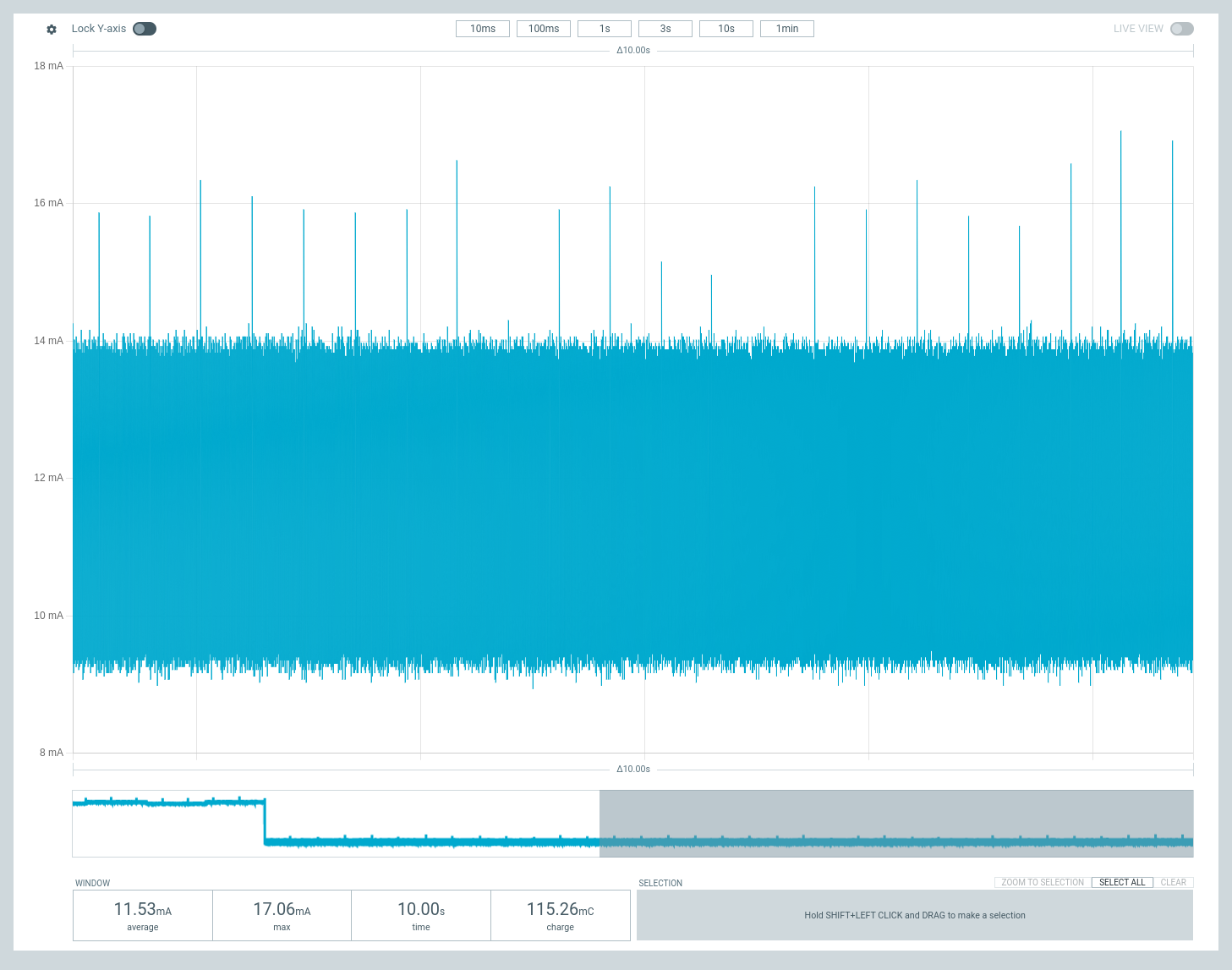
|
243 | 286 |
|
244 | 287 |
|
245 |
| - |
246 |
| -### Portenta H7/Nicla Vison Low Power |
247 |
| -When utilizing Mbed with STM32-based microcontrollers such as the Portenta H7 and Nicla Vision, the approach to managing sleep modes exhibits some unique characteristics. Mbed is designed to transition the board to a sleep-like state—akin to the Sleep Mode found on the Portenta C33—whenever the system is not actively processing tasks. This energy-saving feature can be activated by invoking the `board.enableSleepWhenIdle()` method within your code. |
| 288 | +### Portenta H7 Low Power |
| 289 | +When utilizing Mbed with STM32-based microcontrollers such as the Portenta H7, the approach to managing sleep modes exhibits some unique characteristics. Mbed is designed to transition the board to a sleep-like state—akin to the Sleep Mode found on the Portenta C33—whenever the system is not actively processing tasks. This energy-saving feature can be activated by invoking the `board.enableSleepWhenIdle()` method within your code. |
248 | 290 |
|
249 | 291 | However, initiating this command doesn't guarantee automatic entry into sleep mode due to the presence of Sleep Locks. These locks act as safeguards, preventing the system from sleeping under certain conditions to ensure ongoing operations remain uninterrupted. Common peripherals, such as the USB Stack, may engage a sleep lock, effectively keeping the board awake even during periods of apparent inactivity. This behavior underscores how the effectiveness of sleep mode is closely linked to the specific operations and configurations defined in your sketch.
|
250 | 292 |
|
|
0 commit comments