|
| 1 | +# Java Tutorial: While Loops in Java |
| 2 | +- In programming languages, loops are used to execute a particular statement/set of instructions again and again. |
| 3 | +- The execution of the loop starts when some conditions become true. |
| 4 | +- For example, print 1 to 1000, print multiplication table of 7, etc. |
| 5 | +- Loops make it easy for us to tell the computer that a given set of instructions need to be executed repeatedly. |
| 6 | + |
| 7 | +### Types of Loops : |
| 8 | +- Primarily, there are three types of loops in Java: |
| 9 | +- While loop |
| 10 | +- do-while loop |
| 11 | +- for loop |
| 12 | +- Let's look into these, one by one. |
| 13 | + |
| 14 | +### While loops : |
| 15 | +- The while loop in Java is used when we need to execute a block of code again and again based on a given boolean condition. |
| 16 | +- Use a while loop if the exact number of iterations is not known. |
| 17 | +- If the condition never becomes false, the while loop keeps getting executed. Such a loop is known as an infinite loop. |
| 18 | +``` |
| 19 | +/* |
| 20 | +while (Boolean condition) |
| 21 | +
|
| 22 | +{ |
| 23 | +
|
| 24 | + // Statements -> This keeps executing as long as the condition is true. |
| 25 | +
|
| 26 | +} |
| 27 | +*/ |
| 28 | +``` |
| 29 | +- Example |
| 30 | +``` |
| 31 | +int i=10; |
| 32 | +while(i>0){ |
| 33 | +System.out.println(i); |
| 34 | +i--; |
| 35 | +} |
| 36 | +``` |
| 37 | + |
| 38 | +### Flow control of while loop : |
| 39 | + |
| 40 | +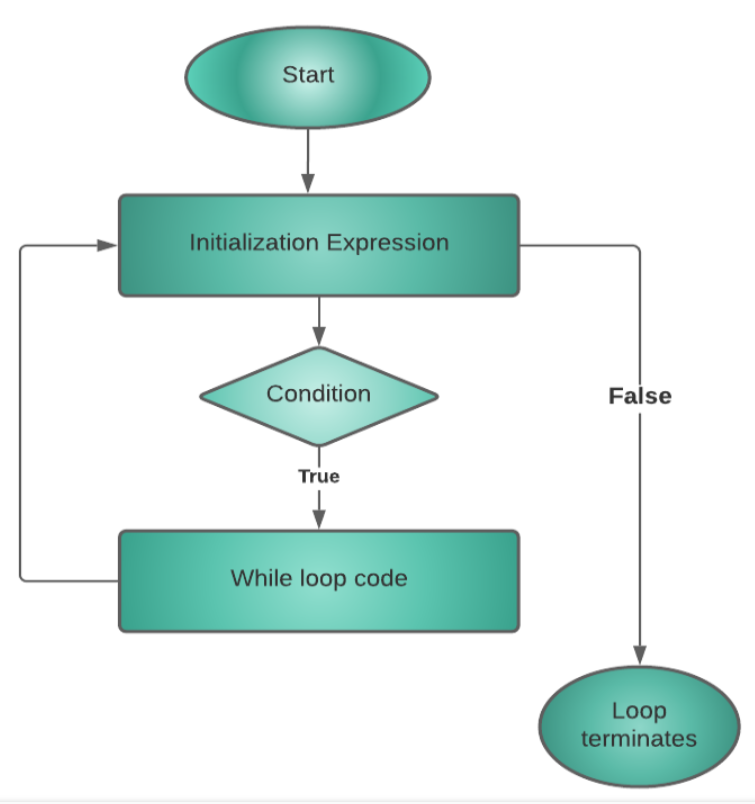 |
| 41 | + |
| 42 | +**Quick Quiz:** Write a program to print natural numbers from 100 to 200. |
| 43 | + |
| 44 | +### Code as described in the video : |
| 45 | + |
| 46 | +``` |
| 47 | +public class cwh_21_ch5_loops { |
| 48 | + public static void main(String[] args) { |
| 49 | + System.out.println(1); |
| 50 | + System.out.println(2); |
| 51 | + System.out.println(3); |
| 52 | +
|
| 53 | + System.out.println("Using Loops:"); |
| 54 | + int i = 100; |
| 55 | + while(i<=200){ |
| 56 | + System.out.println(i); |
| 57 | + i++; |
| 58 | + } |
| 59 | + System.out.println("Finish Running While Loop!"); |
| 60 | +
|
| 61 | +// while(true){ |
| 62 | +// System.out.println("I am an infinite while loop!"); |
| 63 | +// } |
| 64 | + } |
| 65 | +} |
| 66 | +``` |
| 67 | + |
| 68 | +- This is all for this tutorial, and we will discuss the do-while loop in the next tutorial. |
| 69 | + |
| 70 | +**Handwritten Notes: [Click to Download](https://api.codewithharry.com/media/videoSeriesFiles/courseFiles/java-tutorials-for-beginners-21/Chapter_5.pdf)** |
| 71 | + |
| 72 | +**Ultimate Java Cheatsheet: [Click To Download](https://api.codewithharry.com/media/videoSeriesFiles/courseFiles/java-tutorials-for-beginners-21/UltimateJavaCheatSheet.pdf)** |
0 commit comments